Overcoming Performance Hurdles in GraalVM for Polyglot Apps
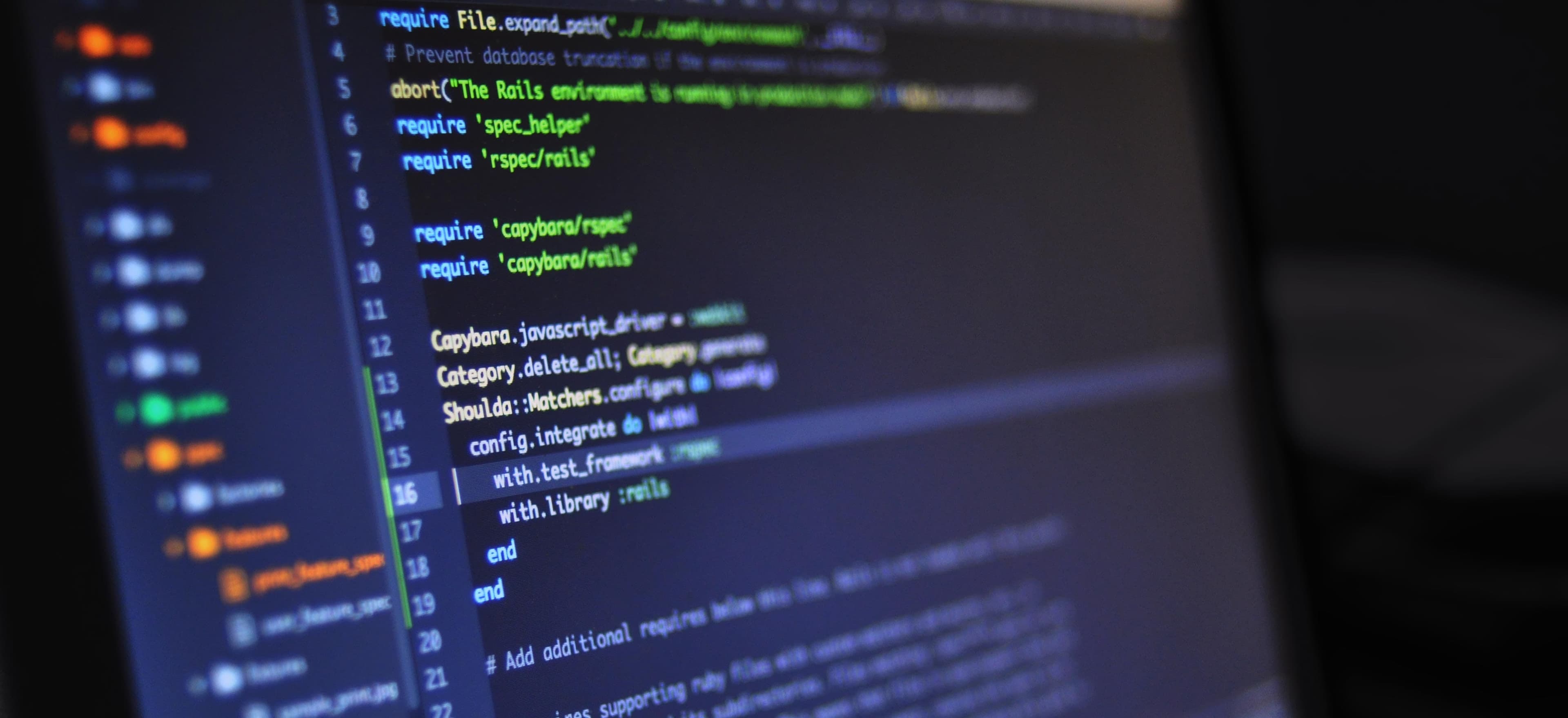
- Published on
Overcoming Performance Hurdles in GraalVM for Polyglot Apps
In the modern world of software development, creating polyglot applications—that is, applications built with multiple programming languages—is increasingly becoming a necessity. This is especially true for organizations that want to leverage the strengths of different languages without compromising on performance. One tool promising to enhance the development and execution of polyglot applications is GraalVM.
However, while GraalVM provides many benefits, it also introduces its own set of performance hurdles. In this blog post, we’ll discuss how to overcome those hurdles, focusing on practical strategies and code examples.
What is GraalVM?
GraalVM is a high-performance runtime that provides support for multiple programming languages, including Java, JavaScript, Ruby, Python, and R. It allows for seamless interoperability between these languages, meaning you can call functions across language boundaries with minimal overhead. It also offers ahead-of-time compilation, which can significantly improve startup time and overall performance.
Why Use GraalVM?
- Polyglot Capabilities: You can use multiple languages in your application and share data between them seamlessly.
- Performance: GraalVM can outperform traditional Java Virtual Machines (JVMs) in many scenarios through optimizations.
- Reduced Latency: It supports native image generation, which significantly decreases startup time.
Performance Hurdles in GraalVM
Despite its advantages, developers may encounter several performance hurdles when deploying polyglot applications on GraalVM. Some common issues include:
- Slow Interoperability: While GraalVM facilitates calling across languages, there may be a performance penalty for those calls.
- Memory Consumption: Native images can sometimes lead to higher memory usage when not tuned properly.
- JIT Compilation Overhead: Just-In-Time (JIT) compilation can introduce latency in long-running applications.
Strategies to Overcome Performance Hurdles
1. Optimize Interoperability
One of the biggest challenges in GraalVM polyglot applications lies in the interoperability between languages. While it supports language intercommunication, it is essential to minimize the overhead where possible.
Code Example: Optimizing Inter-language Calls
import org.graalvm.polyglot.*;
public class PolyglotExample {
public static void main(String[] args) {
try (Context context = Context.create()) {
// Javascript code execution
Value jsFunction = context.eval("js", "function add(a, b) { return a + b; }");
// Call JS function from Java
double result = jsFunction.execute(3, 5).asDouble();
System.out.println("Result from JS: " + result);
}
}
}
Why This Matters: It’s critical to consider how data is passed between functions in different languages. Primitive types are passed more efficiently than complex objects. You should also ensure you optimize your JavaScript or Python functions for performance.
2. Use Native Images Wisely
Using GraalVM’s native image feature is excellent for reducing startup time. However, improper configuration can lead to unexpected performance degradation.
Code Example: Generating a Native Image
mvn clean package
native-image -jar myapp.jar --no-fallback
When you run the above command, GraalVM’s native-image tool will generate an executable from your Java application.
Why This Matters: Pay attention to image parameters, like --no-fallback
. If a compatibility issue arises, the fallback mechanism could introduce unnecessary overhead, defeating the purpose of using native images for performance.
3. Profile Your Application
Profiling allows you to identify the actual performance bottlenecks in your application, whether in Java code or while interacting with other languages.
- Faster Debugging: Profiling tools integrated into GraalVM such as VisualVM can help you analyze method execution times, memory usage, and thread activity.
Code Example: Profiling via Command Line
java -agentlib:flight recorder -jar myapp.jar
Why This Matters: Profiling gives you insights into not just Java-specific performance issues but can also reveal bottlenecks in your polyglot architecture so you can optimize accordingly.
4. Tune JVM Flags
Fine-tuning JVM flags can adjust how the GraalVM runtime behaves. These options can enhance garbage collection, change heap memory allocation, and more.
Effective JVM Flags:
-XX:+UseG1GC
: Enables the G1 garbage collector, which is beneficial for applications with large heaps and is designed for low-latency needs.-Xmx512m
: Sets the maximum heap size limit to optimize memory usage.
5. Apply Best Practices in Code
Coding best practices apply regardless of whether you're working in Java, JavaScript, or other languages. Here are some crucial considerations:
- Avoid excessive object creation across language borders.
- Batch operations when passing data from one language to another.
Simple Example of Batching
function batchAdd(arr) {
return arr.reduce((sum, value) => sum + value, 0);
}
Calling this function with a single array rather than multiple values can be much less costly.
Bringing It All Together
GraalVM is a powerful tool for building polyglot applications that capitalize on the strengths of different programming languages. However, developers must be aware of the potential performance hurdles and actively work to mitigate them using the strategies outlined in this post.
From optimizing interoperability to using native images wisely and profiling your application, each step can contribute to overcoming performance challenges in your GraalVM applications.
By adopting best practices and leveraging GraalVM's capabilities wisely, you can build high-performance polyglot applications that not only function seamlessly but also deliver an excellent user experience.
Additional Resources
- For a deeper dive into GraalVM's capabilities, visit the GraalVM official site.
- Learn about specific memory optimizations in GraalVM by checking the GraalVM documentation.
By implementing these techniques, you will be well on your way to taking full advantage of GraalVM's polyglot capabilities while avoiding performance pitfalls.
Checkout our other articles