Mastering GC Log Analysis: Common Pitfalls to Avoid
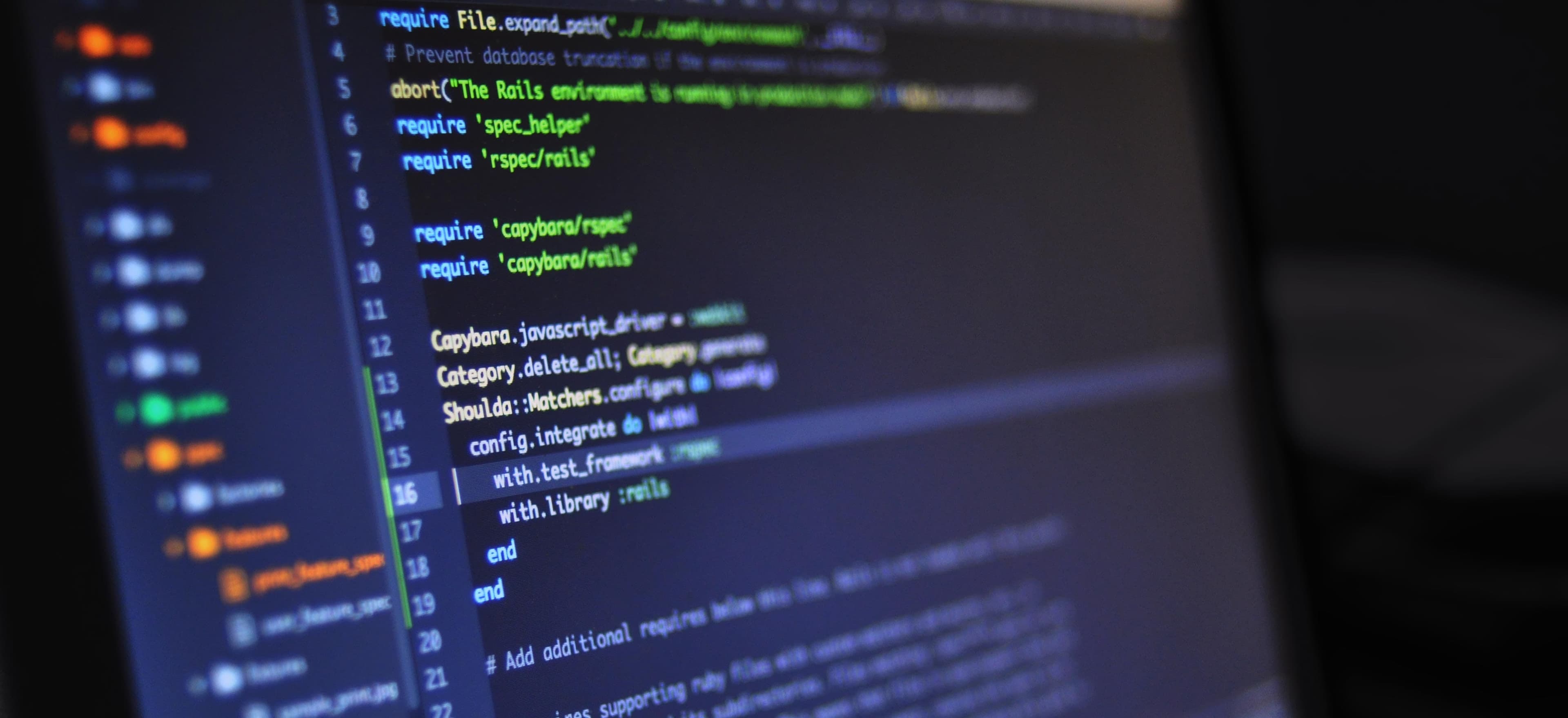
- Published on
Mastering GC Log Analysis: Common Pitfalls to Avoid
Garbage Collection (GC) is a crucial part of Java's memory management system. For developers and system administrators, understanding GC logs is essential for maintaining optimal performance in Java applications. However, analyzing GC logs can be daunting, especially with the multitude of parameters and metrics to consider. In this post, we will explore common pitfalls that developers encounter when analyzing GC logs and provide strategies to avoid them.
What Are GC Logs?
GC logs are records generated by the Java Virtual Machine (JVM) that provide details about the garbage collection process. These logs contain valuable insights about memory usage, garbage collection pauses, and the overall efficiency of the JVM in managing memory.
To enable GC logging, you can use JVM flags like:
-XX:+PrintGCDetails -XX:+PrintGCDateStamps -Xloggc:<filename>
This command line sets the JVM to print detailed GC logs, including timestamps and memory usage before and after collections.
Understanding Common Pitfalls in GC Log Analysis
1. Ignoring GC Frequency and Duration
Many developers focus solely on the duration of GC pauses without considering how often they occur. High-frequency collections can be just as detrimental as long pauses. Monitoring both frequency and duration is essential for a holistic understanding of GC behavior.
// Sample code to calculate GC frequency and duration
long gcStartTime = System.currentTimeMillis();
// Simulate workload
long gcEndTime = System.currentTimeMillis();
long gcDuration = gcEndTime - gcStartTime;
In this example, you're measuring the duration of a simulated GC event. By tracking multiple events, you can calculate frequency and identify patterns.
2. Overlooking Different GC Algorithms
Java offers several garbage collection algorithms:
- Serial GC
- Parallel GC
- Concurrent Mark-Sweep (CMS) GC
- G1 GC
- ZGC (for low-latency applications)
Each algorithm has nuances that affect how memory is managed. Understanding the specific algorithm in use is crucial for accurate analysis. Switching to a different GC algorithm can yield performance benefits.
For instance, G1 GC is typically tuned for applications with large heaps and mostly predictable pause times. You can set it with:
-XX:+UseG1GC
3. Failing to Segregate Application Events
You should separate application-specific events from GC events for clarity. Combining them can hinder your ability to pinpoint performance issues. Leverage tools like Elastic Stack to visualize both application and GC events.
4. Misinterpreting Metrics
It is easy to misinterpret certain metrics without the proper context. For example, if you see high heap usage, it doesn’t necessarily mean that memory management is inefficient—it could indicate your application’s workload characteristics.
Key metrics to monitor include:
- Heap Usage
- Number of Minor vs. Major GC Events
- Throughput (percentage of time spent doing actual work)
Each of these requires careful consideration in conjunction with other system metrics.
// Example metric collection for heap usage
long heapUsage = Runtime.getRuntime().totalMemory() - Runtime.getRuntime().freeMemory();
System.out.println("Heap Usage: " + heapUsage + " bytes");
5. Relying Solely on GC Logs
While GC logs provide valuable information, they should not be your only source of truth. Combine GC log analysis with other monitoring tools like Prometheus or New Relic for a more comprehensive picture of application performance.
6. Neglecting System Parameters
Monitoring system parameters such as CPU and I/O wait time can offer insights that GC logs may not capture. High CPU usage can lead to delayed GC events, while I/O bottlenecks may result in inefficient memory reclamation.
Best Practices for GC Log Analysis
1. Automate Log Analysis
Instead of manually parsing logs, consider using automated tools such as GCViewer or GCeasy which can provide visual analytics to simplify the interpretation of GC logs.
2. Regularly Tune GC Settings
Garbage collection tuning is an iterative process. Regularly review and modify GC flags based on application performance and memory usage.
3. Analyze Over Time
Don't just analyze GC logs from a single instance; study them over time. Trends are easier to identify and analyze when you have a longer dataset to work with.
4. Document Your Findings
It's important to document your observations and any actions taken based on those observations. This record can help inform future tuning efforts and create a repository of knowledge for your team.
Closing Remarks
Mastering GC log analysis is a powerful skill that can significantly optimize your Java application’s performance. By avoiding common pitfalls—like ignoring GC frequency, misinterpreting metrics, and poorly segregating application events—you can take your performance tuning efforts to the next level.
Remember that GC tuning is a continuous learning process. Stay updated with the latest JVM enhancements and keep experimenting with configurations. With practice, you will become adept at deciphering the complex narratives conveyed through your GC logs.
For further reading on Java performance tuning, consider checking out these resources:
- Java Performance Tuning
- The Definitive Guide to Java Performance Testing
Harness the power of GC log analysis, and ensure your Java applications are running at their optimal best!
Checkout our other articles