Maximizing Efficiency: Common Pitfalls with Guava Bloom Filters
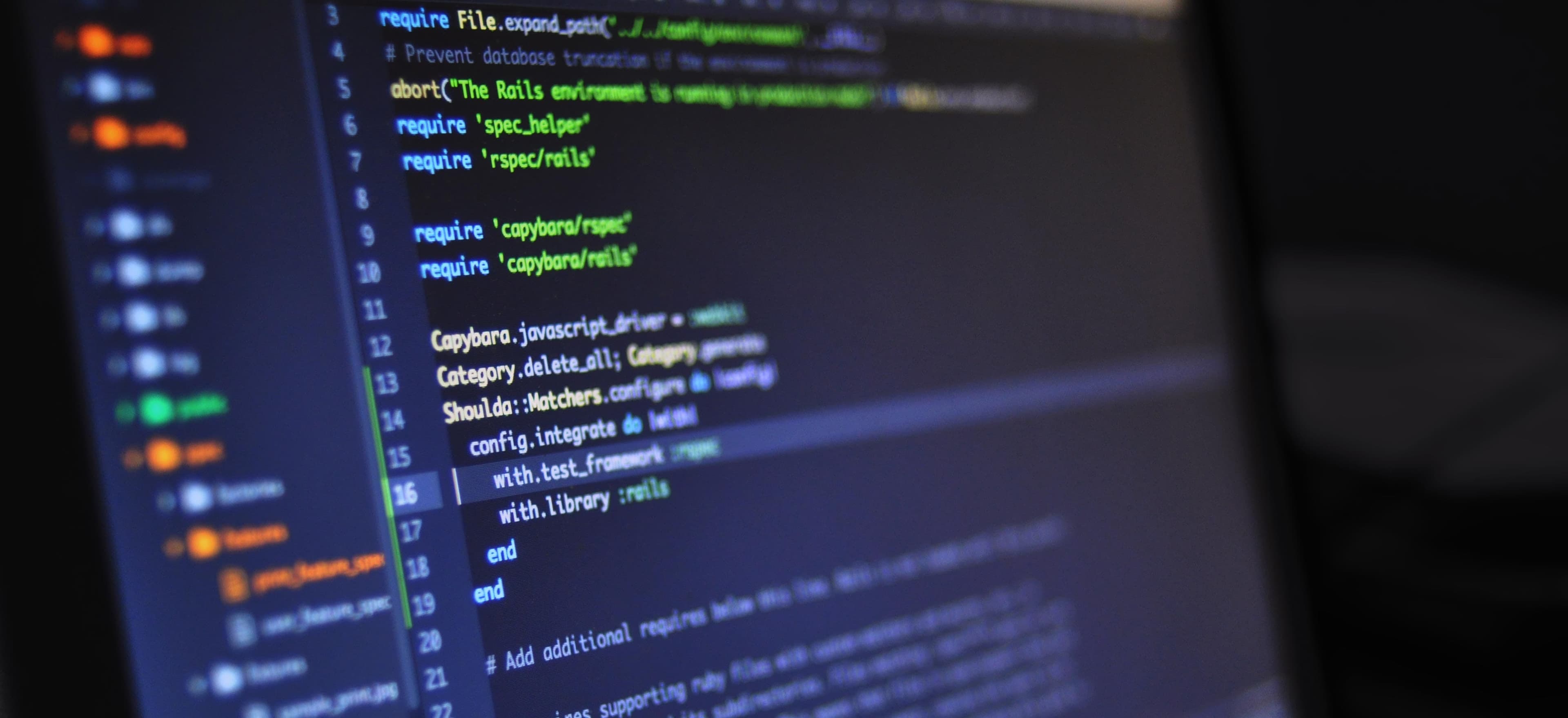
- Published on
Maximizing Efficiency: Common Pitfalls with Guava Bloom Filters
In the world of data structures and algorithms, Bloom filters are widely recognized for their efficiency in space and speed when working with large datasets. However, not all implementations are created equal. In this blog post, we will explore the Guava Bloom filter, a popular choice in Java programming, and uncover common pitfalls that developers encounter. By addressing these challenges, you will be better equipped to utilize Bloom filters effectively in your applications.
What is a Bloom Filter?
A Bloom filter is a space-efficient probabilistic data structure used to test whether an element is a member of a set. It can produce false positives, meaning it may indicate that an element is in the set when it is not, but it guarantees no false negatives. This makes Bloom filters particularly useful in applications where the dataset is large, and the cost of storage or a full membership check is prohibitive.
Basic Working Principle
The general working principle of a Bloom filter involves a bit array and multiple hash functions. Here’s how it works:
- Initialization: You create a bit array of a fixed size initialized to 0.
- Hashing: Each element you add to the filter is processed by several hash functions, which return indexes in the bit array.
- Setting Bits: The bits at these indexes are set to 1.
- Membership Test: To check if an element is in the set, you again use the same hash functions. If all corresponding bits are set to 1, the element is considered to be in the set; otherwise, it is not.
Now, let’s delve into Guava's implementation and the common pitfalls faced when using it.
Setting Up the Guava Bloom Filter
The Guava library, developed by Google, provides a robust and easy-to-use implementation of Bloom filters. Here’s a simple example of how to set up a Bloom filter in Java using Guava:
import com.google.common.hash.BloomFilter;
import com.google.common.hash.Funnels;
public class BloomFilterExample {
public static void main(String[] args) {
// Initialize a Bloom filter for Strings with an expected insertions of 1000 and a false positive probability of 0.01
BloomFilter<String> bloomFilter = BloomFilter.create(Funnels.stringFunnel(), 1000, 0.01);
// Add elements to the filter
bloomFilter.put("apple");
bloomFilter.put("banana");
bloomFilter.put("orange");
// Check membership
System.out.println("Is 'apple' in the filter? " + bloomFilter.mightContain("apple")); // true
System.out.println("Is 'grape' in the filter? " + bloomFilter.mightContain("grape")); // false
}
}
In the code above, we initialize a Bloom filter with a specific number of expected insertions (1000) and a desired false positive probability (0.01). The Funnels.stringFunnel()
method provides the necessary hashing functionality for Strings.
This simple implementation showcases the strengths of Bloom filters, but there are common pitfalls that developers often encounter when using them.
Common Pitfalls with Guava Bloom Filters
1. Incorrect Configuration Parameters
One of the most critical aspects of using a Bloom filter is choosing the right parameters. If your expected number of elements is underestimated, you will likely encounter an excessive number of false positives. Conversely, overestimating can lead to inefficient memory usage.
Pitfall Example:
// Incorrect parameter configurations can lead to issues
BloomFilter<String> bloomFilter = BloomFilter.create(Funnels.stringFunnel(), 500, 0.1); // Bad choice
Solution:
Conduct thorough research to understand your data. Ensure that you have appropriate predictions for your dataset size by examining previous data usage metrics or conducting small-scale experiments.
2. Ignoring False Positive Rates
Another common misunderstanding is how false positive rates work in Bloom filters. A lower false positive probability increases the size of the bit array and the number of hash functions required.
Let’s consider:
// High false positive rate
BloomFilter<String> bloomFilterHighFPR = BloomFilter.create(Funnels.stringFunnel(), 500, 0.5);
In this scenario, the false positive probability is exceedingly high, making it counterproductive for most applications.
Solution:
Choose the false positive rate based on your application's tolerance for errors. In general, a balance must be struck between memory usage and acceptable false positive rates. For example, a 1% false positive rate is often considered reasonable.
3. Not Handling Hash Collisions Properly
Bloom filters depend on several independent hash functions to minimize collisions. Guava automatically handles this via its internal methods. However, if you use a custom setup or other libraries, hash collision management can become an issue:
// Using custom hash function
BloomFilter<MyObject> bloomFilterCustomHash = BloomFilter.create(new MyCustomFunnel());
If your custom funnel doesn’t produce a uniform distribution of hash values, it might trigger collisions and consequently affect the reliability of the Bloom filter.
Solution:
Always use a proven, well-distributed hashing strategy. If you implement your own functions, ensure that they provide uniformity across the output distributions.
4. Forgetting to Consider Memory Usage
While Bloom filters are designed to be space-efficient, they still consume memory. If you're processing numerous datasets, you should consider scaling your Bloom filters according to your memory resources.
// Overusing Bloom filters without assessing resources
BloomFilter<String>[] bloomFilters = new BloomFilter[10000]; // Not feasible in memory
Solution:
Plan your approach, and create a memory budget for your application. Use Java’s memory monitoring tools to evaluate usage during development.
5. Misunderstanding the 'Might Contain' Check
Developers sometimes overlook the probabilistic nature of Bloom filters and use them in contexts demanding strict accuracy:
if (bloomFilter.mightContain("pineapple")) {
// Assumes it's definitely present, which can lead to issues
}
Solution:
Educate yourself and your team about how Bloom filters function. Consider Bloom filters as part of a broader system where additional verification layers are deployed as needed.
My Closing Thoughts on the Matter
Guava Bloom filters offer a powerful way to manage large datasets efficiently. However, understanding their limitations and common pitfalls is essential to leveraging them effectively. Always pay special attention to configuration parameters, false positive rates, hash function behavior, memory usage, and the probabilistic nature of the mightContain
checks.
By applying best practices and avoiding common mistakes, you can maximize the efficiency of Bloom filters in your Java applications. For deeper insights on Bloom filters, consider exploring resources such as Guava’s official documentation, or you may be interested in this informative article that provides a broader overview of the topic.
With this knowledge, you're now equipped to implement Bloom filters confidently and maximize your application's performance. Happy coding!
Checkout our other articles