Maximizing Performance: Choosing the Right JVM Version
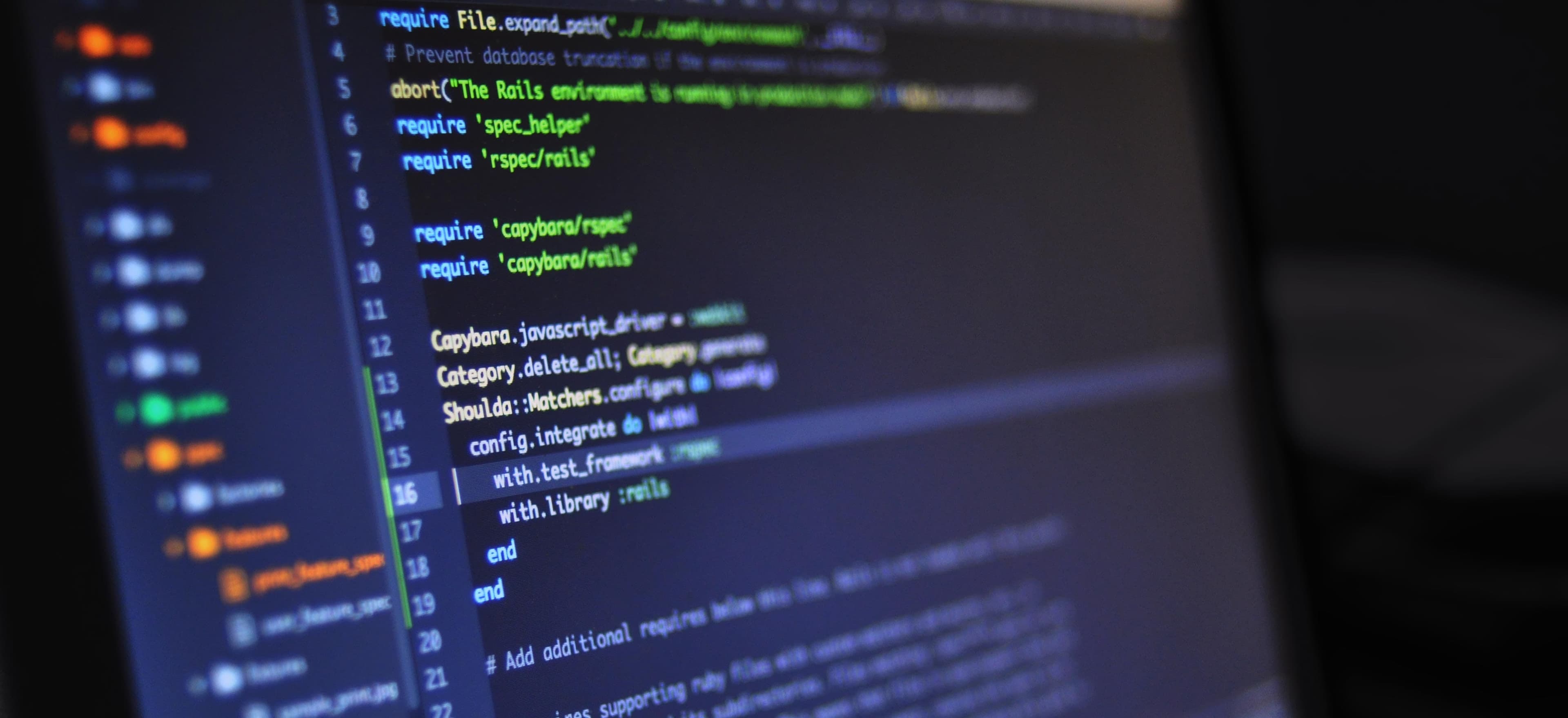
- Published on
Maximizing Performance: Choosing the Right JVM Version
Java, one of the most popular programming languages globally, runs on the Java Virtual Machine (JVM), which plays a crucial role in determining the performance of Java applications. Regularly updating the JVM can lead to significant performance improvements, new features, and security enhancements. However, with multiple versions available, choosing the right one can be a daunting task. This blog post aims to guide you through this process, emphasizing the importance of selecting an appropriate JVM version for maximizing performance.
Understanding JVM Basics
Before diving into the versions and performance metrics, it's essential to understand the fundamental role of the JVM in Java applications.
-
What is the JVM?
The JVM is an abstract computing machine that enables Java bytecode to be executed on any platform. It provides the environment in which Java programs run, handling memory management, code execution, and garbage collection. -
How does it work?
When you compile a Java program, it translates the Java source code into bytecode (.class files). The JVM reads this bytecode and interprets it or compiles it into native code at runtime.
Evolution of JVM Versions
The JVM has evolved significantly over the years. Each version introduces new features, enhancements, and optimizations. Let's take a look at some of the notable versions.
Java 8 (Released in March 2014)
Java 8 marked a pivotal change with significant improvements:
- Lambda Expressions: Simplified syntax for functional programming.
- Java Streams API: A powerful abstraction for processing sequences of elements (lists, arrays).
- Default Methods: Allow interfaces to have method implementations.
Performance Insights
Java 8 introduced the G1 Garbage Collector, designed for applications with large heaps and providing predictable pause times. Below is a simple example to demonstrate how to enable G1:
java -XX:+UseG1GC -jar your-application.jar
This option enhances performance by improving garbage collection timing, making it suitable for large applications.
Java 11 (Released in September 2018)
Java 11 is a Long-Term Support (LTS) version, meaning it receives long-term updates. This version brought:
- New HTTP Client: A more modern API for HTTP requests.
- Flight Recorder: An event recording system for profiling applications without much overhead.
Performance Benefits
The removal of older features like applets and Java EE modules reduces bloat, leading to lower memory consumption. Here’s a basic example of using the new HTTP client:
import java.net.URI;
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class HttpExample {
public static void main(String[] args) throws Exception {
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(new URI("https://api.example.com/data"))
.build();
HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
System.out.println(response.body());
}
}
Using the new API can lead to better performance and less code complexity.
Java 17 (Released in September 2021)
Java 17 is the latest LTS version, bringing even more features such as:
- Sealed Classes: Allowing better control over class hierarchies.
- Pattern Matching for
instanceof
: Simplifying type checks. - Enhanced
switch
statements: Making the syntax cleaner.
Performance Enhancements
The Java 17 release also improved performance optimizations across the board. As an example, let’s look at a sealed class implementation:
sealed class Shape permits Circle, Rectangle {
// common functionality for shapes
}
final class Circle extends Shape {
// Circle specific implementation
}
final class Rectangle extends Shape {
// Rectangle specific implementation
}
This feature enhances performance by reducing the need for polymorphic checks, making the execution path cleaner and faster.
Choosing the Right JVM Version for Your Application
Choosing the right JVM version depends on various factors including application requirements, performance needs, and any dependencies on libraries or frameworks. Below are some guidelines:
1. Long-Term Support Needs
If your application requires a stable environment without frequent upgrades, opt for LTS versions like Java 11 or 17.
2. Performance Requirements
Test your application under different JVM versions to benchmark performance. Use tools like JMH (Java Microbenchmark Harness) to evaluate the performance impacts of specific features.
3. Feature Utilization
For applications that leverage functional programming heavily, Java 8 is often sufficient. If more advanced features are required, moving to Java 11 or 17 is wise.
4. Security and Updates
Staying updated with the JVM is crucial for security. Each version brings important security fixes and updates.
5. Ecosystem Compatibility
Evaluate the libraries and frameworks you are using. Ensure that they are compatible with the JVM version you plan to adopt.
Best Practices for JVM Performance Tuning
No matter which JVM version you choose, performance tuning is crucial for optimal results. Below are some tried-and-tested techniques:
1. Garbage Collection Tuning
Selecting the appropriate garbage collector (like G1 or ZGC for low-latency requirements) can immensely benefit performance.
Example of specifying the garbage collector:
java -XX:+UseZGC -jar your-application.jar
2. Heap Size Configuration
Adjusting the heap size can reduce garbage collections. Tools like -Xms
and -Xmx
allow you to set the initial and maximum heap sizes.
3. JVM Flags
Utilize JVM flags for specific configurations that best fit your application needs. Experimentation can lead to discovering optimal settings.
4. Monitoring Tools
Leveraging tools like JConsole, VisualVM, or Java Mission Control can help monitor JVM performance and detect bottlenecks.
Key Takeaways
Choosing the right JVM version is instrumental in maximizing your Java application’s performance. Regular updates to the JVM not only improve performance but also add new features and enhance security. Whether you are working with Java 8, 11, or the latest 17 version, each offers its unique advantages and optimizations.
Remember to continuously test, monitor, and tune your application to harness the essential capabilities of the JVM effectively. By doing so, you are not just relying on new versions but actively engaging in the process of maximizing your application's performance.
For further details and to stay updated with Java developments, consider following resources like Oracle's Java Documentation and Java Magazine.
By following the guidelines and best practices mentioned here, you will be equipped to choose the right JVM version and enhance the performance of your Java applications significantly. Happy coding!