PermGen Space Exhaustion: The Hidden JVM Challenge
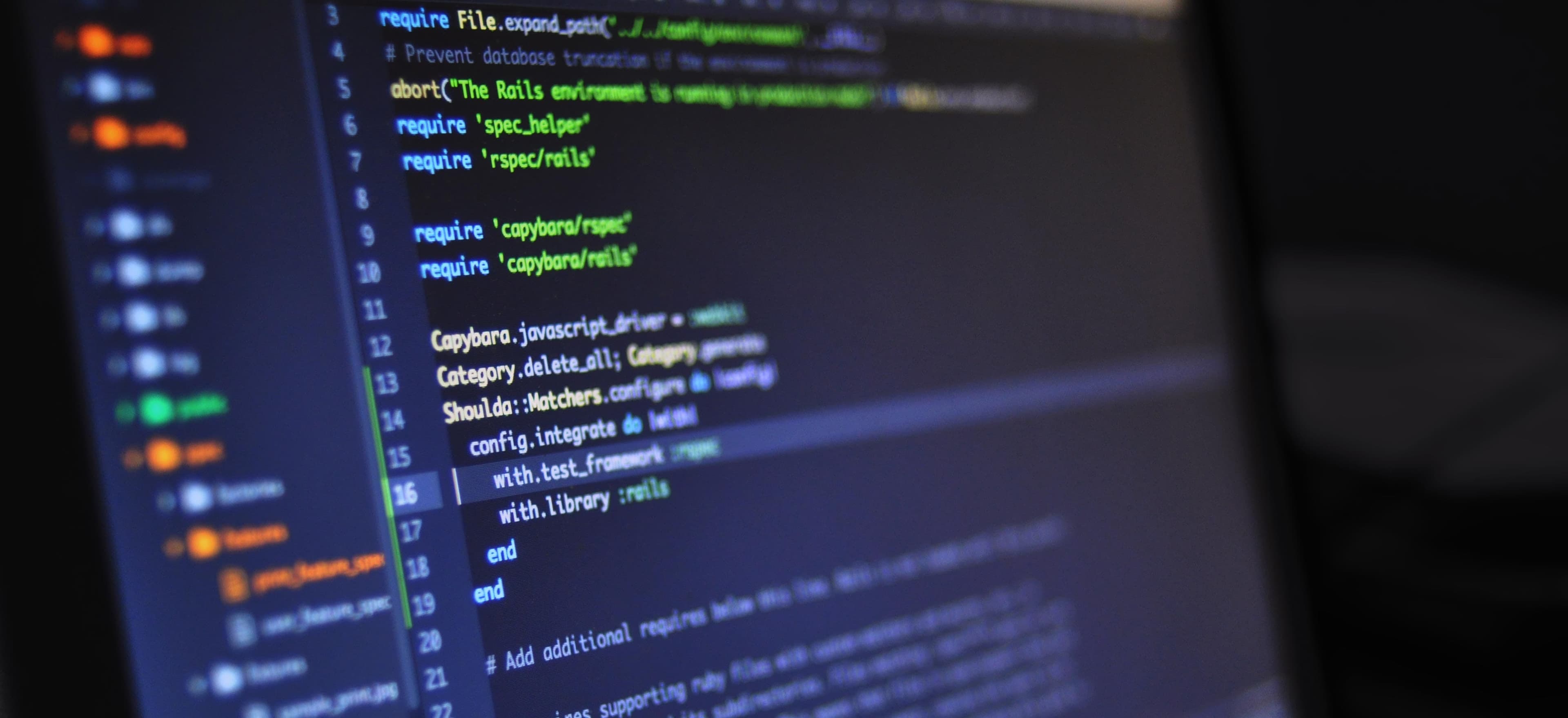
- Published on
PermGen Space Exhaustion: The Hidden JVM Challenge
The Java Virtual Machine (JVM) is a marvel of modern computing, allowing developers to run Java applications across different platforms seamlessly. However, as developers dive deeper into optimizing and maintaining their applications, they may encounter hidden challenges lurking in the JVM, one of which is PermGen Space Exhaustion.
In this blog post, we will explore what PermGen space is, the problems associated with its exhaustion, and how to mitigate these challenges effectively.
Understanding PermGen Space
Before we address exhaustion, let's clarify what PermGen space is. It stands for Permanent Generation, and it's a part of the JVM memory structure used to store metadata related to classes and methods. In simpler terms, PermGen space holds the information the JVM needs to understand the various classes that are loaded at runtime. This includes:
- Class definitions
- Methods
- Static fields
- Some interned strings
Why is PermGen Space Important?
PermGen space is critical for application performance. When a Java application runs, it dynamically loads classes into memory. If there's insufficient space in PermGen, the JVM will not be able to load new classes, leading to errors that can bring your application to a halt.
The Challenge of PermGen Space Exhaustion
When an application continually loads and unloads classes, the use of PermGen space can become a bottleneck. If it fills up, you might see an error message such as:
java.lang.OutOfMemoryError: PermGen space
This typically occurs in applications with:
- Dynamic Class Loading: Frameworks like Spring and Hibernate commonly load classes dynamically.
- Large Applications: Applications with many libraries and dependencies can strain the PermGen space.
Example Scenario
Consider a web application using Spring MVC and Hibernate. If you deploy this web application multiple times, the old class loaders may not be eliminated immediately, consuming PermGen space with each deployment.
@Configuration
@EnableWebMvc
public class WebConfig extends WebMvcConfigurerAdapter {
// Your configuration here
}
In this case, if the application restarts frequently, each restart adds overhead to PermGen, eventually leading to memory exhaustion.
Solutions to PermGen Space Exhaustion
Fortunately, there are various ways to manage and mitigate PermGen space exhaustion.
1. Increasing PermGen Space
A straightforward solution is to increase the PermGen space. This can be done by setting JVM startup options. For example, use the -XX:PermSize
and -XX:MaxPermSize
flags to define the initial and maximum PermGen sizes, respectively.
java -XX:PermSize=256m -XX:MaxPermSize=512m -jar yourapp.jar
-XX:PermSize=256m
: Sets the initial PermGen space to 256 MB.-XX:MaxPermSize=512m
: Sets the maximum PermGen space to 512 MB.
This method may provide temporary relief, but it doesn’t solve the underlying problem of class loading.
2. Avoiding Classloader Leaks
Classloader leaks occur when old classloader instances are not properly garbage collected, often due to static references that persist and prevent the old classloader from being recycled.
To avoid leaks:
- Use weak references for static fields that hold class instances.
- Ensure that listeners and other resources are properly unregistered on application shutdown.
Here’s an example of cleaning up resources properly:
public class MyServletContextListener implements ServletContextListener {
@Override
public void contextDestroyed(ServletContextEvent sce) {
// Clean up resources here.
MyService myService = (MyService) sce.getServletContext().getAttribute("myService");
if (myService != null) {
myService.cleanup();
}
}
}
3. Use Java 8 or Higher (Metaspace)
Starting with Java 8, the JVM replaced PermGen with Metaspace, which grows automatically as needed and is no longer limited by -XX:MaxPermSize
. If you're still using Java 7 or earlier, consider upgrading your Java version to resolve PermGen space exhaustion issues.
For those still using older versions of Java, consider migrating as follows:
- Review your application for compatibility.
- Test your application thoroughly after the migration effort.
4. Optimize Libraries and Frameworks
If you're using numerous libraries, each loading its own classes, it may be worthwhile to:
- Refactor your code to reduce dependencies.
- Use dependency management tools like Maven or Gradle to remove unnecessary libraries.
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.0.RELEASE</version>
<scope>compile</scope>
</dependency>
Ensure that your libraries are up to date and you're not loading outdated or unused dependencies.
5. Monitor and Profile PermGen Usage
Monitoring tools can provide insights into how PermGen space is utilized in your applications. Utilizing tools like Java VisualVM or Eclipse Memory Analyzer can help identify classloader leaks and memory consumption patterns in real-time.
You can integrate tools like JConsole or use profilers for a more thorough analysis.
Bringing It All Together
PermGen space exhaustion can pose significant challenges for Java applications, particularly for those that employ complex frameworks or frequently deploy updated instances. By understanding PermGen's purpose and carefully managing classloading, developers can optimize application performance significantly.
Moreover, the transition to newer Java versions, focusing on resource management, and leveraging monitoring tools will create a more robust Java application environment.
Overall, the goal should be not just to avoid PermGen space exhaustion but also to create efficient, maintainable, and high-performance Java applications.
For further reading on JVM performance tuning, the official Java documentation offers comprehensive insights and guidance.