Improving NPE Error Messages with Mockito Tips
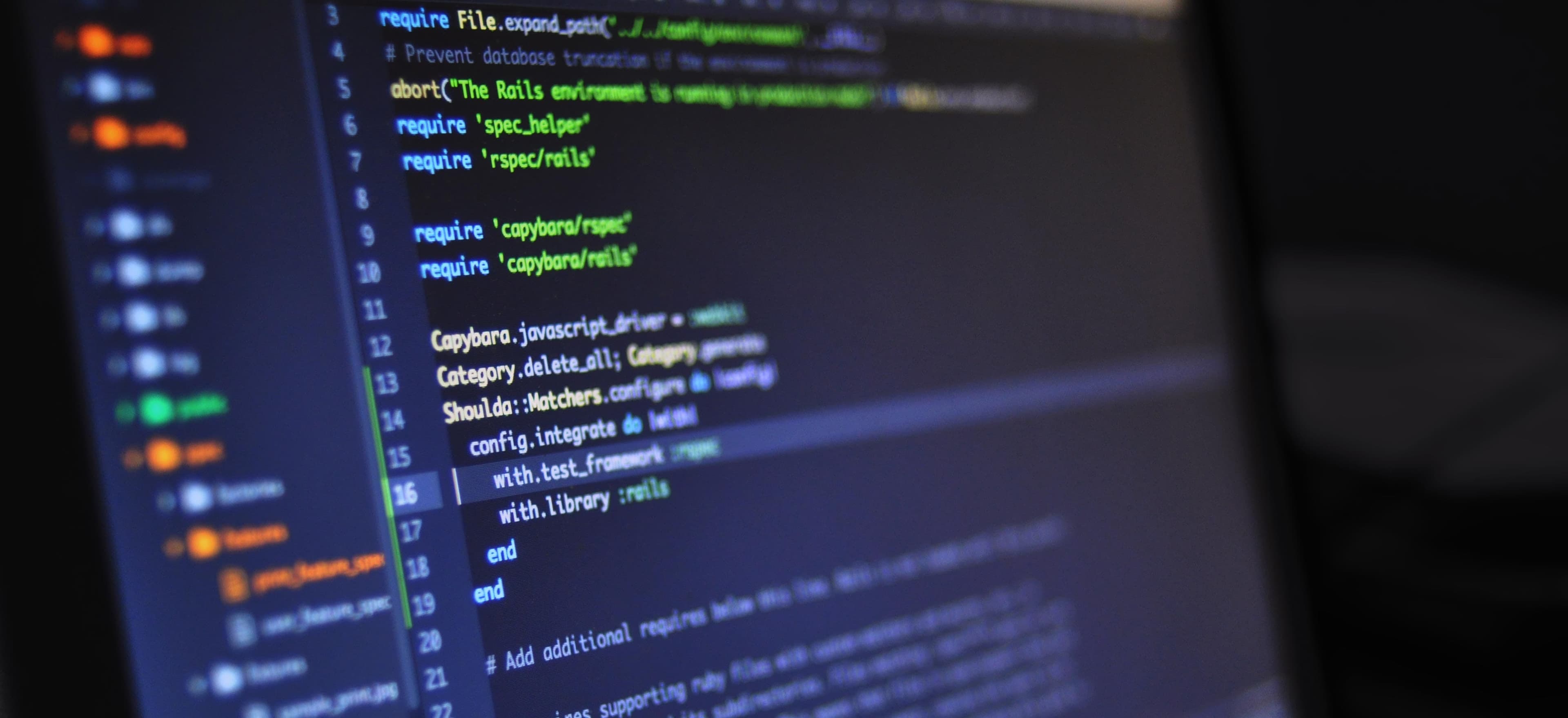
- Published on
Improving NPE Error Messages with Mockito: Tips for Effective Testing
The NullPointerException (NPE) is one of the most common exceptions that developers encounter in Java. It usually occurs when the JVM attempts to access an object or its methods that happen to be null. These errors can be notoriously difficult to diagnose, leading to wasted time and frustration. Fortunately, the Mockito framework can aid in improving the clarity of these NPE error messages, making debugging easier.
In this blog post, we’ll explore how to utilize Mockito effectively to improve error messages associated with NPE in your Java applications. We'll discuss the best practices for mocking objects and provide helpful code snippets to illustrate the concepts.
Table of Contents
- What is Mockito?
- Understanding NullPointerException (NPE)
- Why Improve NPE Messages?
- Mocking Objects in Mockito
- Tips for Improving NPE Messages
- Code Examples
- Conclusion
1. What is Mockito?
Mockito is a popular Java-based mocking framework used for unit testing. It allows developers to create mock objects, which can simulate the behavior of real objects in a controlled way. This becomes incredibly useful when testing components in isolation, particularly when those components rely on external dependencies.
2. Understanding NullPointerException (NPE)
A NullPointerException occurs when a program attempts to use an object reference that has the null value. For instance:
public String getCustomerName(Customer customer) {
return customer.getName(); // Throws NPE if customer is null
}
In the code above, if customer
passed to the getCustomerName
method is null, the JVM throws an NPE, which can lead to confusion without additional context.
3. Why Improve NPE Messages?
Improving the clarity of NPE messages can significantly reduce debugging time. When you're dealing with a large codebase, vague exception messages make it challenging to locate the source of the problem. By improving error messages, you gain valuable insights into what went wrong, especially if you're integrating with third-party services or complex object states.
4. Mocking Objects in Mockito
Mocking in Mockito allows developers to create a simplistic representation of an object to simulate various scenarios without relying on the actual object. This is incredibly useful for testing methods that rely on objects which could be stateful or costly to instantiate.
5. Tips for Improving NPE Messages
Here are some effective strategies you can use to enhance your NPE messages using Mockito:
a. Validate Method Parameters
Always validate that method parameters aren’t null before proceeding with method logic.
public String getCustomerDetails(Customer customer) {
if (customer == null) {
throw new IllegalArgumentException("Customer cannot be null.");
}
return customer.getDetails();
}
This type of validation can prevent NPEs by providing a meaningful message upfront.
b. Use Mockito's Argument Matchers
If you're using Mockito, leverage its argument matchers for more informative assertions:
import static org.mockito.Mockito.*;
@Test
public void testGetCustomerDetails_NPE() {
Customer mockCustomer = mock(Customer.class);
when(mockCustomer.getDetails()).thenReturn(null);
assertThrows(IllegalArgumentException.class, () -> {
getCustomerDetails(mockCustomer);
});
}
In the code above, we anticipate an IllegalArgumentException
before invoking getCustomerDetails
, improving visibility into expected failure cases.
c. Leverage Annotations
Use Mockito's annotations to enhance readability and manage dependencies effectively.
@RunWith(MockitoJUnitRunner.class)
public class CustomerServiceTest {
@Mock
private CustomerRepository customerRepo;
// Other test code...
}
Utilizing @RunWith(MockitoJUnitRunner.class)
and @Mock
minimizes boilerplate code and enhances clarity in your tests.
d. Customize Mocha's Output
Consider customizing how Mockito reports errors:
import static org.mockito.Mockito.*;
public class CustomerServiceTest {
@Test
public void shouldThrowNPEWhenCustomerIsNull() {
CustomerService customerService = new CustomerService();
// Log error message with context
assertThrows(NullPointerException.class, () -> {
customerService.getCustomerName(null);
});
}
}
Here, we've written a test to throw NPE with the assertion clearly stated, encapsulated with meaningful context that aids in debugging.
6. Code Examples
Let's put everything into practice with comprehensive examples:
Example 1: Mocking and Validating Input
public class OrderService {
public double calculateTotal(Order order) {
if (order == null) {
throw new IllegalArgumentException("Order cannot be null");
}
// Assume getItems() could return null
return order.getItems().stream()
.mapToDouble(Item::getPrice)
.sum();
}
}
In this example, a null check prevents an NPE from occurring. The message “Order cannot be null” clearly communicates the issue.
Example 2: Unit Test with Mockito
import org.junit.Test;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
import static org.mockito.Mockito.*;
public class OrderServiceTest {
@InjectMocks
private OrderService orderService;
@Mock
private Order mockOrder;
public OrderServiceTest() {
MockitoAnnotations.openMocks(this);
}
@Test(expected = IllegalArgumentException.class)
public void whenOrderIsNull_calculateTotal_throwsException() {
orderService.calculateTotal(null);
}
}
This unit test clearly asserts the expectation of behavior when the method is called with a null value.
7. Conclusion
The importance of managing NPE correctly cannot be overstated, especially in complex applications where debugging can become a time-consuming task. Utilizing Mockito gives you the tools to clearly articulate your expectations and goals in your tests. Validating parameters, maximizing mock configurations, and leveraging comprehensive assertions can help you pinpoint issues quickly and efficiently.
By following the tips outlined in this blog post, you can significantly reduce the ambiguity surrounding NullPointerExceptions in your Java applications. The next time you’re faced with an NPE, you’ll be better equipped to find and fix the root cause.
For more information on Mockito, you can check out the official documentation. Happy coding!
Feel free to use these techniques in your testing practices to make your code more robust and fault-tolerant!