Mastering Java 7 Try-With-Resources to Avoid Memory Leaks
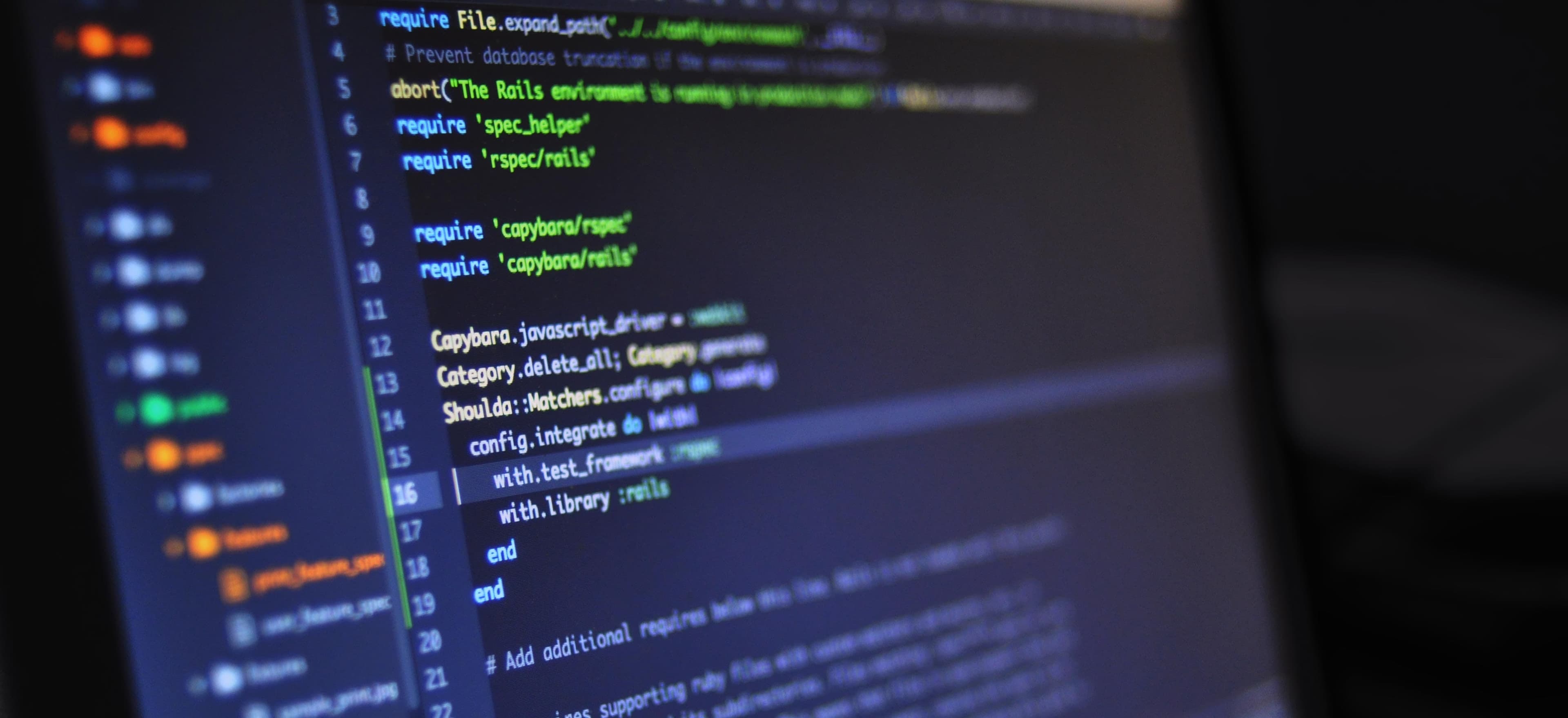
- Published on
Mastering Java 7 Try-With-Resources to Avoid Memory Leaks
Java has continually evolved to address issues that developers face in building robust applications. One notable enhancement introduced in Java 7 is the Try-With-Resources statement. This powerful feature is crucial for managing resources like file streams, database connections, and sockets effectively. By incorporating this feature into your programming practices, you can significantly reduce the risk of memory leaks and make your code cleaner and more efficient.
What is Try-With-Resources?
Try-With-Resources (TWR) is a language construct that manages the lifecycle of resources automatically. In Java, a resource is any object that implements the java.lang.AutoCloseable
interface. When a resource is declared in a try-with-resources statement, Java automatically closes the resource after the try block is executed, even if an exception occurs.
Here’s a simple syntax:
try (ResourceType resource = new ResourceType()) {
// Use the resource
} catch (ExceptionType e) {
// Handle exception
}
Why Use Try-With-Resources?
Before Java 7, developers had to manually close resources in a finally
block, leading to potential memory leaks if the closing method was not called properly. Here’s why TWR is a better option:
- Automatic Resource Management: TWR reduces the boilerplate code associated with closing resources.
- Exception Safety: Even when exceptions are thrown, TWR guarantees that resources are closed.
- Readability and Maintainability: Code becomes cleaner and easier to understand.
Example: Reading a File with Try-With-Resources
Let’s consider an example of reading content from a text file using TWR. Here’s a solution without using TWR:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadWithoutTWR {
public static void main(String[] args) {
BufferedReader reader = null;
try {
reader = new BufferedReader(new FileReader("sample.txt"));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (reader != null) {
try {
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
Commentary on Code
- Manual Resource Handling: We create a
BufferedReader
and handle multiple potential points of failure. - Potential Memory Leak: If an exception occurs in the try block, the reader may not close, leading to a memory leak.
Now, let’s improve this code using Try-With-Resources.
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileReadWithTWR {
public static void main(String[] args) {
try (BufferedReader reader = new BufferedReader(new FileReader("sample.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why This Works
- Automatic Resource Management: The
BufferedReader
will be closed automatically at the end of the try block. - Clearer Code: Less boilerplate code enhances readability and allows developers to focus on the business logic.
Nested Resources in Try-With-Resources
You can also declare multiple resources within a single try-with-resources statement:
try (
BufferedReader reader = new BufferedReader(new FileReader("sample.txt"));
PrintWriter writer = new PrintWriter(new FileWriter("output.txt"))
) {
String line;
while ((line = reader.readLine()) != null) {
writer.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
Key Features of This Code
- Multiple Resources: Two resources are managed automatically within the same try block.
- Simplicity: By handling multiple resources together, code complexity is greatly reduced.
Exception Propagation in Try-With-Resources
When exceptions occur both in the try block and while closing resources, TWR handles this gracefully by propagating the exception from the try block and suppressing the exception generated from closing the resources. Here's how you can check the suppressed exceptions:
try (BufferedReader reader = new BufferedReader(new FileReader("sample.txt"))) {
String line;
while ((line = reader.readLine()) != null) {
// Simulate an exception
if (line.equals("throw")) throw new IOException("Simulated Exception");
}
} catch (IOException e) {
System.err.println(e.getMessage());
for (Throwable t : e.getSuppressed()) {
System.err.println("Suppressed: " + t.getMessage());
}
}
What This Code Does
- Exception Handling: It manages exceptions both during reading and closing smoothly.
- Suppressed Information: It helps debug by providing information about suppressed exceptions that might occur during resource closure.
Common Use Cases for Try-With-Resources
- File I/O Operations: Reading from and writing to files.
- Database Connections: Managing JDBC connections seamlessly.
- Socket Connections: Handling network resources in network applications.
Final Considerations
Java's Try-With-Resources statement is a critical addition for resource management, especially in preventing memory leaks. If you aren’t already using TWR, it’s time to incorporate it into your coding practices.
Make your code cleaner, safer, and more maintainable with TWR. Whether you’re managing file I/O, database connectivity, or other critical resources, the automatic management provided by TWR can save you from potential headaches down the line.
For more detailed insights on managing resources effectively in Java, check out the Oracle documentation on exceptions.
Start implementing Try-With-Resources today, and embrace cleaner coding!
This blog post targets Java developers, aiming for SEO optimization while informing readers about the crucial features of Java 7’s TWR. The structure and content prioritize clarity and practical understanding, making it easier for readers to grasp and apply the discussed concepts.
Checkout our other articles