Top 5 Automation Testing Mistakes to Avoid for Success
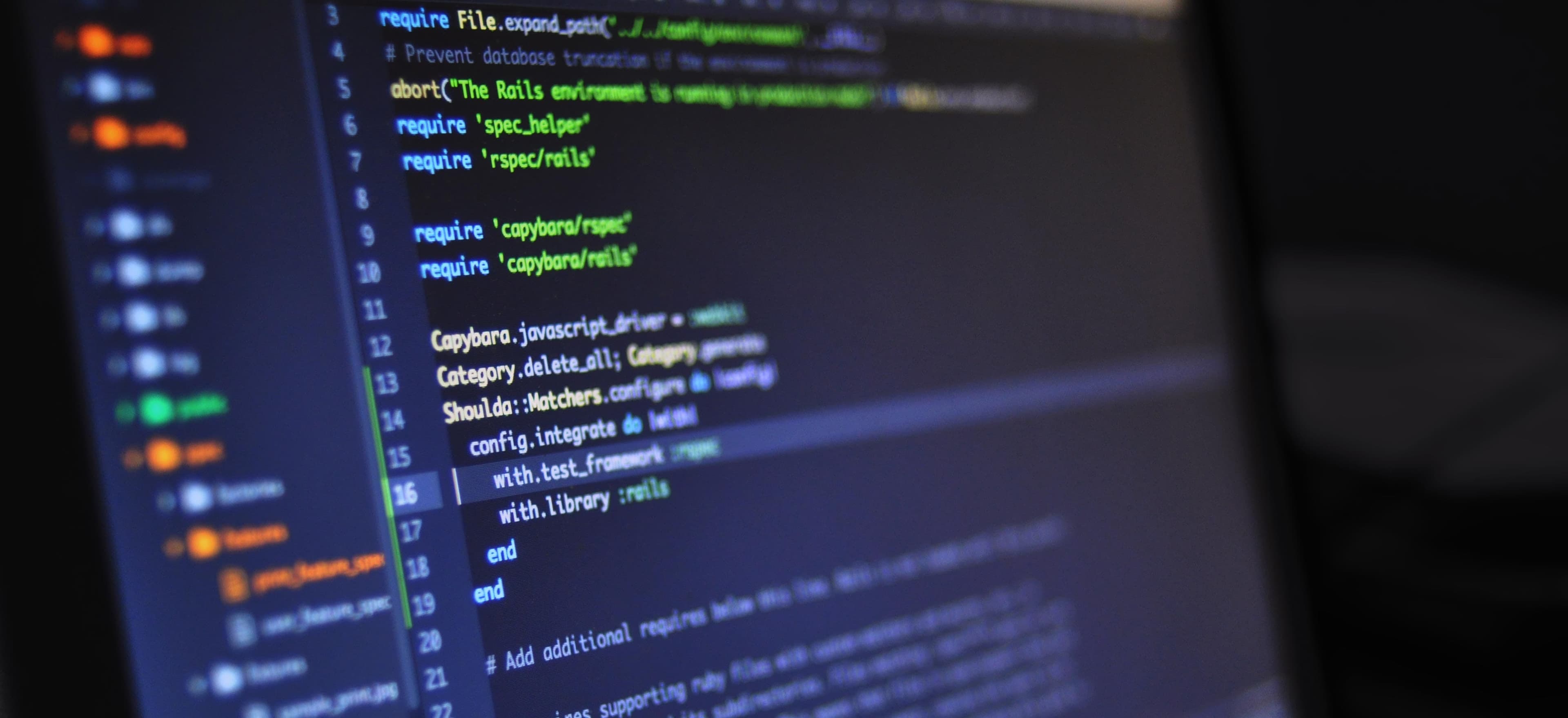
- Published on
Top 5 Automation Testing Mistakes to Avoid for Success
In the rapidly evolving world of software development, automation testing has emerged as a cornerstone for ensuring high-quality deliverables. However, the effectiveness of automation testing is often hindered by common mistakes that teams make during implementation. In this article, we will discuss the top five automation testing mistakes to avoid – helping you streamline your testing process and enhance your project's success.
1. Not Choosing the Right Automation Tool
One of the most significant mistakes teams make is selecting an automation testing tool without thoroughly analyzing their needs.
Why It Matters
A tool should align with your application’s technology stack, team skills, and project requirements. For instance, using a tool designed for web applications when testing a mobile application can complicate your efforts and lead to inefficient testing cycles.
Best Practices
- Conduct a Needs Assessment: Evaluate the technologies your application uses, the testing framework, and your team's familiarity with potential tools.
- Evaluate Tool Features: Look for features that support your specific needs, such as test management, reporting, and platform compatibility.
Example Code Snippet
In Selenium, a popular automation tool for web applications, the following code snippet demonstrates how to initialize a WebDriver for a Chrome browser:
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class SeleniumExample {
public static void main(String[] args) {
// Set system property for ChromeDriver
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
// Initialize WebDriver
WebDriver driver = new ChromeDriver();
// Open a web page
driver.get("https://www.example.com");
// Close the browser
driver.quit();
}
}
This initialization is vital for any automated testing script as it enables you to interact with web elements.
2. Ignoring Test Case Prioritization
Not all test cases hold the same level of importance. Ignoring this fact can result in wasted resources and missed critical bugs.
Why It Matters
Prioritizing test cases ensures that the most crucial functionalities of your application are consistently tested, especially in agile environments where timelines are compressed.
Best Practices
- Implement Risk-based Testing: Identify high-risk areas of your application and focus your automated testing efforts there.
- Create a Test Matrix: Use a test matrix to categorize and prioritize your test cases based on factors like usage frequency and defect density.
Example Code Snippet
Here's how you can use a simple approach to prioritize test cases in a TestNG framework:
import org.testng.annotations.Test;
public class TestPriorityExample {
@Test(priority = 1)
public void criticalFeatureTest() {
// Critical feature test
}
@Test(priority = 2)
public void trustedFeatureTest() {
// Trusted feature test
}
@Test(priority = 3, enabled = false) // Skipping non-critical tests
public void lowImpactFeatureTest() {
// Non-critical feature test
}
}
Setting priorities in your test management helps your team focus on high-impact tests first.
3. Underestimating Maintenance Efforts
Automation isn’t a set-it-and-forget-it solution, and many teams fail to plan for ongoing maintenance.
Why It Matters
Changes in the application—such as new features, bugs, or UI modifications—can break your existing automated tests. Failing to maintain them leads to a high rate of false negatives, causing frustration for developers and testers alike.
Best Practices
- Regularly Review and Refactor Tests: Schedule time for teams to review tests, looking for opportunities to streamline or enhance efficiency.
- Use Version Control: Employ tools like Git for tracking and managing test scripts, making it easier to see changes and roll back if needed.
Example Code Snippet
Let's look at a sample refactoring in this Selenium test:
public void loginTest() {
driver.findElement(By.id("username")).sendKeys("testUser");
driver.findElement(By.id("password")).sendKeys("password123");
driver.findElement(By.id("login_button")).click();
// Verify login success
assertTrue(driver.findElement(By.id("welcome_message")).isDisplayed());
}
As the application evolves, you may need to update element locators or assertions to ensure the test remains accurate.
4. Lack of Integration with CI/CD Pipelines
Modern development practices often involve Continuous Integration/Continuous Deployment (CI/CD) pipelines, yet automation tests can often be an afterthought in these environments.
Why It Matters
Integrating automated tests into your CI/CD pipeline ensures that you catch issues early, reducing the cost of defects and time spent on later-stage tests.
Best Practices
- Automate the Build Process: Incorporate automation testing as part of your build pipeline, enabling tests to run with each integration.
- Monitor Results: Ensure that test results are monitored regularly, using dashboards to keep everyone informed.
Example Code Snippet
Here's a simple Jenkins Pipeline script to run tests:
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
// Build commands
}
}
}
stage('Test') {
steps {
script {
// Command to run your automation tests
sh 'mvn clean test'
}
}
}
}
}
This seamless integration allows for quicker feedback and helps maintain code quality.
5. Not Involving Stakeholders
A common pitfall is not involving all relevant stakeholders, including developers, product owners, and end-users, in the automation process.
Why It Matters
Collaboration helps uncover potential pitfalls early and enhances the relevance and coverage of your tests. Stakeholders have valuable insights into user expectations and potential edge cases that could otherwise be overlooked.
Best Practices
- Regular Communication: Hold regular meetings or discussions with stakeholders to understand their concerns and expectations.
- Gather Feedback: Use beta testing and round-tables with real users to acquire feedback on newly implemented features.
Key Takeaways
Automation testing is a powerful tool, but the effectiveness of your testing initiatives depends on avoiding common pitfalls. By carefully choosing the right tools, prioritizing tests, maintaining scripts, integrating with CI/CD, and involving stakeholders, your team can enhance the effectiveness of your automation testing strategy.
To dive deeper into automation testing and best practices, consider exploring this comprehensive guide which covers advanced techniques and strategies.
By being aware of these top five mistakes, you can position your team for success, ensuring high-quality software deliveries that meet the expectations of stakeholders and users alike.