Troubleshooting FlexyPool's Proxy and Decorator Conflicts
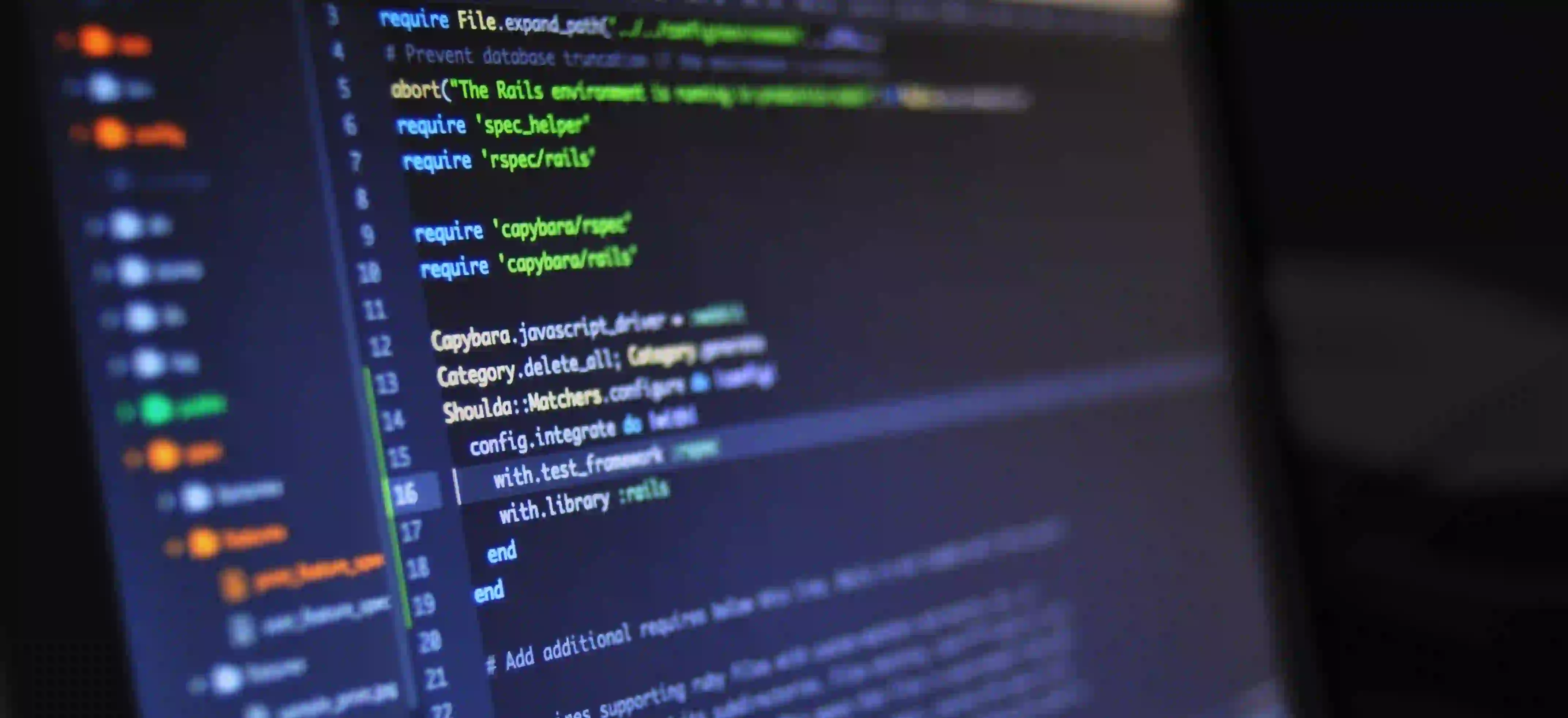
Troubleshooting FlexyPool's Proxy and Decorator Conflicts
In the world of application development, especially within the Java ecosystem, conflicts can arise from various elements such as proxies and decorators. This blog post will focus on troubleshooting these conflicts specifically related to FlexyPool, a popular solution for deployment and management.
Understanding FlexyPool
FlexyPool allows developers to create and manage their own proxies and decorators, thereby increasing the flexibility of application development. While these features are beneficial, they can cause conflicts if not handled properly.
What Are Proxies and Decorators?
-
Proxies: A proxy acts as an intermediary for another object, allowing control over its access. In Java, proxies can be utilized to define behavior before or after method execution.
-
Decorators: The decorator pattern enhances an object’s functionality without changing its structure. In Java, decorators can be implemented through inheritance or by utilizing interfaces.
Why Conflicts Occur
Conflicts typically arise due to:
- Method Overriding: Different layers of proxies or decorators may be redefining the behavior of the same method.
- State Management: If a decorator maintains state that conflicts with the state expected by a proxy.
- Invocation Order: The order in which decorators or proxies are applied can drastically change the behavior of the application.
Example Setup
Before delving into troubleshooting, here's an example setup that outlines a basic usage of FlexyPool's proxies and decorators.
public interface Task {
void perform();
}
public class BasicTask implements Task {
public void perform() {
System.out.println("Performing basic task.");
}
}
public class TaskDecorator implements Task {
private final Task task;
public TaskDecorator(Task task) {
this.task = task;
}
public void perform() {
System.out.println("Before performing the task");
task.perform();
System.out.println("After performing the task");
}
}
public class ProxyTask implements Task {
private final Task task;
public ProxyTask(Task task) {
this.task = task;
}
public void perform() {
System.out.println("Proxy: Checking permissions...");
task.perform();
}
}
Running the Example
To see this setup in operation, consider the following main method:
public class Main {
public static void main(String[] args) {
Task basicTask = new BasicTask();
Task decoratedTask = new TaskDecorator(basicTask);
Task proxyTask = new ProxyTask(decoratedTask);
proxyTask.perform();
}
}
Output:
Proxy: Checking permissions...
Before performing the task
Performing basic task.
After performing the task
Here, the output gives insight into how proxies and decorators interact with each other.
Identifying and Resolving Conflicts
1. Method Overriding Conflicts
Problem:
When methods are overridden in both proxies and decorators, conflicts can become apparent. For example, if a decorator approaches a method differently than a proxy, such an interaction may yield unintended results.
Solution:
To resolve method overriding issues, ensure that the appropriate overrides are maintained and interactions are clearly defined. Use interfaces to create a contract that must be adhered to by all proxies and decorators.
public class ConcreteTaskDecorator extends TaskDecorator {
public ConcreteTaskDecorator(Task task) {
super(task);
}
// Additional behavior
public void perform() {
super.perform();
System.out.println("Additional behavior in ConcreteTaskDecorator.");
}
}
2. Managing State
Problem:
State management issues arise when decorators maintain states that clash with the expectations of the proxy. This can lead to confusing and difficult-to-trace errors.
Solution:
Avoid maintaining shared mutable state between proxies and decorators. If state is necessary, consider encapsulating it within each layer instead of sharing it.
public class StatefulDecorator extends TaskDecorator {
private int state;
public StatefulDecorator(Task task) {
super(task);
this.state = 0; // initial state
}
public void perform() {
state++; // change the state with each call
super.perform();
System.out.println("State: " + state);
}
}
3. Invocation Order
Problem:
When proxies and decorators are applied in the wrong order, it can lead to logic errors where the expected flow of control is broken.
Solution:
To prevent invocation order conflicts, document the intended order of application and ensure that this order is respected during implementation.
Task task = new BasicTask();
// Correct order: first decorate, then proxy
Task taskWithDecorator = new TaskDecorator(task);
Task taskWithProxy = new ProxyTask(taskWithDecorator);
taskWithProxy.perform();
Best Practices for Preventing Conflicts
- Keep It Simple: Strive to limit the layers of proxies and decorators where possible.
- Document Behavior: Clearly comment on and document expected behavior for each layer. This will help others (or yourself in the future) understand the design.
- Use Composition Over Inheritance: Whenever possible, use composition to manage proxies and decorators, as it allows for greater flexibility and fewer conflicts.
- Testing: Implement thorough unit tests to ensure that each proxy and decorator behaves as expected in isolation and in conjunction with other components.
The Bottom Line
Troubleshooting proxy and decorator conflicts in FlexyPool can be a daunting task. However, with a clear understanding of the interplay between proxies and decorators, combined with best practices, these issues can be effectively managed.
For further reference on design patterns and Java programming, consider exploring Java Design Patterns and Oracle’s Java Documentation. Understanding the fundamentals will empower you to write cleaner, more efficient code while mitigating potential conflicts.
By adhering to the provided guidelines and code snippets, you can ensure smoother development processes and create more stable applications that leverage FlexyPool's capabilities. Happy coding!