Overcoming Pipeline Bottlenecks in Docker and Jenkins Integration
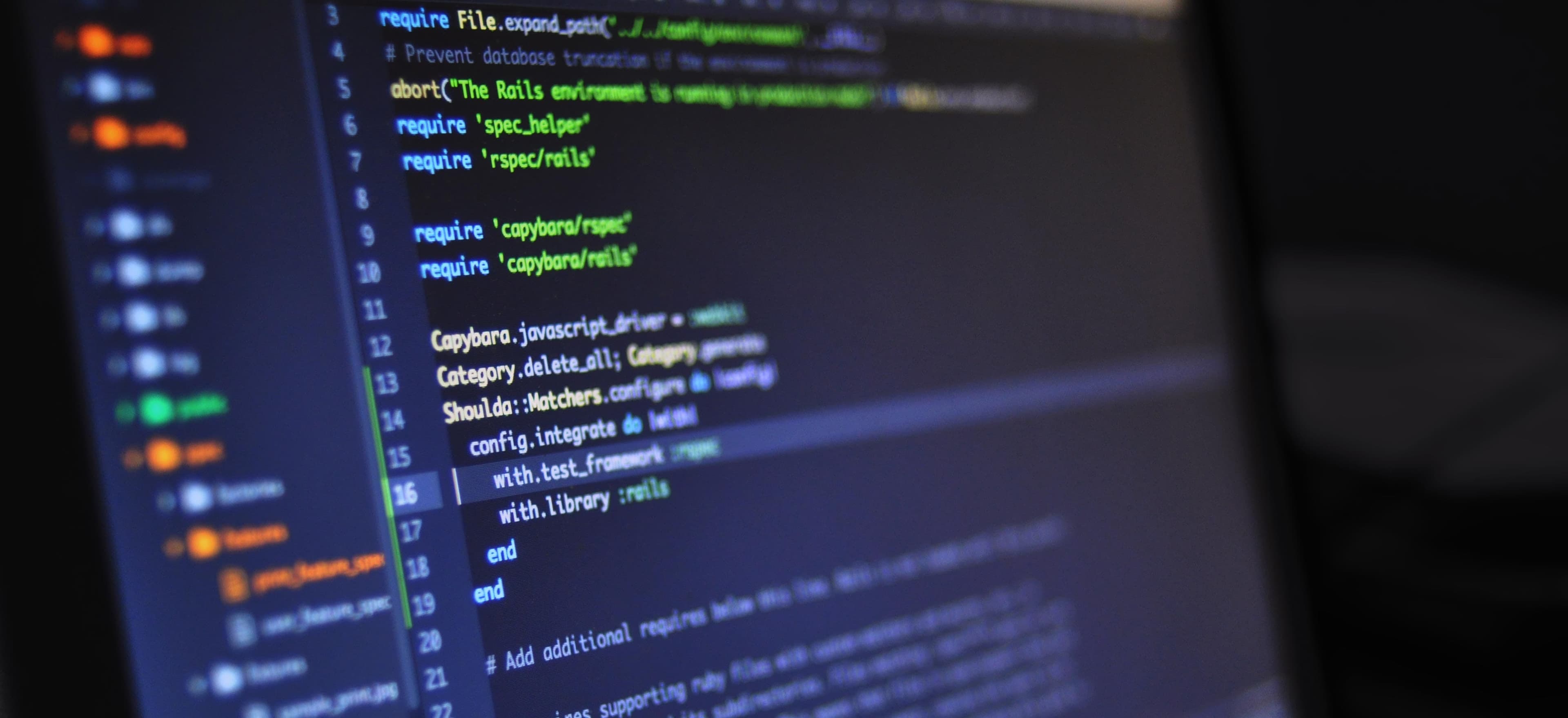
- Published on
Overcoming Pipeline Bottlenecks in Docker and Jenkins Integration
In the world of Continuous Integration and Continuous Deployment (CI/CD), integration tools like Jenkins combined with containerization technologies such as Docker have revolutionized the way software is built, tested, and deployed. However, as with any technology, challenges arise. One significant challenge developers often face is pipeline bottlenecks, which can drastically slow down the integration process. In this blog post, we’ll explore common bottlenecks in Docker and Jenkins integration, how to identify them, and effective strategies to overcome these issues.
Understanding Pipeline Bottlenecks
A bottleneck in a pipeline occurs when a certain stage slows down the overall process, causing all subsequent stages to wait. This might be due to resource limitations, inefficiencies in configuration, or even inadequate code practices. In the context of Docker and Jenkins, bottlenecks can arise during various stages including build, test, and deployment.
Let's break down some of these stages to better understand where bottlenecks might originate.
Common Causes of Bottlenecks
- Build Time: Longer compilation and build times can halt the pipeline. This can be exacerbated when large Docker images are created without optimizations.
- Inefficient Docker Images: Using large or unnecessarily complex images could slow down deployment. A minimal image is optimal for quick deployments.
- Parallel Job Execution: If multiple jobs try to access the same resource, such as a database, this can create contention and slow everything down.
- Insufficient Resource Allocation: Docker containers may consume more resources than allocated, leading to decreased performance.
- Network Latency: When pulling images or accessing remote resources, network issues can introduce delays.
Identifying Bottlenecks
Before we can overcome bottlenecks, we need to identify them. Here are some strategies:
-
Logging and Monitoring: Implement comprehensive logging of your Jenkins pipeline stages. Tools like the Jenkins Build Monitor Plugin can help visualize where time is being spent.
-
Pipeline Stages Timing: Enable timing for each stage in Jenkins to determine which stage takes the most time. For example:
pipeline { agent any stages { stage('Build') { steps { script { def startTime = System.currentTimeMillis() // Building Docker Image sh 'docker build -t myapp:latest .' def endTime = System.currentTimeMillis() echo "Build Time: ${endTime - startTime} milliseconds" } } } } }
-
Use of Profiling Tools: Tools such as Prometheus and Grafana can help visualize usage patterns. By ingesting metrics, you can see where the most resources are being consumed.
Overcoming Bottlenecks
1. Optimize Docker Builds
Optimizing the Docker build process is essential to speed up the pipeline. Here are a few tactics to consider:
-
Minimize Image Size: Use a minimalist base image. For example, changing from a bulky Ubuntu image to a smaller Alpine image can save significant space and time.
FROM alpine:latest RUN apk add --no-cache my-dependencies
-
Layer Caching: Docker builds images in layers. Try to reorder
Dockerfile
commands to leverage caching. Place commands that aren’t likely to change as high up in yourDockerfile
as possible.# Install dependencies first to leverage caching FROM node:alpine WORKDIR /app COPY package.json yarn.lock ./ RUN yarn install COPY . . CMD ["node", "server.js"]
2. Enable Parallel Execution
Allow consistency on multi-node builds and harness the full power of your infrastructure by configuring Jenkins to run jobs in parallel where possible.
pipeline {
agent any
stages {
parallel {
stage('Unit Tests') {
steps { runUnitTests() }
}
stage('Integration Tests') {
steps { runIntegrationTests() }
}
}
}
}
3. Resource Allocation and Scaling
Make sure your Jenkins setup has sufficient resources. Increasing CPU and memory can lead to significant performance improvements, especially when using Docker.
-
Cloud Providers: Utilize cloud-based solutions to scale resources dynamically. For example, AWS EC2 instances can be launched on-demand based on build requirements.
-
Docker Compose for Local Development: When effective development environments are needed, using Docker Compose can simulate your pipeline locally, speeding up debugging times and avoiding slow deployments.
4. Use of Caching Strategies
Implement caching strategies, both in Docker and Jenkins. Here are some approaches to consider:
-
Docker Layer Caching: By using specific build flags (
--cache-from
), reused image layers can significantly speed up builds. -
Jenkins Artifacts: Use Jenkins' artifact stash and unstash capabilities to cache files generated between builds. This decreases the need to regenerate files used in subsequent stages.
stages { stage('Build') { steps { sh 'docker build -t myapp:latest .' stash includes: '**/*.jar', name: 'jar-files' } } stage('Deploy') { steps { unstash 'jar-files' sh 'deploy-command' } } }
5. Image Cleanup
Regularly clean up unused Docker images and containers. Accumulation can lead to space issues on the host machine, slowing down image pull times during deployments.
docker system prune -a --volumes
Key Takeaways
Docker and Jenkins are powerful tools that, when used effectively, can accelerate the software development lifecycle. However, pipeline bottlenecks can significantly hinder efficiency and productivity.
By optimizing builds, allowing parallel execution, managing resources wisely, employing caching strategies, and conducting regular cleanups, you can mitigate these issues.
For a deeper dive into optimizing Docker with CI/CD practices, check out Docker's official documentation and enhance your Jenkins prowess with the Jenkins user guide.
This multifaceted approach will ensure your pipeline remains smooth, fast, and efficient, allowing your development team to focus on what matters most: building great software!
Checkout our other articles