Navigating the Pitfalls of Optional Dependencies in Projects
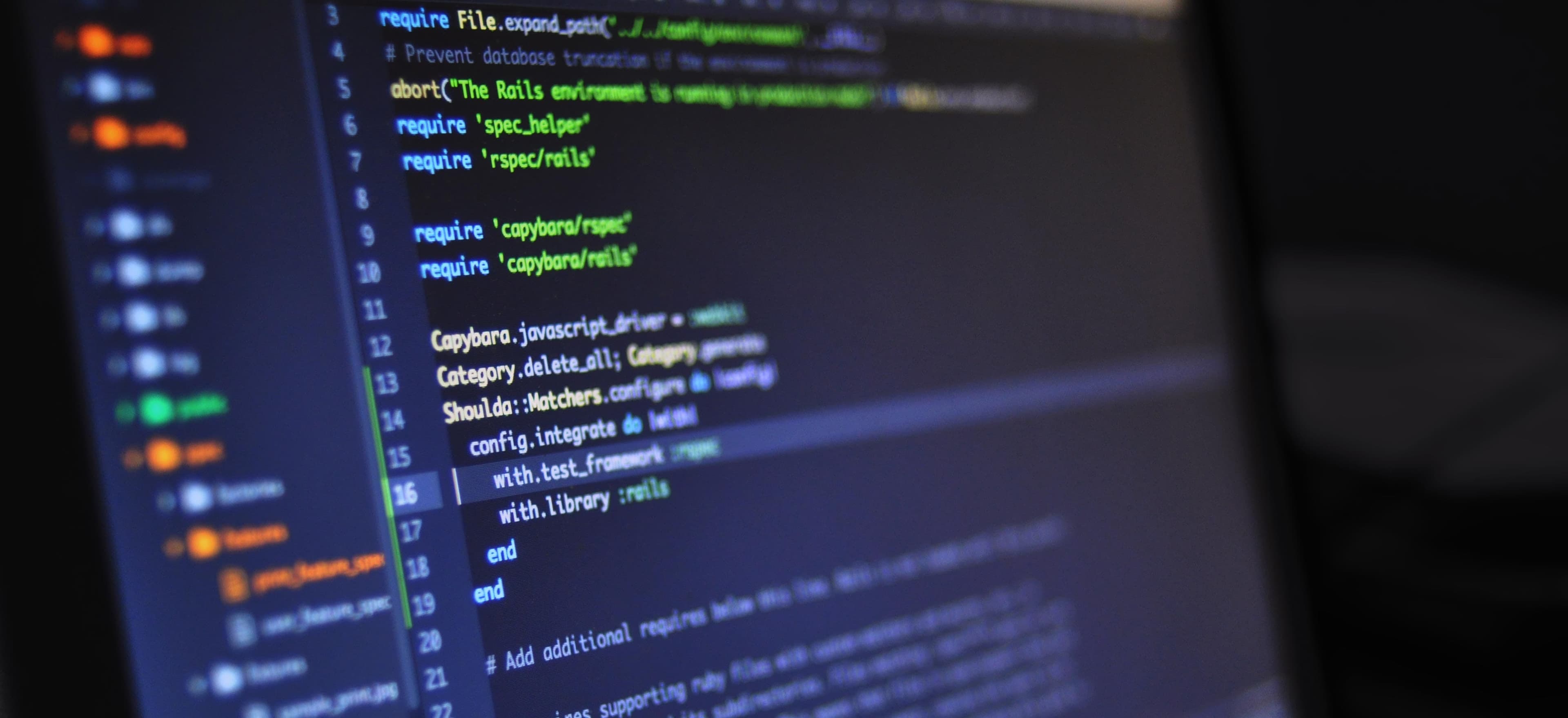
- Published on
Navigating the Pitfalls of Optional Dependencies in Projects
Managing dependencies in software projects is a critical task for developers. While leveraging dependencies can significantly accelerate development and enhance functionality, they can also introduce complexities. Optional dependencies, in particular, pose unique challenges. In this blog post, we'll explore the nature of optional dependencies, their benefits, and pitfalls, and provide best practices to manage them effectively.
What Are Optional Dependencies?
Optional dependencies are libraries or modules that your project can use but are not essential for it to function. They help extend functionality, but the main project remains operational without them. Optional dependencies may be utilized for features that are not crucial to the core functionality of your application.
For example, a web application may optionally use a third-party library for user analytics, but the application can work perfectly fine without it.
Benefits of Optional Dependencies
-
Enhanced Functionality: They allow developers to include features without making them mandatory.
-
Lower Initial Complexity: Projects can be simpler and lighter when optional dependencies are not included.
-
Flexibility: Developers can choose which features to incorporate based on project requirements or deployment scenarios.
Downsides of Optional Dependencies
Despite their benefits, optional dependencies can lead to several pitfalls:
-
Inconsistent Environments: If different environments (development, staging, production) have varying sets of optional dependencies, it can lead to unexpected errors.
-
Documentation Overhead: Developers must document and explain to users which optional dependencies are needed for specific features, adding complexity.
-
Version Conflicts: Optional dependencies can lead to conflicts with other modules, causing runtime issues or application crashes.
-
Performance Overhead: Loading more libraries than necessary can increase the application’s memory footprint and loading time.
Best Practices for Managing Optional Dependencies
Now that we understand the risks associated with optional dependencies, let's discuss effective strategies to manage them.
1. Use Clear Documentation
Why? Clear documentation helps users and developers understand which optional dependencies are required for specific features.
# Documentation Example
### Optional Dependencies
The following libraries are needed for enhanced functionality in our application:
- `analytics-library`: Required for user analytics features.
- `extra-logging`: For additional logging capabilities.
To install, run:
npm install analytics-library extra-logging
This provides a straightforward guideline on what to include for optimal functionality without overwhelming users with complexity.
### 2. Version Control with Dependency Managers
**Why?** Utilize package managers (like Maven for Java, npm for Node.js) to manage versions of optional dependencies effectively.
For Java projects, using Maven's `pom.xml` to define optional dependencies makes version management easier. Below is an example:
```xml
<dependency>
<groupId>com.example</groupId>
<artifactId>analytics-library</artifactId>
<version>1.0.0</version>
<optional>true</optional>
</dependency>
In this snippet:
- The
optional
tag explicitly marks the dependency as optional. - When the project is built, it won’t be included unless explicitly requested.
3. Conditional Loading in Code
Why? Consider loading optional dependencies conditionally within your code. This approach prevents loading unnecessary resources.
In a Java application, you might check if a feature is enabled before loading the optional library:
class Analytics {
private Optional<AnalyticsLibrary> analyticsLibrary;
public Analytics(boolean isEnabled) {
if (isEnabled) {
// Attempting to load optional library conditionally
try {
this.analyticsLibrary = Optional.of(new AnalyticsLibrary());
} catch (LibraryNotFoundException e) {
this.analyticsLibrary = Optional.empty(); // Handle absence gracefully
System.out.println("Optional library not found. Analytics will be disabled.");
}
} else {
this.analyticsLibrary = Optional.empty();
}
}
public void trackEvent(String event) {
analyticsLibrary.ifPresent(lib -> lib.trackEvent(event));
}
}
In this example:
- The optional library is only loaded if analytics is enabled.
Optional.empty()
handles the scenario where the library isn't available.
4. Consistent Environment Setup
Why? Maintain consistent environments across development, staging, and production to avoid unexpected issues.
Utilize environment configuration files (like .env
) to manage optional dependencies. This helps ensure all developers and deployment environments are aligned.
# .env file
ANALYTICS_ENABLED=true
When the application starts, it can read this environment variable to determine whether to load optional dependencies.
5. Monitor Dependency Updates
Why? Regularly update optional dependencies to their latest versions. This ensures you benefit from performance improvements and security patches while minimizing issues.
Use tools such as Dependabot, or for Java projects, Maven’s versions-maven-plugin
, which help you keep track of dependency versions.
My Closing Thoughts on the Matter
Optional dependencies can add significant value to software projects, but they come with their share of pitfalls. By implementing best practices—such as clear documentation, version control, conditional loading, environment consistency, and regular monitoring—you can mitigate the risks and leverage optional dependencies effectively.
To dive deeper into dependency management, check out resources like Maven's Official Documentation or explore Gradle's Dependency Management.
By navigating the pitfalls of optional dependencies wisely, you can enhance your project’s functionality while maintaining maintainability and performance.
This post aimed to provide valuable insights into managing optional dependencies effectively. With these strategies, you're well-equipped to tackle the challenges they present. Happy coding!