Dealing with Java's Optional: The IsEmpty Dilemma
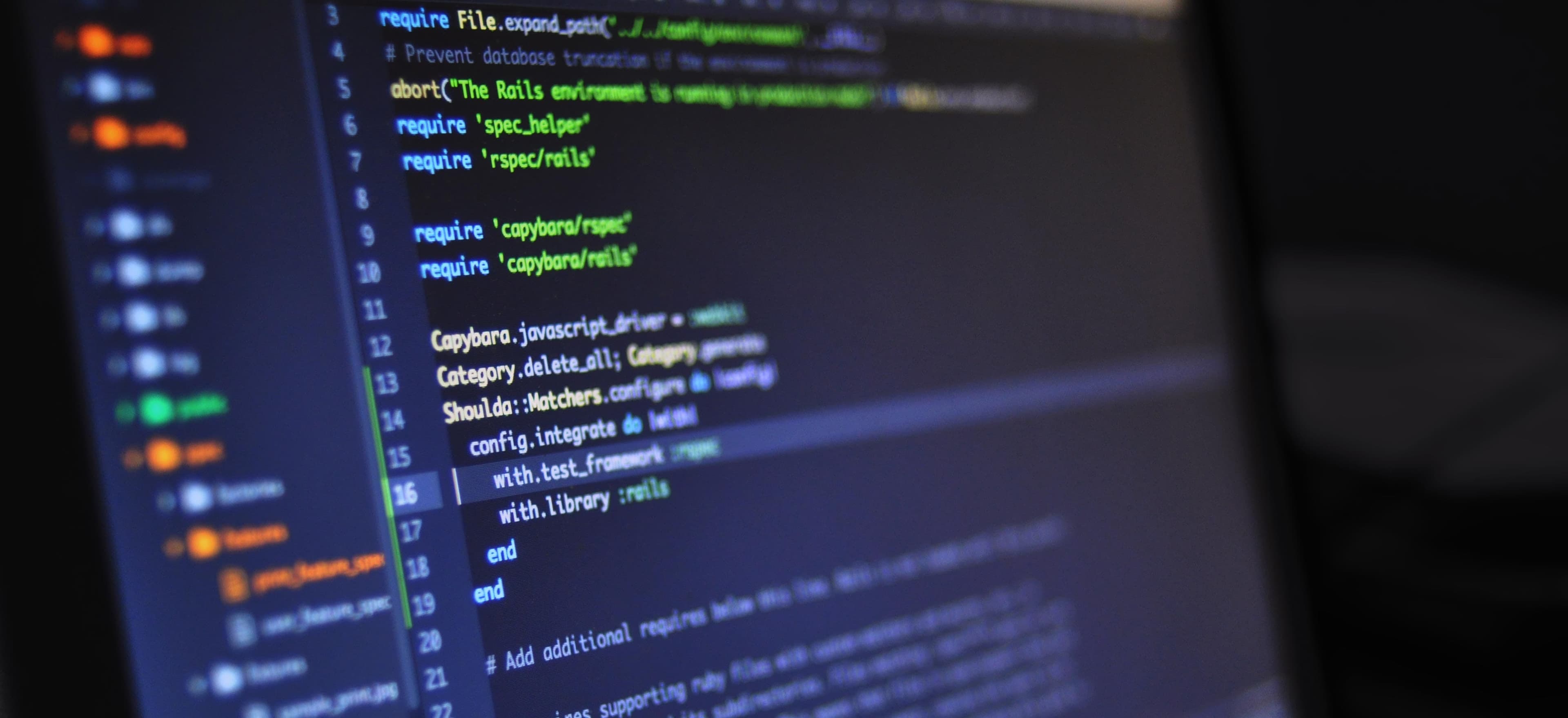
- Published on
Dealing with Java's Optional: The IsEmpty Dilemma
Java's Optional
class, introduced in Java 8, has significantly changed how developers approach nullability and the common pitfalls that arise from it. While it's a powerful tool for handling optional values, it often raises questions, particularly around its methods, such as isPresent
, isEmpty
, and other associated functionalities. This blog will explore the Optional
class in depth, focusing on the isEmpty
dilemma and how to effectively utilize Optional
in your Java projects.
Understanding Optional
Before diving deep, let’s clarify what Optional
is. Essentially, Optional
is a container that can either contain a value or be empty. It's designed to prevent NullPointerExceptions
by enforcing a programming model where values are either present or absent, rather than null.
The Basic Structure
An Optional
instance can be created in various ways. Here’s a basic overview:
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
Optional<String> optionalWithValue = Optional.of("Hello, World!");
Optional<String> emptyOptional = Optional.empty();
System.out.println(optionalWithValue.isPresent()); // true
System.out.println(emptyOptional.isPresent()); // false
}
}
In the above code, Optional.of
creates an Optional
containing a non-null value, while Optional.empty
creates an empty Optional
. The isPresent()
method checks if the Optional
contains a value.
The Dilemma of isEmpty
With the introduction of Java 11, we now have the isEmpty()
method to check if an Optional
is empty. However, this raises some questions among developers:
- Should you use
isPresent()
orisEmpty()
? - When is it appropriate to check for emptiness?
Good Practices
-
Use
isEmpty()
for Clarity:isEmpty()
is more readable than using!optional.isPresent()
. It clearly conveys the intent of checking for absence. -
Avoid Redundant Checks: Using both
isPresent()
andisEmpty()
can lead to redundancy. Opt for one method based on your requirement. -
Embrace Functional Programming: Instead of check-then-act, leverage
Optional
methods likemap
,flatMap
, ororElse
. For example:
String result = optionalWithValue
.map(value -> value.toUpperCase())
.orElse("Default Value");
System.out.println(result); // HELLO, WORLD!
In this example, we transform the value if present, and provide a default if absent, avoiding explicit checks.
Key Functionalities of Optional
Creating Optional Instances
You can create Optional
instances in various ways:
Optional.of(T value)
: ThrowsNullPointerException
if value is null.Optional.ofNullable(T value)
: Creates anOptional
containing the value if non-null, or empty otherwise.Optional.empty()
: Creates an emptyOptional
.
Working with Optional Value
Here’s a breakdown of some useful methods:
isPresent()
: Checks if a value is present.isEmpty()
: Returns true if no value is present.ifPresent(Consumer<? super T> action)
: Executes the given action if a value is present.
Example:
optionalWithValue.ifPresent(value -> System.out.println(value));
orElse(T other)
: Returns the value if present, otherwise returnsother
.
Example:
String message = optionalWithValue.orElse("Default Message");
System.out.println(message); // Hello, World!
-
orElseGet(Supplier<? extends T> other)
: Similar toorElse
, but the other value is generated only if required. -
orElseThrow(Supplier<? extends X> exceptionSupplier)
: Returns the value if present; otherwise, it throws an exception provided by the supplier.
Practical Use Cases
Let's analyze a couple of real-world scenarios where Optional
shines.
Example: Avoiding Null Values in Method Return Types
Suppose you have a service that retrieves user data from a database. Instead of returning null when a user doesn't exist, you could return an Optional
.
public Optional<User> findUserById(int id) {
User user = fetchUserFromDatabase(id); // Simulating a database fetch
return Optional.ofNullable(user); // Wrapping the user in an Optional
}
Usage:
Optional<User> userOptional = findUserById(1);
String userName = userOptional.map(User::getName).orElse("Anonymous");
System.out.println(userName);
This approach eliminates the risk of NullPointerException
and forces consumers of the method to handle the absent case explicitly.
Example: Chaining Operations
Consider a scenario where you have to retrieve and process user preferences. Instead of nesting multiple checks, you can leverage Optional
to chain operations neatly.
public Optional<Preference> getUserPreference(User user) {
return Optional.ofNullable(user)
.flatMap(User::getPreferences) // If user exists, get preferences
.flatMap(preferences -> preferences.stream().findFirst()); // Get the first preference
}
Final Considerations
Java's Optional
is a powerful construct designed to enhance the way we deal with nullable values. By providing clear methods like isPresent()
and isEmpty()
, it encourages better code practices that reduce the likelihood of encountering NullPointerException
.
In the context of isEmpty
, using it can improve code readability and expressiveness. Remember to embrace functional programming paradigms that Optional
facilitates, utilizing methods like map
, flatMap
, and orElse()
which provide elegant solutions to managing optional values.
Further Reading
For more in-depth reading on the Optional
class and its usage, consider these resources:
- Java Optional Documentation
- Java Magazine: Optional - The Java 8 Way of Null Handling
By making the paradigm shift toward using Optional
, you can write cleaner, safer, and more maintainable code. Embrace the power of Optional
and eliminate null-related issues once and for all!
Checkout our other articles