Handling Errors in Non-Blocking Async Java 8 with Scala
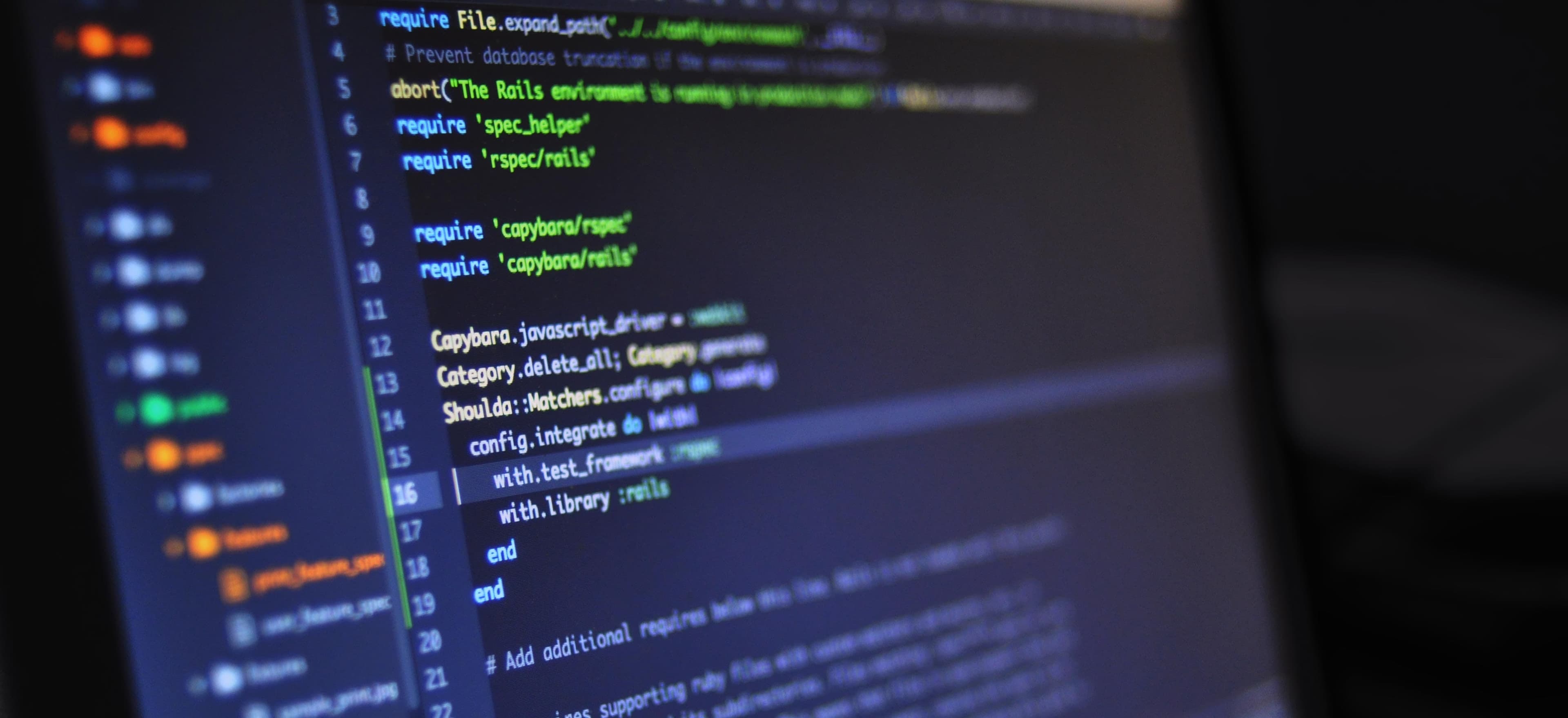
- Published on
Handling Errors in Non-Blocking Async Java 8 with Scala
In today’s programming landscape, the need for responsive, non-blocking systems has become paramount. Java 8, with its introduction of the CompletableFuture
class, provides robust support for asynchronous programming. However, as we delve deeper into the realm of asynchronous programming, we also encounter the challenge of error handling. This blog post will explore how to manage errors in non-blocking asynchronous Java 8 code using Scala principles.
Understanding Non-Blocking Asynchronous Programming
What is Non-Blocking Asynchronous Programming?
In non-blocking asynchronous programming, a thread can initiate a task and then continue executing other work without waiting for the task to complete. This is particularly beneficial in applications that perform I/O operations, as it allows resources to be utilized more efficiently.
Java 8's CompletableFuture
allows you to compose and handle various asynchronous operations. With the advent of functional programming features, it incorporates a cleaner approach to writing asynchronous code.
Why Use Scala Concepts in Java?
Scala offers an intuitive functional programming model, allowing developers to effectively handle errors using constructs like Try
, Future
, and Promise
. By leveraging these concepts while dealing with Java's CompletableFuture
, we can achieve elegance and robustness in error handling.
Basics of CompletableFuture in Java 8
Let’s start by creating a simple asynchronous task using CompletableFuture
.
import java.util.concurrent.CompletableFuture;
public class AsyncExample {
public static void main(String[] args) {
CompletableFuture<Integer> future = CompletableFuture.supplyAsync(() -> {
if (Math.random() > 0.5) {
throw new RuntimeException("Random failure occurred.");
}
return 42;
});
future.thenAccept(result -> System.out.println("Result: " + result))
.exceptionally(ex -> {
System.err.println("Error: " + ex.getMessage());
return null;
});
}
}
In this example:
- We create a
CompletableFuture
that simulates an asynchronous computation, which randomly throws an exception. - By utilizing
thenAccept
, we process the result if the computation is successful. - The
exceptionally
block handles exceptions, allowing us to manage errors in a non-blocking fashion.
Advanced Error Handling Strategies
As our applications grow in complexity, our error handling strategies must too. Let's break down various strategies for effective error handling in asynchronous Java.
1. Using whenComplete
The whenComplete
method allows you to execute some code after a completion of the future, regardless of whether it completed normally or exceptionally.
future.whenComplete((result, ex) -> {
if (ex != null) {
System.err.println("Error: " + ex.getMessage());
} else {
System.out.println("Completed with result: " + result);
}
});
This block runs irrespective of the outcome. It’s essential for logging or clean-up operations, ensuring that we respond to both success and failure.
2. Chaining CompletableFutures
Sometimes operations depend on the completion of previous tasks. The power of thenCompose
and thenCombine
methods can help us handle such cases.
CompletableFuture<Integer> futureTask = CompletableFuture.supplyAsync(() -> {
if (Math.random() > 0.5) {
throw new RuntimeException("Error in first task");
}
return 10;
});
CompletableFuture<Integer> combinedTask = futureTask
.thenCompose(result -> CompletableFuture.supplyAsync(() -> {
if (result < 5) {
throw new RuntimeException("Result too low");
}
return result * 2;
}))
.exceptionally(ex -> {
System.err.println("Error in processing: " + ex.getMessage());
return -1; // Returning a default value in case of error
});
Here we leverage thenCompose
to chain a second asynchronous task that depends on the result of the first. This way, we can have a more granular level of error handling through chained futures.
3. Using Scala's Try and Future for Error Management
If you're familiar with Scala, you might find using Scala’s Try
in conjunction with Java’s CompletableFuture
very beneficial. The Try
monad can capture the result of a computation that might fail without throwing an exception.
First, let’s convert a Java CompletableFuture to Scala’s Future using the scala.compat.java8.FutureConverters
:
import scala.concurrent.Future
import scala.compat.java8.FutureConverters._
def convertToScalaFuture(javaFuture: CompletableFuture[Int]): Future[Int] = {
javaFuture.toScala
}
Now, using Scala's Try
, we can handle errors more elegantly:
import scala.util.{Try, Success, Failure}
val javaFuture: CompletableFuture[Int] = CompletableFuture.supplyAsync(() => 50 / 0) // intentionally dividing by zero
val scalaFuture: Future[Int] = convertToScalaFuture(javaFuture)
scalaFuture.onComplete {
case Success(value) => println(s"Result: $value")
case Failure(exception) => println(s"Error: ${exception.getMessage}")
}
By converting the CompletableFuture
into a Future
, we gain access to Scala's powerful error handling capabilities while still conducting asynchronous operations defined in Java.
The Bottom Line: Combining Paradigms for Robust Error Handling
Handling errors in non-blocking asynchronous programming can be challenging, especially when combining paradigms from two different languages like Java and Scala. However, employing strategies from both CompletableFuture
and Scala’s error handling mechanisms allows developers to create applications that are not only responsive but also resilient to failures.
Integrating classic Java asynchronous practices with Scala's elegant handling of errors can help ensure that your applications remain robust, thus delivering a superior experience for users. Whether you choose to leverage whenComplete
, exceptionally
, or the Scala Try
monad, the goal remains the same: to ensure that your code can gracefully cope with the uncertainties of asynchronous programming.
For further details, you can refer to the official documentation for CompletableFuture and Scala Futures.
Keep experimenting and exploring the possibilities of error handling in asynchronous programming to deliver event-driven and fault-tolerant systems. Happy coding!
Checkout our other articles