Overcoming Java Classloader Issues in Hot Swapping
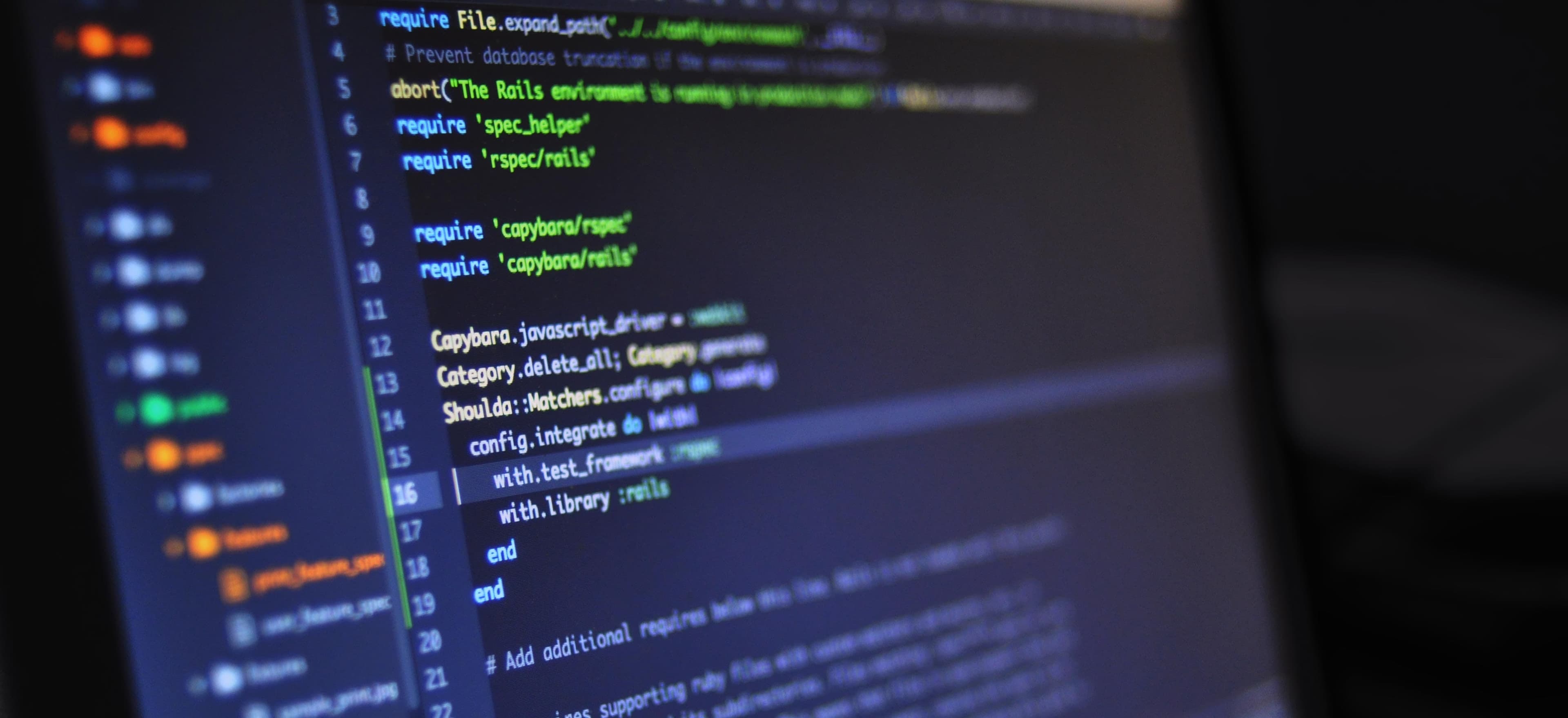
- Published on
Overcoming Java Classloader Issues in Hot Swapping
Java is renowned for its portability, performance, and robustness. But with great power comes great complexity. One area where developers frequently encounter difficulties is the classloading mechanism in Java, particularly during hot swapping. This blog post aims to explore these challenges and provide effective strategies to overcome them.
Understanding Classloaders in Java
Before diving into the challenges of hot swapping, it is essential to have a good grasp of what classloaders are.
Java's Classloader is a part of the Java Runtime Environment (JRE) that is responsible for loading classes into memory. It follows a hierarchical structure, which can be broken down into:
- Bootstrap Classloader: This is the parent of all classloaders and is part of the core Java framework. It loads the core Java libraries located in the JRE.
- Extension Classloader: This loads classes in the extension directories, such as those located in
$JAVA_HOME/lib/ext
. - Application Classloader: The default classloader that loads classes from the application's CLASSPATH.
Challenges with Classloaders
Every classloader instance generates a separate namespace. When a class is loaded by a specific classloader, that class cannot be seen by other classloaders, even if they share the same parent. This encapsulation can lead to issues when trying to replace classes while an application is running, also known as hot swapping.
What is Hot Swapping?
Hot swapping is a feature that allows developers to change class definitions while the application is running, without requiring a restart. This capability can lead to more efficient debugging and faster development cycles. However, even though it sounds appealing, the Java Classloader can introduce various complications.
Common Issues Faced During Hot Swapping
- Class Version Conflicts: When a class is redefined and an object of the previous version exists, this can lead to inconsistent behavior.
- Resource Leaks: Old class definitions may hold onto resources that are not properly cleaned up during the hot swap.
- ClassLoader Isolation: If you use multiple classloaders, you can end up getting ClassCastExceptions, as the classes are considered incompatible.
Strategies to Overcome Classloader Issues
1. Use a Development-Friendly Classloader
One way to mitigate classloading issues during hot swapping is to use a development-friendly classloader. Tools such as JRebel allow developers to modify source code and immediately see the impact in real-time without needing to restart the application.
Example:
Using JRebel, you can modify your Java class like this:
public class ExampleService {
public String greet() {
return "Hello, World!";
}
}
After changing the greet
method to:
public String greet() {
return "Hello, JRebel!";
}
A simple save action triggers JRebel to update the running application. This ensures a fast feedback loop without the repetitive cycle of restart and redeploy.
2. Utilize OSGi Framework
The OSGi (Open Service Gateway Initiative) framework provides a dynamic module system for Java. It allows you to manage bundles (plugins) that can be loaded, started, stopped, and updated without requiring a restart.
In OSGi, each bundle has its own classloader, which helps scope the classes it loads. This means you can have multiple versions of the same class across different bundles, significantly reducing classloader clashes.
3. Reflection and Dynamic Proxies
Consider utilizing Java Reflection and dynamic proxies for better class interoperability. With reflection, you can invoke class methods dynamically, allowing you to handle different versions of classes gracefully.
Here’s an example of using reflection:
public void invokeMethod(Object obj, String methodName) throws Exception {
Method method = obj.getClass().getMethod(methodName);
method.invoke(obj);
}
This can be particularly useful when you’re aware that a class may be reloaded during development. Using reflection helps avoid direct dependencies on class versions.
4. Clean Up Old Objects
When updating classes, it’s also crucial to null out references of old class versions. This is essential in avoiding resource leaks. You can implement a strategy to clean up before updating:
public void shutdownOldVersion(Object oldVersion) {
oldVersion = null; // Clear reference to help garbage collector
}
5. Monitor and Debug ClassLoader Behavior
Sometimes the best approach is to gain an understanding of what’s going wrong. Tools like VisualVM and Java's built-in JMX beans allow you to inspect the classloader details at runtime. Monitoring loaded classes can reveal issues like duplicate class definitions or memory leaks.
My Closing Thoughts on the Matter
While Java's classloading mechanism provides a powerful way to manage your application’s classes, it can also complicate hot swapping. Nevertheless, by using development-friendly frameworks, understanding OSGi, leveraging reflection, performing cleanup with old objects, and actively monitoring classloader behavior, you can thrive in a hot-swapping environment.
Hot swapping is an indispensable asset in your development toolbox. It speeds up the development cycle and helps provide quicker feedback loops. Embrace it with a thorough understanding of classloaders!
Further Reading
For in-depth details, these resources may be beneficial:
- Understanding Java Classloaders
- JRebel Official Documentation
Ensure to regularly check out industry best practices for using these advanced features effectively. Happy coding!
Checkout our other articles