Overcoming Compatibility Issues in Marshmallow App Development
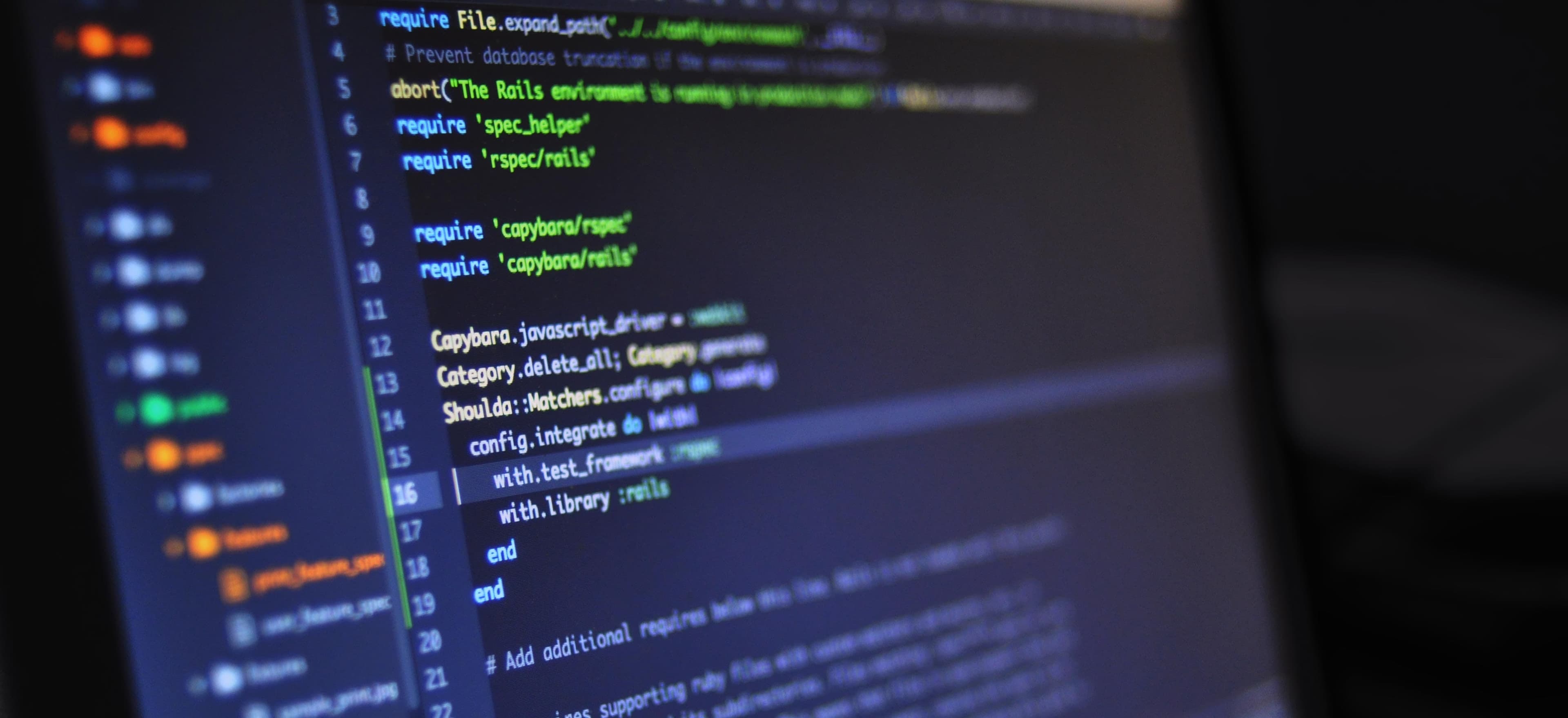
- Published on
Overcoming Compatibility Issues in Marshmallow App Development
As Android developers, we are constantly navigating the complex landscape of version compatibility. With the release of Android Marshmallow (API Level 23), developers faced new challenges and features that could potentially lead to incompatibility issues with existing apps. In this blog post, we'll discuss common compatibility issues with Android Marshmallow, and how you can effectively address them in your app development process.
Understanding Android Permissions
One of the most significant changes introduced in Marshmallow is the revamped permissions model. In previous Android versions, permissions were declared in the manifest file and granted at installation. However, Marshmallow introduced runtime permissions, which require developers to request certain permissions at runtime.
Why It Matters
For users, this means greater control over what data and features apps can access. For developers, it adds a layer of complexity to ensure that your app functions correctly when permissions are denied or not granted yet.
Implementing Runtime Permissions
Here’s a step-by-step example of how you can request permissions at runtime:
if (ContextCompat.checkSelfPermission(this, Manifest.permission.READ_CONTACTS) != PackageManager.PERMISSION_GRANTED) {
// Permission is not granted; request it
ActivityCompat.requestPermissions(this, new String[]{Manifest.permission.READ_CONTACTS}, MY_PERMISSIONS_REQUEST_READ_CONTACTS);
} else {
// Permission has already been granted; proceed with accessing contacts
loadContacts();
}
In the code above, we first check if the permission to read contacts is granted. If not, we request it. If it is granted, we can safely load the contacts. This approach ensures a smooth user experience while adhering to the new permissions model.
Handling Permission Results
Once you request permissions, you need to handle the user’s response. Override onRequestPermissionsResult
to manage this:
@Override
public void onRequestPermissionsResult(int requestCode, String[] permissions, int[] grantResults) {
switch (requestCode) {
case MY_PERMISSIONS_REQUEST_READ_CONTACTS:
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
// Permission granted; access contacts
loadContacts();
} else {
// Permission denied; notify user
Toast.makeText(this, "Permission denied to read contacts", Toast.LENGTH_SHORT).show();
}
break;
}
}
In this snippet, we check if the user granted the permission. Based on that, you can proceed with the desired functionality or notify the user appropriately. Effective handling of permissions is crucial in ensuring a seamless user experience.
Device Storage Changes
Another area where compatibility issues can arise is device storage, particularly with the introduction of the Scoped Storage
model in Android Marshmallow. This model confines your app's access to only its specific directories, enhancing security and privacy.
Why Scoped Storage?
Scoped storage limits access to only your app's files, which prevents potential data breaches. However, it may cause issues if your app relies on external storage.
Code Implementation
To adapt to scoped storage, modify how you access your app's directories:
File photoDir = new File(getExternalFilesDir(null), "MyPhotos");
if (!photoDir.exists()) {
photoDir.mkdirs(); // Create the directory if it does not exist
}
By using getExternalFilesDir(null)
, your app can create and access a private directory within external storage. This change simplifies file management while complying with the new security standards.
Utilizing the New Features of Marshmallow
While there are challenges in adapting to Marshmallow, remember that it also brings valuable enhancements. For example, features like Doze Mode and App Standby have been implemented to improve battery life.
Understanding Doze and App Standby
- Doze Mode restricts background tasks and network access when the phone is not being used, thus conserving battery.
- App Standby limits the actions of infrequently used apps, achieving a balance between performance and battery efficiency.
Implementing WorkManager
To address the intricacies of these features, it’s a good practice to use the WorkManager
API for background tasks, which is optimized to handle conditions such as Doze Mode:
WorkManager workManager = WorkManager.getInstance(context);
OneTimeWorkRequest uploadWorkRequest = new OneTimeWorkRequest.Builder(UploadWorker.class).build();
workManager.enqueue(uploadWorkRequest);
In this case, WorkManager
ensures that your background tasks are handled appropriately, complying with any power-saving restrictions in play.
UI Compatibility
With Marshmallow, there are also updates to UI components that can lead to incompatibility issues if overlooked. Ensuring your app is displayed correctly on various device configurations becomes critical.
Using Styles and Themes
Make sure you're employing the latest styles and themes introduced in Marshmallow:
<resources>
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
</resources>
This enables your app to stay visually consistent across different devices and Android versions.
Testing on Multiple Devices
Testing is crucial when dealing with compatibility issues. It is advisable to test your application on multiple devices running different versions of Android, especially if you target a broad audience.
Use Android Emulator
The Android Emulator lets you replicate various device configurations, including different Android versions and screen sizes, which leads to more reliable results in your testing:
- Open Android Studio.
- Click on the AVD Manager.
- Create a new virtual device selecting multiple configurations.
Incorporate Continuous Integration
Consider employing continuous integration (CI) tools like Jenkins, Travis CI, or GitHub Actions to automate testing across all supported Android versions. This ensures that any compatibility issues are detected early in the development process.
A Final Look
In conclusion, overcoming compatibility issues in Marshmallow app development is not insurmountable. By updating to the new permissions model, accommodating scoped storage, leveraging new background processing APIs, ensuring UI consistency, and thoroughly testing your applications, you can create robust and user-friendly Android applications.
For further reading, you can explore the official Android developer documentation and familiarize yourself with the Android Support Library to ease the transition between different Android versions.
By adhering to these practices, you not only comply with Marshmallow requirements but also set up your app for success in future Android environments. Embrace adaptation as a means for growth, and keep developing!
Happy coding!
Checkout our other articles