Supercharge Your Java Logging: 7 Essential Logback Tips
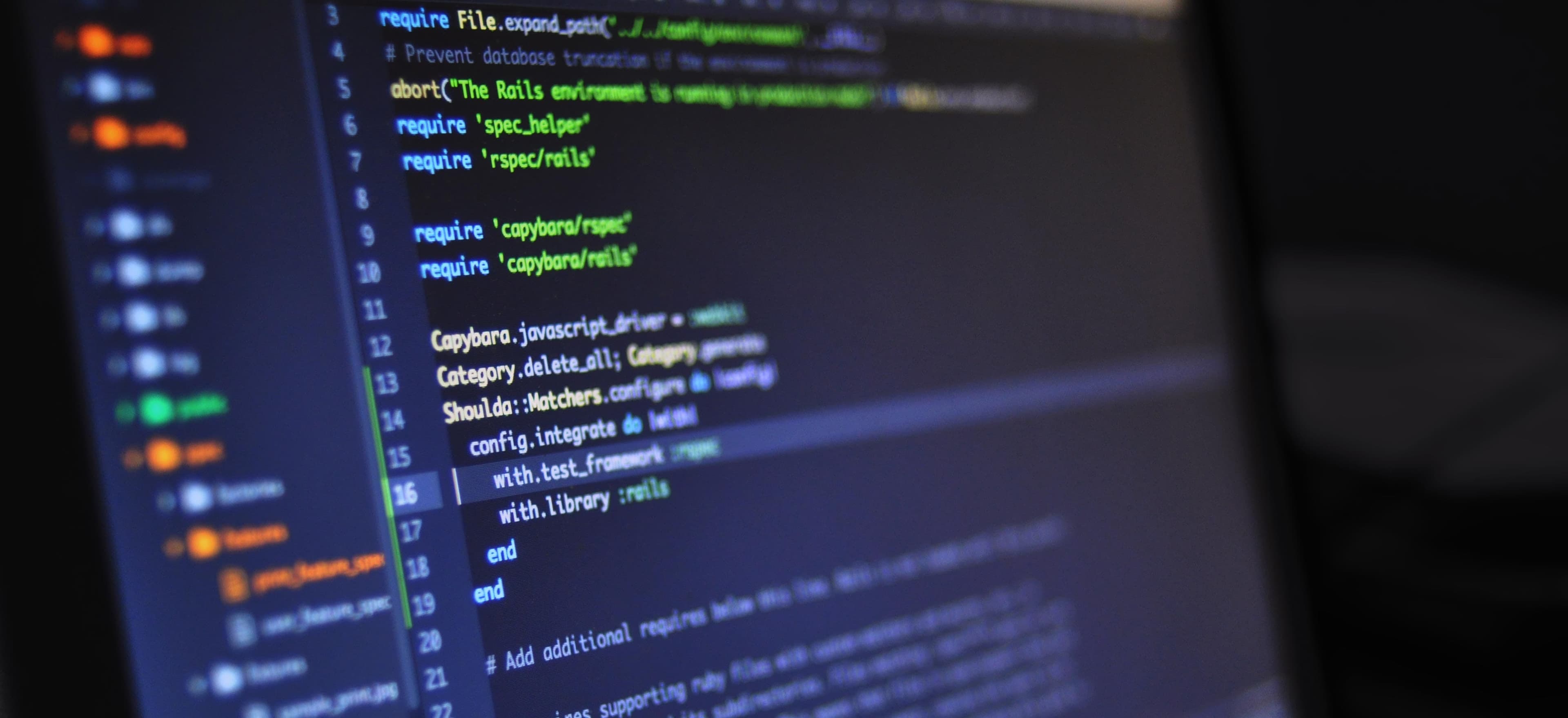
- Published on
Supercharge Your Java Logging: 7 Essential Logback Tips
Logging is a vital aspect of any software application, serving as a lifeline for debugging, monitoring, and auditing your Java applications. As Java developers, we need a reliable and flexible logging framework. Enter Logback, the successor to the popular log4j framework. In this blog post, we'll explore seven essential tips to help you maximize your Java logging with Logback.
What is Logback?
Logback is a robust logging framework written by the founder of the widely-used log4j project. It offers significant improvements in performance and is designed to be natively compatible with SLF4J (Simple Logging Facade for Java). Its features include configuration flexibility, efficient logging, and the ability to package your logs conveniently.
For additional context on logging in Java, you can refer to the Official Logback documentation.
1. Use the Correct Log Level
Choosing the right logging level helps you manage how much information is stored in your logs. Logback supports several levels: TRACE, DEBUG, INFO, WARN, ERROR, and FATAL.
Why It Matters
Using too many logs can clutter your log files, making it difficult to find issues. Conversely, too few logs may result in missed opportunities for debugging.
Code Snippet
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ExampleService {
private static final Logger logger = LoggerFactory.getLogger(ExampleService.class);
public void executeTask() {
logger.trace("Entering executeTask method");
// Your business logic here
logger.debug("Executing task...");
try {
// Some code that could fail
} catch (Exception e) {
logger.error("An error occurred: {}", e.getMessage());
}
logger.info("Task executed successfully");
}
}
In this snippet, TRACE
is used for method entry, while DEBUG
is for general execution details. Use ERROR
for exceptions, ensuring critical information is captured when something goes wrong.
2. Configurable Logging
Logback allows you to configure loggers through XML or Groovy files. This means you can change logging behavior without modifying your code base.
Why It Matters
By externalizing logging configuration, you can adapt your logging levels based on the environment (development, testing, production) without needing to recompile your application.
Example Configuration (logback.xml)
<configuration>
<appender name="FILE" class="ch.qos.logback.core.FileAppender">
<file>application.log</file>
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<root level="debug">
<appender-ref ref="FILE" />
</root>
</configuration>
Here we define a file appender that writes logs to application.log
. Monitor performance and adapt levels based on specifications.
3. Structured Logging
Structured logging enhances your log outputs by adding context through structured formats (like JSON). This can be invaluable for searching logs later.
Why It Matters
Searching unstructured logs is tedious. Structured logs can increase the efficiency of monitoring tools like ELK (Elasticsearch, Logstash, Kibana).
Code Snippet with Structured Logging
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class UserService {
private static final Logger logger = LoggerFactory.getLogger(UserService.class);
public void createUser(String username) {
logger.info("Creating user", Map.of("username", username));
// Logic for creating user
}
}
Utilizing structured data allows you to see relevant facts, making analysis simpler down the line.
4. Async Logging
Traditional logging methods can impact performance due to I/O operations. Logback’s async logging separates logging from the application's primary flow.
Why It Matters
By processing logs asynchronously, you free up your application, allowing faster execution while ensuring logging continues in the background.
Configuration for Async Logging
<configuration>
<appender name="ASYNC" class="ch.qos.logback.classic.AsyncAppender">
<appender-ref ref="FILE"/>
</appender>
<root level="debug">
<appender-ref ref="ASYNC" />
</root>
</configuration>
With this configuration, logs are collected and processed in a separate thread, minimizing performance overhead.
5. Filter Your Logs
Logback lets you filter logs based on customizable conditions. This is particularly useful for reducing noise in your logs.
Why It Matters
By filtering logs, you can concentrate on specific events or errors, making it easier to identify and troubleshoot issues.
Example Logback Filter Configuration
<configuration>
<appender name="FILE" class="ch.qos.logback.core.FileAppender">
<file>application.log</file>
<filter class="ch.qos.logback.classic.filter.ThresholdFilter">
<level>ERROR</level>
</filter>
<encoder>
<pattern>%d{HH:mm:ss} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<root level="debug">
<appender-ref ref="FILE" />
</root>
</configuration>
In this setup, only ERROR
level logs are recorded while maintaining DEBUG
as the root level.
6. Use MDC for Contextual Information
Mapped Diagnostic Context (MDC) allows you to enrich your log messages with specific context such as user IDs, session IDs, etc.
Why It Matters
Including context in your logs can significantly ease the debugging process by providing additional insight into issues as they arise.
Code Snippet using MDC
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.slf4j.MDC;
public class TransactionService {
private static final Logger logger = LoggerFactory.getLogger(TransactionService.class);
public void processTransaction(String userId) {
MDC.put("userId", userId);
logger.info("Processing transaction for user");
// Transaction logic here
MDC.clear(); // Ensure to clear MDC afterwards
}
}
By using MDC
, we can track logs relevant to particular users, improving traceability.
7. Monitor Logging Performance
Regular performance monitoring is crucial. Logback comes with built-in options to analyze logging.
Why It Matters
Understanding your logging performance helps you adjust configurations optimally, ensuring that logging does not bottleneck your application.
Code Snippet to Monitor Performance
import ch.qos.logback.core.status.StatusManager;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MonitorService {
private static final Logger logger = LoggerFactory.getLogger(MonitorService.class);
private StatusManager statusManager;
public void checkLoggingStatus() {
// Add logic to check performance
logger.info("Status: {}", statusManager.getStatus());
}
}
By integrating performance monitoring, you gain insights into how your logging is affecting your app's efficiency.
Key Takeaways
Effective logging is essential in developing and maintaining any Java application. Logback offers features and optimizations that can significantly enhance your logging practices. By leveraging the tips discussed in this post, you can supercharge your Java logging capabilities.
For more insights into enhancing your application's stability and reliability, check out best practices for Java application logging.
Supercharge your logging with Logback, and watch as your applications become more maintainable and easier to troubleshoot!
Checkout our other articles