Fixing Static Resource Issues in Spring Boot SPAs
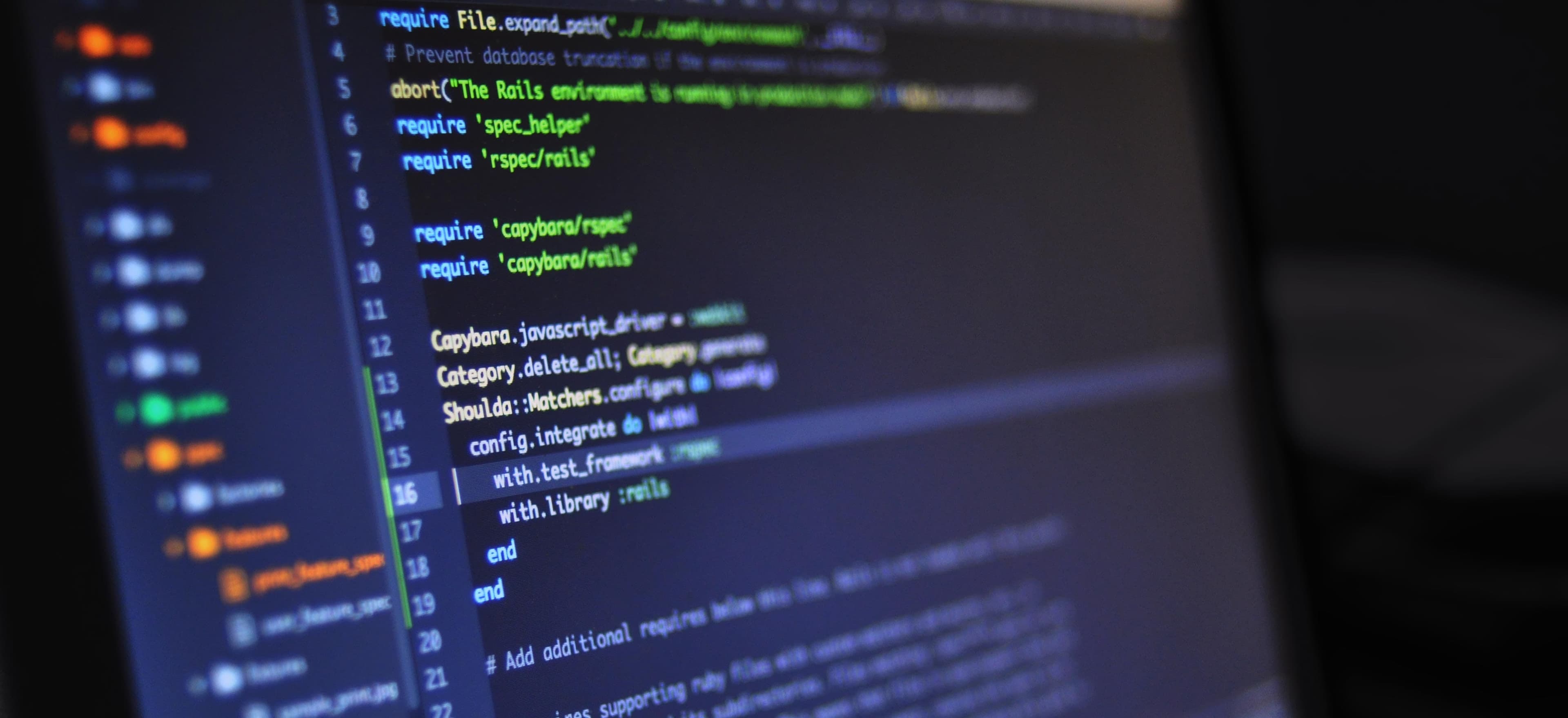
- Published on
Fixing Static Resource Issues in Spring Boot SPAs
Spring Boot has gained immense popularity for developing web applications, especially for Single Page Applications (SPAs). However, developers often face challenges when it comes to serving static resources effectively. This blog post explores some common static resource issues encountered in Spring Boot SPAs, and it provides practical solutions to ensure your application runs smoothly.
Understanding Static Resource Management in Spring Boot
In a typical Spring Boot application, static resources (like HTML, CSS, JavaScript files, etc.) are usually placed in the src/main/resources/static
directory. By default, Spring Boot serves these files over HTTP from the root URL. For example, if you place a file named styles.css
inside the static
folder, it can be accessed via http://localhost:8080/styles.css
.
Here's a brief overview of the default static resource locations in Spring Boot:
src/main/resources/static
src/main/resources/public
src/main/resources/resources
src/main/resources/META-INF/resources
This is where the problem begins—in SPAs, the client-side routing can interfere with the way Spring Boot handles these resources.
Common Issues with Static Resources in SPAs
1. Browser Caching
Browsers usually cache static resources to improve loading times, and sometimes this leads to outdated files being served. If you deploy a new version of your application but don’t change the file names, users might not see the updates.
Fixing Browser Caching
To manage browser caching, you can append a query string or use build tools to generate unique file names based on the content hash.
# Example using Maven for frontend assets
mvn clean package -DskipTests
You can also set cache control for static resources within your application by creating a configuration class.
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
import org.springframework.web.servlet.config.annotation.ResourceHandlerRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/**")
.addResourceLocations("classpath:/static/")
.setCachePeriod(3600); // Cache for 1 hour
}
}
2. Routing Issues
In SPAs, frameworks like React, Angular, and Vue handle client-side routing. If a user refreshes the page or directly navigates to a URL such as http://localhost:8080/home
, the Spring Boot server does not have a corresponding endpoint for "/home" and throws a 404 error.
Fixing Routing Issues
To solve this problem, you can create a fallback route that directs all unknown paths to your index.html
. Here’s how you can implement it:
import org.springframework.boot.web.servlet.error.ErrorAttributes;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class SpaController {
@RequestMapping(value = "{path:[^\\.]*}")
public String redirect() {
// Forward to the index page
return "forward:/index.html";
}
}
This controller redirects all requests that do not have a file extension (like .html
, .js
, etc.) to index.html
, thereby allowing your client-side routing to take over.
3. Resource Not Found Errors
Sometimes, developers place their static resources incorrectly. If you have bundled your SPA using a build tool like webpack
or npm
and the resulting files are in a different location than expected, Spring Boot may not find them.
Fixing Resource Not Found Errors
Ensure that your build process correctly copies static resources to the src/main/resources/static
directory. Here's how you can configure a webpack
setup:
const path = require('path');
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
entry: './src/index.js', // Entry point of your application
output: {
filename: 'bundle.js', // Output file name
path: path.resolve(__dirname, 'dist') // Bundle output location
},
plugins: [
new HtmlWebpackPlugin({
template: './src/index.html', // Template HTML file
filename: 'index.html'
})
],
// Add other configurations as needed
};
After running the build command, ensure that your output files are copied to the correct path:
npm run build
Best Practices for Serving Static Resources
-
Use Versioning: Append version numbers or hashes to static resource files. It ensures users always get the latest version when you deploy updates.
-
Minimize Assets: Use tools to minimize JavaScript and CSS resources. Smaller file sizes lead to faster loading times.
-
Leverage CDNs: Consider hosting common libraries like jQuery, Bootstrap, or others via Content Delivery Networks (CDNs). This can reduce the load on your server.
-
Monitoring with Spring Actuator: Utilize Spring Actuator to monitor your application’s health and performance. It can provide insights into how many static resources are being served and their status.
-
Consider Security Headers: Implement security headers to protect your static resources. You can configure security headers using a filter throughout your application.
My Closing Thoughts on the Matter
Managing static resources in Spring Boot SPAs can introduce unique challenges, but the solutions outlined in this post will help streamline the process, improve user experience, and prevent common pitfalls such as caching issues and routing errors.
By appropriately configuring your Spring Boot application to handle static resources effectively, you ensure that your SPA runs like a well-oiled machine. Remember that keeping your static resources updated and correctly routed is key to delivering a seamless user experience.
For further reading, you can explore the official Spring Boot Documentation for more in-depth details on static content management.
Stay Updated
Have you encountered static resource issues in your applications? What solutions are you employing, or are there any additional challenges you've faced? Let us know in the comments below! Happy coding!