Overcoming Challenges in Java Interface Evolution
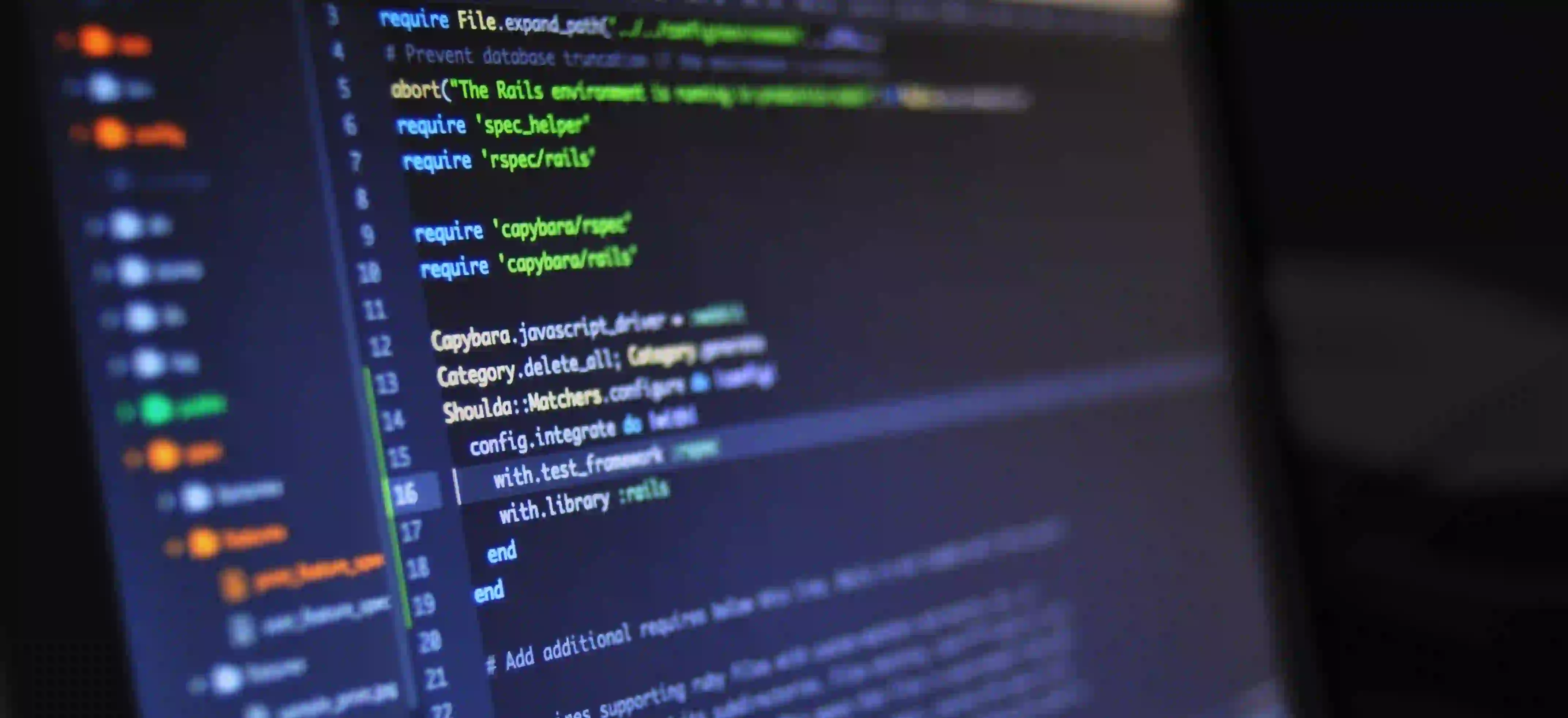
Overcoming Challenges in Java Interface Evolution
Java interfaces serve as contracts between classes and define methods that must be implemented by each class. As systems grow and evolve, the need to update these interfaces becomes a necessity. However, evolving interfaces in Java is not always straightforward. This blog will help you navigate the complexities of interface evolution by exploring challenges, best practices, and practical examples.
What is Interface Evolution and Why is it Important?
Interface evolution refers to the modification of an existing interface while maintaining backward compatibility. It's crucial because it allows developers to add new features without breaking existing implementations. For instance, if you're developing an API for external developers, a change that requires them to modify their code can lead to frustration and potential abandonment of your API.
Challenges in Interface Evolution
1. Backward Compatibility
One of the most prominent challenges in evolving Java interfaces is maintaining backward compatibility. Existing classes implementing the interface must continue to function correctly after any changes. Breaking changes can lead to compile errors or runtime exceptions, negatively impacting users of your API.
2. Method Signature Changes
Changing a method’s signature in an interface (e.g., parameter types, return type, or method name) is often a surefire way to break existing implementations. For example, consider an interface Calculator
:
public interface Calculator {
int add(int a, int b);
}
If you decide to change the add
method to accept double
parameters:
public interface Calculator {
double add(double a, double b);
}
All classes implementing this interface must update their implementation, leading to compatibility issues.
3. Adding New Methods
When you add new methods to an interface, existing classes that implement the interface are not required to implement these new methods, leading to inconsistencies. Thus, it creates a situation where an object of an implementing class may not support all methods of the interface.
4. Default Methods
Java 8 introduced default methods, which allow you to add new methods with a default implementation. This can mitigate some issues associated with new method addition, but can also create problems in cases where multiple interfaces providing default methods result in ambiguity (the infamous "diamond problem").
5. Legacy Code
When working with legacy codebases, evolutionary changes can become increasingly difficult. Existing methods might rely on outdated conventions or have multiple dependencies that, when altered, could lead to a cascading failure in the application.
6. Versioning of Interfaces
Maintaining multiple versions of an interface can be a fallback strategy, which can create additional overhead. This approach can lead to increased maintenance efforts, and developers may find it challenging to keep track of which implementations are compliant with which version.
Best Practices for Evolving Java Interfaces
1. Use Default Methods Judiciously
If you need to add methods to an interface, consider using default methods. This allows you to implement new functionality without forcing all implementing classes to adopt it.
For example:
public interface Calculator {
int add(int a, int b);
default double add(double a, double b) {
return a + b;
}
}
In this example, the addition of a new add
method is made optional for classes that implement Calculator
.
2. Employ Interface Segregation
Rather than having a giant interface with many methods, consider breaking interfaces into smaller, more focused interfaces. This aligns with the Interface Segregation Principle of SOLID principles. Smaller interfaces minimize the impact of changes, allowing for more granular control over implementations.
public interface Addable {
int add(int a, int b);
}
public interface Subtractable {
int subtract(int a, int b);
}
By employing segregation, you can expand your API with minimal risk to existing code.
3. Maintain Clear Documentation
Whenever you make changes to an interface, ensure that documentation is updated. This includes providing clear comments on methods, explaining what is new, what has changed, and the reasons behind the decisions. This transparency is vital for any developer utilizing your interface.
4. Implement Versioning for Interfaces
When you envision major modifications, consider introducing a new version of the interface. For example:
public interface CalculatorV2 {
double add(double a, double b);
}
public class BasicCalculator implements Calculator {
public int add(int a, int b) {
return a + b;
}
}
public class AdvancedCalculator implements CalculatorV2 {
public double add(double a, double b) {
return a + b;
}
}
With versioning, you allow consumers to adopt the new implementation at their own pace.
5. Test Thoroughly
Always add test cases when implementing changes to your interface. Unit tests are invaluable in ensuring backward compatibility. Automated testing can help identify potential breaking changes early in the development cycle.
Example: Evolving a Java Interface
Let's go through an example where we evolve a simple interface Shape
, which only calculates the area.
Original Interface:
public interface Shape {
double area();
}
public class Circle implements Shape {
private final double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
public double area() {
return Math.PI * radius * radius;
}
}
Evolving the Interface
Suppose we want to allow for surface area and volume calculations for shapes in the future.
public interface Shape {
double area();
// New default method for volume calculation
default double volume() {
throw new UnsupportedOperationException("Volume not applicable for this shape.");
}
}
public class Cube implements Shape {
private final double side;
public Cube(double side) {
this.side = side;
}
@Override
public double area() {
return 6 * side * side;
}
@Override
public double volume() {
return side * side * side;
}
}
In this evolution:
- The
Shape
interface now includes a new method forvolume
with a default implementation that throws an exception. - The
Cube
class implements botharea
and the newvolume
method, while theCircle
class remains unaffected.
Summary of Why This Works
- By using a default method, the
Shape
interface has evolved without breaking existing implementations. - Future shapes, like
Cube
, can implement new functionality without affecting current users, keeping the API flexible.
Final Thoughts
Interface evolution in Java requires careful planning and implementation to avoid breaking existing code. By following best practices such as using default methods, versioning interfaces, and maintaining clear documentation, developers can effectively manage the challenges.
Understanding how to navigate these changes is vital for fostering long-term maintainability in your codebase. For additional insights on evolving interfaces, consider checking out Java's official documentation on interfaces or exploring the SOLID design principles.
With the right approach, you can ensure a smooth journey through code evolution, allowing your software products to adapt and thrive in an ever-changing landscape.
Happy coding!