Understanding Limitations of Raw String Literals in Java
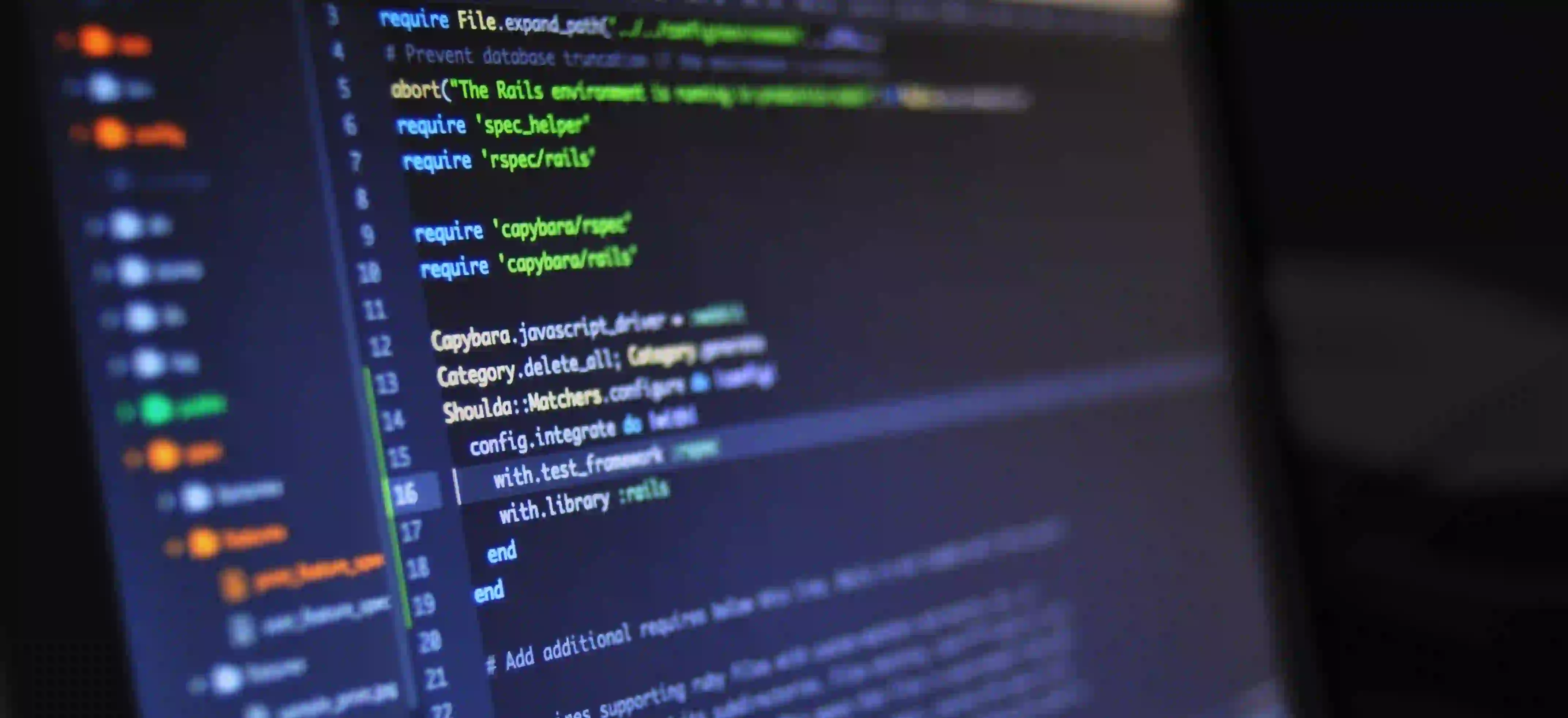
Understanding Limitations of Raw String Literals in Java
Java has always been a versatile programming language with a strong emphasis on readability and maintainability. As part of its ongoing evolution, Java introduced raw string literals in Java 13. This feature allows developers to write multi-line strings without escaping special characters, such as newlines or quotes, leading to cleaner code. However, raw string literals come with their own set of limitations and nuances that are important to understand.
In this blog post, we will explore these limitations while providing clear examples to illustrate the points. So grab your favorite IDE, and let’s dive in!
What Are Raw String Literals?
Raw string literals allow you to create strings that can span multiple lines without the need for escaping quotation marks or line breaks. They are defined using three double-quote characters ("""
) at the start and end. This functionality is particularly useful for embedding large blocks of text such as JSON, SQL queries, or HTML content.
Example of Raw String Literals
Here’s how you can declare a raw string literal:
String query = """
SELECT *
FROM users
WHERE age > 18
""";
In this example, we declare a SQL query as a raw string literal. It retains the formatting, making it much easier to read compared to conventional string literals.
Limitations of Raw String Literals
Despite their advantages, raw string literals in Java are not without limitations. Understanding these will help you make better design choices in your code.
1. Indentation Handling
One of the most immediately noticeable limitations is the handling of indentation. Raw string literals preserve leading white spaces, but you cannot control indentation directly based on context. Here’s a breakdown of what that looks like:
String indentedString = """
This is a line.
This is indented.
This is another line.
""";
In the above snippet, the raw string will retain the leading spaces as is. You may end up with unexpected white spaces when directly using these strings in ways where consistent formatting is critical.
2. No Escape Sequences
While the removal of the need for escape sequences is liberating, it also means that you won’t be able to use them if you need that functionality. For instance, if your string needs to include a single quote ('
), it can be included directly, but if you wish to have a backslash (\
), it may result in confusion.
String specialChars = """
Here's a backslash: \
And a quote: '
""";
In the above code, it's ambiguous whether the backslash will appear as you intend or cause issues with a JSON object later on.
3. Unwanted Newlines
Another limitation involves unwanted newlines at the beginning and end of your strings. When you declare a raw string, Java automatically adds a newline at the end of the string, which could lead to undesired behavior when concatenating strings or passing values.
Consider the following example:
String multilineString = """
This is a string.
""";
When printed, this will include an implicit newline that can introduce errors in output formatting.
4. No String Interpolation
Unlike languages such as Python, Java’s raw string literals don’t support string interpolation. This means you cannot directly insert variable values within your raw strings. You must concatenate strings explicitly, which can deter readability.
String name = "Alice";
String greeting = """
Hello, """ + name + """!
Welcome to our platform.
""";
While you can achieve the desired result, the concatenation approach can clutter your code and make it less readable compared to languages with native interpolative capabilities.
5. Not All Characters Are Allowed
Certain characters are not permissible within raw string literals. For example, you cannot include an unescaped triple quote within a raw string literal as that will prematurely terminate your string.
String invalidString = """
This is a "quote" but we can't have """; // This will cause a compilation error
This forces you to either break down the string or use escape sequences, which undermines the purpose of using raw string literals.
Practical Use Cases
While there are limitations, raw string literals can still be used advantageously in various scenarios. Here are a few use cases.
1. JSON Strings
When working with JSON data, raw string literals provide a clear way to structure data.
String jsonString = """
{
"name": "Alice",
"age": 30,
"city": "Wonderland"
}
""";
This format is clean and directly reflects the structure of the JSON.
2. Multi-line SQL Queries
As previously demonstrated, embedding multi-line SQL queries becomes much easier and more readable.
3. Prose and Documentation
For logging or displaying multi-line messages, raw string literals make for cleaner syntax.
Lessons Learned
Java's raw string literals are a significant improvement for managing multi-line strings, enhancing readability and streamlining code. However, they come with constraints that developers must navigate carefully.
By understanding these limitations, such as indentation handling, escape sequences, and unwanted newlines, you can make informed decisions about where and how to use raw string literals in your code. The flexibility they offer must be balanced against the challenges they present.
For more information about Java string handling and related features, check out the official Java documentation or explore string formatting techniques in Java here.
Happy coding!