Overcoming Common Load Balancer Configuration Challenges
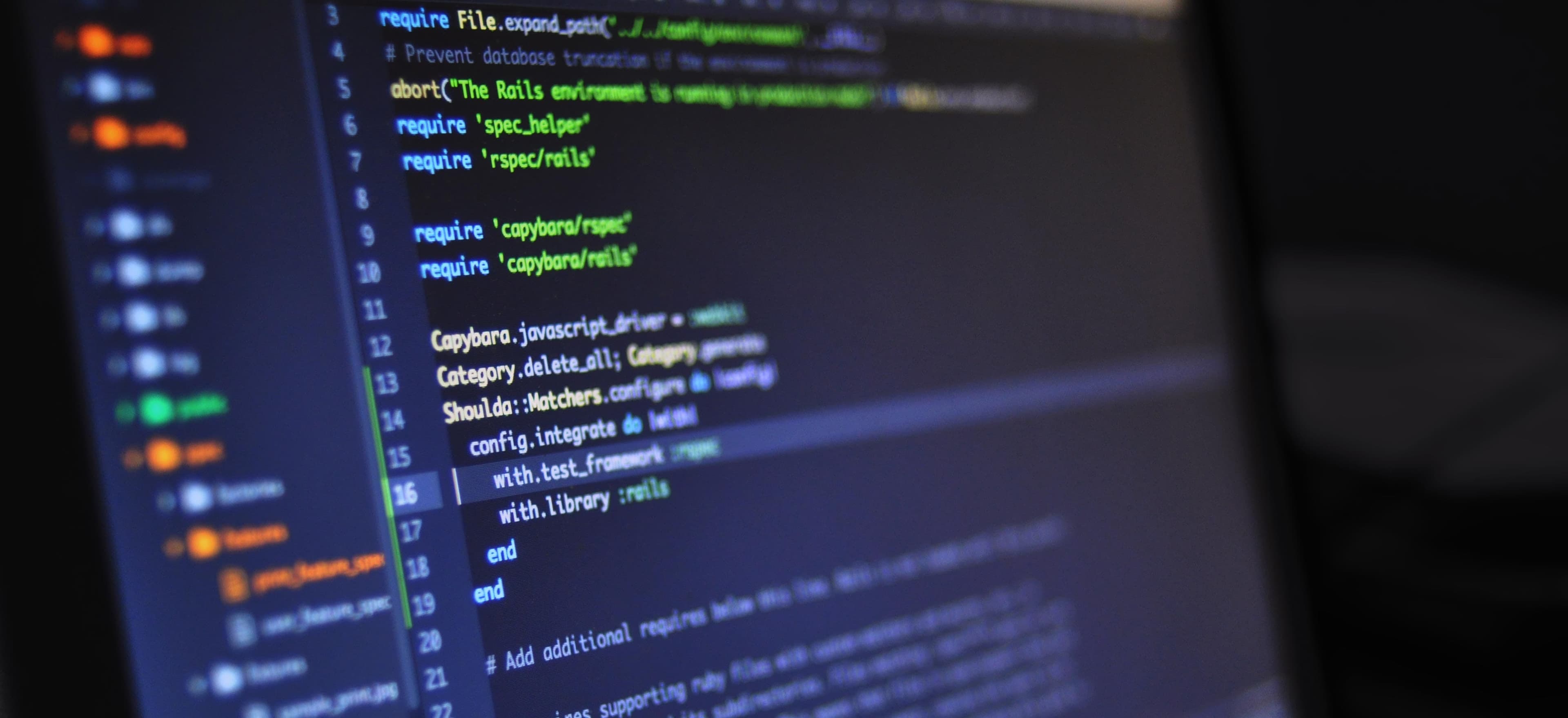
- Published on
Overcoming Common Load Balancer Configuration Challenges in Java Applications
Load balancing is crucial in modern applications, especially when considering the need for high availability, scalability, and fault tolerance. However, configuring load balancers can present several challenges. In this post, we will discuss these common issues, delve into solutions, and highlight Java-specific strategies to optimize your load balancer setup.
What is a Load Balancer?
A load balancer is a device or software that distributes network or application traffic across multiple servers. This process ensures that no single server becomes overwhelmed by too much traffic, which helps enhance responsiveness and availability.
Common Load Balancer Configuration Challenges
1. Understanding Load Balancing Algorithms
Choosing the right load balancing algorithm is essential. Various algorithms, such as Round Robin, Least Connections, and IP Hashing, handle traffic uniquely.
- Round Robin: Distributes traffic evenly across servers.
- Least Connections: Directs traffic to the server with the fewest active connections.
- IP Hashing: Routes requests based on the client’s IP, ensuring that a particular user always connects to the same server.
Solution
To determine the best approach for your application, consider your traffic patterns and server capabilities. For instance:
public class LeastConnectionsBalancing {
private Map<String, Integer> serverConnections = new HashMap<>();
public String routeRequest(List<String> servers) {
String targetServer = findLeastConnectedServer(servers);
incrementConnection(targetServer);
return targetServer;
}
private String findLeastConnectedServer(List<String> servers) {
return servers.stream()
.min(Comparator.comparingInt(serverConnections::get))
.orElseThrow(() -> new RuntimeException("No available server"));
}
private void incrementConnection(String server) {
serverConnections.put(server, serverConnections.getOrDefault(server, 0) + 1);
}
}
This code snippet demonstrates how to implement a least connections strategy programmatically, maintaining a count of active connections for each server. The challenge then becomes updating these counts accurately based on actual traffic.
2. Health Check Configuration
An effective load balancer needs to recognize the health status of each server. If a server goes down, the load balancer should stop directing traffic to it.
Solution
Configuring proper health checks is vital. Load balancers often support HTTP-based health checks, where they periodically request a specific URL from the server. To create a health check for a Java application, consider:
public class HealthCheck {
public boolean isServerHealthy(String serverUrl) {
try {
HttpURLConnection connection = (HttpURLConnection) new URL(serverUrl + "/health").openConnection();
connection.setRequestMethod("GET");
return connection.getResponseCode() == 200; // Returns true if server is healthy
} catch (IOException e) {
return false; // Server is unhealthy
}
}
}
In this example, a simple HTTP GET request checks the health endpoint of a server. The /health
endpoint is customarily implemented in Java applications to provide health status. For frameworks where this is not directly implemented, consider using Spring Boot Actuator which includes an out-of-the-box health check.
3. SSL Termination
Load balancers can handle SSL termination, which takes the burden off the back-end servers. This simplifies certificate management and reduces load on application servers but requires careful implementation.
Solution
To configure SSL termination effectively, generate a certificate and set it up on your load balancer. In a Java application using SSL, ensure configurations are correct both on the server and in your client connections.
For servers running Java applications, use the following in your configuration:
System.setProperty("javax.net.ssl.trustStore", "path/to/keystore.jks");
System.setProperty("javax.net.ssl.trustStorePassword", "password");
This informs your Java application to utilize the specified trust store when making SSL connections, ensuring security during communication while offloading SSL decryption tasks to the load balancer.
4. Session Persistence
Session persistence (or sticky sessions) ensures that user requests are consistently routed to the same server. This is crucial for applications with session information stored in-memory.
Solution
If your application uses sticky sessions, consider implementing session replication or external session stores like Redis. A simple way to store session data in Java might look like this:
public class SessionManager {
private Map<String, UserSession> sessions = new HashMap<>();
public void createSession(String sessionId, UserSession session) {
sessions.put(sessionId, session);
}
public UserSession getSession(String sessionId) {
return sessions.get(sessionId);
}
public void invalidateSession(String sessionId) {
sessions.remove(sessionId);
}
}
With this snippet, you can manage user sessions based on session IDs effectively. Implementing an external store allows for effective session management in load-balanced environments without depending solely on memory.
5. Load Balancer Failover
Setting up failover ensures that if one load balancer fails, traffic seamlessly redirects to another. Redundancy can further enhance availability.
Solution
To create a failover configuration, consider implementing a secondary load balancer and establishing a health monitoring setup to switch traffic in case of a primary failure. It's best to have these monitoring tools run checks at configurable intervals to avoid unnecessary downtime.
public class LoadBalancer {
private LoadBalancer primary;
private LoadBalancer secondary;
public void routeTraffic(String request) {
try {
primary.route(request);
} catch (Exception e) {
secondary.route(request); // Fallback to secondary in case of error
}
}
}
In this example, if the primary load balancer fails while handling a request, it falls back to a secondary load balancer, minimizing downtime.
A Final Look
Overcoming common load balancer configuration challenges is essential to ensure the smooth operation of Java applications in a production environment. By understanding load balancing algorithms, configuring health checks, implementing SSL termination, managing session persistence, and setting up a failover system, teams can significantly improve application performance, availability, and user experience.
As you configure load balancers, consider incorporating best practices, continuous monitoring, and iterative improvements. For further reading on various load balancing techniques, you may find resources like AWS Elastic Load Balancing helpful.
Final Thoughts
Successfully deploying load balancers requires a grasp of both foundational concepts and practical implementation strategies specific to your application environment. By addressing common challenges head-on, you can build robust, scalable, and resilient applications that stand up to varying loads while providing a seamless user experience.
As you embark on optimizing your load balancing configurations, remember—balance is key!
Checkout our other articles