Top Selenium 4 Challenges and How to Overcome Them
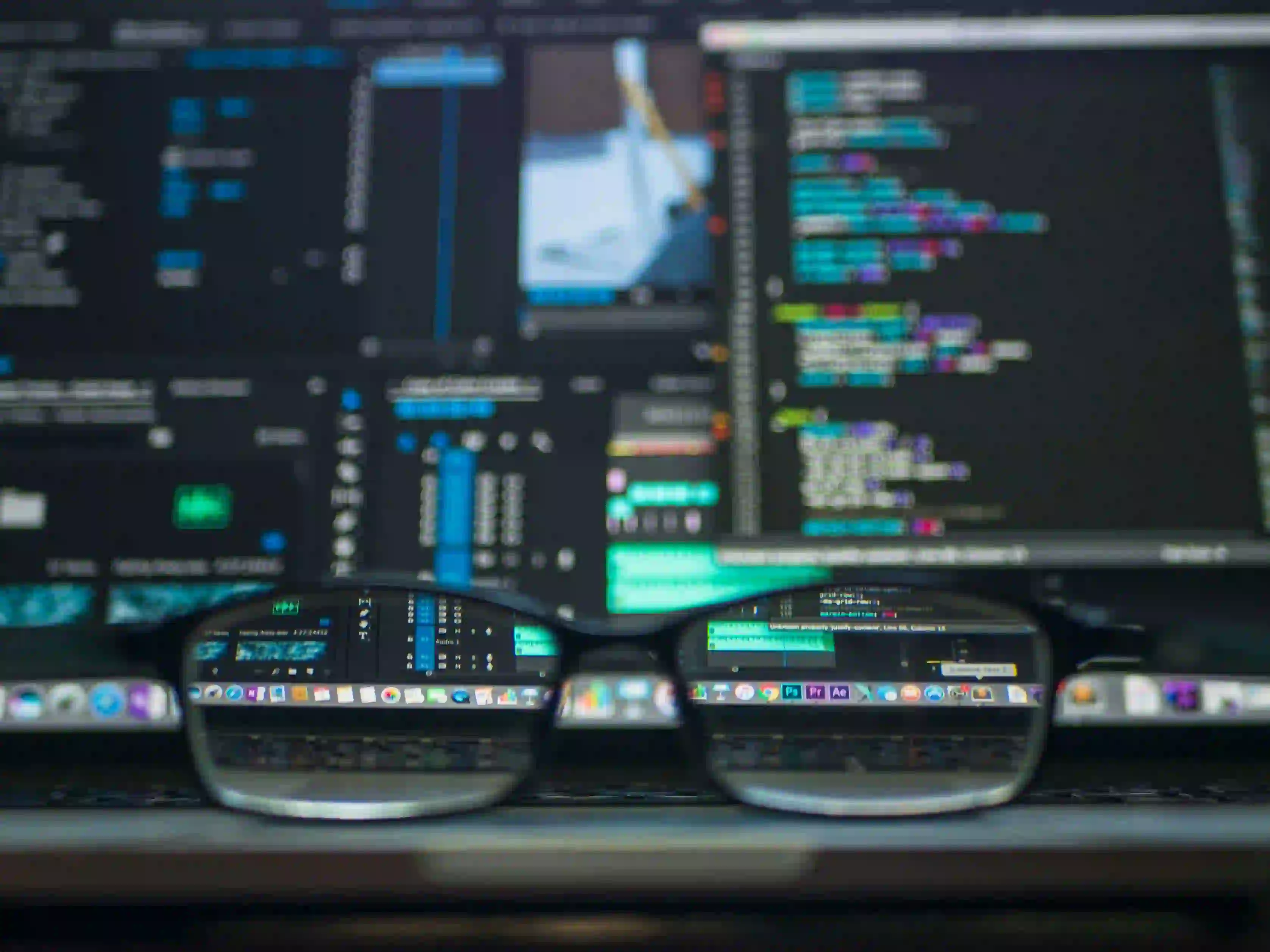
Top Selenium 4 Challenges and How to Overcome Them
Selenium 4 represents a significant upgrade from its predecessor, Selenium 3, bringing new features and enhanced capabilities for automated testing. However, with these advancements come certain challenges. In this blog post, we will explore some of the most common difficulties faced by developers using Selenium 4 and provide solutions to navigate them effectively.
Table of Contents
- Introduction to Selenium 4
- Challenge 1: Browser Compatibility Issues
- Challenge 2: New W3C Protocol Implementation
- Challenge 3: Handling Dynamic Elements
- Challenge 4: Performance Issues
- Challenge 5: Robustness of Tests
- Conclusion
Before We Begin to Selenium 4
Selenium is an open-source suite designed for automating web applications for testing purposes. With the release of Selenium 4, we see a focus on better performance, an improved user experience, and support for the W3C WebDriver protocol.
As we dive deeper into Selenium 4, we will uncover some challenges and how to address them.
Challenge 1: Browser Compatibility Issues
The Problem
While Selenium 4 enhances browser compatibility, developers still encounter issues when different browsers render web elements differently. This can lead to inconsistency in test results.
The Solution
To mitigate these issues, always ensure that you are using the latest browser versions. Additionally, consider using Selenium's built-in capabilities such as:
WebDriver driver = new ChromeDriver();
// You can switch to Firefox or Edge as needed
driver = new FirefoxDriver();
// Or
driver = new EdgeDriver();
This ensures that you're testing across various browsers. To streamline this process, consider using a cross-browser testing tool like BrowserStack or Sauce Labs that allows you to run your tests on a wide range of browser and OS combinations.
Challenge 2: New W3C Protocol Implementation
The Problem
Selenium 4's shift to the W3C WebDriver protocol is transformative, yet it introduces complexity. This could lead to confusion, especially if developers are still accustomed to the JSON Wire Protocol.
The Solution
Familiarizing yourself with the W3C WebDriver standard is key. Here’s how you can do that:
- Read the W3C WebDriver Spec: Understanding the specification will clarify the differences.
- Utilize the updated APIs: Make sure to leverage the new features, which often have clearer syntax.
For example, here is a simple snippet using improved session handling via the W3C implementation:
WebDriver driver = new ChromeDriver();
driver.manage().window().maximize(); // This line is now a W3C standardized method.
By being proactive in understanding these changes, you can quickly adapt your testing efforts.
Challenge 3: Handling Dynamic Elements
The Problem
Web pages today have many dynamic elements that load asynchronously, leading to NoSuchElementException
or stale elements when trying to interact with them too soon.
The Solution
You can use Selenium’s built-in Waits for handling dynamic elements effectively. Here’s a demonstration:
WebDriver driver = new ChromeDriver();
driver.get("https://example.com");
WebDriverWait wait = new WebDriverWait(driver, Duration.ofSeconds(10));
WebElement dynamicElement = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("dynamicElementId")));
dynamicElement.click();
In this snippet, we are using an explicit wait to ensure the element is visible before attempting to click. This significantly increases the robustness of your tests.
Challenge 4: Performance Issues
The Problem
Tests running slowly can lead to significant delays when trying to execute a test suite, which is detrimental in Continuous Integration/Continuous Deployment (CI/CD) environments.
The Solution
To improve performance, consider the following strategies:
- Limit the use of implicit waits: Start using explicit waits instead of implicit ones.
- Optimize test execution: Parallel test execution is another way to boost performance.
Here’s a simple snippet to run tests in parallel using TestNG:
<suite name="Parallel Test Suite" parallel="methods" thread-count="5">
<test name="Test Group 1">
<classes>
<class name="your.package.TestClass1" />
</classes>
</test>
<test name="Test Group 2">
<classes>
<class name="your.package.TestClass2" />
</classes>
</test>
</suite>
The above XML configuration will help you run different test methods simultaneously, drastically improving test execution time.
Challenge 5: Robustness of Tests
The Problem
Selenium tests can easily become brittle, failing on minor changes to the UI or web elements. Maintaining these tests can become a burden.
The Solution
- Adopt Page Object Model (POM): This design pattern helps keep your tests organized and reduces code duplication.
Here is how to structure your tests using the Page Object Model:
public class LoginPage {
WebDriver driver;
@FindBy(id = "username")
WebElement username;
@FindBy(id = "password")
WebElement password;
@FindBy(id = "login")
WebElement loginBtn;
public LoginPage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void login(String user, String pass) {
username.sendKeys(user);
password.sendKeys(pass);
loginBtn.click();
}
}
Using the Page Object Model, your test code becomes cleaner and more maintainable. This encapsulation minimizes the number of changes required if the UI changes.
- Incorporate Assertions: Ensure you're validating outcomes to catch errors early in your test execution.
Assert.assertEquals(driver.getTitle(), "Expected Title");
This line checks if the page title is as expected, ensuring that your tests fail gracefully when something goes wrong.
To Wrap Things Up
While Selenium 4 presents exciting possibilities for automated testing, it also introduces challenges that require attention. By understanding these hurdles and employing effective solutions such as the use of explicit waits, adopting design patterns like POM, and leveraging parallel test execution, you can effectively streamline your testing process.
Automation is not merely about writing tests. It's about creating a reliable testing suite that adapts and scales with your project. The key is to stay informed, practice good coding habits, and continually improve your automation strategy.
If you're looking for more insights into optimizing your Selenium tests, consider checking out the Selenium Official Documentation for detailed guidelines and updates. Happy testing!