Struggling with Akka Testing: Unit vs. Integration Demystified
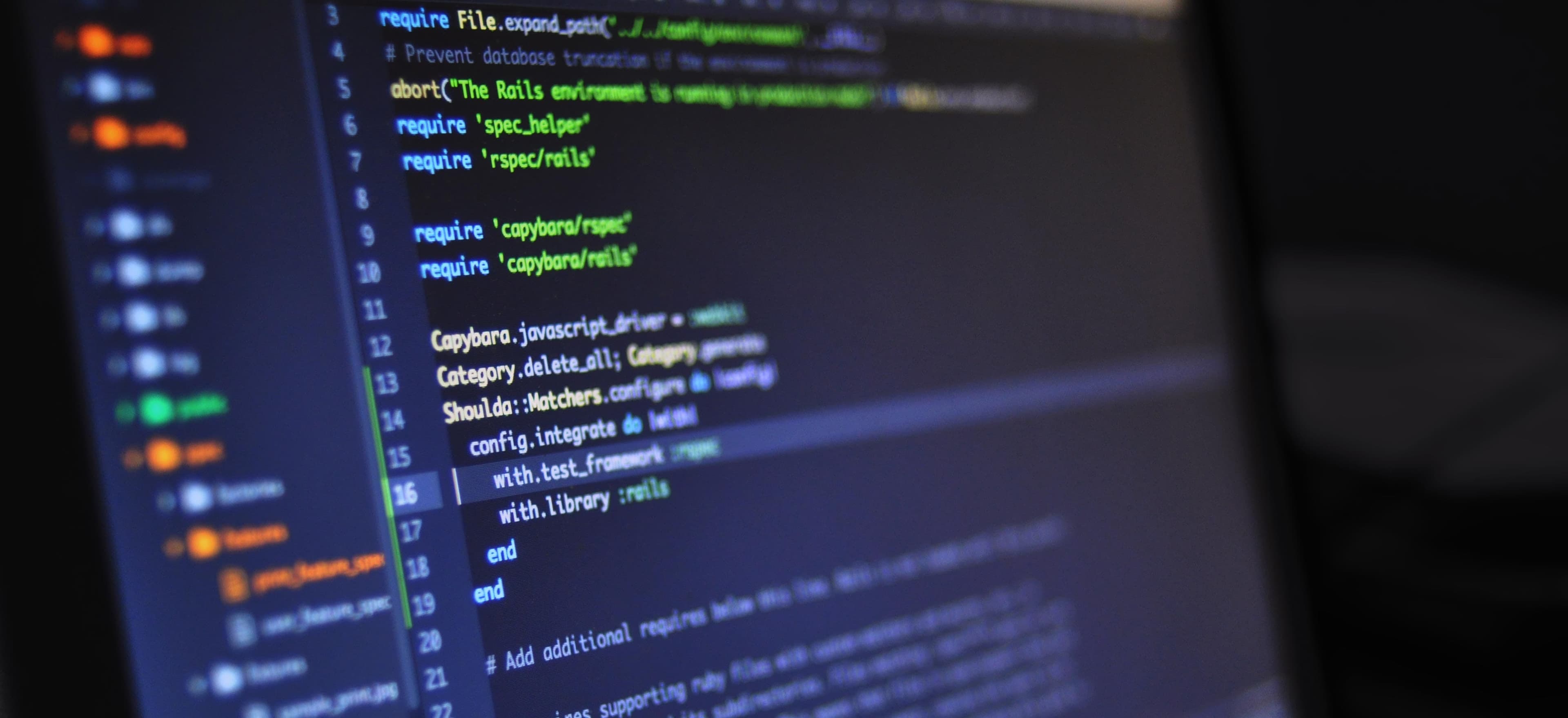
- Published on
Struggling with Akka Testing: Unit vs. Integration Demystified
When working with Akka, a powerful toolkit for building concurrent, distributed, and resilient message-driven applications in Java and Scala, testing can feel daunting. You may often find yourself unsure about how to approach testing: Is it enough to write unit tests, or should you also focus on integration tests? In this blog post, we will clarify the differences between unit and integration tests within the context of Akka, guiding you on how to effectively implement both to ensure a robust application.
The Importance of Testing in Akka
Before we dive into the specifics, it's vital to understand why testing is particularly crucial in an Akka environment:
- Concurrency: Akka's actor model introduces concurrency, which can lead to race conditions and subtle bugs that are challenging to detect without rigorous testing.
- Asynchronous Behavior: Actors operate asynchronously, making it difficult to ascertain the order of message processing.
- Resilience: As Akka promotes the "let it crash" philosophy, it's important to test how your application handles failures.
Given these factors, understanding how to effectively implement unit and integration tests is not just beneficial; it's essential.
Understanding Unit Tests
What Are Unit Tests?
Unit tests focus on testing individual components in isolation. In Akka, this typically means testing one actor or one piece of functionality without involving external systems.
Characteristics of Unit Tests
- Isolated: Each unit test should only focus on a specific functionality.
- Fast: Unit tests should run quickly, making them ideal for immediate feedback during development.
- Independent: They should not rely on other tests to pass.
Example: Unit Testing an Actor
Let's take a look at how to write a unit test for an Akka actor. Here, we will use ScalaTest and Akka TestKit for our examples.
First, let's define a simple actor that performs arithmetic calculations:
import akka.actor.Actor
import akka.actor.Props
class CalculatorActor extends Actor {
def receive: Receive = {
case Add(x: Int, y: Int) => sender() ! (x + y)
case Subtract(x: Int, y: Int) => sender() ! (x - y)
}
}
case class Add(x: Int, y: Int)
case class Subtract(x: Int, y: Int)
Now, let's write a unit test to verify its behavior:
import akka.actor.ActorSystem
import akka.testkit.{TestActor, TestKit, TestProbe}
import org.scalatest.{BeforeAndAfterAll, Matchers, WordSpecLike}
class CalculatorActorSpec extends TestKit(ActorSystem("CalculationSpec"))
with WordSpecLike with Matchers with BeforeAndAfterAll {
override def afterAll(): Unit = {
system.terminate()
}
"A Calculator Actor" should {
"correctly add two numbers" in {
val calculator = system.actorOf(Props[CalculatorActor])
val probe = TestProbe()
probe.send(calculator, Add(3, 4))
probe.expectMsg(7)
}
"correctly subtract two numbers" in {
val calculator = system.actorOf(Props[CalculatorActor])
val probe = TestProbe()
probe.send(calculator, Subtract(10, 4))
probe.expectMsg(6)
}
}
}
Why This Matters
In the code snippet above:
- We create an
ActorSystem
to run our actor within a test environment. - We use
TestProbe
, which acts as a fake actor to send messages and verify the output. - The
expectMsg
method is essential for asserting that the actor responds correctly.
In this example, you ensure that the CalculatorActor
behaves as expected in isolation.
Understanding Integration Tests
What Are Integration Tests?
Integration tests, on the other hand, focus on the interactions between components. In the context of Akka, this means testing an entire sequence of actors or the integration of the actor system with external systems such as databases, HTTP services, or message brokers.
Characteristics of Integration Tests
- Comprehensive: They test more than one unit, often involving all related components.
- Slower: Since they may interact with external systems, integration tests take longer to execute than unit tests.
- Environment-specific: They often depend on the environment, making them less predictable than unit tests.
Example: Integration Testing with Akka
Let's consider an example where we want to test an actor that interacts with an external HTTP service. For this, we may use libraries such as akka-http
for the HTTP requests and responses.
Suppose we have an actor that fetches a user by ID from an external API:
import akka.actor.Actor
import akka.pattern.pipe
import akka.http.scaladsl.Http
import akka.http.scaladsl.unmarshalling.Unmarshal
import akka.http.scaladsl.model._
import scala.concurrent.Future
class UserFetcherActor(apiUrl: String) extends Actor {
implicit val ec = context.dispatcher
def receive: Receive = {
case FetchUser(id: String) =>
val responseFuture: Future[String] = Http(context.system).singleRequest(HttpRequest(uri = s"$apiUrl/users/$id"))
.flatMap { response =>
Unmarshal(response.entity).to[String]
}
responseFuture.pipeTo(sender())
}
}
case class FetchUser(id: String)
Now, for testing this actor integration with the external API, we might mock the HTTP service rather than hitting the actual URL. This allows us to isolate the actor's behavior from the external service.
import akka.actor.ActorSystem
import akka.http.scaladsl.testkit.ScalatestRouteTest
import akka.testkit.{TestKit, TestProbe}
import org.scalatest.{BeforeAndAfterAll, Matchers, WordSpecLike}
class UserFetcherActorSpec extends TestKit(ActorSystem("UserFetcherSpec"))
with WordSpecLike with Matchers with BeforeAndAfterAll with ScalatestRouteTest {
override def afterAll(): Unit = {
system.terminate()
}
"A UserFetcher Actor" should {
"return a user for a valid ID" in {
val userFetcher = system.actorOf(Props(new UserFetcherActor("http://fakeapi.com")))
val probe = TestProbe()
// Simulate receiving a FetchUser message from another actor
probe.send(userFetcher, FetchUser("1"))
probe.expectMsg("User data for ID 1") // Assuming this is what the mock API returns
}
}
}
Why This Matters
In the integration test example:
- We're using a mock HTTP endpoint to simulate the behavior of an external service.
- By testing how the
UserFetcherActor
interacts with this endpoint, we ensure that the logic in the actor is functioning as intended without coupling the test to an actual third-party service.
When to Use Unit vs. Integration Tests
In a project that utilizes Akka:
- Use unit tests for fast feedback when developing individual components. This allows for more granular testing, helping catch potential bugs in isolation.
- Use integration tests to ensure multiple components work together seamlessly. This helps uncover issues that can arise from the interactions between your actors or when integrating with external services.
In Conclusion, Here is What Matters
Understanding the nuances of unit testing and integration testing in Akka is key to building reliable applications. By focusing on isolated behavior with unit tests and comprehensive integration tests, you safeguard your application against both simple and compound issues.
For further reading on Akka, check the official Akka documentation and learn more about testing strategies through ScalaTest.
Building a resilient, robust application requires the appropriate testing strategies in place. By utilizing both unit and integration tests effectively, you can ensure that your Akka-based application stands strong in the face of complexity and uncertainty.
Checkout our other articles