Maximizing Efficiency: The Art of HEAD Operation in Servers
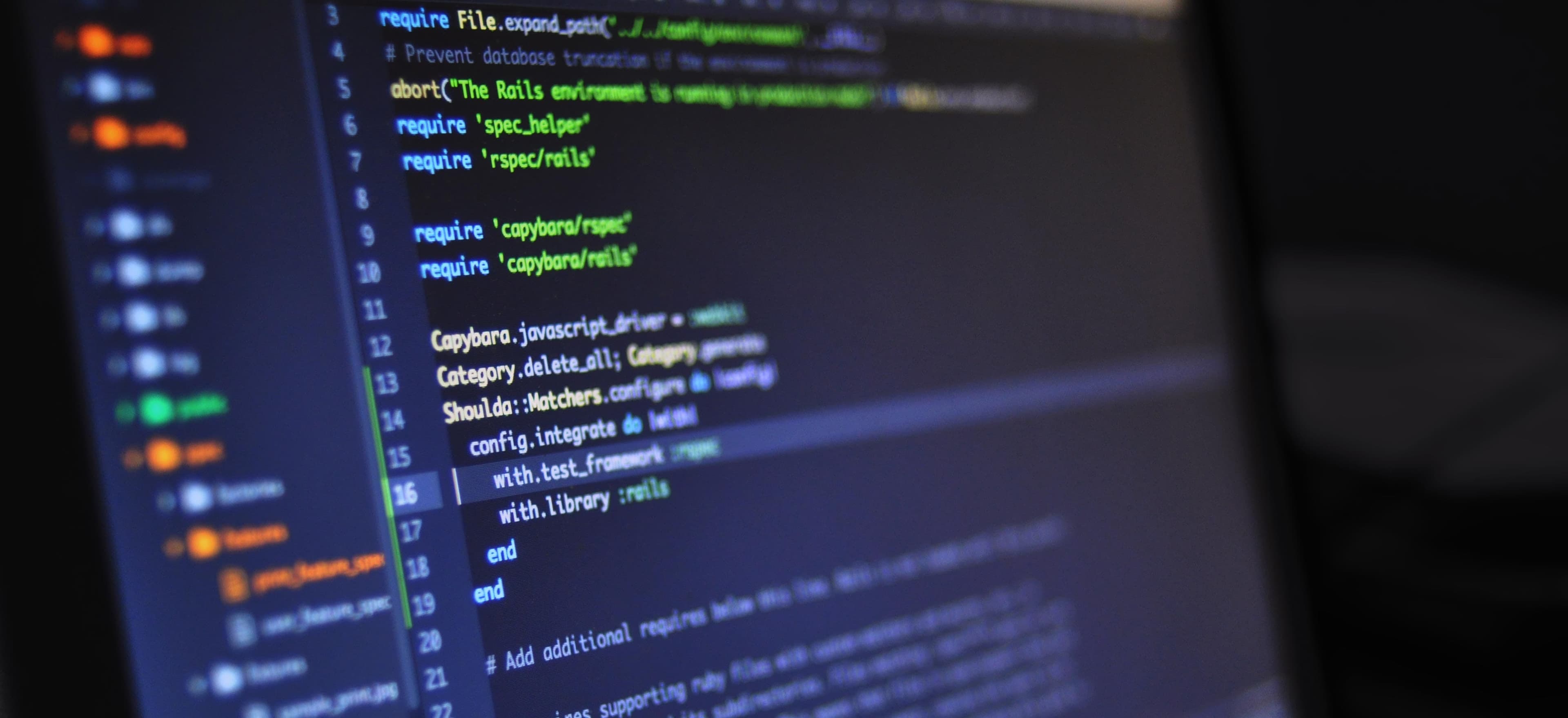
- Published on
Maximizing Efficiency: The Art of HEAD Operation in Servers
In the realm of web development and server management, HTTP methods play a vital role in how clients and servers communicate. Understanding these methods, particularly the often-overlooked HEAD operation, can lead to significant improvements in efficiency and performance. This blog post delves into the HEAD method, its applications, advantages, and usage in Java applications.
Understanding the HEAD Method
The HEAD method is one of the lesser-known HTTP request methods, but its utility is both profound and practical. Essentially, the HEAD request is identical to a GET request, except that the server responds with headers only, leaving out the body. This difference may seem minor, yet it has significant implications in many scenarios.
Why Use HEAD?
-
Reduced Bandwidth Usage: Since the server transmits only headers, it consumes less bandwidth. This is especially beneficial for resource-constrained environments or when dealing with large payloads.
-
Resource Verification: The HEAD method allows clients to check whether a resource has changed without downloading it. This is useful for caching mechanisms and maintaining efficient data synchronization.
-
Link Checking: It can be beneficial for tools that verify broken links, as it enables the existence of resources to be confirmed without downloading actual content.
Basic Syntax of the HEAD Request
The syntax of a HEAD request is straightforward. Here is a simple illustration:
HEAD /path/to/resource HTTP/1.1
Host: www.example.com
Server Response Example
Here’s an example of what a typical server response might look like:
HTTP/1.1 200 OK
Date: Wed, 13 Mar 2023 12:00:00 GMT
Server: Apache/2.4.1 (Unix)
Content-Type: text/html; charset=UTF-8
Content-Length: 1234
Implementing the HEAD Method in Java
Java provides robust libraries that simplify working with HTTP requests. Below, we will use HttpURLConnection to implement a simple HEAD request.
Sample Java Code
import java.io.IOException;
import java.net.HttpURLConnection;
import java.net.URL;
public class HeadRequestExample {
public static void main(String[] args) {
String urlString = "http://www.example.com";
try {
// Create a URL object
URL url = new URL(urlString);
// Open a connection
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
// Set the request method to HEAD
connection.setRequestMethod("HEAD");
// Connect to the server
connection.connect();
// Get the response code
int responseCode = connection.getResponseCode();
System.out.println("Response Code: " + responseCode);
// Display the headers
System.out.println("Headers:");
connection.getHeaderFields().forEach((key, value) -> {
System.out.println(key + " : " + value);
});
} catch (IOException e) {
e.printStackTrace();
}
}
}
Code Commentary
In this code snippet:
- We create a
URL
object pointing to the desired resource. - We open a connection through
HttpURLConnection
and set the request method to HEAD. - On connecting, we retrieve and display the response code and header fields.
This code aids in understanding the server's response without downloading the entire resource. You may want to use this in contexts where checking the status of links or caching is essential.
Use Cases of the HEAD Method
1. Caching Management
When managing cached resources, the HEAD method can help determine if the cached content is still valid. Before downloading potentially large files, a client may issue a HEAD request to check if the Content-Length or Last-Modified headers indicate any change:
Long cachedContentLength = ...; // retrieved from cache
if (responseHeaderContentLength > cachedContentLength) {
// Download the new content
}
2. Automated Testing and Monitoring Tools
Automated monitoring tools can use the HEAD method to periodically check the status of resources. This is a common scenario in both performance testing and monitoring pipelines.
3. Pre-flight Validation for Downloads
In cases where download operations might incur significant resource costs, a HEAD request allows clients to verify resource availability and size before proceeding.
Performance Implications
Using the HEAD request can lead to improved performance in a few key ways:
-
Lower Latency: As a HEAD request typically requires less data to be transferred, it can result in faster responses in scenarios like health checks or status checks.
-
Resource Optimization: In high-traffic environments, reducing the amount of data transferred can lead to better use of available bandwidth.
-
Less Server Load: Servers can handle more HEAD requests concurrently compared to GET requests, as they are computationally lighter.
Closing the Chapter
The HEAD operation, while often overlooked, can significantly enhance the efficiency of server-client communication. By using it wisely in Java applications, developers can create applications that not only perform well but also utilize bandwidth and resources effectively.
For further reading on HTTP methods and their implementations, consider these resources:
Engaging with the HEAD method opens the door to more efficient web applications that resonate better with modern performance expectations. Embrace the HEAD approach and maximize your application’s efficiency today!
Checkout our other articles