Common EJB Lookup Issues in Vaadin Applications
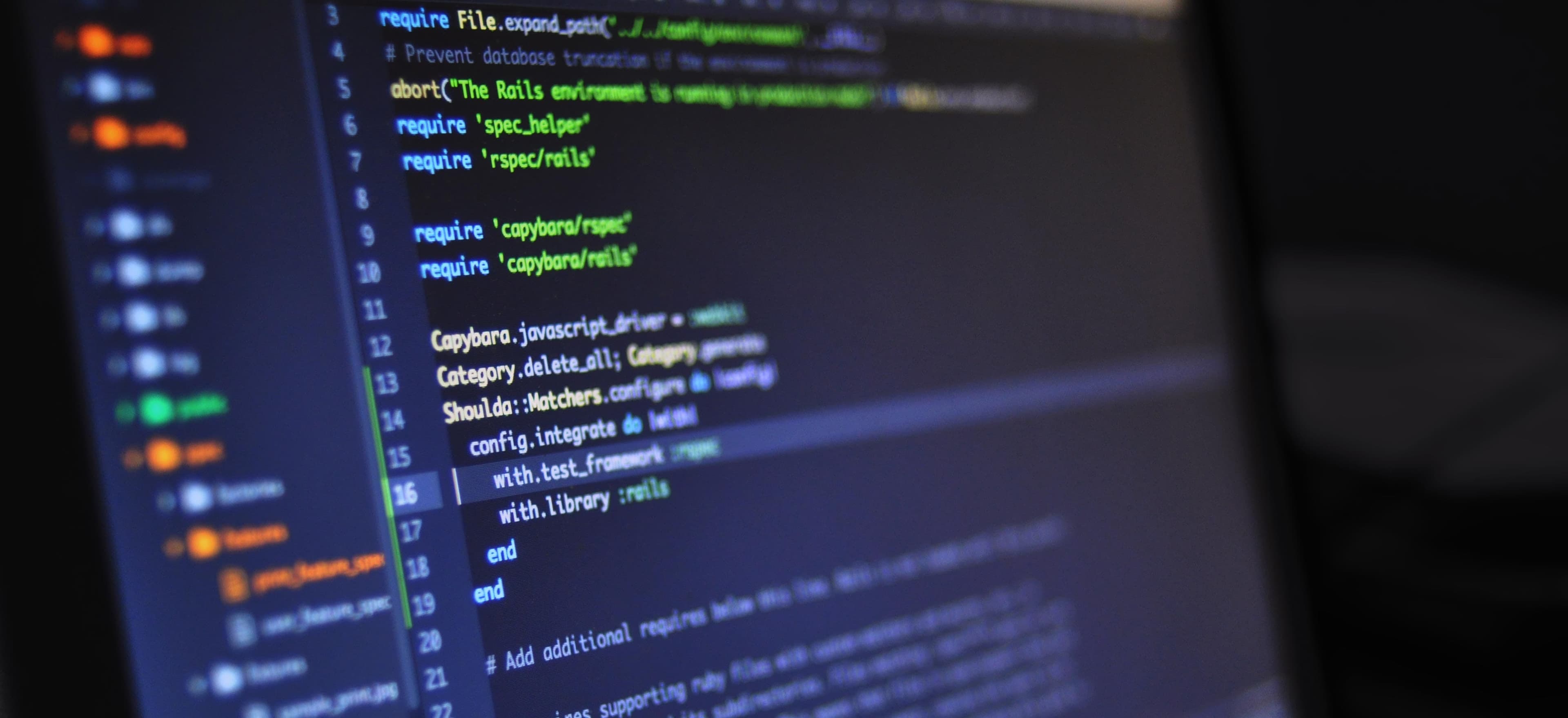
- Published on
Common EJB Lookup Issues in Vaadin Applications
Enterprise JavaBeans (EJB) and Vaadin are powerful technologies that, when used together, can create dynamic and robust web applications. However, developers often encounter challenges, particularly with EJB lookup issues, which can hinder application performance and functionality. In this blog post, we will explore common EJB lookup issues in Vaadin applications, how to troubleshoot them, and best practices to prevent these problems.
Understanding EJB and Vaadin
EJB is a server-side software component that encapsulates business logic of an application. With features like concurrency, transaction management, and security, EJB is great for large-scale applications. Vaadin, on the other hand, is a popular Java framework for building modern web user interfaces. It allows developers to create rich web applications using Java, eliminating the need for complex JavaScript and other front-end technologies.
The integration of EJB with Vaadin applications can streamline the process of accessing server-side business logic from the UI layer. However, effective communication between the two components requires a sound understanding of how EJB lookup works.
Common EJB Lookup Issues
1. JNDI Name Mismatch
The Java Naming and Directory Interface (JNDI) name is essential for looking up EJB instances. A common mistake made by developers is using an incorrect JNDI name. In a Vaadin application, if the lookup name of the EJB does not match the registered name in the application server, it will result in a NamingException
.
InitialContext ctx = new InitialContext();
MyBean myBean = (MyBean) ctx.lookup("java:global/myapp/MyBean");
Why It Matters: Always ensure that the JNDI name matches the configuration in your application server (e.g., WildFly, Payara, GlassFish). You can verify your JNDI name by checking your server logs or using tools such as java:comp/env
.
2. Application Server Configuration
Sometimes the configuration of the application server can lead to EJB lookup issues. If your EJB is not correctly defined in the web.xml
or ejb-jar.xml
, or if the deployment descriptor is missing, your Vaadin application may not be able to find the EJB.
<ejb>
<ejb-name>MyBean</ejb-name>
<jndi-name>java:global/myapp/MyBean</jndi-name>
</ejb>
Advice: Regularly review the configuration files and ensure that they are accurate and updated according to the deployment descriptor specifications.
3. Classloader Issues
Classloader issues can arise when your EJB and Vaadin application are not deployed in the same classloader hierarchy, leading to ClassNotFoundException
. This is common when using different deployment methods or containers.
Best Practice: Ensure that your EJB and web components are packaged properly in the same EAR file when possible, or share libraries that both the EJBs and Vaadin application can access.
4. Transaction Management
EJBs are usually transactional by default, which can lead to issues if your Vaadin application does not handle transactions properly. If you start a transaction within your EJB but fail to commit or rollback, it could cause various exceptions.
@Stateless
public class MyBean {
@PersistenceContext
private EntityManager em;
public void doWork() {
// Perform database operations
// Ensure to handle transactions properly
...
}
}
Recommendation: Utilize Java Transaction API (JTA) features to appropriately manage transactions and make sure resources are released in case of failure.
5. EJB Scope Conflicts
Confusion between different EJB scopes—stateless, stateful, and singleton—can often lead to application errors. If your Vaadin application expects a stateful bean but uses a stateless bean mistakenly, it may result in unexpected behavior.
Example of Stateful EJB:
@Stateful
public class ShoppingCartBean {
private List<Item> items = new ArrayList<>();
public void addItem(Item item) {
items.add(item);
}
}
Conclusion: Always choose the appropriate EJB scope according to your application's requirements.
Troubleshooting EJB Lookup Issues
When you encounter an EJB lookup issue, follow these troubleshooting steps:
-
Check Server Logs: Start with checking your application server's logs for errors or warnings related to EJB deployment and lookup.
-
Verify JNDI Names: Ensure the JNDI names used in your lookup are correct and match those declared in your EJB setup.
-
Review EJB Configuration: Look through XML configuration files (like
web.xml
orejb-jar.xml
) for misconfigurations or omissions. -
Test EJB Independently: Simplify your deployment by testing your EJB independently using a unit testing framework like JUnit or Arquillian to ensure it behaves as expected.
Best Practices for Smooth EJB Lookup
To prevent EJB lookup issues in your Vaadin applications, consider implementing these best practices:
- Use Dependency Injection: Utilize CDI (Contexts and Dependency Injection) to inject EJB components instead of performing JNDI lookups directly. This allows for cleaner code and reduces errors.
@Inject
private MyBean myBean;
-
Centralize Configuration: Keep a consistent naming convention and centralized configuration for all your JNDI names. This streamlines the maintenance of your application.
-
Regularly Update Documentation: Maintain documentation of EJBs and their JNDI lookup names for easy reference by your development team.
-
Utilize Testing Tools: Employ tools like Arquillian for testing EJB components in configuration similar to production, which can help surface potential issues early in the development cycle.
-
Monitor Performance: Use monitoring tools to keep an eye on the performance of your application and its components, allowing you to spot EJB-related issues before they become critical.
A Final Look
In conclusion, integrating EJBs into Vaadin applications can greatly enhance the functionality and scalability of your web applications. However, understanding and troubleshooting common EJB lookup issues is crucial for successful implementation. By following the best practices laid out in this blog post, you can streamline your development process and avoid the pitfalls commonly associated with EJB lookups.
For further reading, you might find these resources helpful:
- Java EE Tutorial: Enterprise Java Beans
- Vaadin Documentation
- Understanding JNDI
By being proactive and informed, you'll set yourself up for a successful experience with EJB and Vaadin integrations. Happy coding!