Common Debugging Pitfalls and How to Avoid Them
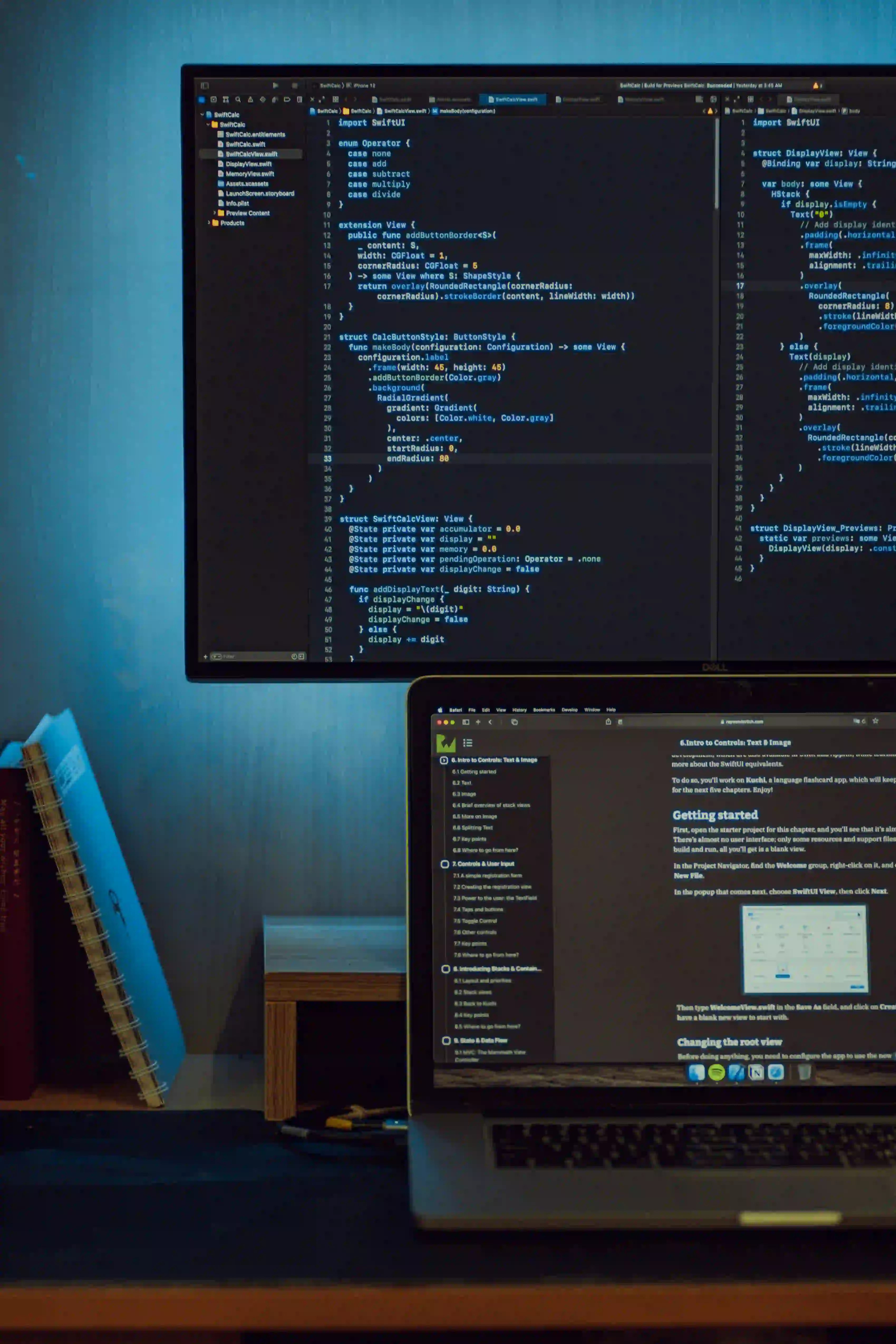
Common Debugging Pitfalls and How to Avoid Them
Debugging is an essential skill for any software developer, especially when working with languages like Java. Efficient debugging can distinguish between a smooth development process and a frustrating ordeal that seems to stretch endlessly. In this blog post, we will explore common debugging pitfalls and how to avoid them, enhancing your Java coding experience and productivity.
Understanding the Debugging Process
Before delving into the common pitfalls, it is crucial to understand what debugging entails. Debugging is the process of identifying and fixing bugs or errors in your code. These bugs can range from semantic mistakes to logical flaws. Mastering debugging is as important as mastering the programming language itself.
Common Debugging Pitfalls
1. Overlooking Compilation Errors
Java is a statically typed language, meaning it enforces strict type checks at compile time. Thus, compilation errors are common in Java applications.
Pitfall: Developers often underestimate these errors and proceed to run their applications without resolving them.
How to Avoid: Always pay attention to compilation errors. Fix them immediately as they provide vital information about the faulty line of code and its type.
Code Snippet Example:
// Wrong data type assignment
int num = "123"; // Compilation error: incompatible types
In this example, the developer has tried to assign a string to an integer variable. The compiler catches this mismatch, preventing runtime errors.
2. Ignoring Stack Traces
Stack traces show where an exception occurred in your code. They can be invaluable in debugging.
Pitfall: Developers might glance over the stack trace or fail to read it in its entirety.
How to Avoid: Always analyze stack traces. They typically specify the class, method, and line number where the issue occurred.
Code Snippet Example:
try {
int[] array = new int[5];
System.out.println(array[10]); // This will throw an ArrayIndexOutOfBoundsException
} catch (Exception e) {
e.printStackTrace(); // Prints stack trace for debugging
}
// Expect output showing the error and where it occurred
When an ArrayIndexOutOfBoundsException
occurs, the stack trace will guide you straight to the source of the error.
3. Assumptions in Variable States
Making assumptions about variable states can lead to overlooking issues in your code.
Pitfall: Developers assume that variables’ states at certain points in code execution remain constant.
How to Avoid: Utilize logging to print out variable states to confirm their values before and after key operations.
Code Snippet Example:
public void process(int value) {
System.out.println("Value before processing: " + value);
// Processing logic
value += 10;
System.out.println("Value after processing: " + value);
}
By logging variable states, you can track changes and identify issues easier.
4. Neglecting to Use Debug Tools
Tools such as Integrated Development Environments (IDEs) offer debugging features that can simplify the process.
Pitfall: Some developers debug by adding print statements and fail to leverage the debugging tools available in their IDE.
How to Avoid: Familiarize yourself with your IDE’s debugging tools. Learn to set breakpoints, inspect variables in real-time, and step through code line by line.
Example of Using a Debugger
In IntelliJ IDEA or Eclipse, you can right-click on the editor and select "Debug". This allows you to set breakpoints. When you run the debugger, the execution stops at the breakpoint letting you inspect current states.
5. Focusing on Symptoms Rather Than Causes
It is easy to become distracted by the immediate symptoms of a bug, instead of investigating what caused the issue in the first place.
Pitfall: Applying quick fixes to symptoms instead of the root cause can lead to recurring problems.
How to Avoid: Use a methodical approach to tracing back from symptoms to their root causes. Ask, "What led to this condition?"
6. Lack of Version Control
Version control systems like Git are invaluable for managing your codebase.
Pitfall: Developers sometimes find it hard to revert to previous code versions when a new change introduces bugs.
How to Avoid: Commit your code frequently with meaningful messages. This way, you can easily track down changes that led to bugs.
Code Snippet Example:
git commit -m "Fix indexing in the data processing algorithm"
Having clear commit messages can help you rapidly identify why a change was made.
7. Ignoring Test Cases
Unit tests are critical in preventing bugs from slipping into production.
Pitfall: It's tempting to skip writing tests, thinking they add unnecessary overhead to the development process.
How to Avoid: Incorporate test-driven development (TDD) into your routine. Write test cases before implementing features. This will encourage you to think critically about potential pitfalls.
Unit Test Example:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class ArithmeticTest {
@Test
public void testAddition() {
Arithmetic arithmetic = new Arithmetic();
assertEquals(5, arithmetic.add(2, 3)); // Testing the add method
}
}
Unit tests validate that your code functions as expected, catching errors early.
Resources for Further Learning
- Effective Java by Joshua Bloch - A definitive guide rich with advice on best practices.
- The Pragmatic Programmer - Contains insightful tips and best practices for software development, including debugging strategies.
Key Takeaways
Debugging is an invaluable skill for software developers, especially when written in Java. By avoiding common pitfalls and adopting best practices, you can streamline your debugging process. Ensure to always pay attention to compilation errors, analyze stack traces thoroughly, and employ the debugging tools in your development environment. Remember, methodical investigation will address the root of issues rather than just its symptoms.
By incorporating testing into your routine and leveraging version control, you will not only become a more effective debugger but also enhance the quality of your code. Happy coding and debugging!
Feel free to share your thoughts and experiences concerning debugging in Java in the comments below!