Solving Common Twilio Integration Issues in Spring Boot
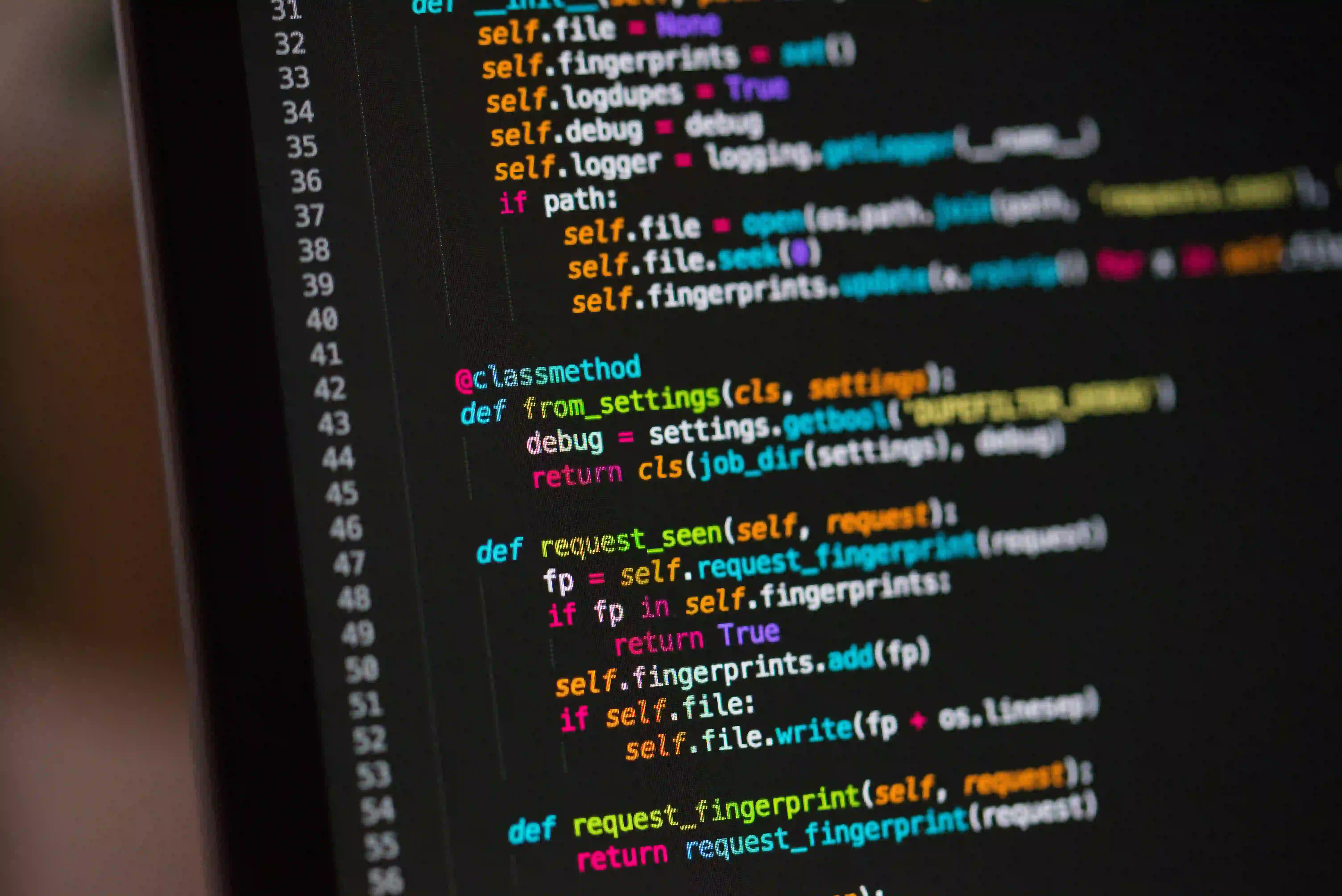
Solving Common Twilio Integration Issues in Spring Boot
Integrating Twilio into a Spring Boot application can enhance your project's capabilities by adding powerful communication features such as SMS, voice calls, and video. However, like any integration, developers may encounter challenges along the way. In this blog post, we'll explore some common Twilio integration issues and how to resolve them in a Spring Boot environment.
Table of Contents
- Understanding Twilio and Spring Boot Integration
- Common Issues and Solutions
- Authentication Failures
- Handling Twilio Webhooks
- Sending SMS Messages
- Managing Dependencies
- Conclusion
Understanding Twilio and Spring Boot Integration
Twilio's APIs allow developers to build communication solutions seamlessly. Spring Boot, known for its ease of use and rapid application development, serves as an excellent backend framework for such integrations.
To start using Twilio, you need to create a Twilio account, acquire relevant API credentials, and set up a Spring Boot application if you haven’t already. For more information on how to set up a Spring Boot project from scratch, check Spring Boot Official Documentation.
Getting Started
Firstly, include the Twilio SDK in your pom.xml
:
<dependency>
<groupId>com.twilio.sdk</groupId>
<artifactId>twilio</artifactId>
<version>8.30.0</version>
</dependency>
This dependency allows you to access a rich set of functionalities provided by Twilio's API. The next steps will unveil challenges that developers commonly face while integrating this SDK with a Spring Boot application.
Common Issues and Solutions
1. Authentication Failures
One of the most frequent issues developers face during integration is authentication failure due to improper API credentials setup. Twilio requires Account SID
, Auth Token
, and other identifiers for authentication.
Solution: Ensure that your credentials are set correctly. You should store sensitive data like API keys in environment variables or configuration files, for example:
# application.properties
twilio.account_sid=your_account_sid
twilio.auth_token=your_auth_token
twilio.phone_number=your_twilio_phone_number
Next, in your Spring Boot service, you can access these properties as follows:
import com.twilio.Twilio;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Service;
@Service
public class TwilioService {
@Value("${twilio.account_sid}")
private String accountSid;
@Value("${twilio.auth_token}")
private String authToken;
// Constructor
public TwilioService() {
Twilio.init(accountSid, authToken);
}
}
This code ensures that you initialize Twilio with the correct credentials whenever your service class is instantiated.
2. Handling Twilio Webhooks
Webhooks may present integration challenges, especially when dealing with message status updates or incoming messages. These callbacks can be tricky to manage if not properly configured.
Problem: You may not receive callbacks in your application if your endpoint isn't correctly set up.
Solution: Create a controller to handle incoming webhook requests effectively:
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class TwilioWebhookController {
@PostMapping("/twilio/webhook")
public String receiveWebhook(
@RequestParam("From") String from,
@RequestParam("Body") String body) {
System.out.println("Message received from: " + from);
System.out.println("Message body: " + body);
// Process the incoming message
return "Message Received";
}
}
Make sure your Twilio console is configured to point to the correct URL for your webhook. You can set this under your Twilio phone number settings.
3. Sending SMS Messages
Sending SMS may seem straightforward, but developers often face issues with message failures and delivery reports.
Problem: A common error when sending messages is due to an invalid phone number format or lack of sender permissions.
Solution: When sending SMS messages, use the following code to ensure proper handling:
import com.twilio.rest.api.v2010.account.Message;
import com.twilio.type.PhoneNumber;
public void sendSMS(String to, String messageBody) {
Message message = Message.creator(
new PhoneNumber(to),
new PhoneNumber("+1234567890"), // Your Twilio number
messageBody)
.create();
System.out.println("Message Sent: " + message.getSid());
}
Note the importance of validating phone numbers using E.164 format. Ensure that your to
variable is formatted correctly. This is crucial for preventing errors when configuring message sending functionality.
4. Managing Dependencies
When integrating Twilio with Spring Boot, dependency management may become problematic, especially when trying to resolve conflicts with other libraries.
Solution:
Ensure that all dependencies in your pom.xml
are compatible. Use the Spring Boot dependency management plugin to manage versions consistently:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.5.4</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
This approach allows Spring Boot to manage compatible versions of the Twilio SDK and other libraries, reducing the chances of dependency conflicts.
My Closing Thoughts on the Matter
Integrating Twilio with a Spring Boot application can significantly enrich your communication capabilities. By proactively addressing common issues associated with Twilio integration, you not only save development time but also ensure a smoother implementation process.
Remember to keep your API keys secure, handle webhooks carefully, validate your message formats, and manage dependencies effectively. With these strategies, you’ll be well on your way to successfully integrating Twilio into your Spring Boot projects.
For more on using Twilio, explore Twilio API Documentation for deeper insights into its capabilities and usage. Happy coding!