Why Reflection Can Break Your Code: A Cautionary Guide
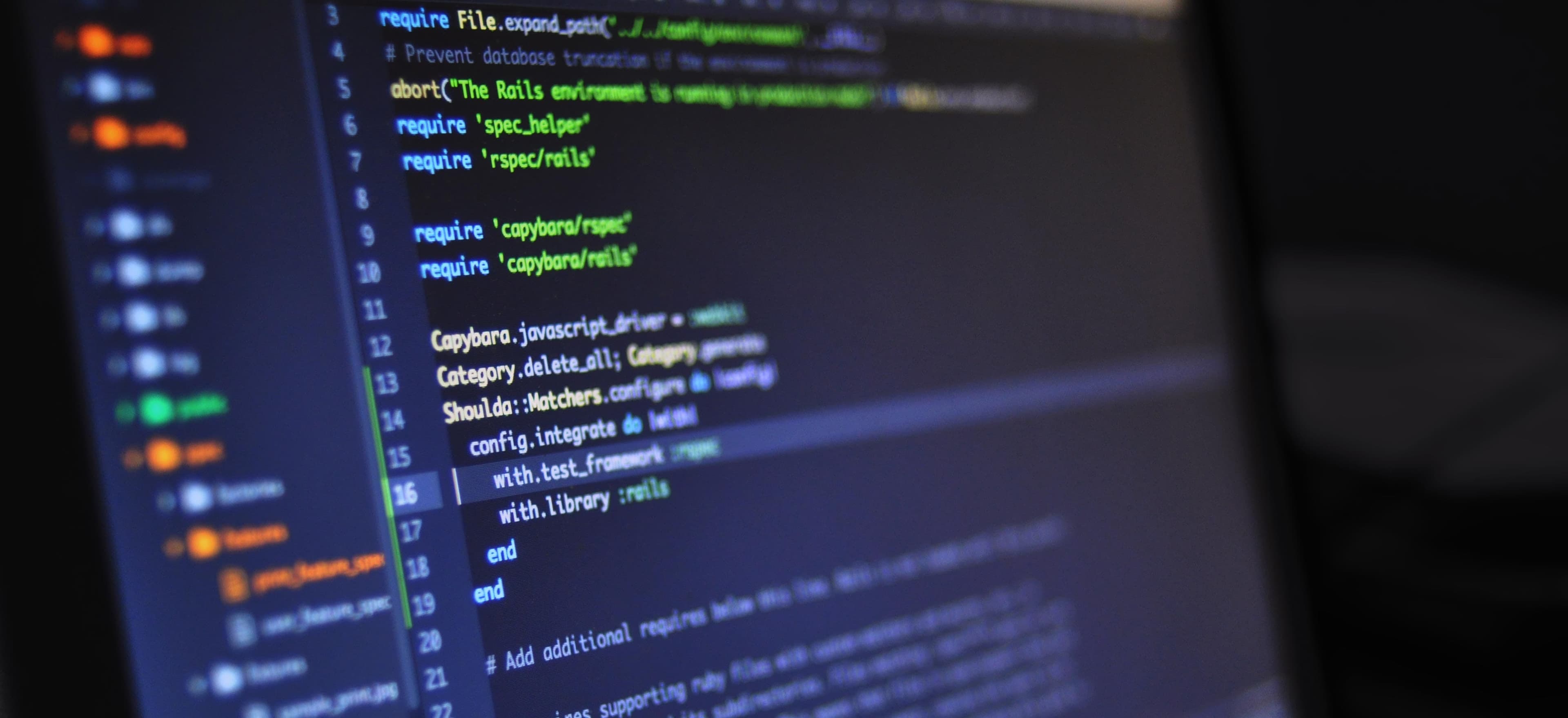
- Published on
Why Reflection Can Break Your Code: A Cautionary Guide
Reflection is a powerful feature of the Java programming language that allows developers to inspect classes, interfaces, fields, and methods at runtime, without knowing the names of the classes beforehand. It can be extremely useful for certain tasks, like building frameworks, serialization, and even for testing. However, with great power comes great responsibility. In this blog post, we will explore the potential pitfalls of using reflection, how it can break your code, and what developers should consider to avoid these issues.
Understanding Reflection in Java
Before we delve into the downsides, let’s clarify what reflection is and how it is implemented in Java.
Java's java.lang.reflect
package includes classes that provide the ability to examine and manipulate classes and objects at runtime. This is what makes reflection so flexible. Here’s a simple example of using reflection to obtain the class name and its methods.
import java.lang.reflect.Method;
public class ReflectionExample {
public static void main(String[] args) throws Exception {
// Get the class object associated with a given class name
Class<?> clazz = Class.forName("java.lang.String");
// Print the name of the class
System.out.println("Class Name: " + clazz.getName());
// Get and print all declared methods
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
System.out.println("Method: " + method.getName());
}
}
}
Why Use Reflection?
- Dynamic Access: Access methods and fields regardless of visibility (private, protected, etc.).
- Framework Development: Easily implement dynamic object creation and manipulation.
- Testing: Access private methods for testing purposes when necessary.
The Dark Side of Reflection
Despite its advantages, over-relying on reflection can lead to several problems. Let's break down the major concerns:
1. Performance Overhead
Using reflection is typically slower than direct method invocation. This is primarily because:
- The Java Virtual Machine (JVM) cannot optimize reflective calls as well as regular calls.
- Reflection requires some additional checks and operations, which add up in performance-critical applications.
Example:
Consider the difference between invoking a method directly versus using reflection.
public class PerformanceExample {
public void display() {
System.out.println("Display method called");
}
}
// Using reflection
public void invokeDisplayMethodWithReflection(Object obj) throws Exception {
Method method = obj.getClass().getMethod("display");
method.invoke(obj);
}
Here, the reflection call to invoke
is slower due to the extra checks performed by the JVM.
2. Lack of Compile-Time Safety
With reflection, many errors only surface at runtime, making code less safe. For example, you might miss a typo in a method name until you attempt to run the code.
// Incorrect method name 'displey'
Method method = obj.getClass().getMethod("displey");
This mistakes would cause a NoSuchMethodException
at runtime, which can lead to unpredictable application behavior.
3. Code Maintenance Issues
Using reflection can obscure what your code is doing, making it harder to read and maintain. It may not be immediately clear to other developers (or even yourself later on) which methods or fields are being utilized.
// Reflection can hide implementation details
Field privateField = obj.getClass().getDeclaredField("privateField");
privateField.setAccessible(true);
Object value = privateField.get(obj);
In this example, accessing private fields through reflection can lead to confusion. Future modifications to the class structure may inadvertently break the reflective accesses.
4. Security Concerns
Because reflection bypasses access control checks, it can be a potential security risk. If you expose reflective access in a public API, malicious users might gain access to sensitive data.
For example, the code below will throw an IllegalAccessException
if a security manager is present and not allowing access.
Field secretField = SecurityClass.class.getDeclaredField("secret");
secretField.setAccessible(true); // This could lead to security breaches
5. Fragile Code
As applications evolve, minor changes in your codebase can lead to breaking reflective calls, as they rely heavily on specific structures. This unpredictability creates fragile code that is prone to unexpected behavior when refactoring.
Whenever class methods or names are altered, existing reflective calls may break, leading to potential runtime errors.
Best Practices to Mitigate Reflection Risks
To harness the power of reflection without losing code stability, consider the following best practices:
1. Avoid Reflection When Possible
Before resorting to reflection, ask if there's an alternative approach that doesn't compromise performance and maintainability. For instance, using interfaces or strategy patterns often yields cleaner and more maintainable code.
2. Limit Its Scope
Use reflection only in areas of your application where it is truly necessary, such as framework development or testing. Keep usage localized to minimize the risk of widespread issues.
3. Use Strong Typing
Consider wrapping reflective calls in methods that perform necessary type checks or handle exceptions gracefully. By doing so, you can centralize the handling of reflection-related issues.
public class SafeReflector {
public static <T> T invokeMethod(Object obj, String methodName) {
try {
Method method = obj.getClass().getMethod(methodName);
return (T) method.invoke(obj);
} catch (Exception e) {
// Handle specific cases or log
e.printStackTrace();
return null;
}
}
}
4. Document Reflection Usage
Ensure that all reflective accesses in your code are well-documented so that other developers can easily understand the intent and underlying mechanisms.
5. Monitor Performance
If reflection is a necessity, keep a keen eye on performance metrics. Profiling tools can help you evaluate the impact of reflection on your application’s overall performance.
Final Thoughts
Reflection in Java is a double-edged sword. While it can offer the flexibility and power needed in certain situations, it also presents significant risks in terms of performance, safety, and maintainability. By understanding the drawbacks and following prudent guidelines, you can effectively harness this feature without compromising the stability and reliability of your code.
If you are interested in diving deeper into the topic of reflection or exploring additional resources, don’t hesitate to check out Oracle's official documentation for a comprehensive overview.
Feel free to share your own experiences with reflection and how you managed its potential pitfalls in the comments below!