Common Pitfalls When Transitioning to Microservices with Spring
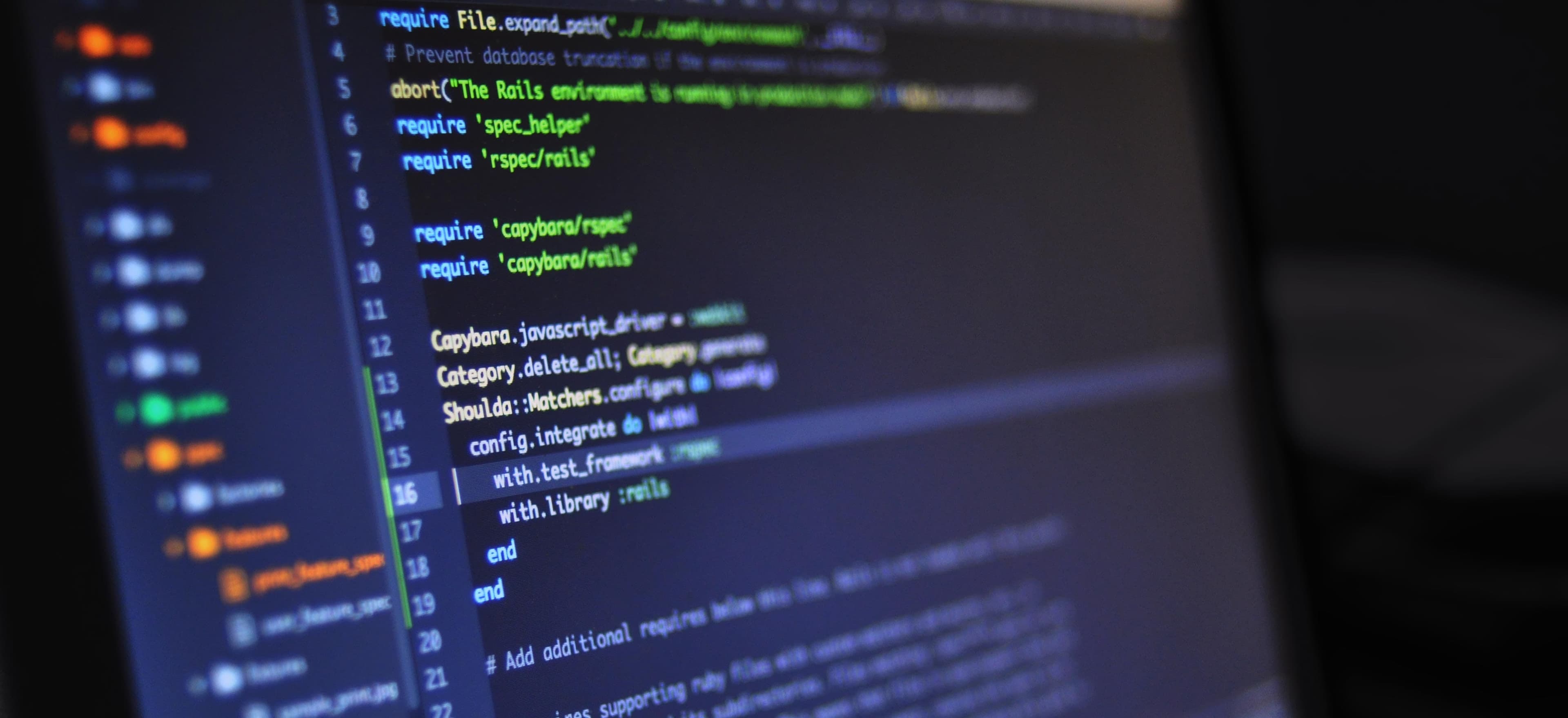
- Published on
Common Pitfalls When Transitioning to Microservices with Spring
The transition from a monolithic architecture to microservices is a daunting yet rewarding journey for many organizations. Spring Framework has made this transition easier with Spring Boot and Spring Cloud, providing tooling and frameworks to ease development and deployment. Nevertheless, there are common pitfalls teams can stumble into. In this blog post, we will walk through some of these pitfalls and how to effectively navigate them.
Understanding Microservices
Before diving into the pitfalls, let’s clarify what microservices are. Microservices architecture breaks down an application into smaller, independent services that communicate over a network. This approach offers several advantages:
- Scalability: Services can be scaled independently.
- Flexibility: Different services can use different tech stacks.
- Resilience: Failure in one service doesn’t mean the entire application goes down.
However, these advantages can quickly become disadvantages if the transition isn't handled carefully.
Pitfall 1: Overlooking Domain Design
A microservices architecture is centered around specific business capabilities. Poor domain design can lead to services that are either too granular or too large, making them hard to maintain.
Solution: Use Domain-Driven Design (DDD)
Investing time in identifying bounded contexts is crucial. Bounded contexts help define the boundaries within which a specific domain model applies. A proper DDD also assists in understanding the relationships between different services.
For example, consider an e-commerce application that may consist of services like Product
, User
, and Order
. Here’s a simplistic representation of a Product
service:
@RestController
@RequestMapping("/products")
public class ProductController {
private final ProductService productService;
public ProductController(ProductService productService) {
this.productService = productService;
}
@GetMapping("/{id}")
public ResponseEntity<ProductDTO> getProduct(@PathVariable String id) {
ProductDTO product = productService.findProductById(id);
return ResponseEntity.ok(product);
}
@PostMapping
public ResponseEntity<ProductDTO> createProduct(@RequestBody ProductDTO productDTO) {
ProductDTO createdProduct = productService.createProduct(productDTO);
return ResponseEntity.status(HttpStatus.CREATED).body(createdProduct);
}
}
In this example, the ProductController
manages the lifecycle of products, promoting a separation of concerns crucial to effective microservice design.
Pitfall 2: Ignoring Service Communication
One of the fundamental principles of microservices is inter-service communication. This could be synchronous or asynchronous, depending on your design. Overlooking communication strategies can lead to performance bottlenecks and tight coupling between services.
Solution: Choose the Right Communication Method
- Synchronous communication: Useful for real-time data requirements (e.g., REST).
- Asynchronous communication: Beneficial for decoupled service interactions (e.g., messaging queues).
An example of an asynchronous event-driven architecture using Spring Cloud Stream might look like this:
@EnableBinding(ProductEventSource.class)
public class ProductService {
private final ProductRepository productRepository;
public ProductService(ProductRepository productRepository) {
this.productRepository = productRepository;
}
public void createProduct(ProductDTO productDTO) {
Product product = productRepository.save(productDTO.toEntity());
ProductEvent event = new ProductEvent(product.getId(), product.getName());
productEventSource.output().send(MessageBuilder.withPayload(event).build());
}
}
This code snippet sends a ProductEvent
whenever a new product is created, allowing other microservices to react accordingly.
Pitfall 3: Lack of Monitoring and Logging
Tracking the performance and health of individual microservices can be challenging. Lack of proper monitoring can lead to prolonged downtime and a lack of understanding about the application's behavior.
Solution: Implement Centralized Logging and Monitoring
Utilize tools like Spring Cloud Sleuth for distributed tracing and ELK Stack (Elasticsearch, Logstash, Kibana) for centralized logging. This will help in diagnosing issues across microservices.
Here’s a simple logging setup:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@RestController
public class OrderController {
private static final Logger logger = LoggerFactory.getLogger(OrderController.class);
@GetMapping("/orders/{id}")
public ResponseEntity<OrderDTO> getOrderDetails(@PathVariable String id) {
logger.info("Fetching order with ID: {}", id);
// Order retrieval logic
}
}
With logging in place, every key action is recorded, enabling you to trace problems back in time.
Pitfall 4: Data Management Challenges
Managing data in a microservice architecture is complex. Each service typically has its own database, which can lead to data consistency issues.
Solution: Consider the CAP Theorem
Understanding the CAP theorem (Consistency, Availability, Partition Tolerance) is essential. You have to make tradeoffs between consistency and availability in a distributed environment.
Here's a simplified example where we may use an Event Sourcing
pattern and CQRS
(Command Query Responsibility Segregation) to manage data:
public class OrderEvent {
private final String orderId;
private final String status;
public OrderEvent(String orderId, String status) {
this.orderId = orderId;
this.status = status;
}
// Getters...
}
@Service
public class OrderService {
// Event store can be a dedicated microservice
public void placeOrder(OrderDTO orderDTO) {
OrderEvent orderEvent = new OrderEvent(orderDTO.getId(), "CREATED");
eventStore.publish(orderEvent); // Publish order event
}
}
In this case, the OrderService
handles the creation of an OrderEvent
, which can be processed by other services asynchronously.
Pitfall 5: Underestimating Deployment Complexity
Microservices offer flexibility, but they also bring deployment complexity. Managing multiple services, each evolving at a different pace, can be challenging.
Solution: Automate Your Deployment Pipeline
Utilize CI/CD tools such as Jenkins, GitHub Actions, or GitLab CI for automatic builds, tests, and deployment. Docker and Kubernetes can further simplify deployment, providing container orchestration.
Here’s a basic Dockerfile for a Spring Boot application:
FROM openjdk:11-jre-slim
COPY target/myapp.jar app.jar
ENTRYPOINT ["java", "-jar", "/app.jar"]
Using containerization ensures that applications run seamlessly in different environments.
The Last Word
Transitioning to microservices with Spring can significantly improve your software's architecture and maintainability when approached thoughtfully. Avoid pitfalls such as poor domain design, improper communication strategies, lack of monitoring, data management challenges, and deployment complexities.
By addressing these common pitfalls, you can ensure a smoother transition and harness the full potential of microservices. Remember, the key to success lies in understanding the fundamentals of microservices and continuously evaluating your architecture. For more insights on microservices, consider reading Martin Fowler's article or exploring the official Spring documentation.
Happy coding!
Checkout our other articles