The Hidden Costs of Java Util Stack: A Developer's Dilemma
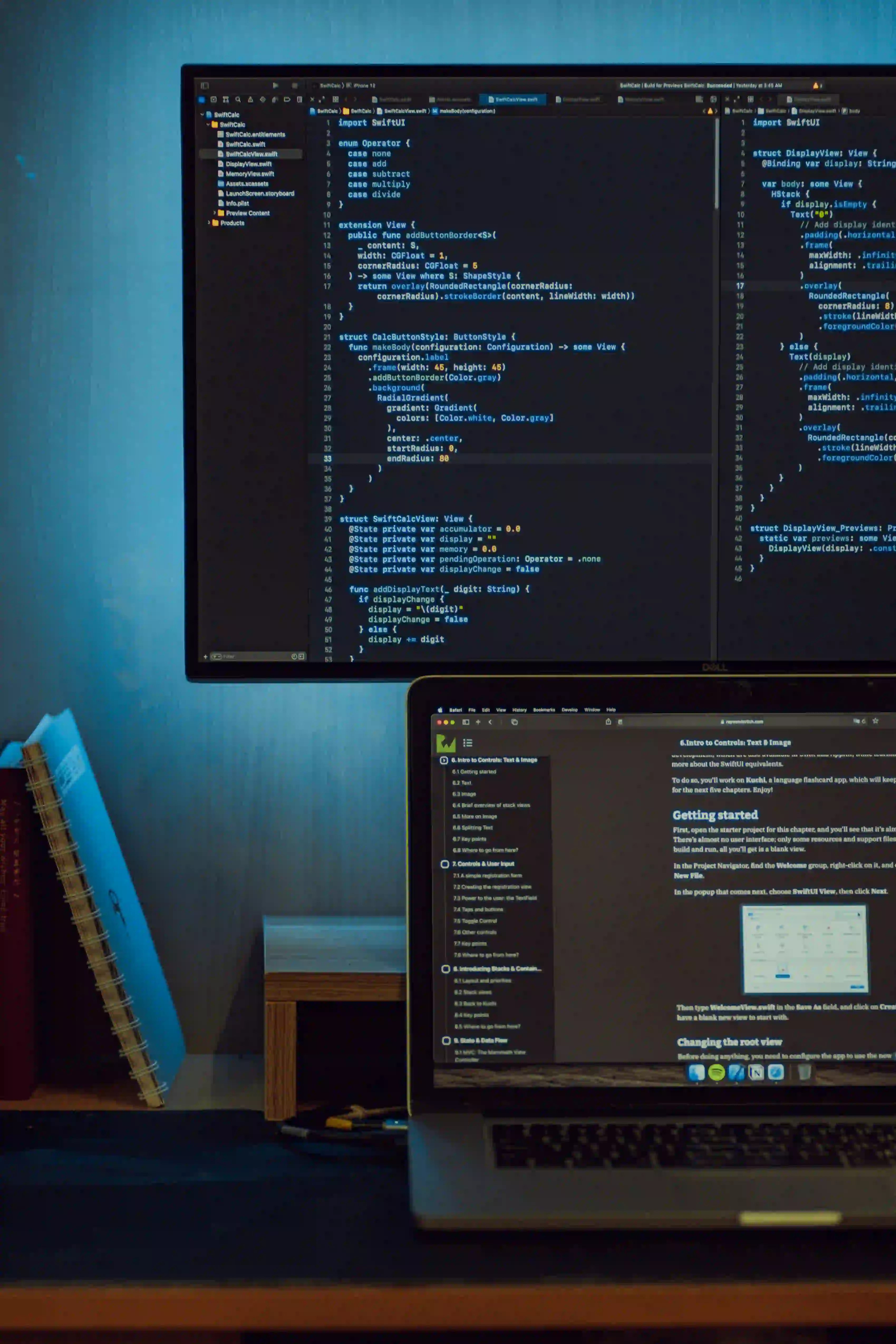
The Hidden Costs of Java Util Stack: A Developer’s Dilemma
In the world of Java programming, the java.util.Stack
class is often seen as a helpful utility for managing data in a last-in, first-out (LIFO) manner. However, while it may seem like a straightforward solution, there are hidden costs involved that every developer should be aware of. This post explores these costs, providing insight into better alternatives and practical code snippets to enhance your software development journey.
Understanding Java Util Stack
The Stack
class extends Vector
and represents a stack of objects. It allows for pushing, popping, and peeking at elements efficiently. However, as developers integrate this class into their projects, they may encounter several unforeseen issues.
The Basic Operations of a Stack
Let's quickly recap the core operations available in a java.util.Stack
:
- push(E item): Adds an item to the top of the stack.
- pop(): Removes and returns the item at the top of the stack.
- peek(): Returns the item at the top of the stack without removing it.
- isEmpty(): Checks if the stack is empty.
- search(Object o): Returns the 1-based position of the object from the top of the stack.
Here’s a simple illustration of these operations:
import java.util.Stack;
public class StackExample {
public static void main(String[] args) {
Stack<Integer> stack = new Stack<>();
// Push elements onto the stack
stack.push(1);
stack.push(2);
stack.push(3);
// Peek at the top element
System.out.println("Top element: " + stack.peek()); // Outputs: 3
// Pop elements from the stack
System.out.println("Popped: " + stack.pop()); // Outputs: 3
System.out.println("Popped: " + stack.pop()); // Outputs: 2
// Check if stack is empty
System.out.println("Is stack empty? " + stack.isEmpty()); // Outputs: false
}
}
While these operations are straightforward, relying on java.util.Stack
may introduce numerous hidden costs.
The Hidden Costs of Using Java Util Stack
1. Performance Overhead
Although java.util.Stack
derives from Vector
, which makes it inherently synchronized, this synchronization can introduce unnecessary performance overhead. If your application does not require concurrent access, this can lead to decreased performance.
Solution: Consider using ArrayDeque
as a more performant alternative for stack behavior without the overhead of synchronization:
import java.util.ArrayDeque;
public class DequeExample {
public static void main(String[] args) {
ArrayDeque<Integer> deque = new ArrayDeque<>();
// Push operations (add to the front)
deque.push(1);
deque.push(2);
deque.push(3);
// Peek at the top element
System.out.println("Top element: " + deque.peek()); // Outputs: 3
// Pop operations (remove from the front)
System.out.println("Popped: " + deque.pop()); // Outputs: 3
}
}
2. Inheritance from Vector
The Stack
class inherits the methods (and their costs) associated with Vector
, such as addElement
, removeElement
, and more. Many of these methods may not be relevant in the context of a stack and lead to potential misuse or confusion.
Approach: When creating custom stack structures, it is better to implement the interface behaviors rather than extending Vector
.
3. Thread Safety and Concurrency Issues
The Stack
class is inherently thread-safe due to synchronized methods. However, this does not protect against concurrent modifications from multiple threads, leading to unpredictable behavior in multi-threading scenarios.
Recommendation: If you need a stack in a multi-threaded environment, use java.util.concurrent.BlockingDeque
or synchronized collections like Collections.synchronizedList(new ArrayList<>())
.
4. Resizing Costs
As Stack
is built on Vector
, it utilizes an array that may need to be resized as elements are pushed. This resizing operation can significantly increase time complexity, especially when it involves copies of large arrays.
Alternative: ArrayDeque
manages its resizing without performance hits typical of Vector
, making it a better choice for stack operations.
5. API Design Choice
The design of java.util.Stack
can lead to poor API practices, encouraging methods better suited for a list, which can encourage bad architectural choices. Pushing and popping should ideally not be mixed with API designs intended for lists.
My Closing Thoughts on the Matter: Choose Wisely
Choosing the right data structure is crucial for performance and maintainability in any application. While java.util.Stack
may signal simplicity at first glance, the hidden costs can accumulate, causing blowback in performance and functionality.
As a developer, you should always keep the following factors in mind:
- Performance needs: Assess if synchronization matters.
- Use Case: Consider the type of data structure that best suits your needs, such as
ArrayDeque
, for simple LIFO requirements. - Maintainable code: Keep your APIs clean and make sure that the right tool is used for the right job.
For further exploration of data structures and performance considerations in Java, check out the Java Collections Framework.
By understanding the hidden costs associated with java.util.Stack
, you pave the way for more efficient, effective, and maintainable code. Happy coding!