Mastering CSV Parsing in Java: Iterator vs. Decorator Dilemma
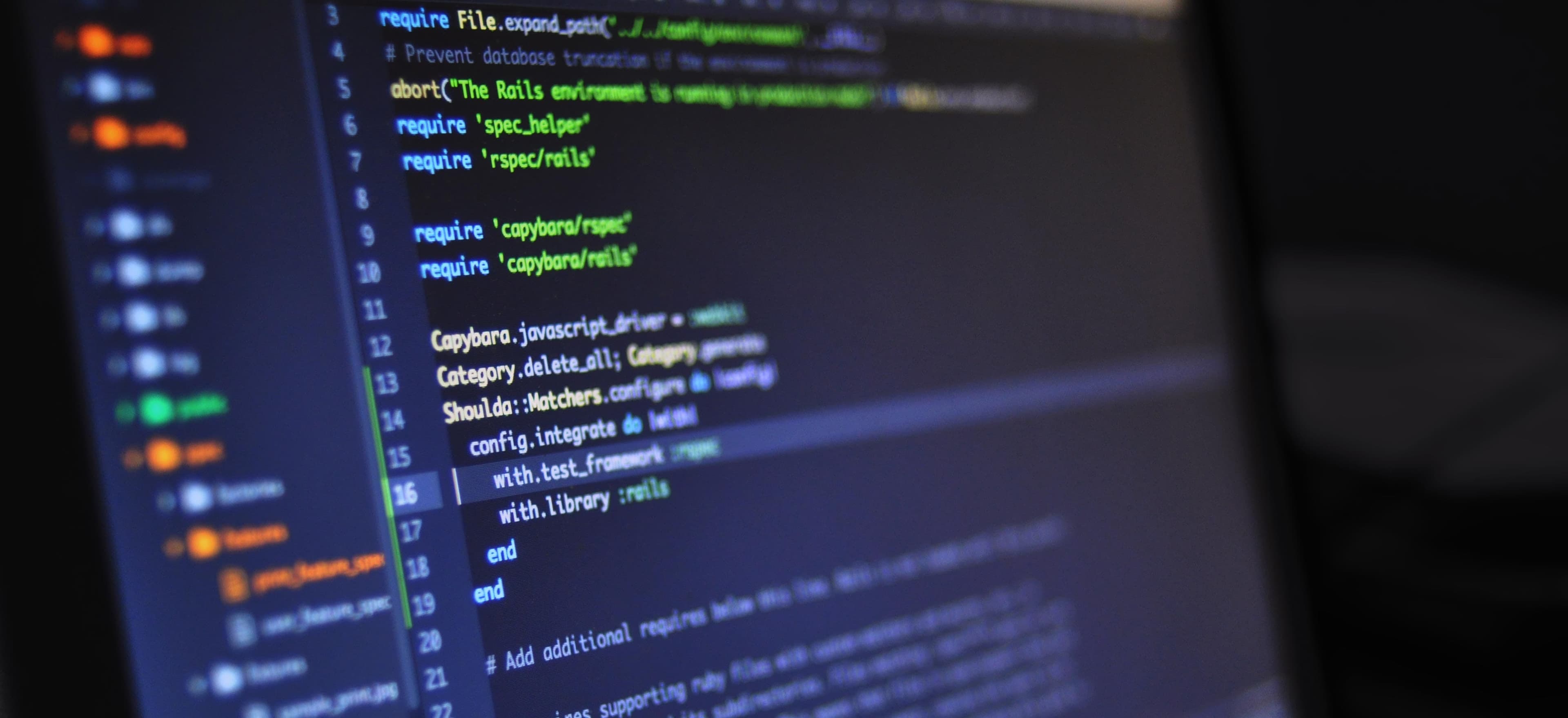
- Published on
Mastering CSV Parsing in Java: Iterator vs. Decorator Dilemma
Parsing CSV files is a common task in software development. Several libraries are available to help developers streamline this process. In Java, two common approaches to parsing CSV files are to utilize the Iterator pattern or the Decorator pattern. This blog post will break down both paradigms, discuss their pros and cons, and help you choose the best one for your project.
What is CSV?
CSV stands for Comma-Separated Values. It is a simple file format used to store tabular data, such as spreadsheets or databases, in plain text. Each line in the file corresponds to a row in the table, and each field in the row is separated by a comma. CSV is ubiquitous due to its simplicity and ease of use, but it can sometimes lead to complications, especially when handling large datasets or accounting for various delimiters.
Choosing the Right Parsing Strategy: Iterator vs. Decorator
Both the Iterator pattern and the Decorator pattern have their merits, but they serve different purposes.
1. Iterator Pattern
The Iterator pattern allows you to traverse the elements of a collection one at a time without exposing the underlying representation. It enables you to read the contents of a CSV file in a streamlined manner.
Pros:
- Simplicity: It’s straightforward and easy to implement.
- Memory Efficiency: By reading one record at a time, it can be less memory-intensive for large datasets.
- Direct Access: You can easily iterate over elements without additional transformations.
Cons:
- Single Responsibility: If your requirements change to include additional functionalities (like aggregation or processing), the iterator alone may not suffice.
- Limited Features: An Iterator typically provides basic read capabilities without built-in data manipulation features.
Implementation Example
Here is an example of implementing a simple CSV parser using the Iterator pattern in Java:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.Iterator;
import java.util.NoSuchElementException;
public class CSVIterator implements Iterator<String[]> {
private BufferedReader reader;
private String nextLine;
public CSVIterator(String filePath) throws IOException {
reader = new BufferedReader(new FileReader(filePath));
nextLine = reader.readLine(); // Initialize with the first line
}
@Override
public boolean hasNext() {
return nextLine != null;
}
@Override
public String[] next() {
if (nextLine == null) {
throw new NoSuchElementException();
}
String[] values = nextLine.split(","); // Split the line into values
try {
nextLine = reader.readLine(); // Move to the next line
} catch (IOException e) {
nextLine = null; // End of file
}
return values;
}
public void close() throws IOException {
reader.close();
}
}
Commentary on the Code
This code defines a CSVIterator
class that takes a file path as input. It implements the Iterator<String[]>
interface, which allows us to iterate over the array of strings (each array represents a row in the CSV). The hasNext()
method checks if there are more lines to read, while the next()
method reads the next line and splits it into a string array.
2. Decorator Pattern
The Decorator pattern allows you to add new functionality to an existing object dynamically. When applied to CSV parsing, it can be used to enrich the data or add overhead before parsing.
Pros:
- Flexibility: You can dynamically add features like filtering, transformation, or validation.
- Enhanced Reusability: You can decorate existing parsers with additional functionalities without modifying the original code.
Cons:
- Complexity: This pattern can make your code harder to understand and maintain.
- Performance Overhead: Each decoration adds a layer of complexity, which may impact performance, particularly on large files.
Implementation Example
Using the Decorator pattern, we can create a CSV parser that adds functionality for filtering rows based on specific criteria:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
import java.util.Iterator;
public class FilteredCSVIterator implements Iterator<String[]> {
private Iterator<String[]> baseIterator;
private String filterKeyword;
private String[] nextFilteredLine;
public FilteredCSVIterator(CSVIterator baseIterator, String filterKeyword) {
this.baseIterator = baseIterator;
this.filterKeyword = filterKeyword;
findNext();
}
@Override
public boolean hasNext() {
return nextFilteredLine != null;
}
@Override
public String[] next() {
if (nextFilteredLine == null) {
throw new NoSuchElementException();
}
String[] current = nextFilteredLine;
findNext();
return current;
}
private void findNext() {
nextFilteredLine = null;
while (baseIterator.hasNext()) {
String[] line = baseIterator.next();
if (containsFilter(line)) {
nextFilteredLine = line;
break;
}
}
}
private boolean containsFilter(String[] line) {
for (String value : line) {
if (value.contains(filterKeyword)) {
return true;
}
}
return false;
}
public void close() throws IOException {
if (baseIterator instanceof CSVIterator) {
((CSVIterator) baseIterator).close();
}
}
}
Commentary on the Code
In this implementation, FilteredCSVIterator
takes a CSVIterator
instance and a filtering keyword. The findNext()
function looks for the next line that contains the specified keyword. This allows users to skip irrelevant rows while iterating through the CSV.
When to Use Each Pattern
Use the Iterator Pattern when:
- You are primarily focused on reading and traversing CSV records.
- The requirements are simple and do not demand advanced features.
Use the Decorator Pattern when:
- You need to introduce additional functionalities to the existing functionality dynamically.
- Your application might evolve, requiring filtering, data transformation, or validation of the CSV data.
Key Takeaways
Both the Iterator and Decorator patterns offer unique benefits. Your choice will largely depend on your project's requirements and complexity. If your goal is straightforward CSV reading, the Iterator pattern will suffice. However, if you foresee the need for enhanced functionality, the Decorator pattern will set you up for success.
Feel free to explore popular libraries like Apache Commons CSV or OpenCSV for advanced features and capabilities in CSV parsing.
Always remember, whether you choose Iterator or Decorator, clean, maintainable code should be your primary goal. Happy coding!