Common Mistakes in Calculating Averages with Java Arrays
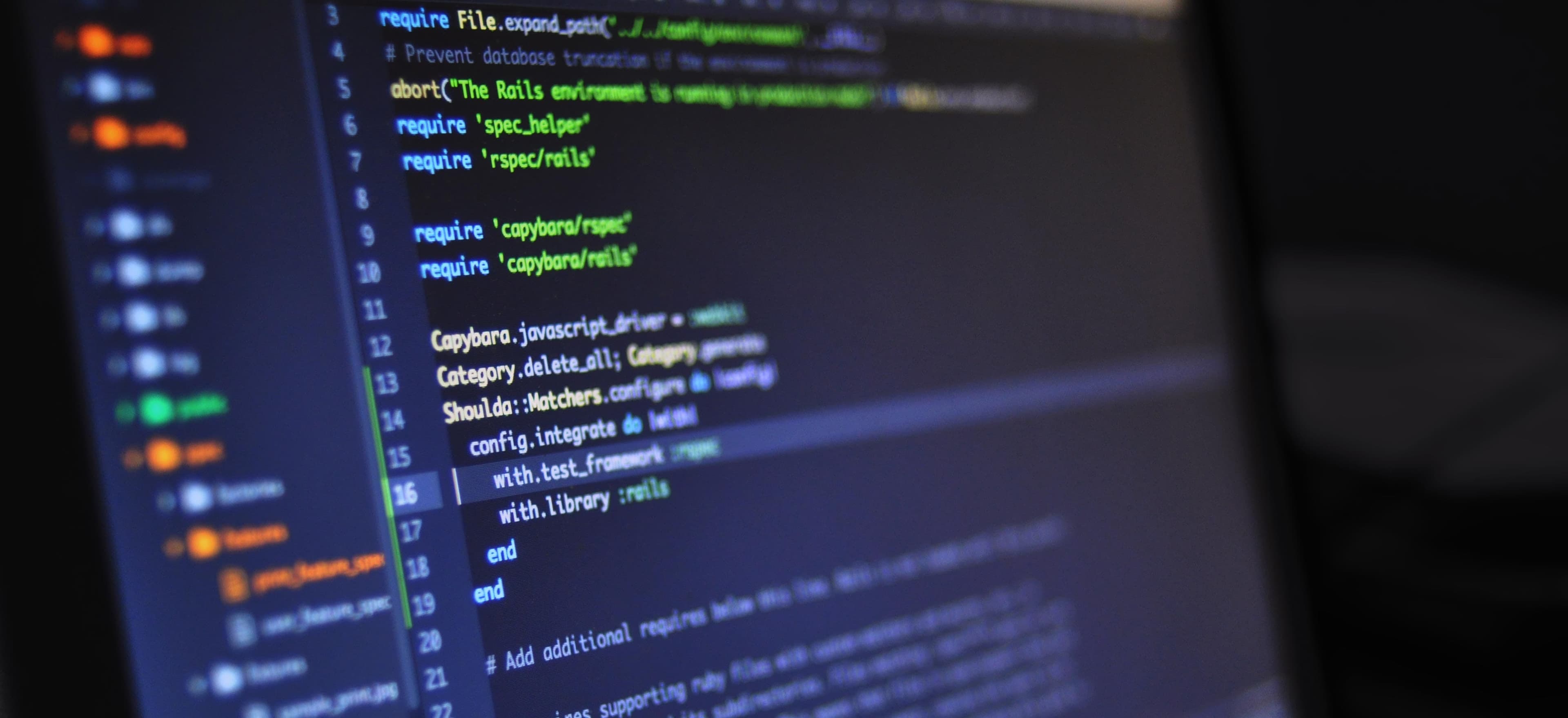
- Published on
Common Mistakes in Calculating Averages with Java Arrays
When it comes to programming, especially in Java, calculating averages using arrays is a fundamental skill every developer should possess. However, mistakes can often creep in, leading to erroneous results and inefficiencies in code. In this blog post, we'll dissect common pitfalls in calculating averages with Java arrays and provide clear, actionable solutions to enhance your coding skills.
The Basics of Averages
Before diving into common mistakes, let’s clarify what an average is. The average, or mean, of a set of numbers is calculated by adding all the numbers together and dividing by the count of those numbers.
The formula for calculating the average is straightforward:
average = (sum of all numbers) / (count of numbers)
In the context of Java and arrays, we are often dealing with primitive data types, such as int
, double
, or float
. The data type you choose can have an impact on your final result, particularly regarding precision and rounding.
A Simple Example
Let’s start with a simple example of calculating an average of integers in an array:
public class AverageCalculator {
public static void main(String[] args) {
int[] numbers = {2, 4, 6, 8, 10};
double average = calculateAverage(numbers);
System.out.println("The average is: " + average);
}
public static double calculateAverage(int[] arr) {
int sum = 0;
for (int number : arr) {
sum += number;
}
return (double) sum / arr.length;
}
}
Commentary on the Example
-
Data Type for Average: Notice the cast
(double)
before the division. This is done to ensure we get a floating-point result rather than an integer division. In Java, if both operands in a division are integers, the result will also be an integer. -
Using Enhanced For-Loop: The enhanced for-loop (for-each loop) simplifies iteration significantly and increases readability.
-
Returning Results: The function returns a
double
, ensuring you have enough precision for the average.
Common Mistakes Made
While the example provided is straightforward, developers often overlook a few key points when calculating averages. Let's explore these common mistakes.
1. Integer Division
One of the most common mistakes occurs when programmers forget to cast the sum to a double. As stated earlier, integer division truncates the decimal, thus leading to incorrect averages.
return sum / arr.length; // Incorrect if both are integers
Solution:
Always ensure at least one of the operands in the division is a double:
return (double) sum / arr.length; // Correct
2. Division by Zero
When working with arrays, it is crucial to handle cases where the array might be empty. If it is, dividing by arr.length
results in a java.lang.ArithmeticException
.
if (arr.length == 0) {
throw new IllegalArgumentException("Array cannot be empty");
}
3. Not Validating Input
It’s essential to validate the array before processing it. If the input can potentially contain invalid data (e.g., non-numeric values, nulls), consider adding checks to ensure the integrity of your data.
4. Floating-Point Precision
Averages can lead to floating-point precision issues, especially with a large number of operations. For instance, adding large float values can lead to loss of precision.
Better Approach Using BigDecimal
For critical applications requiring high precision, consider using BigDecimal
for your calculations.
import java.math.BigDecimal;
public static BigDecimal calculateAverage(int[] arr) {
BigDecimal sum = BigDecimal.ZERO;
for (int number : arr) {
sum = sum.add(BigDecimal.valueOf(number));
}
return sum.divide(BigDecimal.valueOf(arr.length), RoundingMode.HALF_UP);
}
Commentary on the BigDecimal
Example
-
Precision:
BigDecimal
allows you to perform high-precision arithmetic, which is critical in financial calculations or when high accuracy is necessary. -
Rounding Mode: The
divide
method supports different rounding modes, providing control over how results are rounded.
Closing the Chapter
Calculating an average in Java is straightforward, but it’s easy to overlook key details. By understanding the potential pitfalls of integer division, validating your inputs, and considering the precision of your calculations, you can avoid common mistakes.
For further reading on arrays and methods, check out the Java Arrays Documentation and Best Practices for Java Programming.
By being mindful of these common mistakes and applying best practices, you can enhance your programming skills and write more efficient, reliable code. Happy coding!