Essential Dependencies for Spring Data JPA Simplified
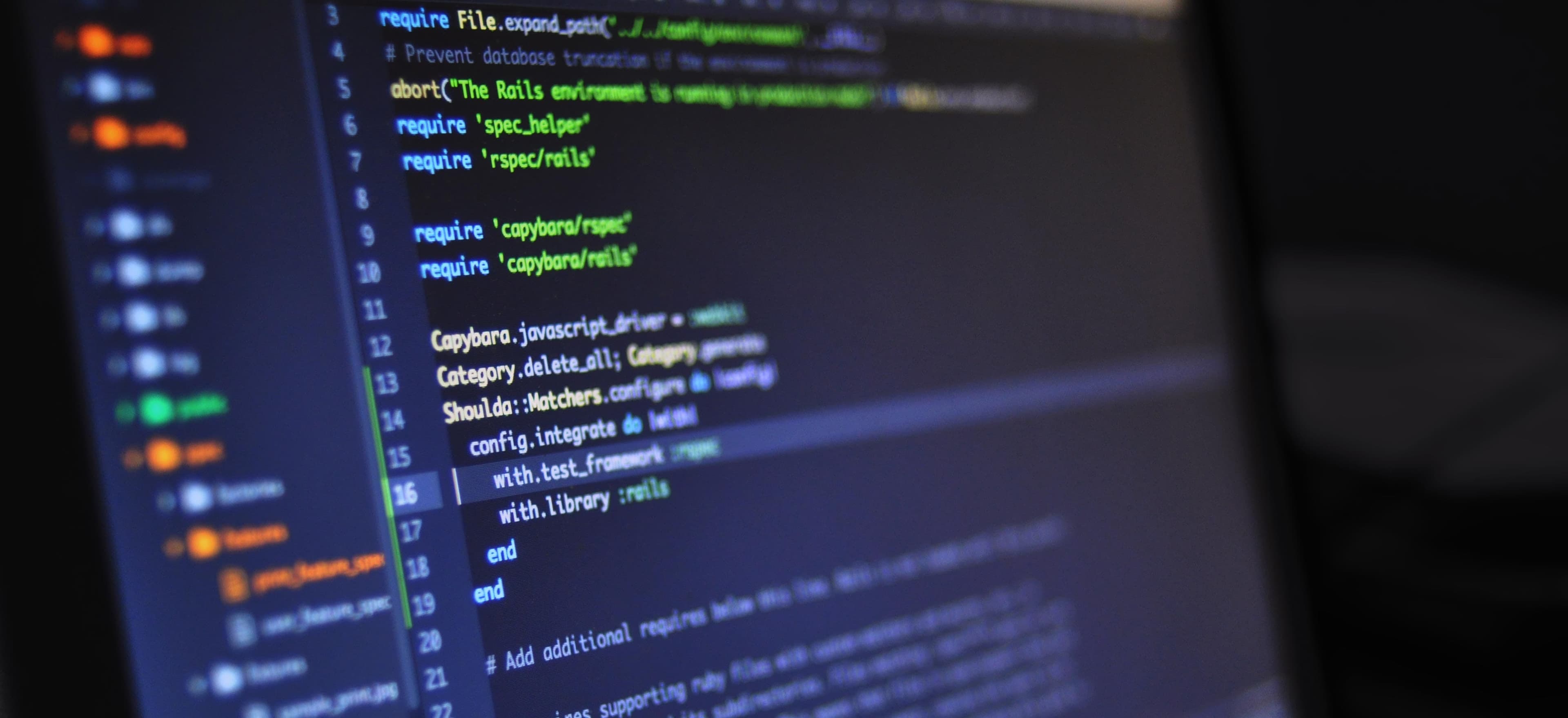
- Published on
Essential Dependencies for Spring Data JPA Simplified
Spring Data JPA is a powerful framework that simplifies database interactions in Java applications by providing a set of useful features to work with JPA (Java Persistence API). The framework allows developers to access data in a relational database without writing boilerplate code, making the development process smoother and more efficient.
In this blog post, we will explore the essential dependencies for Spring Data JPA, their significance, and how to set up a Spring Boot project that incorporates these dependencies. We'll cover the key components you'll need to get started using Spring Data JPA effectively.
What is Spring Data JPA?
Before diving into dependencies, let's clarify what Spring Data JPA is. Spring Data JPA is part of the larger Spring Data project that aims to provide a simplified approach to accessing data in databases through JPA. It allows developers to write much less boilerplate code when dealing with database operations, thanks to features like repository support and derived queries.
Why Use Spring Data JPA?
- Simplified Data Access: You can reduce the amount of code needed to perform CRUD (Create, Read, Update, Delete) operations.
- Custom Query Methods: The framework allows the creation of custom query methods directly from repository interfaces.
- Integration with Spring Boot: Spring Data JPA works seamlessly with Spring Boot, simplifying configuration and setup.
Setting Up a Spring Boot Project
To use Spring Data JPA, you typically start with a Spring Boot application. You can create a new Spring Boot application via Spring Initializr (https://start.spring.io) and include the necessary dependencies from the beginning.
Essential Dependencies
Here are the essential dependencies for using Spring Data JPA in your project. You can add these dependencies using Maven or Gradle.
Maven Dependencies
<dependencies>
<!-- Spring Boot Starter Parent -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.5</version>
<type>pom</type>
<scope>import</scope>
</dependency>
<!-- Spring Data JPA -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- H2 Database for Development -->
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<!-- PostgreSQL for Production -->
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<scope>runtime</scope>
</dependency>
<!-- Spring Boot Starter Web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Boot Starter Test (for testing purposes) -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
Gradle Dependencies
For Gradle users, here’s how your build.gradle
file would typically look:
plugins {
id 'org.springframework.boot' version '2.7.5'
id 'io.spring.dependency-management' version '1.0.11.RELEASE'
id 'java'
}
group = 'com.example'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '17'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
runtimeOnly 'com.h2database:h2'
runtimeOnly 'org.postgresql:postgresql'
implementation 'org.springframework.boot:spring-boot-starter-web'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
Explanation of Key Dependencies
-
spring-boot-starter-data-jpa: This dependency provides the fundamental tools to work with Spring Data JPA, including the required libraries for JPA functionality and basic configurations.
-
H2 Database: The H2 database is a lightweight database, useful for development and testing purposes. It's easy to set up and requires minimal configuration. For production, it's common to switch to a more robust solution, such as PostgreSQL or MySQL.
-
PostgreSQL: This is a powerful, open-source relational database. By including this dependency, you can connect to a PostgreSQL database in your application, making it more capable of handling complex data queries in a production environment.
-
spring-boot-starter-web: This dependency includes everything necessary to create RESTful web services, enabling your application to expose API endpoints.
-
spring-boot-starter-test: This starter includes tools for testing your Spring Boot application effectively.
Configuring Your Application
After adding the necessary dependencies, you'll need to configure your Spring Boot application. Most of the configuration can be done through the application.properties
file.
Configuration Example
Here’s an example of a simple application.properties
configuration file:
# Data source configuration for PostgreSQL
spring.datasource.url=jdbc:postgresql://localhost:5432/mydatabase
spring.datasource.username=myuser
spring.datasource.password=mypassword
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
Explanation of Properties
-
spring.datasource.url: This specifies the database connection URL including the database name.
-
spring.datasource.username: The username for accessing the database.
-
spring.datasource.password: The password for the database user.
-
spring.jpa.hibernate.ddl-auto: This property controls how the application manages the database schema.
update
automatically updates the schema based on the entity classes. -
spring.jpa.show-sql: When set to
true
, this property allows you to see the SQL queries generated by Hibernate in the console.
Creating a Simple Entity
Let’s create a simple JPA entity to illustrate how the entire setup comes together.
Example Entity
Here’s a basic User
entity class:
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity // Declaring this class as a JPA entity
public class User {
@Id // The primary key of this entity
@GeneratedValue(strategy = GenerationType.IDENTITY) // Auto-generated ID
private Long id;
private String name;
private String email;
// Getters and setters
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
Explanation of the Code
This User
class is annotated with @Entity to indicate it as a JPA entity. The @Id annotation specifies this field as the primary key. @GeneratedValue instructs JPA to auto-generate the primary key value, making it easy for you to manage unique identifiers without manual intervention.
Creating a Repository Interface
To enable CRUD operations for the User
entity, create a repository interface:
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository // Indicating this interface is a Spring-managed component
public interface UserRepository extends JpaRepository<User, Long> {
// Custom query methods can be defined here
}
Explanation of the Interface
This interface extends JpaRepository
, which provides built-in methods to perform operations like saving, deleting, and finding users. By extending this interface, you can add custom query methods as needed.
Lessons Learned
The Spring Data JPA dependencies are essential for creating modern, maintainable, and efficient web applications that interact seamlessly with relational databases. By leveraging Spring Boot and its auto-configuration capabilities, you can focus more on business logic rather than boilerplate code.
In summary, setting up Spring Data JPA requires a few crucial dependencies to be included in your project. Ensure to select the right database for your environment and carefully configure your application properties to facilitate smooth database connections. With Spring Data JPA at your disposal, building robust data-driven applications becomes a more straightforward and enjoyable task.
For further reading, check out the official Spring Data JPA documentation for comprehensive guides and advanced topics.
Happy coding!
Checkout our other articles