Avoiding Common Pitfalls in Test-Driven Development
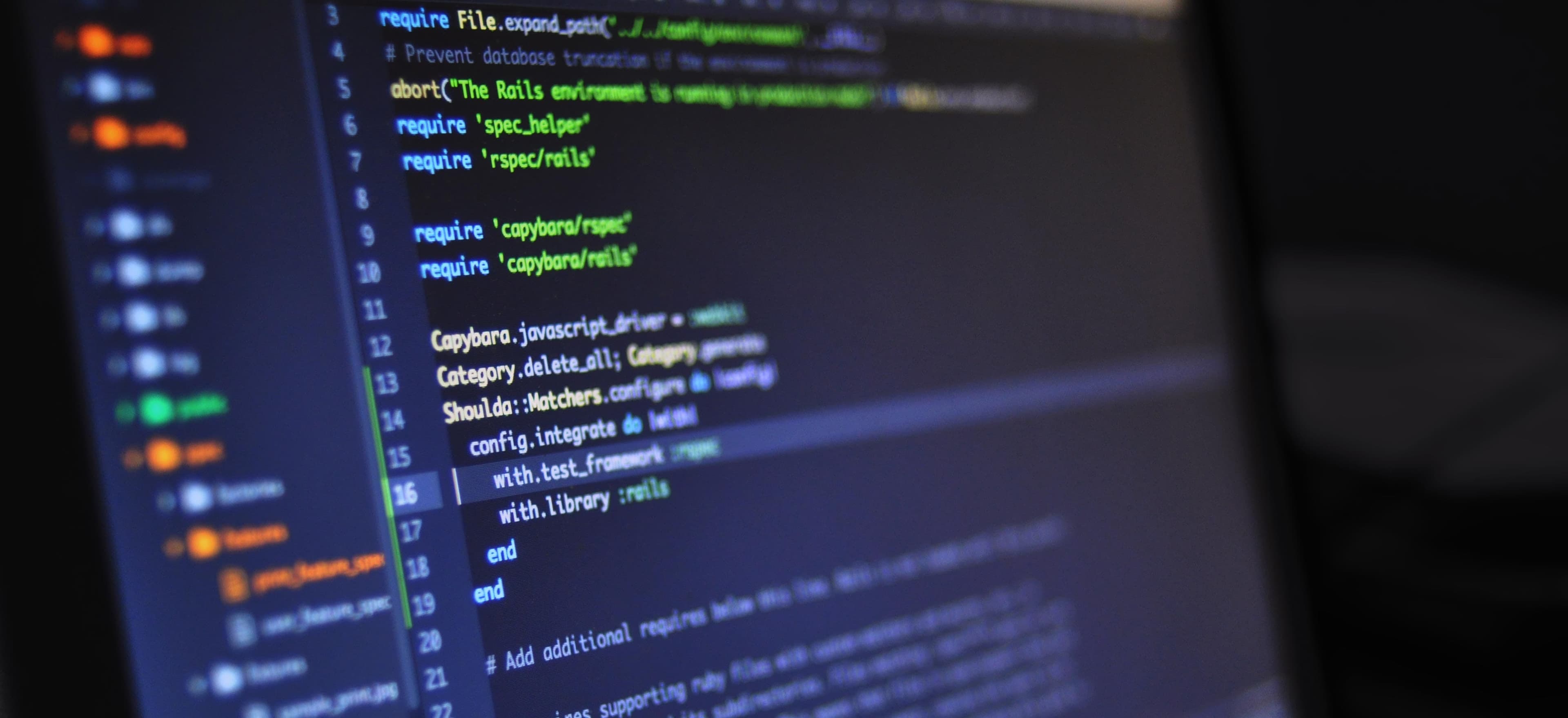
- Published on
Avoiding Common Pitfalls in Test-Driven Development
Test-Driven Development (TDD) is a powerful programming paradigm that emphasizes writing tests before the actual code. While TDD can lead to better-designed, more maintainable software, the journey is not without its duplicitous paths. In this blog post, we'll explore common pitfalls encountered during TDD and strategies to avoid them.
What is Test-Driven Development?
Before diving into the pitfalls, let's clarify what TDD entails. TDD is based on repeating a very short development cycle:
- Write a Test: Start with a failing test that defines a desired improvement or new function.
- Make the Test Pass: Write the minimum amount of code necessary to pass the test.
- Refactor: Clean up the code while ensuring that all tests still pass.
This cycle, often referred to as "Red-Green-Refactor," helps guide development and ensures that the code remains testable.
Common Pitfalls in TDD
1. Writing Too Many Tests
Pitfall: It's tempting to add tests for every possible scenario, leading to an overwhelming test suite.
Solution: Focus on writing meaningful tests. Identify the most critical functions and edge cases that will add value. Every test should serve a purpose, ideally related to real-world user stories.
Code Snippet Example
@Test
public void shouldReturnCorrectSumWhenAddingTwoNumbers() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result); // Simple, focused test
}
Why: A focused test like this one ensures clarity without unnecessarily complicating the test suite.
2. Not Running Tests Frequently
Pitfall: Developers sometimes write extensive tests but forget to run them regularly.
Solution: Integrate testing into your development workflow. Use continuous integration tools like Jenkins or Travis CI to automatically run tests when code is pushed to the repository.
Code Snippet Example
# .travis.yml for CI integration
language: java
jdk: openjdk11
script:
- mvn test
Why: This ensures that any change to the codebase doesn't break existing functionality and helps in early detection of issues.
3. Ignoring Test Maintenance
Pitfall: Tests morph into unmanageable beasts over time. As the code changes, so too do the tests, and if not addressed, tests can become obsolete or provide incorrect feedback.
Solution: Regularly revisit and refactor tests. Treat them as first-class citizens in your codebase.
Code Snippet Example
@Test
public void shouldThrowExceptionWhenDividingByZero() {
Calculator calculator = new Calculator();
assertThrows(IllegalArgumentException.class, () -> {
calculator.divide(5, 0); // Handles edge case
});
}
Why: By testing edge cases explicitly and anticipating potential errors, you enhance the test's robustness.
4. Focusing on Implementation Instead of Behavior
Pitfall: Developers often write tests that are too closely tied to the implementation rather than the expected behavior.
Solution: Focus on testing what the code does rather than how it does it. This will help keep your tests relevant even if the underlying implementation changes.
Code Snippet Example
@Test
public void shouldReturnExpectedGreeting() {
Greeter greeter = new Greeter();
String greeting = greeter.greet("Alice");
assertEquals("Hello, Alice!", greeting); // Behavior-focused
}
Why: Behavior-driven tests are less susceptible to changes in the underlying code structure, promoting maintainability.
5. Neglecting Edge Cases
Pitfall: Developers sometimes forget to test edge cases, assuming that their code works under normal conditions.
Solution: Always consider boundary conditions and edge cases when writing tests. Keep a checklist to remind yourself of potential edge scenarios.
Code Snippet Example
@Test
public void shouldHandleNegativeNumbers() {
Calculator calculator = new Calculator();
int result = calculator.add(-5, 3);
assertEquals(-2, result); // Tests edge case
}
Why: Edge tests may reveal hidden bugs, providing a more robust solution.
6. Pair Testing Abuse
Pitfall: While pair programming can enhance coding practices, dependencies can form that limit individual growth.
Solution: Foster independence. Encourage developers to occasionally write tests alone, allowing them to build confidence.
7. Over-complicating Tests
Pitfall: Tests become complex, with interdependencies that may confuse rather than clarify.
Solution: Keep tests simple. Ensure each test focuses on a single behavior, avoiding intricate setups.
Code Snippet Example
@Test
public void shouldReturnValidUsername() {
UserValidator validator = new UserValidator();
assertTrue(validator.isValid("validUser"));
}
Why: Simple, straightforward tests enhance readability, making it easy for developers to identify issues.
8. Failing to Communicate with the Team
Pitfall: Developers may not align on TDD methodologies, leading to a fragmented development process.
Solution: Conduct regular team discussions around TDD best practices to ensure a collective understanding.
9. Skipping Refactoring
Pitfall: The final step of TDD, refactoring, is often overlooked.
Solution: Treat refactoring with the same importance as writing tests. Refactor for clarity, performance, and maintainability.
My Closing Thoughts on the Matter
Test-Driven Development is an invaluable approach when properly executed. By avoiding common pitfalls like writing excessive tests, neglecting test maintenance, and focusing too closely on implementation, developers can produce better, more reliable software. Remember that the goal of TDD is not just to write tests but to ensure the code behaves as expected while remaining adaptable to change.
Embrace TDD! Frequently run your tests, keep them maintainable, and focus on the behavior rather than the implementation. As the codebase grows, so too will your confidence in the quality of your software.
For more resources on TDD, consider exploring the following:
- Martin Fowler's Introduction to TDD
- JUnit 5 User Guide
- Effective Unit Testing Techniques
Happy coding, and best of luck on your TDD journey!
Checkout our other articles