Resolving PSQLEXCEPTION: UUID vs Character Varying Error
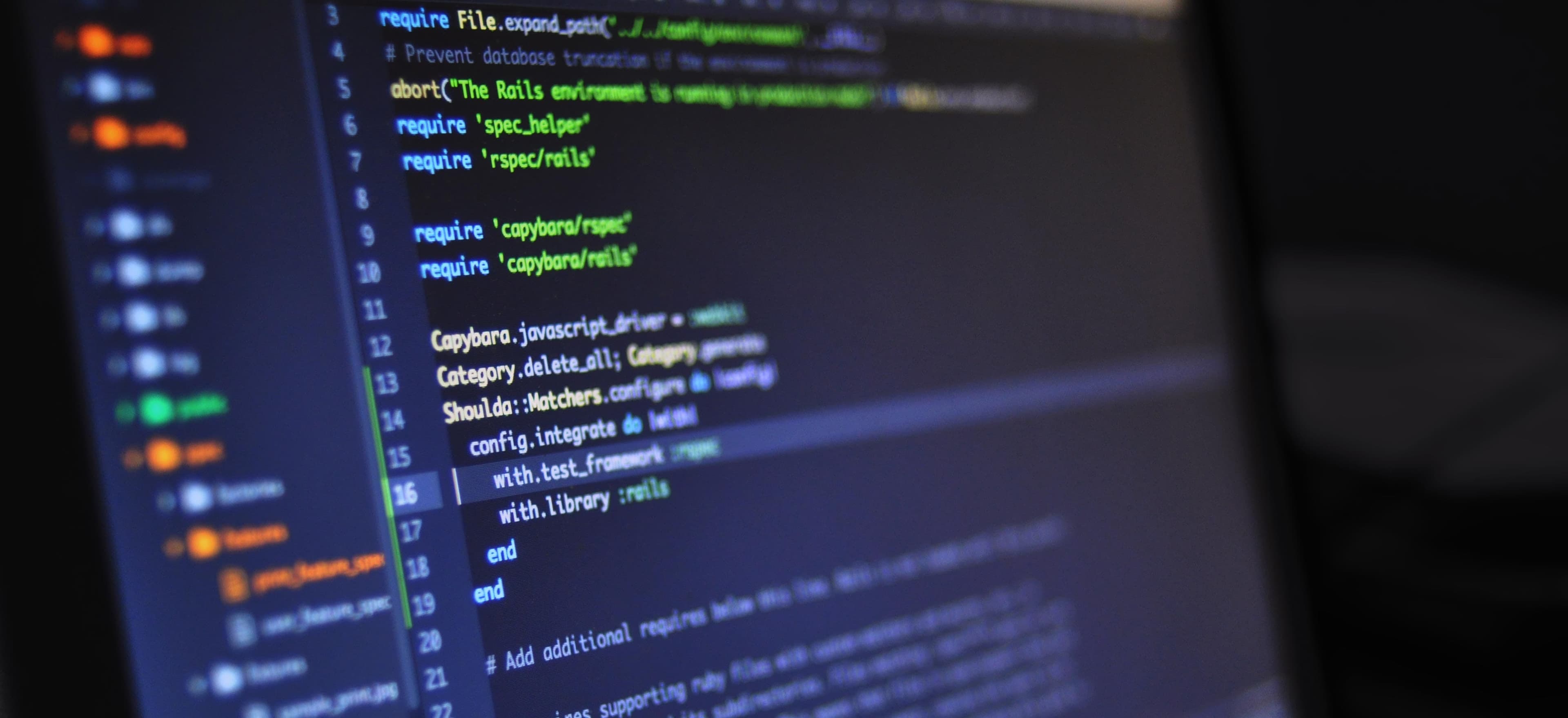
- Published on
Resolving PSQLEXCEPTION: UUID vs Character Varying Error in Java
When it comes to developing applications with PostgreSQL databases using Java, one might occasionally run into the notorious PSQLEXCEPTION that highlights a conflict between UUID and character varying types. This post will guide you through understanding this error, troubleshooting it, and ultimately resolving it effectively.
What is the PSQLEXCEPTION?
The PSQLEXCEPTION
is thrown in Java whenever there is a problem with the SQL operation, be it an incorrect statement, a connectivity issue, or, as in our context, data type mismatches.
The error we will focus on specifically arises when there is a conflict between UUID (Universally Unique Identifier) and VARCHAR data types.
When an application tries to insert or manipulate a UUID value while treating it as a VARCHAR (or vice versa), PostgreSQL doesn’t know how to handle it, and consequently, throws this exception.
Understanding UUID and VARCHAR in PostgreSQL
PostgreSQL comes with several data types, but both UUID and VARCHAR are widely used.
-
UUID: This is a 128-bit number used to uniquely identify information in computer systems. PostgreSQL has a specific UUID type which provides functionalities that make working with unique identifiers easier and more efficient.
-
Character varying (VARCHAR): This data type is used for variable-length strings. It is useful for textual data that could change in size.
Confusion often arises when a UUID is stored or manipulated as a VARCHAR.
Example Scenario
Let’s say you have a table defined as follows:
CREATE TABLE users (
id UUID PRIMARY KEY,
name VARCHAR(100),
email VARCHAR(255)
);
If you try to insert a row with an ID that is not appropriately formatted as a UUID, or if you try to query this table with the wrong type, you're likely to see a PSQLEXCEPTION similar to:
org.postgresql.util.PSQLException: ERROR: invalid input syntax for type uuid: "some-string-value"
Best Practices to Avoid UUID vs VARCHAR Issues
Use the Correct Data Type
Always ensure you are using the UUID
data type when working with UUID values. For getters and setters in your Java application, ensure they correspond correctly.
Check Entity Mappings
If you are using an Object-Relational Mapping (ORM) tool like Hibernate, make sure your entity field definitions are correctly set up:
import org.hibernate.annotations.Type;
import javax.persistence.Entity;
import javax.persistence.Id;
import java.util.UUID;
@Entity
public class User {
@Id
@Type(type = "uuid-char") // Ensures the mapping is correct
private UUID id;
private String name;
private String email;
// Constructors, getters, and setters
}
By specifying @Type(type = "uuid-char")
, we inform Hibernate how to handle the UUID properly.
Utilize Proper Utility Methods
When generating UUIDs in Java, use the built-in UUID
class.
import java.util.UUID;
public class UUIDExample {
public static void main(String[] args) {
UUID uniqueKey = UUID.randomUUID(); // Generates a random UUID
System.out.println("Generated UUID: " + uniqueKey.toString());
}
}
This method ensures you always handle UUIDs in their appropriate form without accidental conversion to strings.
Code Snippet: Insert Using PreparedStatement
To prevent SQL injection attacks and to ensure data types align correctly, utilize PreparedStatement
for inserts.
Here's how you can insert into the users
table correctly:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.UUID;
public class DatabaseInsertExample {
private static final String INSERT_USER_SQL = "INSERT INTO users (id, name, email) VALUES (?, ?, ?)";
public static void main(String[] args) {
UUID userId = UUID.randomUUID();
String name = "John Doe";
String email = "john.doe@example.com";
try (Connection connection = DriverManager.getConnection("jdbc:postgresql://localhost:5432/your_db", "username", "password");
PreparedStatement preparedStatement = connection.prepareStatement(INSERT_USER_SQL)) {
preparedStatement.setObject(1, userId); // This handles the UUID correctly
preparedStatement.setString(2, name);
preparedStatement.setString(3, email);
int rowAffected = preparedStatement.executeUpdate();
System.out.println("User Inserted: " + rowAffected);
} catch (SQLException e) {
e.printStackTrace();
}
}
}
In this code:
- We connect to the PostgreSQL database.
- We prepare the SQL statement. The
setObject
method correctly handles the UUID type. - Finally, we execute the update and print the result.
Console Output
If our process is correct, the console should print the number of rows affected, thus confirming the successful insertion of a user.
Handling Exceptions Graciously
When working with databases, it is essential to handle exceptions to avoid abrupt application crashes. Here's a refined try-catch block that can help in dealing with PSQLEXCEPTION
:
try {
// Database operations
} catch (SQLException e) {
if (e.getSQLState().equals("22023")) { // SQL State for Invalid UUID
System.err.println("Invalid UUID format: " + e.getMessage());
} else {
System.err.println("Database error: " + e.getMessage());
}
}
Understanding SQL state codes allows you to respond specifically to errors.
To Wrap Things Up
Resolving the PSQLEXCEPTION
regarding UUID versus VARCHAR in PostgreSQL is all about ensuring data type fidelity. Use the correct data types, ensure your Java application correctly aligns with the database schema, utilize efficient utilities, and always handle exceptions gracefully.
For further reading on Java and PostgreSQL integration, consider visiting:
By following the suggestions laid out in this post, you can significantly reduce the chances of encountering UUID and VARCHAR data type conflicts in your Java applications. Happy coding!
Checkout our other articles