Common Pitfalls in Java EE to Microservices Migration
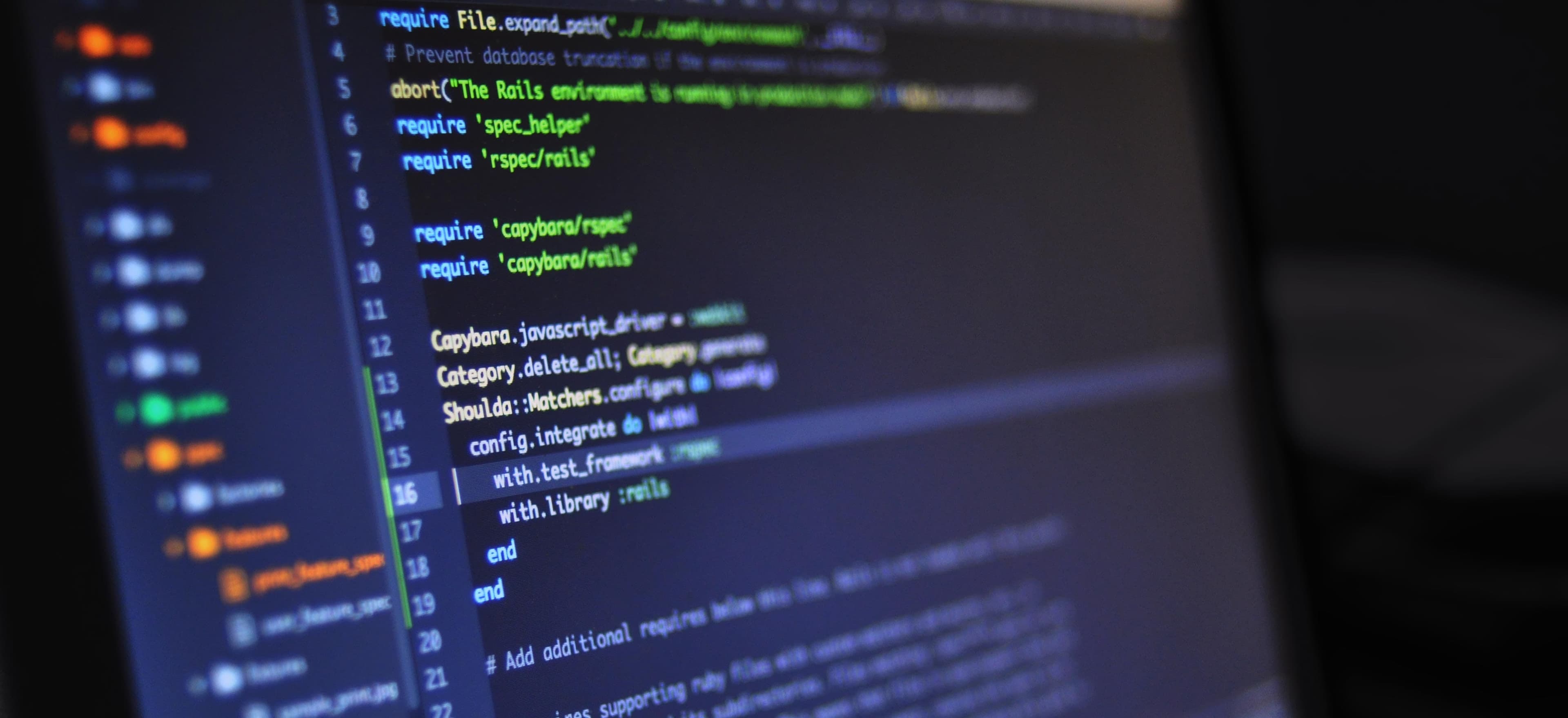
- Published on
Common Pitfalls in Java EE to Microservices Migration
Migrating a Java EE application to a microservices architecture can be a daunting task. While the benefits of microservices are plentiful—improved scalability, greater resilience, and faster deployment cycles—there are several common pitfalls that organizations may encounter during this transition. This blog post aims to cover these pitfalls in detail while offering insights into how to navigate around them.
Understanding Java EE and Microservices
Before diving into the pitfalls, let’s briefly clarify what we mean by Java EE and microservices.
-
Java EE (Enterprise Edition): This is a set of specifications that extend the Java SE (Standard Edition) with specifications for enterprise features such as distributed computing and web services. It has traditionally favored monolith architecture, where all application components are tightly coupled and deployed as a single unit.
-
Microservices: This architectural style enables an application to be developed as a suite of small, independent services, each running in its own process. The primary attribute of microservices is that they can be developed, deployed, and scaled independently of each other.
Common Pitfalls During Migration
1. Lack of Understanding of Microservices
Pitfall: A common mistake is assuming that just breaking a monolith into smaller parts automatically results in microservices.
Resolution: It’s crucial to understand the principles of microservices architecture. The focus should be on building services that are independently deployable and maintainable, with a clear definition of service boundaries.
Example:
// Example of a microservice for user management
@RestController
@RequestMapping("/users")
public class UserController {
private final UserService userService;
public UserController(UserService userService) {
this.userService = userService;
}
@GetMapping("/{id}")
public ResponseEntity<User> getUser(@PathVariable String id) {
return ResponseEntity.ok(userService.findById(id));
}
}
Commentary: This controller demonstrates a typical REST endpoint in a microservice architecture. Notice how it's not attached to the rest of the application—which allows it to be scored independently.
2. Underestimating the Complexity of Distributed Systems
Pitfall: Transitioning to microservices often leads to underestimating the complexity of managing a distributed system. Issues like latency, eventual consistency, and data management become critical.
Resolution: Invest time in mastering distributed systems concepts. Leverage patterns like Circuit Breaker and Event Sourcing to deal with these complexities effectively.
Example:
// Using a Circuit Breaker to handle potential outages in a service
@Service
public class InventoryService {
@CircuitBreaker(fallbackMethod = "fallbackInventoryCheck")
public Inventory checkInventory(String productId) {
// Call to an external service to check inventory
return inventoryClient.getInventory(productId);
}
public Inventory fallbackInventoryCheck(String productId, Throwable t) {
// Provide a default response in case of failure
return new Inventory(productId, 0);
}
}
Commentary: Here, the Circuit Breaker pattern helps ensure that the system remains resilient in the face of service outages.
3. Not Decoupling Services Properly
Pitfall: Merely splitting a monolith into services without decoupling them can lead to a situation called "micro-monoliths," where services are still tightly coupled.
Resolution: Use Domain-Driven Design (DDD) to define service boundaries clearly. Each microservice should be able to operate independently without depending on the others.
4. Ignoring DevOps Practices
Pitfall: The migration process often overlooks the importance of DevOps practices, which are essential for deploying and managing microservices effectively.
Resolution: Establish a strong CI/CD (Continuous Integration/Continuous Deployment) pipeline to streamline the development and deployment processes.
Example:
# Sample GitHub Actions configuration for CI/CD
name: CI
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up JDK 11
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Build with Maven
run: mvn clean install
Commentary: Automating the build process through CI/CD helps to quickly validate and deploy changes made to the microservices.
5. Failing to Monitor and Log Effectively
Pitfall: Lack of proper monitoring can result in an inability to diagnose issues quickly, leading to downtimes.
Resolution: Implement robust logging and monitoring tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Prometheus for metrics collection.
6. Neglecting Security Aspects
Pitfall: Security measures can often take a back seat in the rush to migrate. Each microservice has its own security considerations, and overlooking them can lead to vulnerabilities.
Resolution: Implement security at both the network and application levels. Use OAuth2 for authentication and consider API Gateway solutions for managing service-to-service communication.
Example:
// Example of securing a REST endpoint with Spring Security
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/users/**").authenticated()
.and()
.oauth2Login();
}
}
Commentary: Security configurations can protect your services while ensuring that users can log in without compromising their data.
7. Over-Engineering Microservices
Pitfall: It's easy to fall into the trap of over-engineering when designing microservices.
Resolution: Keep it simple and start with a few services. Focus on delivering functionality before implementing complex solutions like service meshes unless necessary.
8. Underestimating Data Management
Pitfall: In a monolithic architecture, it’s easier to manage data using a single database. In microservices, each service often has its own database, leading to challenges.
Resolution: Enforce data ownership principles. Design your data models to fit the domain, and understand that consistency may be different.
Example:
// A simple model showing how a microservice may handle its data
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
// relationships, etc.
}
Commentary: Each service manages its database schema, ensuring service autonomy but requiring careful consideration for data consistency.
To Wrap Things Up
Migrating from Java EE to a microservices architecture can be fraught with pitfalls, but it can also yield immense benefits when done correctly. By understanding the principles of microservices, managing complexity, and applying best practices in DevOps, security, and data management, organizations can navigate the migration process successfully.
Additional Resources
For further reading on migrating to microservices, consider the following resources:
- Microservices Patterns
- Domain-Driven Design: Tackling Complexity in the Heart of Software
- Spring Boot Documentation
By being mindful of these pitfalls and leveraging the right tools and frameworks, you will be well on your way to successfully adopting microservices architecture in your organization. Happy coding!