Unlocking PostgreSQL's Hidden Power: Tips for jOOQ Integration
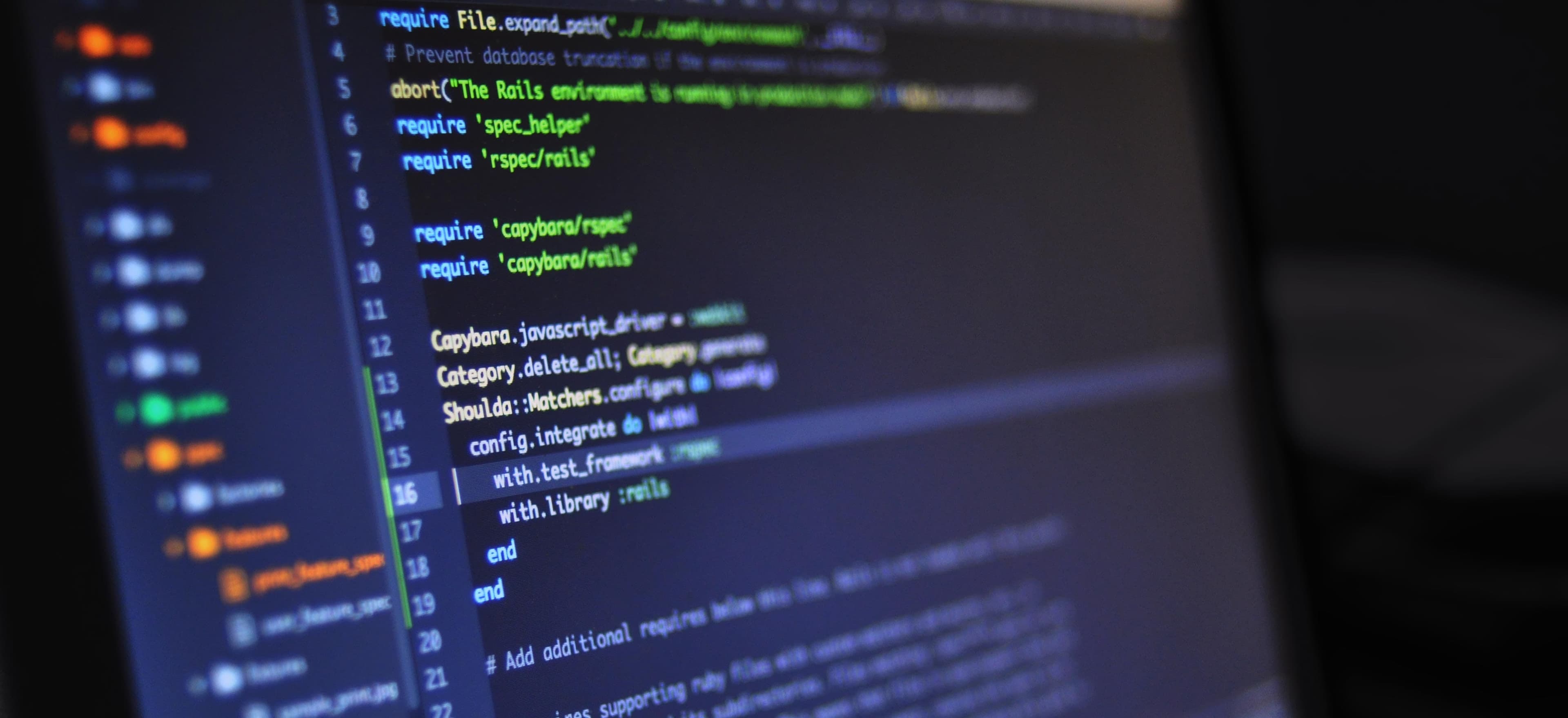
- Published on
Unlocking PostgreSQL's Hidden Power: Tips for jOOQ Integration
Java developers often seek powerful solutions for interacting with databases. When working with PostgreSQL, jOOQ (Java Object Oriented Querying) stands out as an exceptional choice for achieving type-safe queries and offering a fluent API. This post explores actionable tips to fully leverage PostgreSQL's unique features through jOOQ integration, helping you enhance your applications' capabilities.
Understanding jOOQ
jOOQ is a library that provides a way to build SQL queries in a type-safe manner. It translates Java code to SQL by generating Java classes from your database schema. This creates a seamless interchange between your application and the database.
Here are key advantages of using jOOQ:
- Type-Safety: Avoids runtime errors caused by SQL syntax issues.
- Fluency: Makes writing SQL less verbose and more intuitive.
- Database Features: Enables utilizing PostgreSQL's specific features effortlessly.
Setting Up jOOQ with PostgreSQL
1. Maven Dependency
To integrate jOOQ into your Java project, you should include it as a dependency. If you are using Maven, add the following snippet to your pom.xml
:
<dependency>
<groupId>org.jooq</groupId>
<artifactId>jooq</artifactId>
<version>3.15.6</version>
</dependency>
<dependency>
<groupId>org.postgresql</groupId>
<artifactId>postgresql</artifactId>
<version>42.3.1</version>
</dependency>
The jOOQ dependency enables you to utilize its querying capabilities, while the PostgreSQL driver allows jOOQ to communicate effectively with your database.
2. Generate jOOQ Classes
Before proceeding, you need to set up code generation to create Java classes based on your PostgreSQL database schema. Configure the maven-jooq-codegen plugin in your pom.xml
:
<plugin>
<groupId>org.jooq</groupId>
<artifactId>jooq-codegen</artifactId>
<version>3.15.6</version>
<executions>
<execution>
<id>generate-sources</id>
<goals>
<goal>generate</goal>
</goals>
<configuration>
<jdbc>
<driver>org.postgresql.Driver</driver>
<url>jdbc:postgresql://localhost:5432/yourdatabase</url>
<user>yourusername</user>
<password>yourpassword</password>
</jdbc>
<generator>
<database>
<name>org.jooq.meta.postgres.PostgresDatabase</name>
<includes>.*</includes>
</database>
<target>
<packageName>com.yourpackage.jooq</packageName>
<directory>target/generated-sources/jooq</directory>
</target>
</generator>
</configuration>
</execution>
</executions>
</plugin>
This setup will automatically generate jOOQ classes based on your PostgreSQL database setup each time you run Maven.
Leveraging PostgreSQL Features Through jOOQ
Now that you have jOOQ set up, let’s dive into some specific PostgreSQL features and how to utilize them effectively with jOOQ.
1. JSON and JSONB Support
PostgreSQL offers extensive support for JSON data types, allowing you to store, query, and manipulate JSON data effectively. jOOQ includes query capabilities specifically for these data types.
Example Code Snippet
Suppose you have a table users
with a JSON column preferences
:
CREATE TABLE users (
id SERIAL PRIMARY KEY,
name VARCHAR(50),
preferences JSONB
);
You can query for users whose preferences contain a specific key using jOOQ:
import static com.yourpackage.jooq.Tables.USERS;
import org.jooq.DSLContext;
import org.jooq.JSONB;
DSLContext create = DSL.using(connection, SQLDialect.POSTGRES);
Result<Record> result = create.select()
.from(USERS)
.where(USERS.PREFERENCES.containsKey("theme").isTrue())
.fetch();
for (Record r : result) {
System.out.println("User: " + r.get(USERS.NAME));
}
Commentary on the Code
In this snippet, we utilized the containsKey
method available in jOOQ for JSONB types. This level of integration ensures that you enhance your application's functionality while maintaining clean code. Always remember that leveraging PostgreSQL's JSON features enables handling complex data structures efficiently.
2. Common Table Expressions (CTEs)
With PostgreSQL supporting Common Table Expressions (CTEs), jOOQ allows you to utilize this SQL feature for better query structuring, especially when dealing with complex data relationships.
Example Code Snippet
Imagine needing to calculate the average age of users who have been active recently. You can create a CTE to simplify this query:
Result<Record> result = create.with("active_users")
.as(create.select(USERS.ID, USERS.NAME, USERS.AGE)
.from(USERS)
.where(USERS.ACTIVE.isTrue()))
.select(avg(field("AGE", Integer.class)).as("average_age"))
.from(table("active_users"))
.fetch();
Commentary on the Code
Using CTEs aids in breaking down large queries into manageable parts. This code snippet enhances readability and reuse of complex SQL logic by encapsulating it in a distinct block. Always consider CTEs for queries that require recursive computations or repeated logic structures.
3. Window Functions
PostgreSQL’s window functions allow performing analytics and summations across a set of rows related to the current row. jOOQ gives you the tools to access these powerful SQL features easily.
Example Code Snippet
Suppose you wish to rank users based on their scores:
Result<Record> result = create.select(USERS.NAME,
DSL.rowNumber().over().orderBy(USERS.SCORE.desc()).as("rank"))
.from(USERS)
.fetch();
for (Record r : result) {
System.out.println("User: " + r.get(USERS.NAME) + ", Rank: " + r.get("rank"));
}
Commentary on the Code
This code illustrates how you can use window functions like rowNumber()
efficiently. The use of DSL.rowNumber().over()
simplifies the invocation of ranking logic while preserving code clarity. Consider combining window functions with PARTITION BY
for more advanced analytics.
Wrapping Up
Integrating PostgreSQL with jOOQ opens up a vast potential to harness the full power of your database in a safe and type-controlled environment. We discussed establishing jOOQ, generating classes from your database schema, and leveraging PostgreSQL-specific features like JSON, CTEs, and window functions.
Additional Resources
By implementing these techniques, your application development can achieve a higher level of sophistication and performance when working with PostgreSQL. Start experimenting with these jOOQ features and unlock your database's true potential!