Why Automated Tests Fail as Effective Documentation
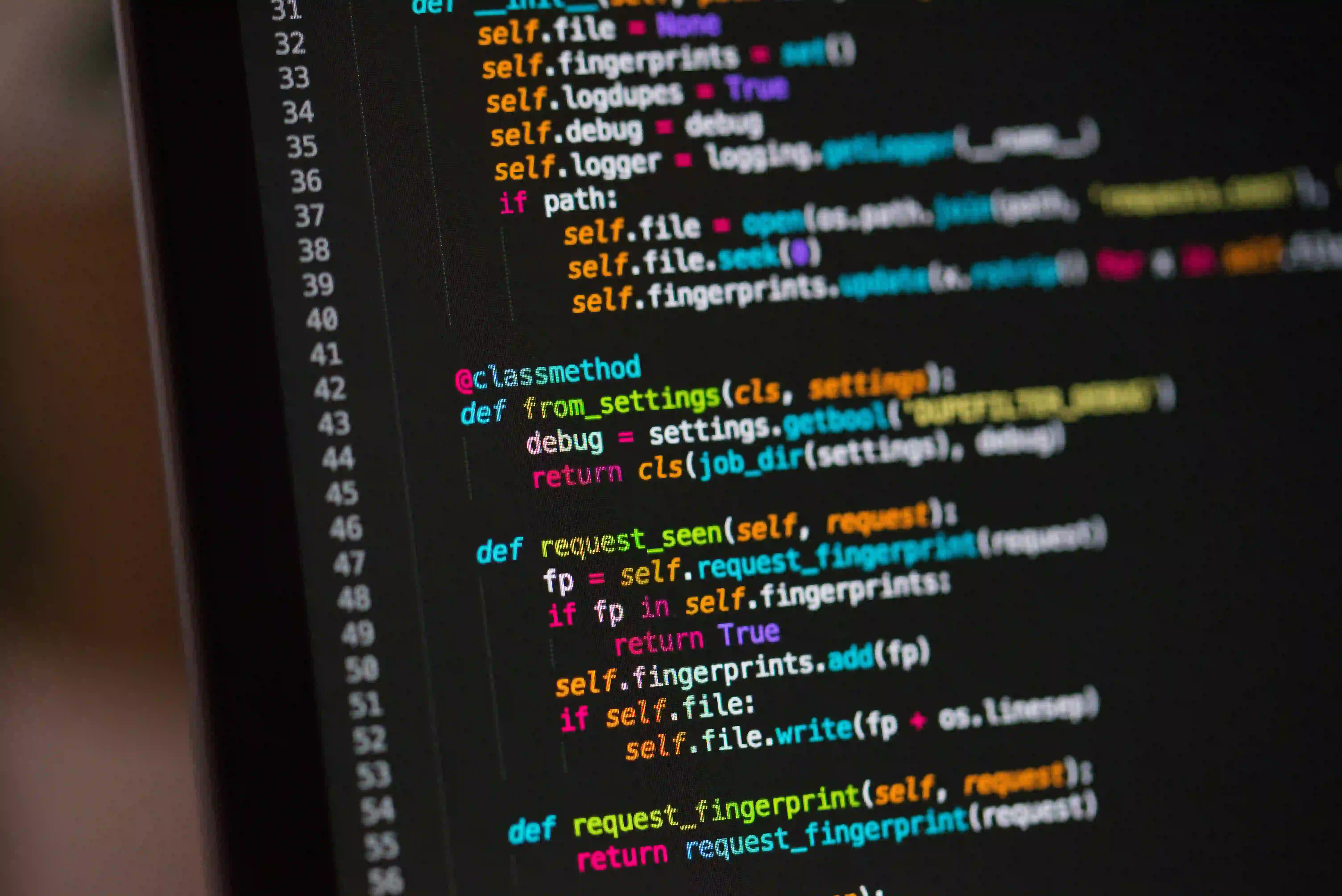
Why Automated Tests Fail as Effective Documentation
Automated tests are often touted as a panacea for software quality assurance, promising faster feedback loops and consistent validation of application functionality. However, they frequently fall short in one critical area: effective documentation. This article explores why automated tests fail to serve as comprehensive documentation, what it means for software development teams, and how to create better documentation practices.
Understanding Automated Tests
Before diving into the pitfalls of automated tests as documentation, it’s essential to understand what automated tests are and why they are used. Automated tests include unit tests, integration tests, and end-to-end tests designed to execute application code automatically to verify that it is functioning as intended.
For developers, automated tests are invaluable. They help identify bugs early in the development cycle, facilitate code refactoring without fear of breaking existing functionality, and provide a safety net that assures feature behaves as expected after changes.
Example: Simple Unit Test
Consider the following basic example of a Java unit test using JUnit:
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
assertEquals(5, calculator.add(2, 3), "Adding 2 + 3 should equal 5");
}
}
In this code, a simple test ensures that the add
method of the Calculator
class works correctly. However, the test does not provide any context. A newcomer examining this test won’t glean the rationale behind the choice of inputs or the broader functionality of the Calculator
class.
The Limitations of Automated Tests as Documentation
1. Lack of Context
Automated tests tend to focus on functionality rather than providing context. They validate whether a piece of code works but do not explain why that code exists or how it fits into the larger system.
For example, consider a test for a user authentication process. The test verifies that valid credentials grant access to the application but fails to explain what “valid credentials” are, or why they are structured in a particular way. This omission leads to ambiguity, particularly for new developers or non-technical stakeholders.
2. Evolving Requirements
Software requirements frequently evolve, and automated tests must be updated accordingly. When requirements change, tests can become outdated or irrelevant without corresponding updates to the documentation. This lack of synchronization can lead to confusion over whether the tests accurately represent the current state of the system.
3. Fragility of Tests
Automated tests can be brittle, meaning they might fail due to changes in the environment or minor adjustments in the codebase, even if the core functionality still works. For instance, a refactoring might improve code quality but inadvertently break a test that does not sufficiently isolate the behavior being tested.
4. Incomplete Coverage
Automated tests often fail to cover all aspects of a system. Certain edge cases might not be tested, leaving significant gaps in documentation. Additionally, tests may focus solely on the "happy paths" – the scenarios in which everything goes right – while neglecting alternative flows that reveal how the system behaves under different conditions.
5. Technical Language Barrier
Automated tests are inherently technical, written in code that may not be easily understood by non-developers. This creates barriers for product managers, business analysts, and other stakeholders who may not have the technical skills to interpret test results, leading to gaps in the overall documentation.
Moving Beyond Automated Tests
To bridge the gap between automated tests and effective documentation, a more holistic approach is needed. Here are several best practices:
1. Complement Tests with Descriptive Documentation
Automated tests should always be accompanied by comprehensive documentation. Each test case should have a well-defined purpose and be tied to clear documentation that explains:
- The overall system architecture.
- Key components and their responsibilities.
- The reasoning behind specific implementation choices.
This approach ensures that anyone reading the tests can also refer to the contextual information required to understand them.
2. Use Code Comments Wisely
Properly commenting code can help clarify complex logic, describe design decisions, and inform the reader about the intention behind certain implementations. Each test case should implement comments to explain its functionality clearly.
Example: Commented Unit Test
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class CalculatorTest {
// Test addition operation of two integers
@Test
public void testAddition() {
Calculator calculator = new Calculator();
// Verifies that the sum of 2 and 3 equals 5
assertEquals(5, calculator.add(2, 3), "Adding 2 + 3 should equal 5");
}
}
3. Centralize Documentation
Consider using a centralized documentation tool to create a living document that captures updated requirements, architecture, and lessons from past failures. Tools such as Confluence or MkDocs can help make documentation accessible.
4. Encourage Team Collaboration
Foster an environment where testers, developers, and stakeholders collaborate on writing both automated tests and documentation. Regular discussions can ensure everyone understands the codebase, requirements, and how tests fit into the overall development cycle.
5. Regularly Review and Update
Automated tests, like any document, should be periodically reviewed and updated. Establish a routine for revisiting both the tests and the documentation they accompany to ensure alignment with the current state of the system.
Summary
While automated tests are essential for maintaining software quality, they need not become the primary source of documentation. Their limitations, including lack of context, evolving requirements, and technical barriers, prevent them from serving this purpose effectively. By adopting complementary documentation practices, encouraging collaboration, and maintaining an up-to-date understanding of the system, teams can create a holistic approach that bridges the gap between testing and effective documentation.
A combination of clear documentation and robust automated testing will not only strengthen a project but also improve communication among team members, leading to successful outcomes in the fast-paced world of software development.
For further reading on best documentation practices and the role of testing in software development, consider checking out The Twelve-Factor App and Clean Code by Robert C. Martin.