Solving Common Mistakes in Java Array Sorting
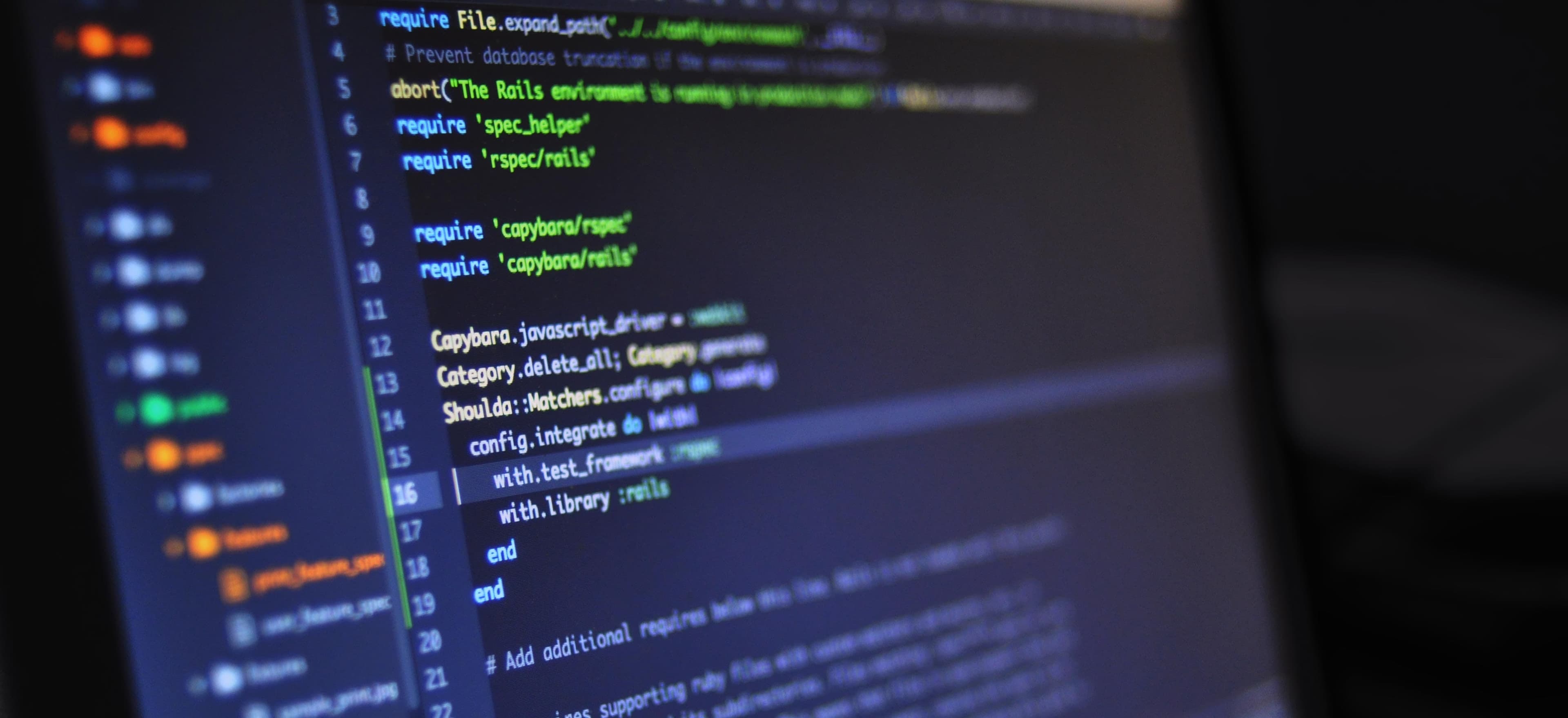
- Published on
Solving Common Mistakes in Java Array Sorting
Sorting is a fundamental operation in programming, essential for organizing data efficiently. In Java, arrays are often used to store collections of data that need to be sorted. However, many developers, especially beginners, make mistakes when implementing sorting algorithms for arrays. This blog post will aim to identify common pitfalls and provide solutions for them while using both built-in Java methods and custom sorting algorithms.
Understanding Arrays in Java
Before diving into sorting, let's briefly review what arrays are. An array in Java is a collection of elements, all of the same type, accessible via an index. They are a fundamental data structure but can be tricky to handle when it comes to sorting.
Basic Array Declaration
A basic array can be declared and initialized as follows:
int[] numbers = {5, 3, 8, 1, 2};
In this example, we have an integer array numbers
with five elements.
Mistake #1: Not Understanding In-Place Sorting
A common mistake is not realizing that some sorting algorithms sort the elements in place, while others require additional memory. In-place sorting means that the original array is modified directly, requiring no extra storage space.
Example of In-Place Sorting with Selection Sort
Selection sort is a simple comparison-based sorting algorithm. Here's an implementation with commentary:
public static void selectionSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
int minIdx = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIdx]) {
minIdx = j;
}
}
// Swap the found minimum element with the first element
if (minIdx != i) {
int temp = arr[i];
arr[i] = arr[minIdx];
arr[minIdx] = temp;
}
}
}
Why This Works
In this code, we iterate through the array, selecting the minimum element from the unsorted portion of the array and swapping it with the first unsorted element. This sorting method changes the original array, which is efficient in terms of space but can be slow for large data sets.
Mistake #2: Using the Wrong Comparison Logic
When implementing sorting algorithms, especially custom ones, developers often misconfigure the comparison logic. This can lead to incorrect sorting orders.
Example with Bubble Sort
Here is a bubble sort example that showcases effective comparison logic:
public static void bubbleSort(int[] arr) {
int n = arr.length;
boolean swapped;
for (int i = 0; i < n - 1; i++) {
swapped = false;
for (int j = 0; j < n - i - 1; j++) {
// Swap if the elements are out of order
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
swapped = true;
}
}
// If no two elements were swapped in the inner loop, then break
if (!swapped) break;
}
}
Why This Works
The bubbleSort
function compares adjacent elements and swaps them if they're in the wrong order, which effectively "bubbles" the largest element to the end of the array. The inclusion of the swapped
flag improves efficiency by terminating early if no swaps are made in a complete pass.
Built-In Sorting with java.util.Arrays
Java's standard library provides efficient sorting through the Arrays.sort()
method. It is highly optimized and handles various data types, eliminating many common pitfalls.
Example of Using Arrays.sort()
import java.util.Arrays;
public class SortExample {
public static void main(String[] args) {
int[] numbers = {5, 3, 8, 1, 2};
Arrays.sort(numbers);
System.out.println(Arrays.toString(numbers));
}
}
In diesem Beispiel wird Arrays.sort()
aufgerufen, um das Array numbers
schnell zu sortieren. It handles comparison logic internally, minimizing the risks of errors.
Mistake #3: Overlooking Edge Cases
Ignoring edge cases, such as empty arrays or arrays with a single element, can lead to unexpected behavior. These scenarios won't usually cause errors but can result in inefficient or incorrect behavior.
How to Handle Edge Cases
Before performing any sorting operations, check if the array is null or has fewer than two elements:
public static void sortIfNeeded(int[] arr) {
if (arr == null || arr.length < 2) {
return; // No need to sort
}
Arrays.sort(arr);
}
Why This Matters
This approach prevents unnecessary charges and ensures that the function behaves correctly no matter the input.
Resources for Further Learning
For those looking to deepen their understanding of sorting algorithms in Java, two excellent resources are:
Closing the Chapter
Sorting arrays in Java doesn't have to be a daunting task. Understanding basic array manipulation, recognizing common mistakes, and utilizing robust methods like Arrays.sort()
can significantly improve your coding efficiency. Whether you choose to implement sorting algorithms yourself or leverage built-in capabilities, taking care to consider edge cases and correct comparison logic will lead to more reliable code.
By learning from these common mistakes, you can enhance your skills in Java and build robust applications with confidence. Happy coding!
Checkout our other articles