Spring Framework 3.2 M1: Common Upgrade Pitfalls to Avoid
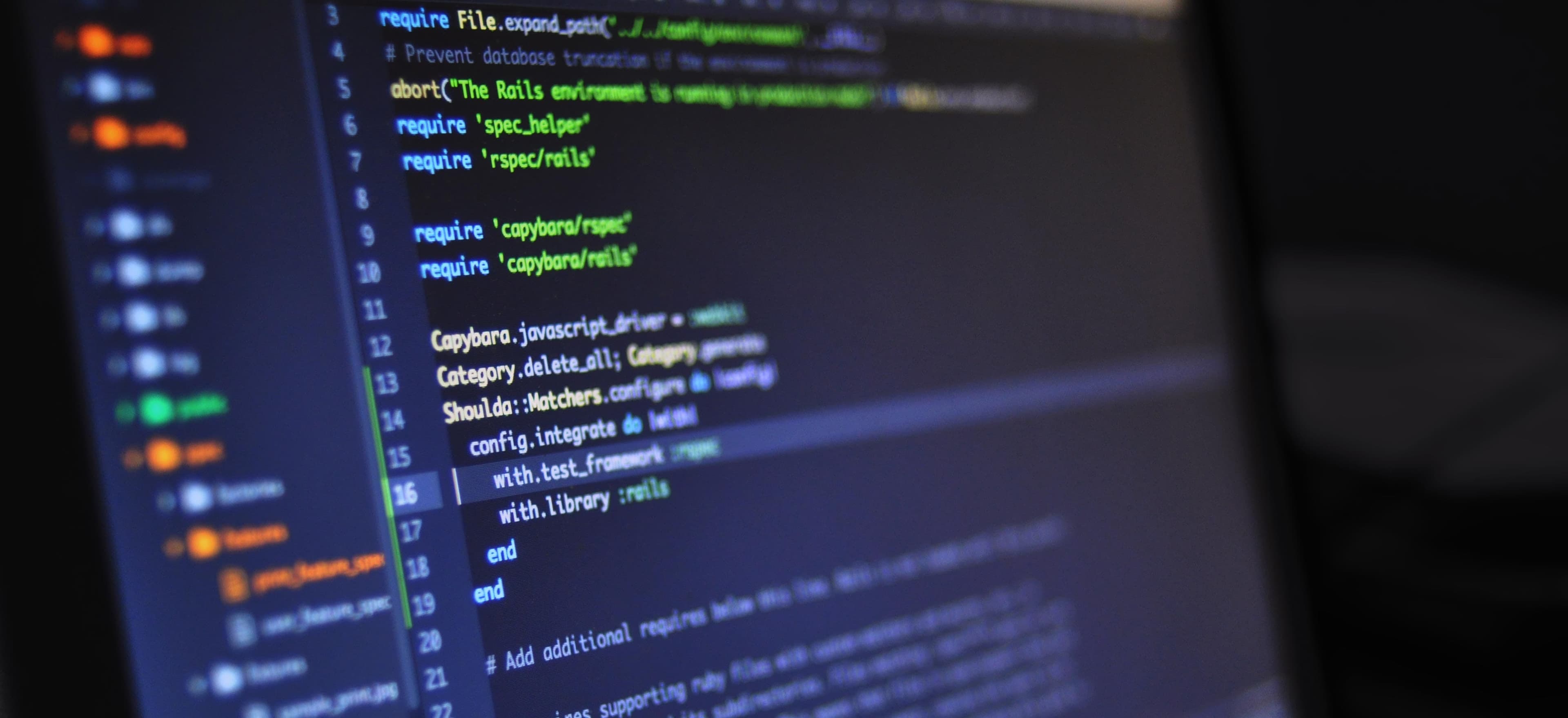
- Published on
Spring Framework 3.2 M1: Common Upgrade Pitfalls to Avoid
When it comes to software development, keeping up with frameworks and libraries is essential. Upgrades often come with new features, performance improvements, and enhanced security. However, upgrading to a new version also introduces challenges, especially with a framework as widely used as the Spring Framework. In this blog post, we'll explore common pitfalls when upgrading to Spring Framework 3.2 M1 and how to avoid them.
Understanding the Spring Framework
The Spring Framework is a powerful framework for building enterprise-level applications in Java. It provides comprehensive infrastructure support for developing Java applications and offers a versatile programming and configuration model. With the release of Spring Framework 3.2 M1, it’s crucial to understand what changes have been made, the new features introduced, and potential issues that may arise during upgrades.
Key Changes in Spring Framework 3.2 M1
Before diving into pitfalls, let’s briefly outline some of the notable features and changes in Spring 3.2 M1:
-
Support for Java 8: This version adds a variety of enhancements that leverage features introduced in Java 8, which can lead to more concise and functional-style programming in your applications.
-
Improved Performance: The Spring team has been working on performance enhancements to provide a faster and more responsive framework.
-
WebSocket Support: This version introduces native support for WebSocket, which is crucial for building real-time applications.
-
Spring Expression Language (SpEL) Enhancements: You can use new expressions and functions with increased flexibility, aiding complex configurations.
Common Upgrade Pitfalls
Let's discuss the common pitfalls developers face during this upgrade process, along with how to avoid them.
1. Incompatibility with Legacy Code
The Issue: One of the first issues encountered during an upgrade is incompatibility with legacy code. Libraries that worked well in previous versions might not function as expected after the upgrade.
How to Avoid:
Before upgrading, ensure a thorough code review of your current project. Identify and document dependencies, especially those that are outdated. Check the Spring Reference Documentation for breaking changes to APIs and potential alternatives.
// Example of a deprecated feature in a controller
@Controller
public class MyController {
@RequestMapping("/oldPath")
public String oldMethod() {
return "outdatedPage"; // Older method that may no longer be supported
}
}
2. Changes in Dependency Management
The Issue: Spring 3.2 M1 introduces refined dependency management that might conflict with your existing configurations.
How to Avoid:
Update your Maven or Gradle files respectively, and ensure that all dependencies are compatible with Spring 3.2. Always refer to the Maven Central Repository for updated versions.
For example, in your pom.xml
, you can update dependencies like this:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>3.2.0.RELEASE</version>
</dependency>
Make sure dependencies are well-aligned with the Spring version you are utilizing.
3. Misuse of New Features
The Issue: While new features can enhance functionality, they can also lead to misuse. For example, the introduction of WebSocket may tempt developers to implement it without fully understanding its implications in an existing architecture.
How to Avoid:
Take the time to understand new features and their best practices. A thorough review of the latest Spring Documentation will provide insights into the correct implementation of these features.
For example, establishing a WebSocket configuration might look like this:
@Configuration
@EnableWebSocket
public class WebSocketConfig implements WebSocketConfigurer {
@Override
public void registerWebSocketHandlers(WebSocketHandlerRegistry registry) {
registry.addHandler(new MyWebSocketHandler(), "/mySocket")
.setAllowedOrigins("*");
}
}
This simple setup allows for messaging over WebSocket with appropriate origin handling.
4. Ignoring Configuration Changes
The Issue: Spring 3.2 M1 has introduced changes in configuration methods. Ignoring these can lead to runtime errors and misbehaving applications.
How to Avoid:
Go through your configuration files meticulously. Make updates where prompted by the new configuration styles.
For instance, moving from XML to Java-based configurations promotes type safety and eliminates the XML verbosity.
@Configuration
public class AppConfig {
@Bean
public MyBean myBean() {
return new MyBean();
}
}
This configuration helps you avoid common XML errors and provides a cleaner structure.
5. Testing Not Caught Up
The Issue: When upgrading, it’s easy to overlook the testing ecosystem. Deprecated methods or altered functionality can lead to broken tests.
How to Avoid:
Revisit your test cases after the upgrade and ensure that they reflect the changes in code. Use JUnit along with Spring's test context framework to effectively test your application.
Example of a basic test setup for your service layer:
@RunWith(SpringJUnit4ClassRunner.class)
@ContextConfiguration(classes = AppConfig.class)
public class MyServiceTest {
@Autowired
private MyService myService;
@Test
public void testServiceMethod() {
assertEquals("Expected Value", myService.methodToTest());
}
}
Ensure tests are updated to reflect code changes and utilize any new features for improved testing.
6. Not Reviewing Third-Party Libraries
The Issue: Third-party libraries may not be compatible with Spring 3.2 M1 and can introduce critical bugs.
How to Avoid:
Conduct a thorough review of all third-party libraries in use. Check each library’s documentation for compatibility with Spring 3.2 M1 and consider alternatives if necessary.
7. Neglecting Performance Testing
The Issue: New features and upgraded functionalities can inadvertently affect application performance.
How to Avoid:
After upgrading, ensure you perform thorough performance testing. Use tools like JMeter or Gatling to simulate loads and view how your application handles demands.
Bringing It All Together
Upgrading to Spring Framework 3.2 M1 can significantly enhance your application, but it comes with its own challenges. By being aware of the common pitfalls and taking proactive steps to avoid them, you can make the upgrade process smoother and your application more robust.
Remember to take advantage of the Spring Community for assistance and updated practices.
Whether you are a seasoned developer or just starting your journey, learning how to effectively manage upgrades is a valuable skill. Keep your code clean and well-documented, and always stay ahead of the curve with framework updates!
This blog post has given you an overview of potential challenges when upgrading to Spring Framework 3.2 M1 and practical steps to navigate them smoothly. Happy coding!