Modernizing Code: 7 Essential NetBeans Hints You Need
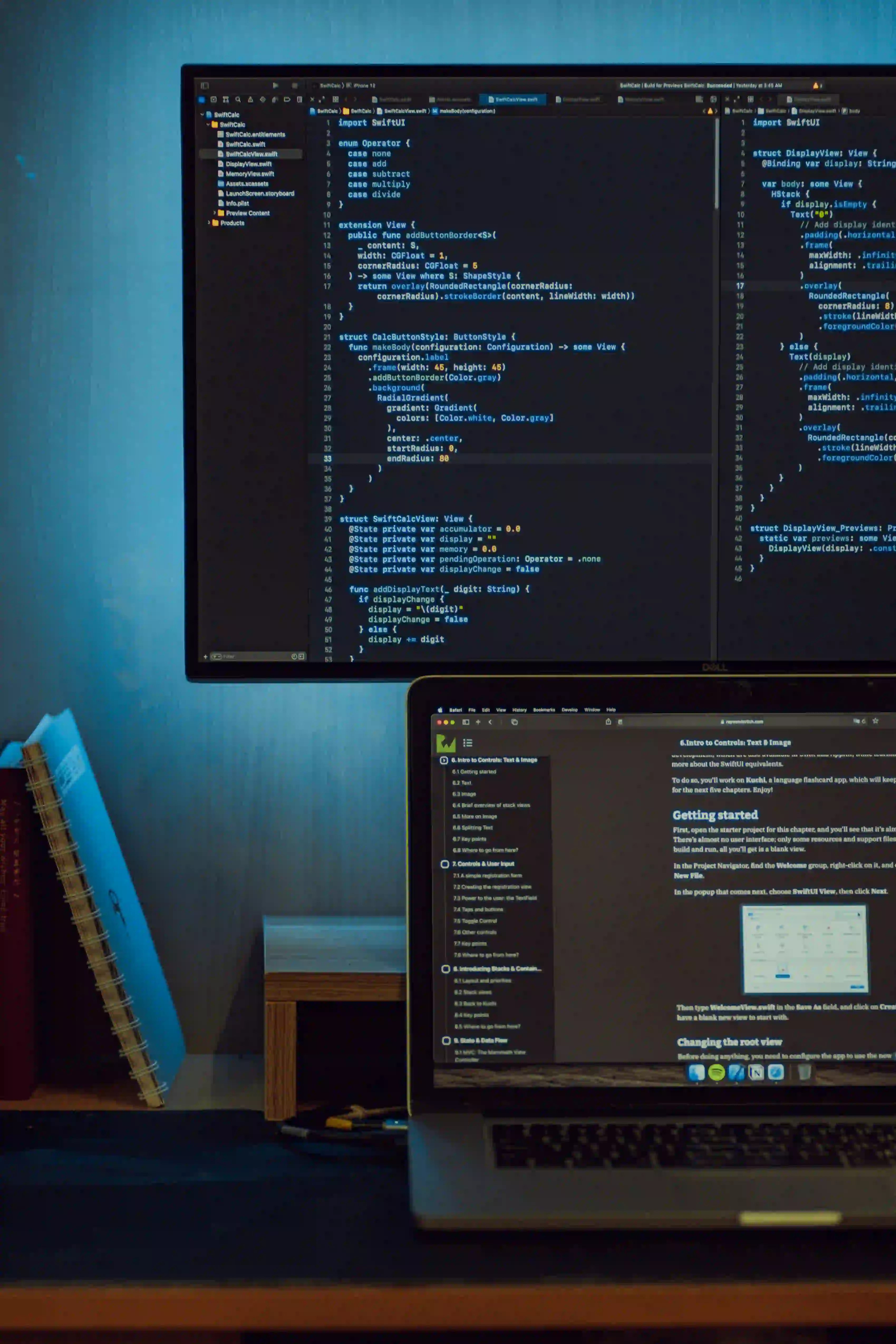
Modernizing Code: 7 Essential NetBeans Hints You Need
In the fast-paced world of software development, keeping up with the latest technologies and practices is crucial for maximizing efficiency and code quality. NetBeans, an open-source Integrated Development Environment (IDE) for Java, provides various tools and hints to help developers refine and modernize their code.
This blog post presents seven essential hints and tips you can use in NetBeans to enhance your Java coding practices.
1. Embrace Code Formatting
Proper formatting makes your code more readable and easier to maintain. In NetBeans, you can automatically format your code by selecting the code block you want to format and pressing Alt + Shift + F
. This functionality can be crucial when working in teams, as consistent formatting improves collaboration.
Code Example
public class FormattingExample {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Using the automatic formatter will align brackets, indentations, and other formatting elements to follow Java conventions. Such visual clarity reduces the cognitive load on developers, making it easier to focus on logic rather than structure.
2. Discover and Remove Dead Code
Dead code refers to portions of your program that are never executed. NetBeans includes hints that can help you identify and eliminate these redundant sections. Highlighting unreachable code enhances performance and readability.
How to Remove Dead Code
- Enable Warnings: Go to
Tools > Options > Editor > Hints
. - Enable "Dead Code" under the "Java" category.
- Follow the hints in your code and delete unnecessary sections.
Code Example
public class DeadCodeExample {
public static void main(String[] args) {
System.out.println("You should see this message.");
// This line is never reached and can be removed
return;
System.out.println("This will never execute.");
}
}
Removing the unreachable code above will keep your application efficient and maintainable.
3. Leverage Code Templates
Code templates speed up coding by allowing you to insert common code structures easily. Using the tab key, you can expand templates into full code snippets. This can greatly enhance productivity, especially when writing repetitive structures.
Create a Template
- Navigate to
Tools > Options > Editor > Code Templates
. - Create a new template, such as a commonly used method structure.
Code Example
// Template: forloop
for (int i = 0; i < /*condition*/; i++) {
/*body*/
}
When you start typing the template name, you will see it can expand into the given format. By utilizing code templates, you reduce typing time and potential human errors.
4. Use Refactoring Tools
Refactoring is crucial for keeping your codebase clean without changing its functionality. NetBeans provides comprehensive refactoring options such as renaming, extracting methods, and changing method signatures, allowing you to improve structure without rewriting code from scratch.
Refactoring Example
To rename a variable:
- Right-click the variable.
- Select Refactor > Rename.
- Enter the new name and NetBeans will handle all references.
This action can drastically reduce the chances of error due to missing variable substitutions in your code.
5. Incorporate Annotations
Annotations provide metadata to your code, improving both functionality and clarity. You can use them to indicate which methods are part of a framework (like Spring), handling dependencies and connections seamlessly.
Code Example
import javax.annotation.PostConstruct;
public class MyComponent {
@PostConstruct
public void init() {
// Initialization code...
}
}
Annotations like @PostConstruct
make your intentions clear to other developers, allowing different parts of the codebase to interact more effectively. Read more on Java annotations here.
6. Utilize Version Control Integration
NetBeans integrates well with version control systems, like Git. Utilizing version control ensures that changes are tracked and enables collaborative work with other developers seamlessly.
Key Features
- Version History: View changes over time.
- Branch Management: Create and manage branches to experiment without affecting the main codebase.
Simple Git Commands in NetBeans
- Commit changes:
Ctrl + K
- Pull updates:
Ctrl + Shift + V
Integrating version control helps maintain a robust workflow and prevents data loss due to errors.
7. Optimize for Performance Using Profiler
NetBeans includes a built-in profiler that allows you to analyze performance bottlenecks. Profiling provides insights into memory usage and CPU consumption, helping you make data-driven decisions to improve your application.
How to Profile in NetBeans
- Open your project.
- Navigate to
Profile > Profile Project
. - Use the profiler to trace memory leaks or slow code sections.
Identifying performance issues early can save hours of debugging time and enhance user experience.
The Bottom Line
Modernizing your Java code is not just about adopting new technologies; it involves refining your development practices to enhance productivity and code quality. NetBeans provides essential hints that help in formatting, removing dead code, utilizing templates, refactoring, annotations, version control, and profiling.
Approaching coding with these practices in mind can lead to cleaner, more maintainable code. Embrace these strategies in your next project, and watch how your coding efficiency improves. Don't forget to explore the comprehensive NetBeans documentation for further insights and updates on new features.
Stay modern, stay efficient!