Navigating Localization Challenges in Spring 3 Internationalization
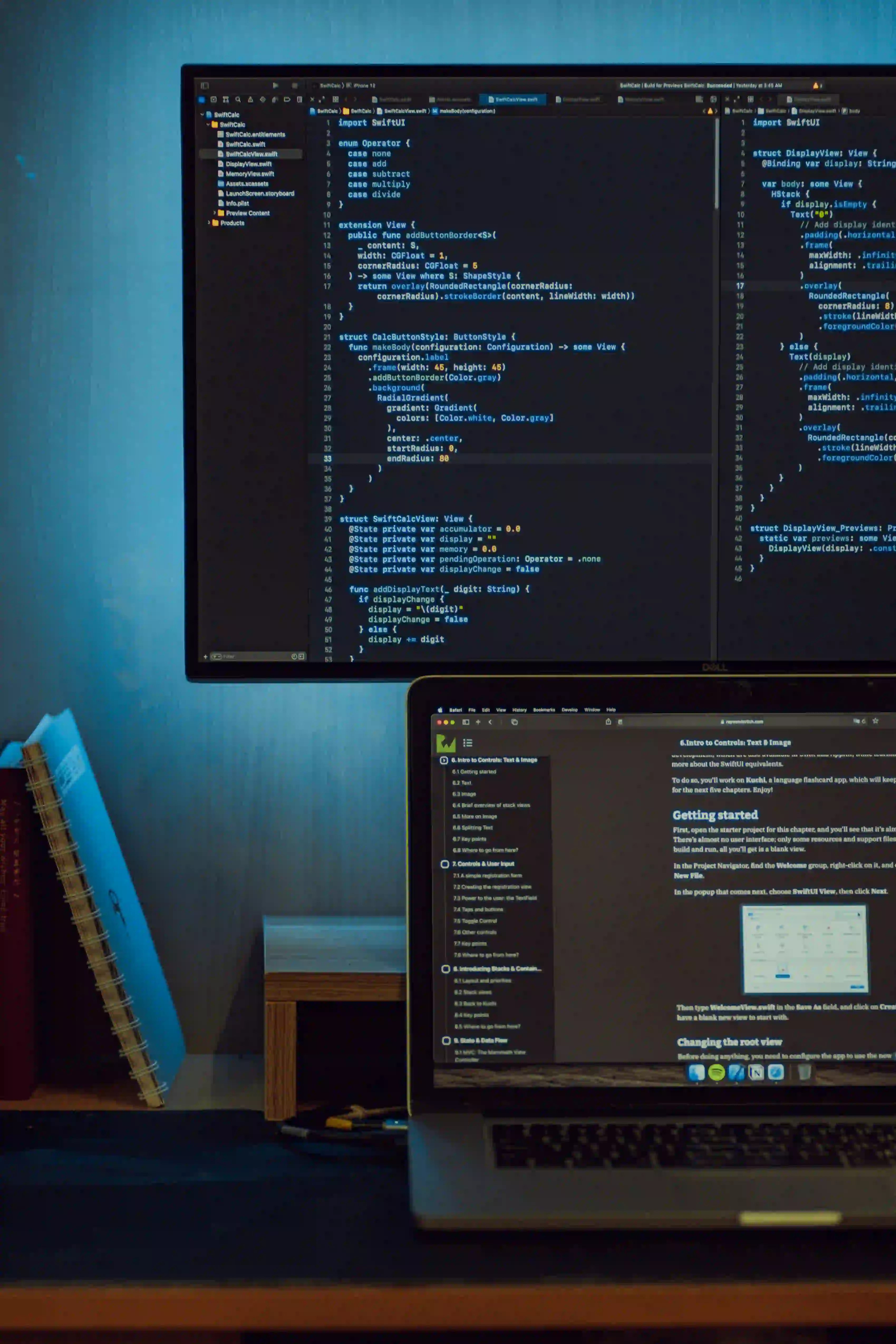
Navigating Localization Challenges in Spring 3 Internationalization
As businesses expand globally, the need for software applications to cater to different languages and cultures has become paramount. In Java, the Spring Framework provides robust tools for internationalization (i18n). In this blog post, we will delve into the intricacies of Spring 3 internationalization, address common localization challenges, and guide you through solutions with code snippets and explanations.
Understanding Internationalization and Localization
Internationalization (i18n) is the process of designing software applications so they can be adapted to various languages and regions without requiring changes to the source code. Localization (l10n), on the other hand, is the actual process of translating the application into different languages and adjusting it to fit local culture.
Spring offers a powerful i18n support system that simplifies the complexities of localization.
Setting Up Spring for Internationalization
To get started, ensure that you have the necessary dependencies in your pom.xml
if you are using Maven:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>3.2.18.RELEASE</version>
</dependency>
You can create properties files for different languages. For example:
messages.properties
(default)messages_en.properties
(for English)messages_fr.properties
(for French)
Within these files, you will define key-value pairs for your messages.
Example of a Messages Properties File
# messages.properties
greeting=Hello
welcome=Welcome to our application!
# messages_en.properties
greeting=Hello
welcome=Welcome to our application!
# messages_fr.properties
greeting=Bonjour
welcome=Bienvenue dans notre application!
Configuring the Locale Resolver
To enable localization, you'll need to set up a LocaleResolver
. In Spring MVC, this can be easily done using a LocalContextLoader
. Below is an example configuration in your WebMvcConfigurerAdapter
:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.ViewResolverRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
import org.springframework.web.servlet.i18n.LocaleChangeInterceptor;
import org.springframework.web.servlet.i18n.SessionLocaleResolver;
import java.util.Locale;
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Bean
public SessionLocaleResolver localeResolver() {
SessionLocaleResolver slr = new SessionLocaleResolver();
slr.setDefaultLocale(Locale.ENGLISH);
return slr;
}
// Additional configurations...
}
Why Set Default Locale?
Setting a default locale ensures that your application has a fallback language for users who do not specify their preferred language. This increases usability and accessibility of your application.
Implementing Locale Change Interceptor
To switch languages dynamically, you need a LocaleChangeInterceptor
. This interceptor captures user input and changes the locale accordingly.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.InterceptorRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(localeChangeInterceptor());
}
@Bean
public LocaleChangeInterceptor localeChangeInterceptor() {
LocaleChangeInterceptor lci = new LocaleChangeInterceptor();
lci.setParamName("lang");
return lci;
}
}
Why Use LocaleChangeInterceptor?
This provides a seamless way for users to change language preferences on the fly. Users can simply append the parameter ?lang=fr
or ?lang=en
at the end of your URL to switch languages.
Handling Date and Number Formats
Localization extends beyond simple text translation; it also encapsulates formatting. If your application handles dates and numbers, you should also consider localized formats.
You can leverage @InitBinder
to customize how date formats are handled:
import org.springframework.web.bind.WebDataBinder;
import org.springframework.web.bind.annotation.InitBinder;
import java.text.SimpleDateFormat;
import java.util.Date;
public class UserController {
@InitBinder
public void initBinder(WebDataBinder binder) {
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
dateFormat.setLenient(false);
binder.registerCustomEditor(Date.class, new CustomDateEditor(dateFormat, true));
}
}
Importance of Formatting
Different cultures have unique ways of representing numbers and dates. For example, the U.S. uses MM/DD/YYYY for dates, while many European countries utilize DD/MM/YYYY. Hence, adapting formats accordingly enhances user experience.
Managing Pluralization and Gender Forms
One of the challenges in localization arises with languages that have different grammatical structures, including pluralization and gender. Traditional properties files do not adequately address this.
Consider using ResourceBundleMessageSource
in your Spring configuration to manage these nuances. You can create pluralized keys in your properties files, though it might become cumbersome.
Example of Pluralized Messages
# messages_en.properties
item.one=There is one item.
item.few=There are few items.
item.many=There are many items.
# messages_fr.properties
item.one=Il y a un article.
item.few=Il y a quelques articles.
item.many=Il y a de nombreux articles.
How to Decide Which Message to Display
You can create a utility function to determine plurality based on count:
public String getItemMessage(int count, Locale locale) {
ResourceBundle messages = ResourceBundle.getBundle("messages", locale);
if (count == 1) {
return messages.getString("item.one");
} else if (count < 5) {
return messages.getString("item.few");
} else {
return messages.getString("item.many");
}
}
Testing and Debugging Localizations
Localization is a complex area that involves multiple variables and settings. Comprehensive testing is crucial. Here are some strategies:
- Unit Tests: Implement unit tests that validate the return values of your resource bundles based on locale.
- Integration Tests: Create real scenarios to test locale change functionality.
- User Feedback: Consider languages and cultural nuances by gathering feedback from native speakers.
Resources for Further Learning
- Spring Documentation on Internationalization
- Java Internationalization Guide
Wrapping Up
Navigating localization challenges in Spring 3 internationalization requires strategic planning and implementation. By leveraging properties files, locale resolvers, and innovative programming solutions, you can create a seamless user experience for multilingual users. Always remember that effective localization goes beyond mere translation; it embodies cultural sensitivity.
Implement these strategies in your next project, and watch as your application resonates across diverse audiences. Happy coding!