When to Choose Refactoring Over Redesign for Your Codebase?
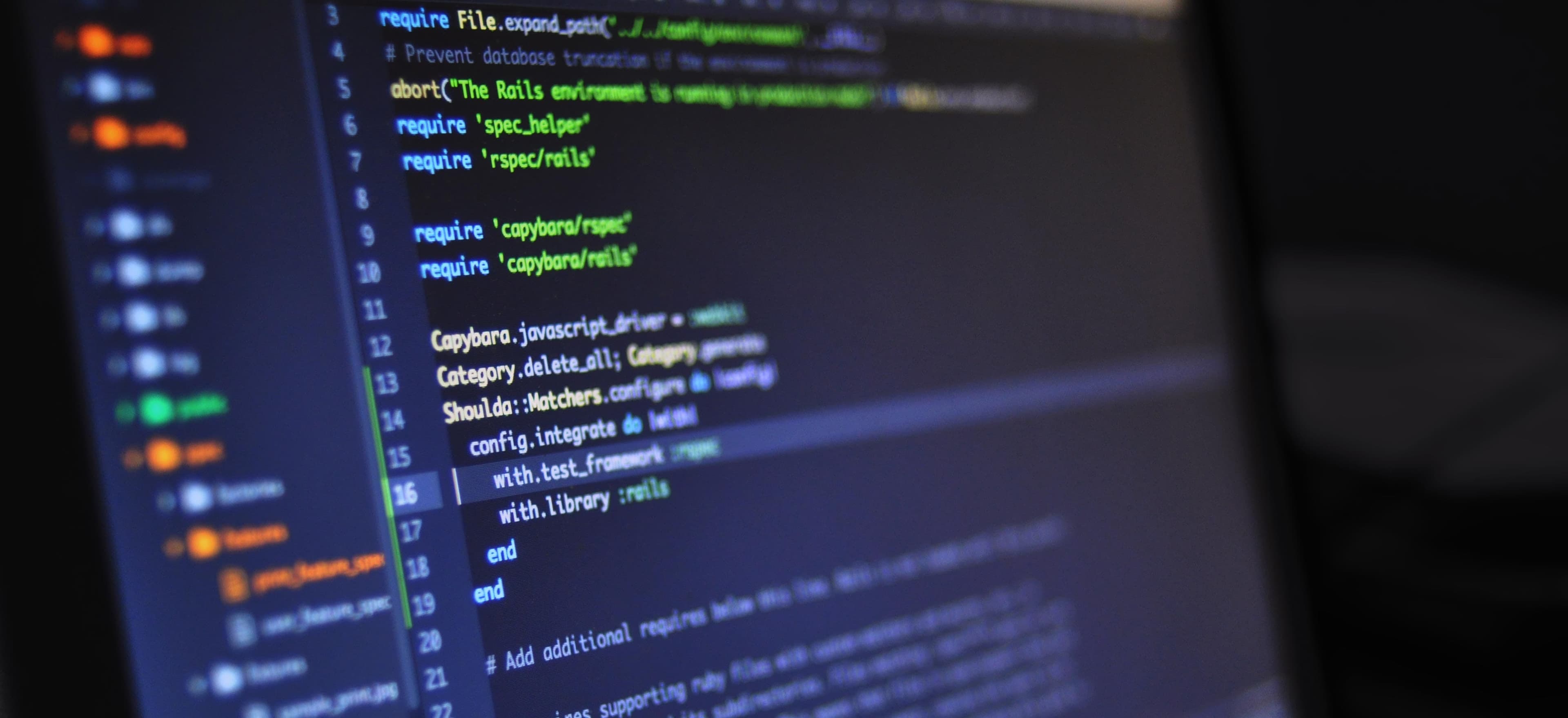
- Published on
When to Choose Refactoring Over Redesign for Your Codebase
In the ever-evolving landscape of software development, engineers frequently encounter the dilemma of whether to refactor existing code or undertake a complete redesign. This decision encompasses a myriad of factors, including code maintainability, project timelines, team dynamics, and user needs. In this post, we will delve into the nuances of refactoring versus redesigning, providing insights that aid in determining the best approach for your specific circumstances.
Understanding Refactoring and Redesign
Before making an informed choice, it is crucial to understand what these terms imply.
Refactoring refers to the process of restructuring existing code without altering its external behavior. The goal here is to improve nonfunctional attributes like readability, maintainability, and performance. Common strategies include renaming variables, simplifying complex methods, or removing duplicate code.
Redesign, by contrast, involves a fundamental overhaul of the software architecture. This might be necessary when the current design becomes too cumbersome or fails to accommodate new requirements. Redesign often leads to a complete rewrite or a significant restructuring of components to enhance scalability and adaptability.
When to Choose Refactoring
1. Incremental Improvement
Refactoring is entirely beneficial when you're looking for incremental improvements. If the current codebase functions correctly but has areas of inefficiency or poor readability, refactoring can enhance the quality without introducing new risks.
Example: Refactoring a Method
// Before Refactoring
public void calculateUserBalance(User user) {
// Long method doing many things
double balance = 0;
// Complex logic
balance += user.getDeposits().stream().mapToDouble(Deposit::getAmount).sum();
balance -= user.getWithdrawals().stream().mapToDouble(Withdrawal::getAmount).sum();
logBalance(balance);
}
// After Refactoring
public void calculateUserBalance(User user) {
double balance = getTotalDeposits(user) - getTotalWithdrawals(user);
logBalance(balance);
}
private double getTotalDeposits(User user) {
return user.getDeposits().stream().mapToDouble(Deposit::getAmount).sum();
}
private double getTotalWithdrawals(User user) {
return user.getWithdrawals().stream().mapToDouble(Withdrawal::getAmount).sum();
}
In this refactor, the original method is simplified into smaller, more focused methods that improve readability. The code still performs the same function—calculating a user’s balance—but it’s much clearer.
2. Near-Term Deadlines
If you are under tight deadlines, refactoring may be your best friend. The risk involved in a complete redesign during critical periods can thwart your timelines. Opting for refactoring allows you to make improvements while keeping the project moving forward.
3. High Code Quality
When your code quality is generally high but contains a few pain points, refactoring can effectively address those issues. For instance, a legacy codebase with some messy sections might benefit from targeted refactoring to bring those sections up to modern standards.
4. Team Familiarity
If the team is familiar with the current codebase, refactoring is often a smoother path. The knowledge team members possess about the existing functionalities reduces the learning curve and minimizes the likelihood of introducing bugs.
When to Choose Redesign
1. Major Architectural Limitations
However, there are times when redesign is not just beneficial but necessary. If the existing architecture poses significant limitations, it’s time to rethink the structure thoroughly. For instance, if your app is struggling with performance and scalability due to its design, a redesign can alleviate those issues.
2. Dramatic Feature Additions
When significant new features need to be integrated, it may be more efficient to redesign certain modules than to struggle with patching them onto an outdated structure. Redesign allows you to create new functionalities without the constraints of legacy code.
3. Technology Updates
As technology evolves, so do the best practices regarding software design. If your codebase relies heavily on outdated technologies or methods, redesigning it with modern paradigms can make it more maintainable and adaptable for future growth.
4. Team Restructuring
If your team has undergone significant changes (e.g., new developers joining with divergent coding styles), it might benefit from a redesign. A fresh start can foster a more cohesive environment, with unified coding standards eliminating past inconsistencies.
Making the Choice: Refactoring or Redesign?
1. Evaluate Your Goals
Begin by clarifying the objectives for your codebase. What problems are you currently facing? Is maintainability a primary concern, or do you need a more robust design that accommodates growth?
2. Assess the Risk
Every change carries some degree of risk, whether it is refactoring or redesigning. Evaluate the potential impact on your system and consider running tests to identify possible issues before proceeding.
3. Involve Your Team
Involve your development team in the discussion. Gather insights on their experiences with the current codebase. Practitioners often provide unique perspectives that can inform the decision-making process.
4. Conduct a Cost-Benefit Analysis
Perform a cost-benefit analysis for both refactoring and redesign. Consider the time investment, potential downtime, and long-term benefits. Often, a blend of both approaches provides the best results.
Final Considerations
The decision between refactoring and redesign isn’t purely binary. You might find a solution that combines both strategies as you evolve your codebase. Engaging in situational assessments to identify the right approach is imperative for sustaining an effective software development cycle.
To enhance your understanding of code quality and maintainability, consider referring to additional resources:
- Martin Fowler - Refactoring
- Robert C. Martin - Clean Code
Ultimately, whether you choose to refactor or redesign, the goal remains the same: to maintain a codebase that serves its purpose efficiently and evolves to meet future challenges. Making informed, thoughtful decisions about your code will yield dividends for your project and your team.
Happy coding!