Why Unique Hashcodes Can't Prevent Data Collisions
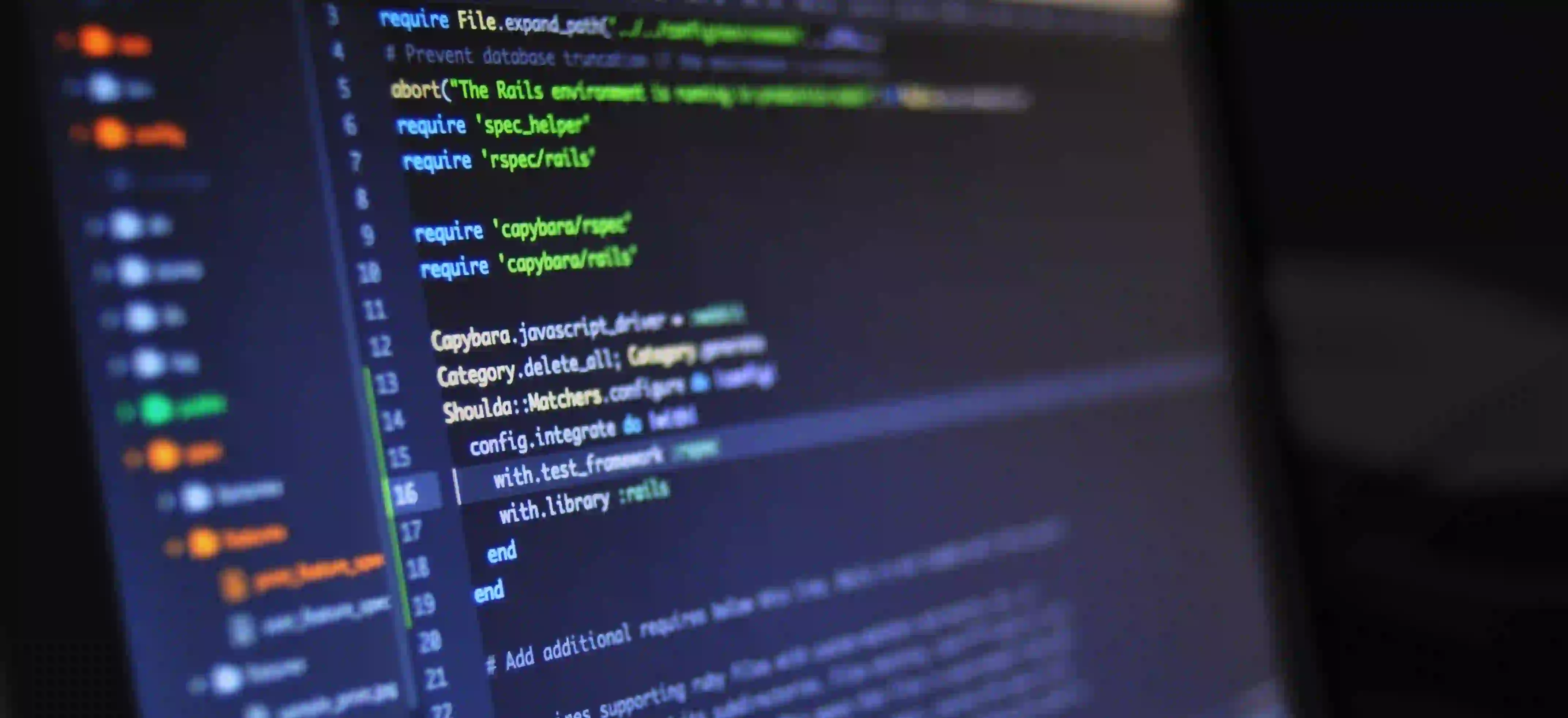
Why Unique Hashcodes Can't Prevent Data Collisions
Establishing the Context
In programming, hashcodes play a pivotal role in the efficient storage and retrieval of data. Many data structures, such as hash tables, rely heavily on hash functions to map keys to values. However, one of the critical challenges in using hashcodes is the possible occurrence of data collisions. In this blog, we'll dive into why unique hashcodes can't completely prevent data collisions. We'll discuss hash functions, their properties, and provide insights into how collisions occur despite the representation of unique hashcodes.
Understanding Hashcodes and Hash Functions
A hashcode is an integer value that is generated from an object (or a key). This integer acts as an index in a hash table, allowing for quick access to the data stored at that index. A hash function, which generates this hashcode, takes an input (or a key) and outputs a fixed-size string of bytes.
Characteristics of a Good Hash Function
A well-designed hash function should possess the following properties:
- Deterministic: The same input will always produce the same output.
- Efficient: It must compute the hashcode quickly.
- Uniform Distribution: It should distribute the hashcodes uniformly across its output range to minimize collisions.
- Collision Resistance: Ideally, it should be difficult to find two different inputs that produce the same hashcode.
Example of a Simple Hash Function
Let's look at a basic implementation of a hash function in Java:
public class SimpleHash {
public static int hash(String key) {
int hash = 0;
for (char c : key.toCharArray()) {
hash += c;
}
return hash;
}
public static void main(String[] args) {
System.out.println("Hash of 'apple': " + hash("apple"));
System.out.println("Hash of 'banana': " + hash("banana"));
}
}
Why This Code? In this example, we create a simple hash function that sums the ASCII values of the characters in a string. While this function is deterministic and efficient, it does not provide a uniform distribution, leaving room for collisions between different inputs.
Data Collisions Explained
A collision occurs when two different inputs produce the same hashcode. To understand why unique hashcodes can't prevent collisions entirely, consider the following:
-
Pigeonhole Principle: This principle states that if you have more items (inputs) than containers (possible hashcodes), at least one container must hold more than one item. For example, if our hash function returns values from a small integer range, and we input a larger number of strings, collisions are inevitable.
-
Limited Range of Output: Most hash functions generate a fixed-size output. Given a finite number of hashcodes, infinite potential inputs would mean that different inputs would inevitably map to the same hashcode. For instance, a hash function producing 32-bit integers can represent a maximum of 2^32 (around 4 billion) unique hashcodes.
-
Different Inputs, Same Output: Certain inputs can be specifically crafted or coincidentally structured to generate the same hashcode, a phenomenon known as a collision attack. For instance, a clever user might use two different inputs that are specifically designed to yield the same result in a poorly designed hash function.
Strategies to Handle Collisions
While hash functions can't avoid collisions, there are several strategies to handle them effectively:
1. Separate Chaining
In separate chaining, each bucket of the hash table holds a list (or any other data structure) of entries that hash to the same index. When a collision occurs, the new item is simply added to the list.
import java.util.LinkedList;
public class HashTable {
private LinkedList<Entry>[] table;
public HashTable(int size) {
table = new LinkedList[size];
for (int i = 0; i < size; i++) {
table[i] = new LinkedList<>();
}
}
public void put(String key, String value) {
int hash = SimpleHash.hash(key) % table.length;
table[hash].add(new Entry(key, value));
}
static class Entry {
String key;
String value;
Entry(String key, String value) {
this.key = key;
this.value = value;
}
}
}
Why This Code? Here we implement a basic hash table using separate chaining. Each index points to a linked list where we can store all entries mapping to the same hashcode, helping us handle collisions effectively.
2. Open Addressing
Another collision resolution technique is open addressing, which looks for the next available spot in the hash table for a new entry if a collision occurs.
public class OpenAddressHashTable {
private String[] table;
private int size;
public OpenAddressHashTable(int size) {
this.size = size;
this.table = new String[size];
}
public void put(String key) {
int hash = SimpleHash.hash(key) % size;
while (table[hash] != null) {
hash = (hash + 1) % size; // Linear probing
}
table[hash] = key;
}
}
Why This Code? This example demonstrates the linear probing technique used in open addressing. In the event of a collision, the program checks subsequent indices until it finds an empty slot.
To Wrap Things Up
While unique hashcodes provide a foundational concept in data organization, they do not completely eliminate the risk of collisions. Due to limitations in range and the pigeonhole principle, multiple inputs can map to the same hashcode. However, understanding how to effectively manage these collisions—with techniques such as separate chaining and open addressing—can significantly enhance the performance and efficiency of data retrieval and storage systems.
For more in-depth understanding of hash functions and handling collisions, consider reviewing the following links:
Always remember, while we strive for uniqueness in hashcodes, being prepared to manage collisions is equally vital for building robust systems. Happy coding!