Common JPA Pitfalls: Avoiding Common Mistakes in Java
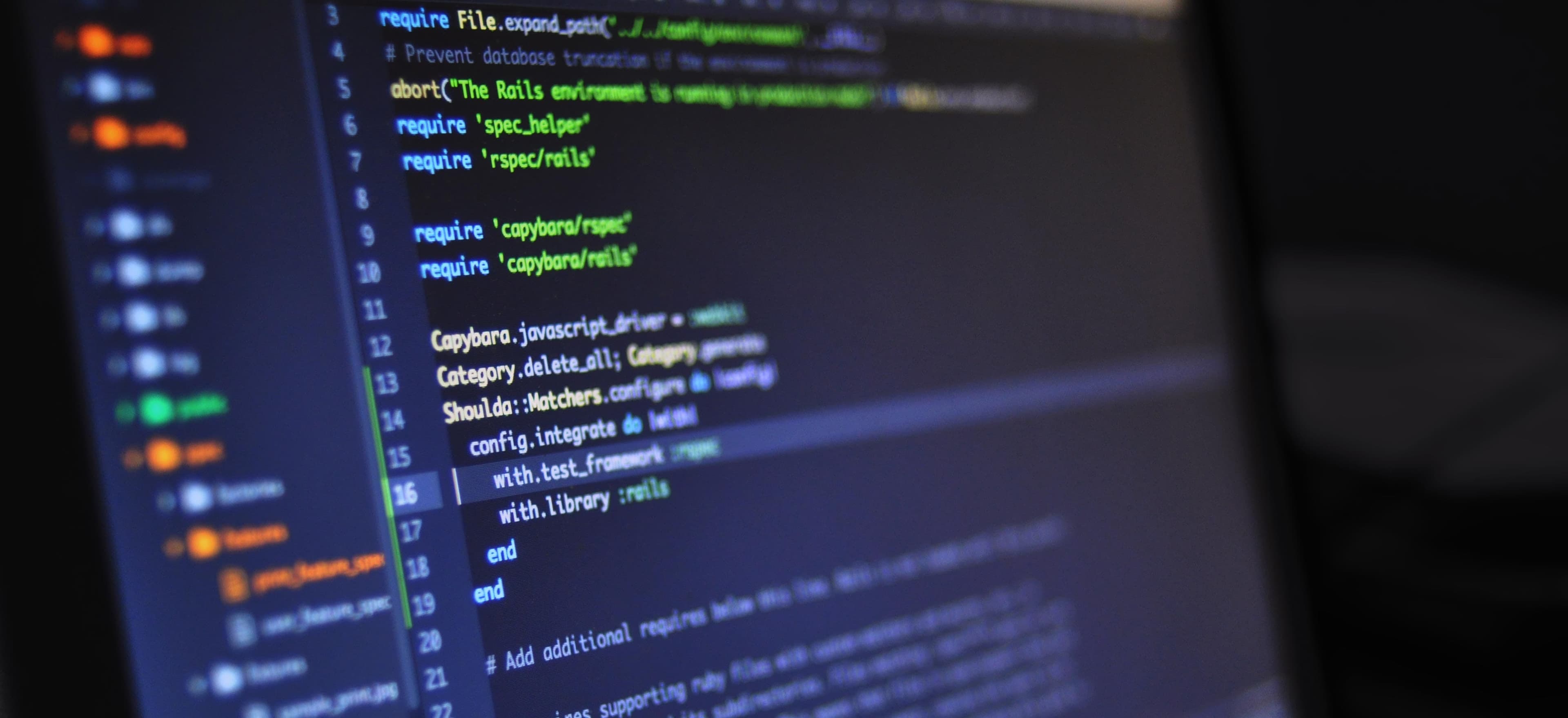
- Published on
Common JPA Pitfalls: Avoiding Common Mistakes in Java
When it comes to Java Persistence API (JPA), developers often face challenges that can lead to performance issues, bugs, and even data integrity problems. While JPA provides a powerful framework for managing relational data in Java applications, there are common pitfalls that can hinder your success. This blog post will help you identify and avoid those pitfalls, ensuring a smoother development experience with JPA.
Understanding JPA
JPA is a specification for accessing, persisting, and managing data between Java objects and relational databases. It abstracts the complexity of JDBC and allows developers to work with Java objects as if they're directly interacting with the database. This abstraction, however, comes with its own set of challenges.
1. Misunderstanding Entity Lifecycle
One of the first common pitfalls developers face is misunderstanding the lifecycle of JPA entities. The JPA entity lifecycle has several states: New, Managed, Detached, and Removed.
Why It Matters
Failing to grasp the lifecycle can lead to unexpected behaviors. For example, if you improperly manage entity state, changes may not be persisted in the database as you might expect.
Example
EntityManager em = entityManagerFactory.createEntityManager();
em.getTransaction().begin();
// New state
User user = new User("JohnDoe", "john@example.com");
em.persist(user); // Now in Managed state
em.getTransaction().commit();
em.close();
In this example, we create a new User
instance. The em.persist(user)
method transitions the entity from the New state to the Managed state, ensuring it’s tracked by the persistence context.
Important Note
Always close your entity manager to avoid memory leaks and ensure proper cleanup. This keeps your application robust and prevents unexpected behaviors.
2. Ignoring Fetch Strategies
Fetching strategies directly impact your application's performance and ability to handle large datasets. JPA provides three primary fetching strategies: EAGER, LAZY, and JOIN FETCH.
Performance Implications
Using EAGER fetching can lead to the "N+1 Selects Problem," where the application performs one query to fetch the parent entity and then additional queries for each child entity.
Example
@Entity
public class User {
@Id
private Long id;
@OneToMany(fetch = FetchType.LAZY, mappedBy = "user")
private List<Order> orders;
}
In this example, we are using LAZY loading for the orders
property, meaning the associated Order
entities will only be loaded when explicitly accessed. This is typically more efficient, especially when dealing with large datasets.
Additional Resources
For a deeper understanding of JPA fetching strategies, check out this article on fetching strategies.
3. Improper Transactions Management
Transaction management is at the heart of any data manipulation task in JPA. Mismanagement of transactions can lead to data corruption or unexpected application behavior.
Avoiding Mistakes
Make sure that transactions are correctly demarcated. This means ensuring begin
, commit
, and rollback
operations are clearly defined.
Example
EntityTransaction transaction = em.getTransaction();
try {
transaction.begin();
// Perform data operations
transaction.commit();
} catch (Exception e) {
if (transaction.isActive()) {
transaction.rollback();
}
e.printStackTrace();
}
In this snippet, we start a transaction, perform operations, and commit or rollback as necessary to maintain data integrity.
4. Forgetting about Caching
Caching is vital to the performance of any application that constantly interacts with the database. JPA provides a first-level cache (the persistence context) and a second-level cache that you can implement with various solutions like Ehcache or Hazelcast.
Why Use Caching?
Caching reduces the number of times the application needs to reach for data in the relational database, significantly improving performance.
Example of First-Level Cache
User user1 = em.find(User.class, 1L);
User user2 = em.find(User.class, 1L); // This pulls from the first-level cache, no SQL query fired.
In this example, the second em.find
call retrieves the User
entity from the first-level cache instead of executing a new SQL query.
Implementing Second-Level Caching
Make sure you configure your JPA provider for second-level caching. Check your provider's documentation on how to properly enable and configure this feature.
5. Poorly Designed Entity Relationships
When designing your entities, it is crucial to understand the relationships (One-to-One, One-to-Many, Many-to-One, Many-to-Many) and correctly map them.
Design Challenges
Incorrectly mapping relationships can lead to data redundancy, performance issues, and even runtime exceptions.
Example
@Entity
public class Author {
@Id
private Long id;
@OneToMany(mappedBy = "author")
private List<Book> books;
}
@Entity
public class Book {
@Id
private Long id;
@ManyToOne
@JoinColumn(name = "author_id")
private Author author;
}
In this example, we establish a One-to-Many relationship between Author
and Book
. Careful mapping ensures the associated entities are correctly related without redundancy.
6. Not Leveraging JPQL and Criteria API
JPQL (Java Persistence Query Language) and Criteria API allow for powerful and type-safe querying of data, yet many developers revert to using native SQL or forget to utilize these capabilities.
Advantages of JPQL
JPQL is much more readable and maintainable compared to SQL as it operates on JPA's entity objects, not directly on database tables.
Example
String jpql = "SELECT u FROM User u WHERE u.email = :email";
TypedQuery<User> query = em.createQuery(jpql, User.class);
query.setParameter("email", "john@example.com");
User user = query.getSingleResult();
In this example, we use JPQL to select a User
by their email, improving readability and leveraging the power of the JPA with fewer risks of errors and maintenance issues.
Closing Remarks
Navigating through the world of JPA can be daunting due to the many pitfalls it presents. However, a solid understanding of entity lifecycles, fetching strategies, transaction management, caching, relationship design, and the use of JPQL will position you for success.
By avoiding these common mistakes, you’ll create applications that are not only functional but also efficient and maintainable. Always stay updated on best practices and emerging frameworks in the JPA ecosystem for continual improvement in your development skills.
If you have further questions or insights on JPA, feel free to share in the comments or reach out!
Further Reading
- Understanding JPA and Hibernate
- JPA Performance Tuning
By investing time into understanding these concepts, you empower yourself to leverage JPA to its fullest potential while avoiding detrimental pitfalls. Happy coding!