Understanding Volatile Variables in Java: Common Pitfalls
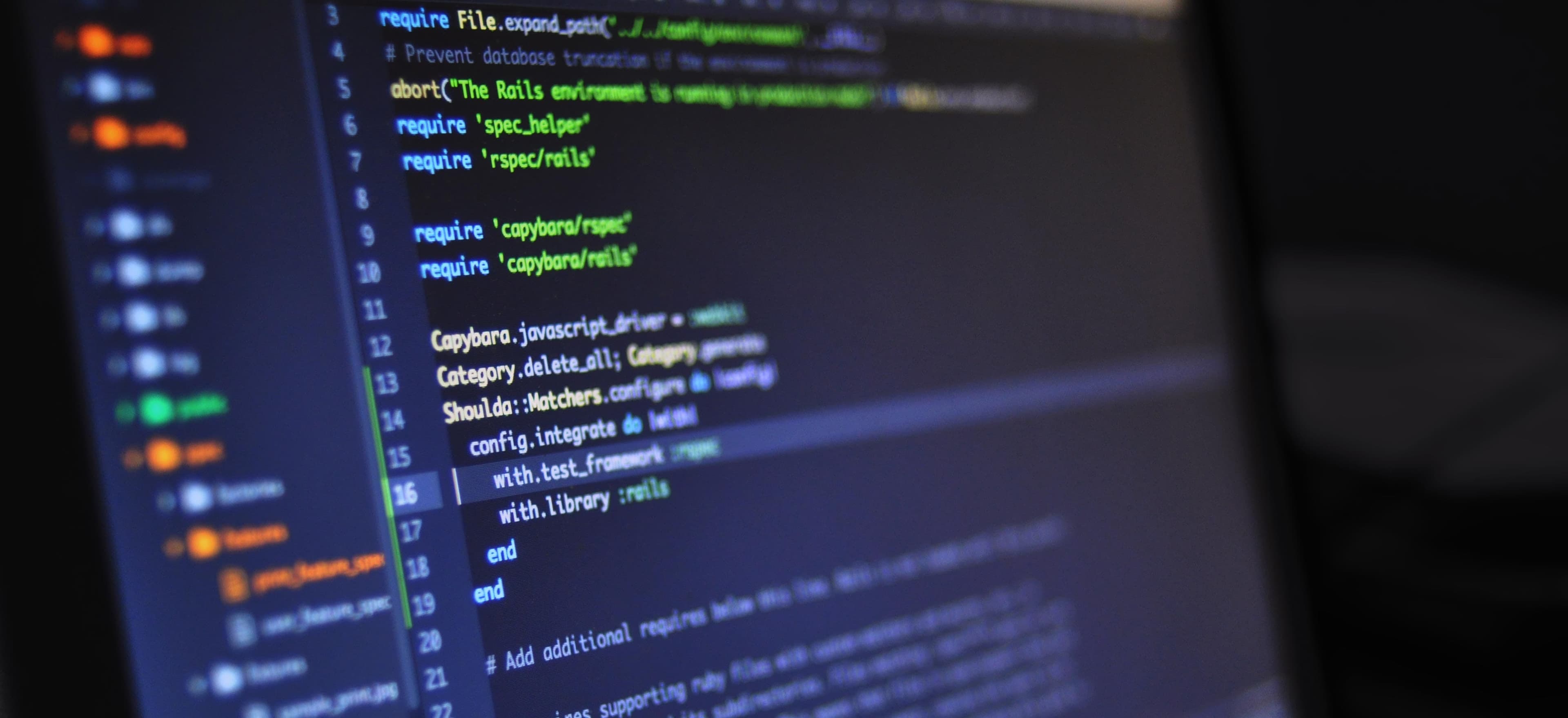
- Published on
Understanding Volatile Variables in Java: Common Pitfalls
Java is a robust and versatile programming language that facilitates multithreading and concurrency. At the heart of concurrent programming in Java lies the volatile
keyword. It plays an essential role in ensuring visibility and ordering when working with shared variables across multiple threads. However, despite its utility, there are common pitfalls that developers often encounter when using volatile variables. This blog post will delve into the ins and outs of volatile
variables, emphasizing the potential hazards and best practices associated with them.
What is a Volatile Variable?
In Java, when a variable is declared with the volatile
keyword, it signals to the Java compiler and the JVM that the variable may be accessed by multiple threads. This has two critical implications:
-
Visibility: Changes made to a volatile variable by one thread are immediately visible to all other threads. Without
volatile
, there's no guarantee when one thread's changes will be visible to another. -
Ordering: The JVM is not allowed to reorder accesses to a volatile variable. This means the operations before and after reading or writing a volatile variable cannot be swapped across threads.
Here's a simple example:
class VolatileExample {
private volatile boolean flag = false;
public void writer() {
flag = true; // Thread A writes true to flag
}
public void reader() {
while (!flag) {
// Thread B reads flag
}
System.out.println("Flag changed to true");
}
}
In this case, if the flag
variable were not declared as volatile
, Thread B might never see the update from Thread A because of caching and compiler reordering optimizations.
Common Pitfalls with Volatile Variables
While volatile variables can provide a lightweight synchronization mechanism, they are often misunderstood. Here are some common pitfalls and how to avoid them.
1. Volatile Isn't Atomic
One of the most significant misconceptions about volatile variables is that they ensure atomicity. The keyword only guarantees visibility and ordering. Therefore, compound actions—like checking a condition and then updating a variable—are not atomic, leading to unpredictable behavior.
class NonAtomicExample {
private volatile int counter = 0;
public void increment() {
counter++; // Not atomic!
}
}
In this case, if two threads call increment()
simultaneously, both might read the same initial value of counter
, leading to inconsistencies. To fix this, you should use synchronized blocks or java.util.concurrent.atomic
classes:
import java.util.concurrent.atomic.AtomicInteger;
class AtomicExample {
private final AtomicInteger counter = new AtomicInteger(0);
public void increment() {
counter.incrementAndGet(); // Atomic operation!
}
}
2. Use Cases for Volatile Variables
Volatile variables are best suited for flags, particularly when state changes can be independently controlled, such as in a graceful shutdown scenario. Here’s an example:
class ShutdownExample {
private volatile boolean shuttingDown = false;
public void shutdown() {
shuttingDown = true;
}
public void run() {
while (!shuttingDown) {
// Some work
}
System.out.println("Shutting down.");
}
}
In this scenario, the volatile keyword ensures that the shuttingDown
flag is visible across threads, allowing for the loop to terminate safely.
3. Volatile and Inheritance
Another often overlooked aspect of volatile variables pertains to inheritance. If a volatile variable is declared in a superclass, a subclass does not inherit the volatile property. Consider the following scenario:
class Parent {
private volatile boolean flag = false;
}
// This subclass does NOT inherit the volatile property of flag
class Child extends Parent {
public void setFlag(boolean value) {
flag = value; // This is NOT volatile
}
}
In the code above, even if flag
is declared volatile in Parent
, it loses that guarantee when accessed from Child
. Consequently, if any thread reads flag
from an object of Child
, it might not observe the writes made to flag
by other threads.
4. Volatile and Synchronization
Despite the visibility guarantees of volatile, many developers mistakenly believe it can replace synchronization entirely. In reality, volatile variables cannot provide the atomicity or mutual exclusion features that traditional synchronization offers.
If a method modifies a volatile variable and needs additional operations that also require thread safety, synchronization is still necessary. For instance:
class SyncExample {
private volatile int value = 0;
public synchronized void increment() {
value++; // This needs to be synchronized
}
public synchronized int getValue() {
return value;
}
}
In the example above, both increment()
and getValue()
are synchronized to ensure atomicity.
5. Read-Modify-Write Operations
Many developers make the mistake of using volatile variables for read-modify-write operations, thinking that the visibility guarantees of volatile suffice. Here's why that's problematic:
class ReadModifyWriteExample {
private volatile int sharedVar = 0;
public void updateSharedVar() {
sharedVar++; // Not atomic and not safe!
}
}
In this scenario, due to the non-atomic nature of the increment operation, race conditions can occur. It’s better to use atomic classes, as mentioned earlier, or traditional synchronization.
A Final Look
Understanding the use of volatile variables in Java is crucial for writing robust concurrent applications. By recognizing the common pitfalls, developers can leverage the power of the volatile
keyword while avoiding potential bugs and unpredictable behavior.
When should you use volatile then? Use it for flags and states where visibility across threads is necessary, but never as a replacement for synchronization in compound actions. Always consider thread safety and atomicity when choosing your concurrency mechanisms.
For more insights on Java concurrency, check out resources like Baeldung and Oracle's Java Documentation for a deeper exploration of threading principles.
By being cautious and informed, you can harness the power of concurrency in your Java applications effectively, ensuring they are not only performant but also safe. Happy coding!
Checkout our other articles