Common Swagger UI Issues in Spring Boot and How to Fix Them
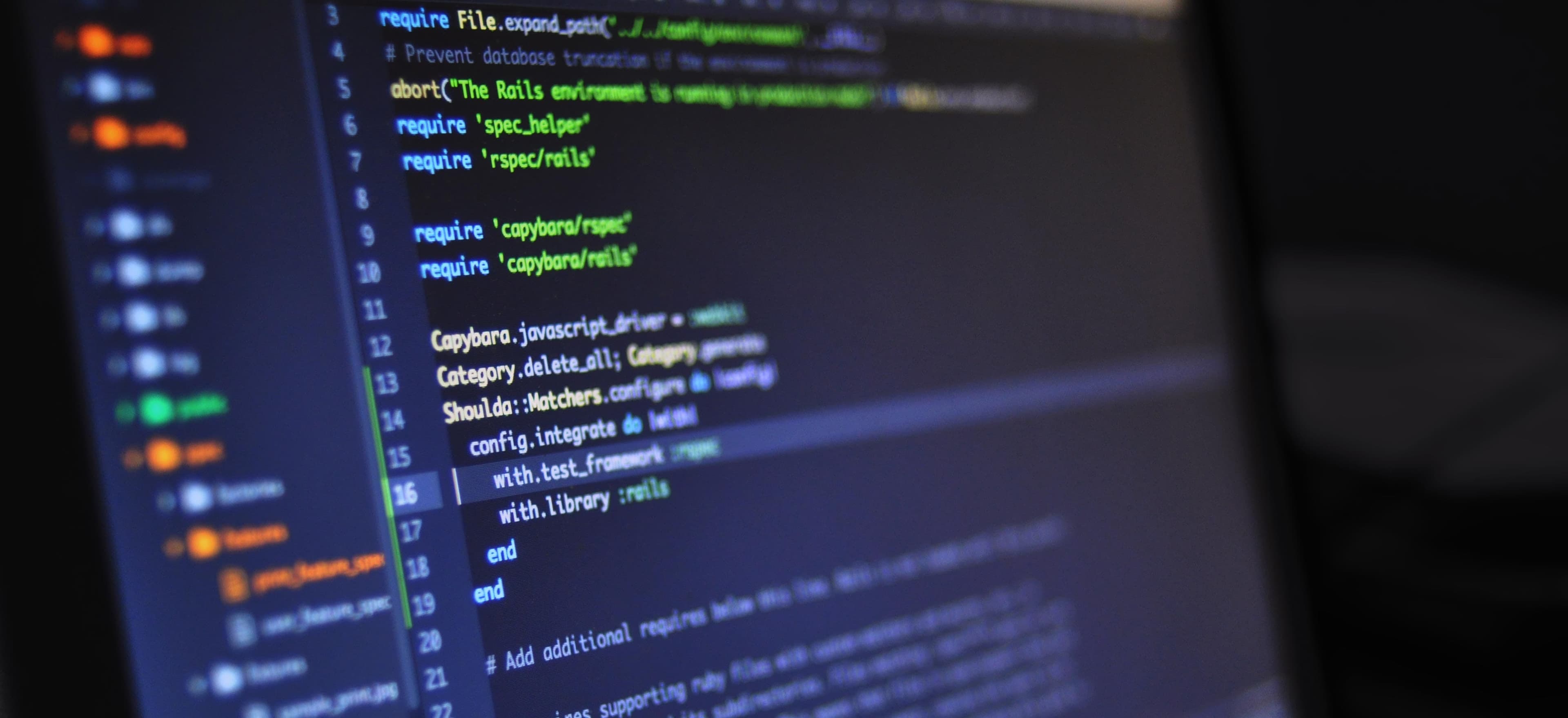
- Published on
Common Swagger UI Issues in Spring Boot and How to Fix Them
Spring Boot is a popular framework for building web applications and RESTful APIs in Java. When developing APIs, proper documentation is crucial for ease of use and maintenance. Swagger UI provides an effective way to visualize and interact with your API. However, developers often encounter various issues while integrating Swagger UI into their Spring Boot applications. In this article, we will explore common issues, understand their roots, and provide solutions to enhance your Swagger UI experience.
What is Swagger UI?
Swagger UI is a tool that allows developers to visualize and interact with REST API resources easily. It generates interactive documentation that displays available APIs, their parameters, responses, and possible error codes, making the API more accessible.
Why Use Swagger in Spring Boot?
Using Swagger UI with Spring Boot has several advantages:
- Ease of Documentation: Automatically generates documentation from your Java code using annotations.
- Interactive Testing: Allows developers and users to test endpoints directly from the browser.
- Standardization: Follows the OpenAPI Specification, making APIs easier to understand across teams.
Setting Up Swagger in Spring Boot
First, make sure you have the following dependencies in your pom.xml
:
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-boot-starter</artifactId>
<version>3.0.0</version>
</dependency>
Common Swagger UI Issues and Their Solutions
1. Swagger UI Not Loading
Issue
Sometimes, when you navigate to /swagger-ui/
, you may encounter a blank page or a 404 error, indicating that the Swagger UI resources are not found.
Solution
Ensure that you have the correct dependency and that your application is correctly configured. Make sure to include the following properties in your application.properties
:
springfox.documentation.enabled=true
springfox.documentation.swagger-ui.base-url=/swagger-ui/
2. API Annotations Not Appearing
Issue
After setting up Swagger, your API endpoints may not appear in the Swagger UI.
Solution
This usually relates to missing annotations on your controller. Ensure that you are using the appropriate annotations on your REST controller. Here’s an example:
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiOperation;
@RestController
@RequestMapping("/api")
@Api(value = "API for managing users")
public class UserController {
@GetMapping("/users")
@ApiOperation(value = "Fetch all users", response = List.class)
public List<User> getAllUsers() {
// Logic to retrieve users
return userService.findAll();
}
}
Here, the use of @Api
and @ApiOperation
helps document the controller and its methods effectively.
3. CORS Issues
Issue
If your Swagger UI is hosted on a different domain, you might face CORS (Cross-Origin Resource Sharing) issues which will prevent the UI from accessing the API endpoints.
Solution
You can solve this by adding a CORS configuration to your Spring Boot application. Create a WebMvcConfig
class to customize CORS settings:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebMvcConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://localhost:8080") // change to your allowed origin
.allowedMethods("GET", "POST", "PUT", "DELETE", "OPTIONS");
}
}
4. Swagger Not Showing Models
Issue
Sometimes, the models for your API responses do not appear in the documentation.
Solution
Ensure that your models are properly annotated. Use @ApiModel
and @ApiModelProperty
for models, as follows:
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
@ApiModel(description = "Details about the user")
public class User {
@ApiModelProperty(notes = "The unique ID of the user")
private Long id;
@ApiModelProperty(notes = "The user's name")
private String name;
// Getters and Setters
}
5. Endpoint Not Found (404 Error)
Issue
When trying to access your endpoints, you might get a 404 error, even though they seem to be set up correctly.
Solution
Verify that your controller’s @RequestMapping
and method-specific mappings (like @GetMapping
, @PostMapping
, etc.) are defined correctly:
@RestController
@RequestMapping("/api")
public class ProductController {
@GetMapping("/products/{id}")
public Product getProductById(@PathVariable Long id) {
// Logic to retrieve product
}
}
Make sure that the mapping path matches what you are trying to access in the Swagger UI.
Additional Swagger Configuration
To further enhance your Swagger configuration, consider adding custom API info:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import springfox.documentation.builders.PathSelectors;
import springfox.documentation.builders.RequestHandlerSelectors;
import springfox.documentation.spi.DocumentationType;
import springfox.documentation.spring.web.plugins.Docket;
import springfox.documentation.swagger.web.SwaggerResourcesProvider;
@Configuration
public class SwaggerConfig {
@Bean
public Docket api() {
return new Docket(DocumentationType.SWAGGER_2)
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.yourapp"))
.paths(PathSelectors.any())
.build()
.apiInfo(apiInfo());
}
private ApiInfo apiInfo() {
return new ApiInfoBuilder()
.title("Your API Title")
.description("Description of your API")
.version("1.0.0")
.build();
}
}
References
If you are keen to dive deeper into integrating Swagger with Spring Boot, consider checking out these resources:
Lessons Learned
Swagger UI is an invaluable tool for documenting and testing your Spring Boot APIs. By understanding the common issues that can arise during its integration and knowing how to resolve them, you ensure a smoother development process. Remember to regularly check Swagger's official documentation for updates and best practices. Happy coding!
Checkout our other articles