Optimizing Java Performance on NUMA Architectures
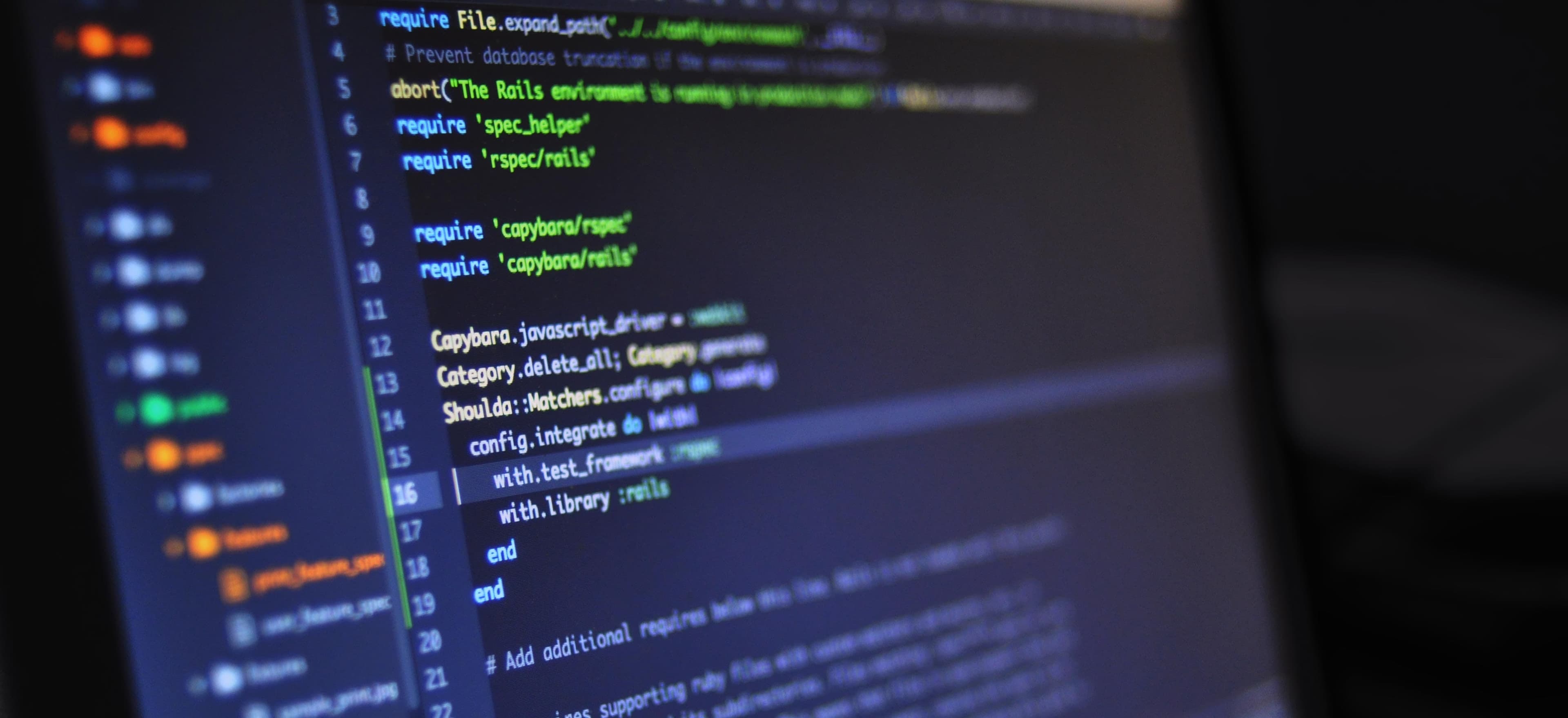
- Published on
Optimizing Java Performance on NUMA Architectures
Java is a versatile programming language that thrives in various computing environments, but when it comes to Non-Uniform Memory Access (NUMA) architectures, specific optimizations can significantly enhance performance. In this blog post, we will explore how NUMA impacts Java applications and discuss practical techniques to optimize performance on such systems.
Understanding NUMA Architectures
In a traditional single memory space, all CPU cores share a common memory. However, in NUMA architectures, memory is divided into multiple nodes, with each node containing its own memory and one or more CPUs. Accessing memory local to the CPU is faster than accessing memory from a remote node. Understanding this is key to optimizing Java applications on NUMA systems.
The Importance of Memory Locality
Memory locality is crucial because accessing data from a remote node incurs higher latency. Applications should be designed to maximize the use of local memory. In Java, this translates to object allocation, garbage collection, and thread affinity considerations.
Strategies for Optimizing Java Performance
Below are several strategies to optimize Java applications on NUMA architectures:
1. Choosing the Right Garbage Collector
Garbage collection (GC) can significantly affect performance. Java provides different garbage collectors, each with its trade-offs. Using a garbage collector optimized for low-latency applications, such as the G1 or ZGC, can improve performance on NUMA systems.
Example of Setting the Garbage Collector
java -XX:+UseG1GC -jar YourApplication.jar
Why: The G1 Garbage Collector is designed for applications requiring short pause times and may perform better in a NUMA environment by trying to prioritize local memory.
2. Allocating Memory Strategically
Java developers can take advantage of NUMA-aware memory allocation techniques by allocating memory closer to the thread or CPU using libraries like numacp.
Code Snippet
import com.numa.NumaAlloc;
public class NumaMemoryExample {
public static void main(String[] args) {
// Allocate memory on NUMA node 0
NumaAlloc alloc = new NumaAlloc(0);
int[] memory = alloc.allocateIntArray(1000);
// Use the memory
for (int i = 0; i < memory.length; i++) {
memory[i] = i;
}
alloc.free(memory);
}
}
Why: By explicitly allocating memory on a specific NUMA node, you minimize remote memory access. This improves locality and reduces latency significantly.
3. Thread Affinity
Binding threads to specific CPUs can greatly enhance performance by ensuring that threads consistently access local memory. Java does not natively support thread affinity, but you can use native libraries for this purpose.
Example with JNA (Java Native Access)
import com.sun.jna.Library;
import com.sun.jna.Native;
public class ThreadAffinityExample {
public interface CLib extends Library {
CLib INSTANCE = Native.load("c", CLib.class);
void sched_setaffinity(int pid, int cpusetsize, byte[] mask);
}
public static void setThreadAffinity(int coreId) {
byte[] mask = new byte[8]; // Assuming 64 core size
mask[coreId / 8] |= (1 << (coreId % 8));
CLib.INSTANCE.sched_setaffinity(0, mask.length, mask);
}
public static void main(String[] args) {
setThreadAffinity(0); // Bind current thread to core 0
// Run your application logic here
}
}
Why: By setting thread affinity, you ensure that threads consistently run on specific cores, which helps maintain cache locality and decreases memory access time.
4. Profiling and Monitoring
Profiling tools such as VisualVM or JConsole can help you understand memory allocation patterns and CPU usage in NUMA systems. You might find areas where the application can be optimized further.
Using Java Mission Control is also an excellent way to monitor Java applications that run on NUMA architectures.
5. Use of Thread Pools
When dealing with threads in a Java application, utilizing thread pools is a good practice. Thread pools can help manage resources effectively across different NUMA nodes. The ForkJoinPool
can help optimize task distribution based on the availability of CPU resources.
Pooling Example
import java.util.concurrent.ForkJoinPool;
public class ThreadPoolExample {
public static void main(String[] args) {
ForkJoinPool forkJoinPool = new ForkJoinPool(Runtime.getRuntime().availableProcessors());
forkJoinPool.submit(() -> {
// Execute parallel tasks
});
forkJoinPool.shutdown();
}
}
Why: By using thread pools, you can control how threads are created and managed, optimizing the workload distribution across cores effectively.
The Closing Argument
Optimizing Java applications on NUMA architectures can significantly enhance performance, especially for memory-intensive applications. By understanding memory locality, choosing the right garbage collector, strategically allocating memory, enforcing thread affinity, utilizing profiling tools, and implementing thread pools, developers can make substantial performance improvements.
Incorporating these techniques will help you leverage the benefits of modern multi-core, NUMA-enabled systems, leading to faster and more responsive applications. As the computing landscape continues to evolve, keeping abreast of these optimizations will be vital for any serious Java developer.
Further Reading
- Java Performance Tuning
- Understanding NUMA Architectures
- Best Practices for Java Garbage Collection
By diligently applying these strategies, you will be well-equipped to tackle the challenges posed by NUMA architectures and optimize your Java applications accordingly.
Checkout our other articles