Struggling with Mockito Extra Interfaces? Here's How!
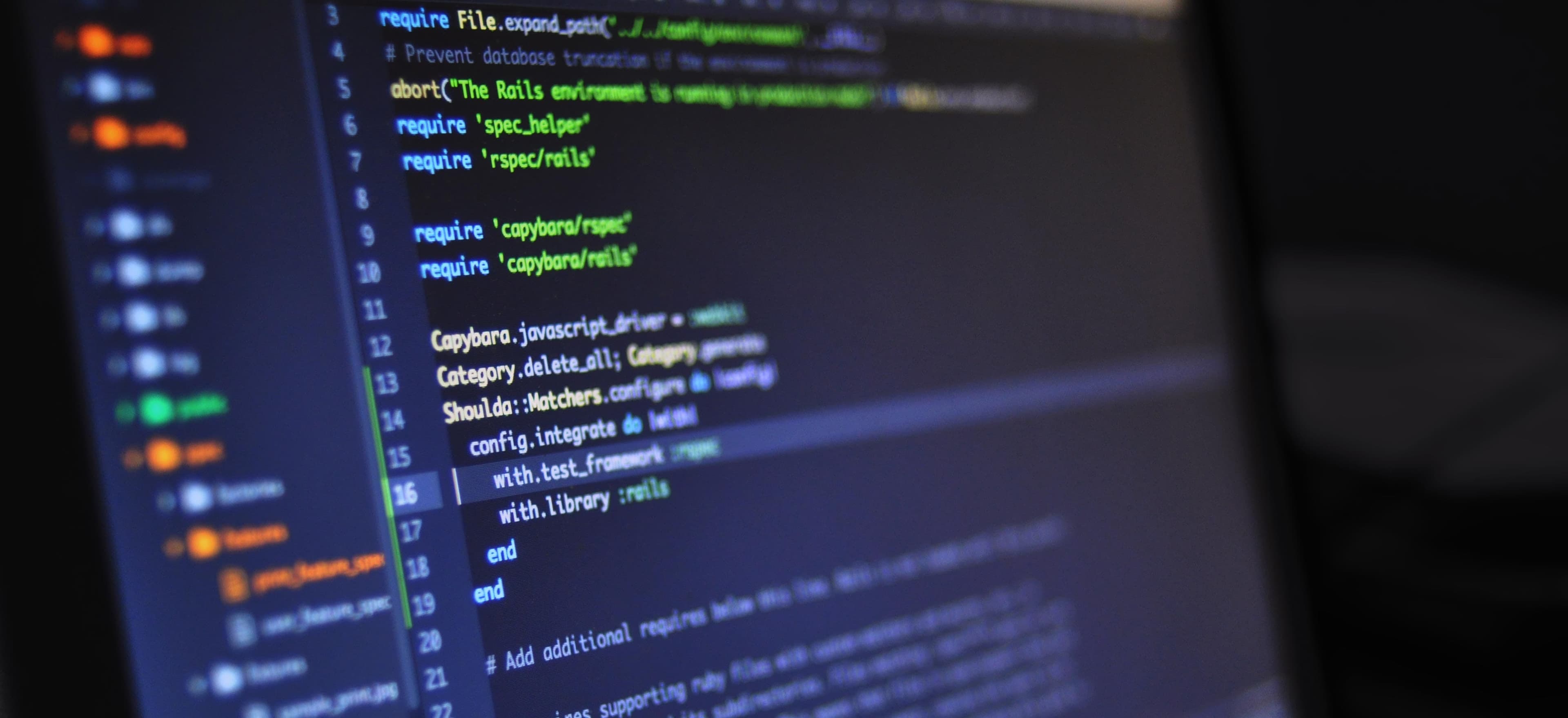
- Published on
Struggling with Mockito Extra Interfaces? Here's How!
When it comes to unit testing in Java, Mockito stands out as an indispensable tool for many developers. This popular mocking framework allows you to create test doubles, helping you isolate and test different components of your code. However, things can get tricky when you're dealing with extra interfaces. In this blog post, we'll explore how to effectively use Mockito with extra interfaces, discussing the why and providing practical examples.
What is Mockito?
Mockito is a mocking framework that allows developers to create and manipulate mock objects in order to test their Java code. It aims to streamline the process of testing by allowing you to focus on what to verify about a unit without having to invoke the underlying implementation details.
Why Use Mockito?
- Isolation: Focus on the specific unit in your tests.
- Simplicity: Write cleaner, more comprehensible tests.
- Flexibility: Mock complex systems with minimal setup.
For an in-depth understanding of Mockito, refer to the official Mockito Documentation.
Understanding Extra Interfaces in Mockito
What Are Extra Interfaces?
In Java, an interface defines a contract that classes can implement. Extra interfaces refer to the additional interfaces a class might implement beyond the main functionality. When testing, these extra interfaces can complicate your mocking setup.
Common Issues with Extra Interfaces
- Initial Complexity: Adding extra interfaces can lead to confusion regarding which methods to mock.
- Over-Mocking: Extra interfaces may tempt developers to mock too much, leading to overly complicated test cases.
- Inconsistent Behavior: The interaction between mocks and real objects can produce unexpected results if not handled correctly.
The Solution: Mockito's spy
and @Mock
Mockito provides tools like spy
and @Mock
to help you navigate this complexity.
Example Code Snippet
Let's look at an example where we have a PaymentProcessor
interface and an additional Logger
interface:
public interface PaymentProcessor {
void processPayment(double amount);
}
public interface Logger {
void log(String message);
}
public class PaymentService {
private final PaymentProcessor paymentProcessor;
private final Logger logger;
public PaymentService(PaymentProcessor paymentProcessor, Logger logger) {
this.paymentProcessor = paymentProcessor;
this.logger = logger;
}
public void makePayment(double amount) {
logger.log("Processing payment of $" + amount);
paymentProcessor.processPayment(amount);
logger.log("Payment processed");
}
}
Writing a Test with Mockito
Now, let's write a unit test for PaymentService
using Mockito:
import org.junit.jupiter.api.Test;
import org.mockito.ArgumentCaptor;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import static org.mockito.Mockito.*;
public class PaymentServiceTest {
@Mock
private PaymentProcessor paymentProcessor;
@Mock
private Logger logger;
private PaymentService paymentService;
@BeforeEach
public void setUp() {
MockitoAnnotations.openMocks(this);
paymentService = new PaymentService(paymentProcessor, logger);
}
@Test
public void testMakePayment() {
double paymentAmount = 100.0;
paymentService.makePayment(paymentAmount);
ArgumentCaptor<String> logCaptor = ArgumentCaptor.forClass(String.class);
verify(logger, times(2)).log(logCaptor.capture());
assertThat(logCaptor.getAllValues()).containsExactly(
"Processing payment of $100.0",
"Payment processed"
);
verify(paymentProcessor).processPayment(paymentAmount);
}
}
Explanation of the Code
- Mockito Annotations:
@Mock
marksPaymentProcessor
andLogger
for Mockito to instantiate them as mock objects. - Setup Method: The
setUp
method initializes the mocks and creates a new instance ofPaymentService
. - Test Method: In the
testMakePayment
, we:- Call the method
makePayment
. - Capture the log messages as they are logged.
- Verify that the payment processor's method was called once with the specified amount.
- Call the method
Why This Approach Works
This structure helps isolate the PaymentService
from its dependencies. By mocking the extra interfaces, you can focus on the core logic while ensuring that the interactions with the Logger
and PaymentProcessor
are correctly verified.
Advanced Mockito Techniques
To take your tests further, consider these advanced techniques:
Using spy
for Partial Mocks
When working with classes that have complex behavior you want to preserve in your tests, consider using spy
:
PaymentProcessor realProcessor = new RealPaymentProcessor(); // Real implementation
PaymentProcessor paymentProcessorSpy = spy(realProcessor);
doNothing().when(paymentProcessorSpy).sendReceipt(any());
The spy allows you to override only specific methods while keeping the default behavior for others. This is particularly useful if a method calls another method that you do not want to mock.
Handling Extra Method Calls
If an interface has many methods that you do not want to implement in your tests, consider using lenient()
:
lenient().when(logger.log(anyString())).thenReturn(null);
This informs Mockito to ignore any strict verification requirements for that specific method.
Final Considerations
Even though working with Mockito and extra interfaces may initially seem daunting, understanding the framework and utilizing its full capabilities can help alleviate those worries. The flexibility it offers, combined with the significant isolation and focus it provides during testing, makes it a powerful ally for Java developers.
For additional information, you can also explore the Mockito Cheat Sheet, which serves as a quick guide for common mocking patterns.
Happy testing, and may your unit tests always pass!
Checkout our other articles