Mastering Git Bisect for Efficient Automated Bug Detection
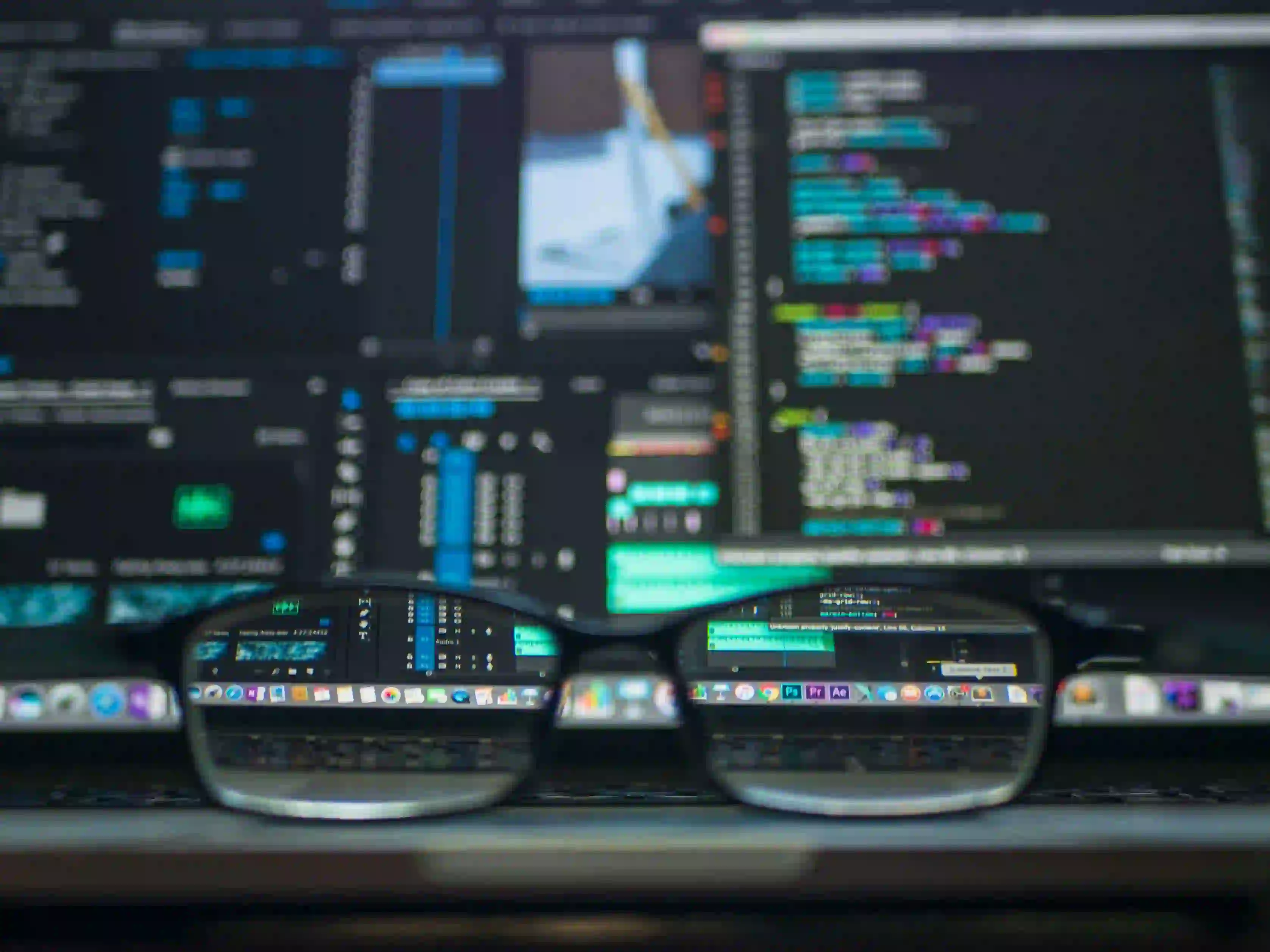
Mastering Git Bisect for Efficient Automated Bug Detection
When embarking on a software development journey, encountering bugs is practically inevitable. The challenge lies in tracing the roots of these bugs swiftly and efficiently. Enter Git Bisect, a powerful built-in tool within Git that allows developers to pinpoint the exact commit that introduced a bug. This blog post dives deep into mastering Git Bisect for automated bug detection, providing clear explanations, practical examples, and a structured approach that updates your understanding of this essential tool.
What is Git Bisect?
Before delving into the operational mechanics of Git Bisect, let's clarify its purpose. Git Bisect employs a binary search algorithm through the commit history of a repository. This enables developers to minimize the number of commits they need to inspect to find where a bug was introduced. Instead of checking every single commit sequentially, Git Bisect efficiently narrows down the search.
Setting Up the Environment
To demonstrate the effectiveness of Git Bisect, we will first simulate a scenario where a bug is introduced. For the purpose of this example, we are using a simple Java application.
Step 1: Create a Sample Project
Create a directory for your project:
mkdir SampleJavaApp
cd SampleJavaApp
Now create a basic Java file, Main.java
, and use the following code snippet:
public class Main {
public static void main(String[] args) {
System.out.println("Welcome to the Sample Java Application!");
int result = addNumbers(5, 10);
System.out.println("The result is: " + result);
}
public static int addNumbers(int a, int b) {
return a + b; // A simple addition operation
}
}
Step 2: Initialize Git and Commit Changes
Initialize a Git repository and make your first commit:
git init
git add Main.java
git commit -m "Initial commit: Add main application file"
Step 3: Introduce Bugs with Subsequent Commits
Add some functionality and intentionally introduce a bug:
// Adding a buggy feature
public static int subtractNumbers(int a, int b) {
return a - b + 1; // Intentional bug: incorrect logic
}
Commit these changes:
git add Main.java
git commit -m "Add buggy subtract method"
Now, let's fix that bug in the next commit:
public static int subtractNumbers(int a, int b) {
return a - b; // Bug fixed
}
And again, commit:
git add Main.java
git commit -m "Fix subtract method"
Now we have three commits: one initial, one buggy, and one with the bug fixed.
Using Git Bisect
With our commits in place, let's explore how to use Git Bisect.
Step 4: Start Git Bisect
Git bisect works by marking commits as "good" or "bad". First, begin the bisecting process:
git bisect start
Next, specify the bad commit (the latest commit):
git bisect bad
Then specify the good commit (the one before the bug was introduced):
git bisect good <commit-hash-of-initial-commit>
Now, Git will start checking out different commits between the good and bad commits.
Step 5: Test Commits
Once Git checks out a commit, it's your responsibility to test the code manually or automate the testing process with a script. If a bug exists in the current commit, let Git know:
git bisect bad
If not, mark it as good:
git bisect good
Repeat this process until Git identifies the commit that introduced the bug.
Step 6: Automating Bug Detection
To streamline this process, you could create a test script. Hereβs a simple example of how to automate the detection of a bug in the Java code:
#!/bin/bash
# This script compiles the Java code and checks for expected output
javac Main.java
output=$(java Main)
if [[ "$output" == *"The result is: 15"* ]]; then
exit 0 # Good commit
else
exit 1 # Bad commit
fi
Make sure to make it executable:
chmod +x test.sh
Now invoke Git Bisect using your script:
git bisect run ./test.sh
Git will now run the script automatically for each checked-out commit. It makes the entire process fast and efficient.
Step 7: Completing the Bisect Process
Once the bisect process is over and the offending commit is identified, you can end the bisecting session:
git bisect reset
This command takes you back to the original state of your repository.
Best Practices for Git Bisect
-
Consistency in Testing: Ensure that your tests are accurate and consistent to avoid false positives or negatives during the bisecting process.
-
Automate: The more you can automate the testing process, the faster you'll achieve results using Git Bisect.
-
Frequent Commits: Make smaller, frequent commits. It helps in isolating bugs to a smaller section of the commit history.
-
Document: Always document your commits clearly. It helps in understanding the modifications and tracking back changes.
Final Thoughts
Git Bisect is an indispensable tool for developers who want efficiency in bug detection. The ability to quickly narrow down the commits that introduced a bug can save countless hours of debugging time. By integrating Git Bisect into your workflow and leveraging automation, you can elevate your productivity and code quality.
Resources for Further Learning
- For detailed Git documentation: Git Documentation
- Enhance your knowledge on debugging with Effective Java by Joshua Bloch.
With this guide, you should now feel confident in using Git Bisect as part of your development process. Happy coding!