Code Coverage vs. Test Coverage: Which Offers Real Insights?
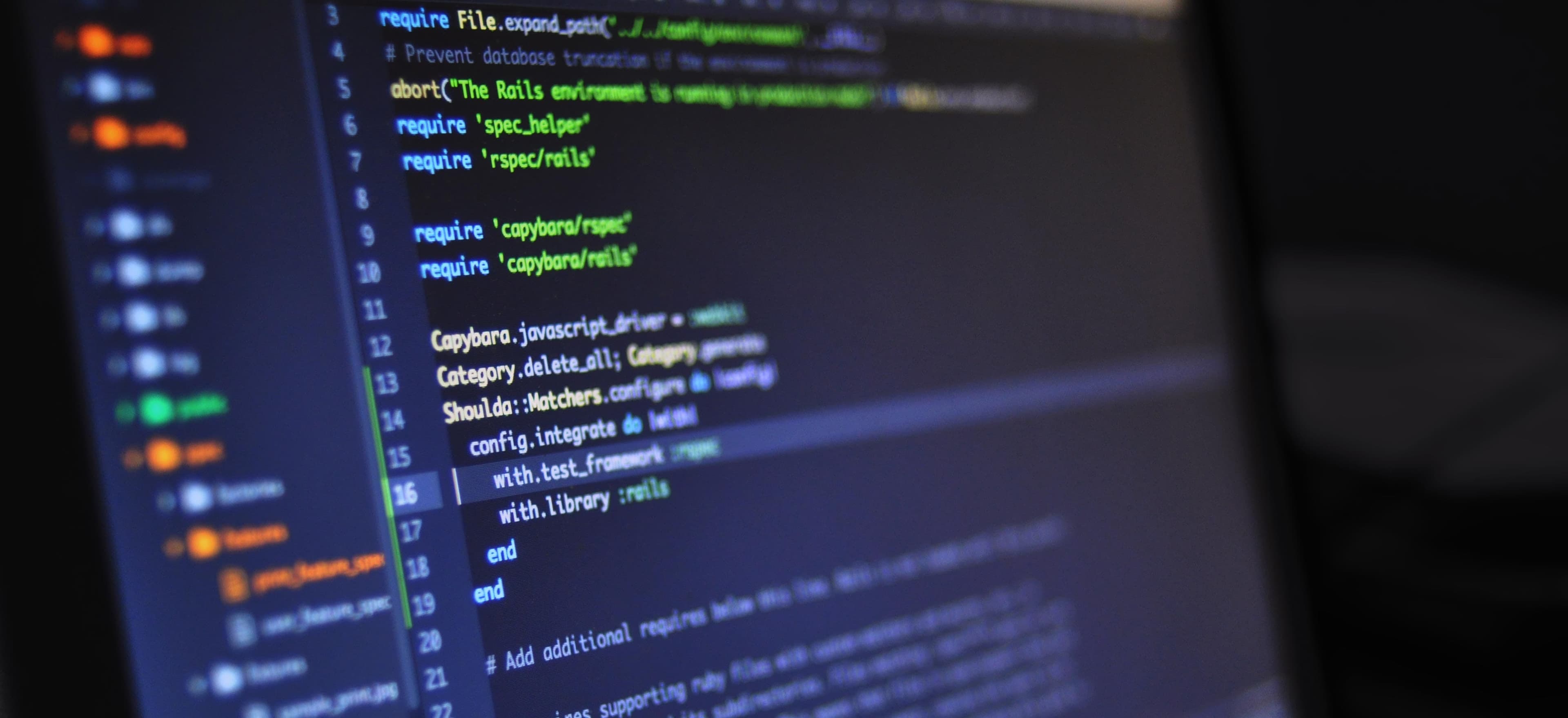
- Published on
Code Coverage vs. Test Coverage: Which Offers Real Insights?
When it comes to software development, quality assurance is a critical aspect that cannot be overlooked. Among the many tools and methodologies in a developer's toolkit, two concepts that often arise in discussions about testing are code coverage and test coverage. While they sound similar, they serve different purposes and provide unique insights into your application’s quality. In this post, we will demystify these concepts, explore their significance, and discuss which offers real insights for developers and teams.
What is Code Coverage?
Code coverage is a measure of how much of your source code is executed during testing. This metric gives you an idea of how many lines, branches, or functions of your codebase are tested at least once. It is often expressed as a percentage — e.g., 75% code coverage means that 75% of your code has been executed during tests.
Types of Code Coverage
- Line Coverage: Measures the number of executed lines of code.
- Branch Coverage: Checks whether all possible branches (if statements, loops) are executed.
- Function Coverage: Evaluates how many functions were called.
Importance of Code Coverage
- Identifying Dead Code: Code coverage helps identify sections of the codebase that are not utilized or tested, which may be candidates for future refactoring.
- Improving Test Quality: High code coverage typically indicates a more thoroughly tested application.
- Facilitating Debugging: When errors arise, knowing which parts of the code were exercised can focus your debugging efforts where they are needed most.
Code Coverage Example
Here's a simple Java example that demonstrates line coverage using JUnit and JaCoCo, a popular Java code coverage tool.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
}
The corresponding test class might look like this:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTest {
private final Calculator calculator = new Calculator();
@Test
public void testAdd() {
assertEquals(5, calculator.add(2, 3));
}
}
Why Use Code Coverage?
Using tools like JaCoCo, we can measure how much of the Calculator
class has been tested. In this example, we've achieved 100% line coverage for the add
method, but the subtract
method remains untested. This highlights the importance of writing comprehensive tests.
What is Test Coverage?
On the other hand, test coverage refers to a broader concept. It encompasses the aspects of the application that are verified through tests, including not only the code that has been executed but also the various conditions, scenarios, and edge cases that a piece of functionality may encounter.
Importance of Test Coverage
- Holistic View of Quality: While code coverage focuses on lines of code, test coverage provides insight into the user experience, business scenarios, and edge cases.
- Risk Mitigation: Understanding test coverage helps teams identify untested areas that could lead to bugs in production.
- Feedback Loop: Ensures continuous learning and improvement in testing strategies.
Test Coverage Example
Here’s an expanded Java example that demonstrates better test coverage by capturing different scenarios.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int subtract(int a, int b) {
return a - b;
}
}
And the improved test class:
import static org.junit.Assert.assertEquals;
import org.junit.Test;
public class CalculatorTest {
private final Calculator calculator = new Calculator();
@Test
public void testAdd() {
assertEquals(5, calculator.add(2, 3)); // Normal Case
assertEquals(0, calculator.add(0, 0)); // Edge Case
assertEquals(-1, calculator.add(-1, 0)); // Negative Case
}
@Test
public void testSubtract() {
assertEquals(1, calculator.subtract(3, 2)); // Normal Case
assertEquals(-2, calculator.subtract(0, 2)); // Edge Case
assertEquals(2, calculator.subtract(0, -2)); // Negative Case
}
}
Which is More Valuable?
So the question arises: which one offers real insights?
-
Code Coverage is valuable for confirming that your tests are executing a significant portion of your code. However, simply achieving high code coverage does not equate to high-quality tests. You might achieve 90% line coverage while still missing critical edge cases or user scenarios.
-
Test Coverage, while possibly less quantifiable, provides more meaningful insight into the quality and reliability of your application. It emphasizes the different paths through code that a user might take or various inputs they might provide.
Combining Both for Optimal Quality
In an ideal testing strategy, both code coverage and test coverage should be utilized in tandem.
- Use code coverage tools to ensure that adequate parts of the codebase are being executed during tests.
- Meanwhile, maintain a test coverage approach that considers testing all possible scenarios, including edge cases, user behaviors, and integration points with other systems.
Here are some tools that can assist in enhancing both code and test coverage in Java:
- JaCoCo: A popular tool for Java that provides line and branch coverage metrics.
- JUnit: The de facto testing framework for Java, allowing for straightforward unit tests.
- Mockito: For unit testing, making it easier to mock dependencies to ensure each unit of code behaves as expected.
The Last Word
In the end, both code coverage and test coverage are important metrics in the realm of software quality assurance. While code coverage can guide you on the amount of code exercised during testing, true insights come from understanding what scenarios and user experiences have been validated via test coverage.
By using both metrics wisely, along with comprehensive testing practices, you position your software for success not just in functionality but in quality and performance as well. The combination of these approaches will lead to more robust applications, minimizing bugs in production, and creating a better experience for users.
For further reading, check out Understanding Code Coverage, which dives deeper into real-world applications and practices.
Final Thoughts
Adopting a holistic perspective towards your testing strategies is key to sustainable software development. Prioritize quality, and you will build resilient applications that meet user needs effectively and efficiently. Happy coding!